Pattern Recognition: The Key to Solving Coding Problems on First Sight
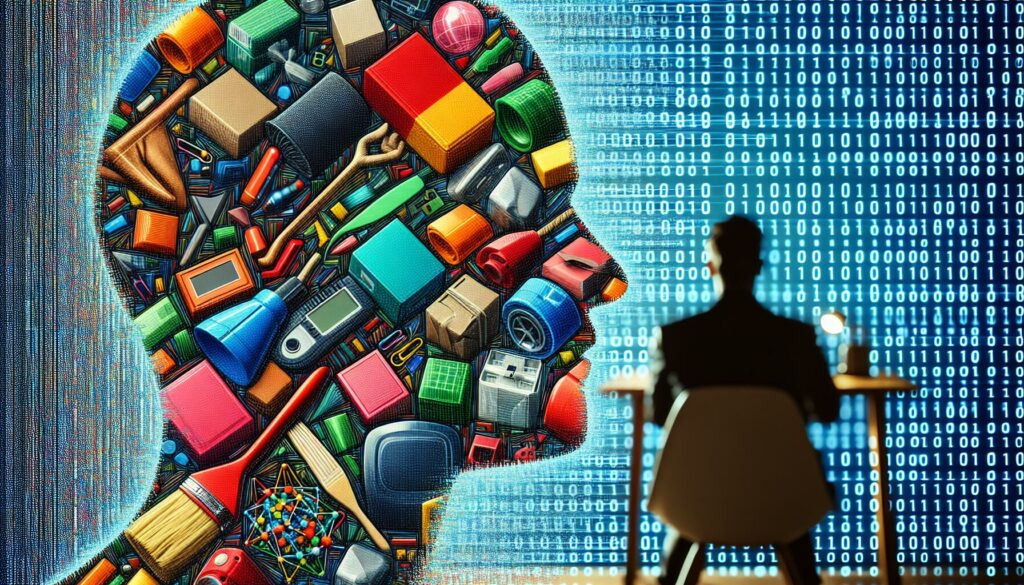
In the world of programming, the ability to quickly recognize patterns and apply them to solve complex problems is a highly valued skill. Pattern recognition is not just about memorizing solutions; it’s about understanding the underlying structures and principles that govern various types of problems. This skill allows developers to approach new challenges with confidence and efficiency, often solving coding problems on first sight.
Why Pattern Recognition Matters in Coding
Pattern recognition in coding is crucial for several reasons:
- Efficiency: Recognizing patterns allows you to solve problems faster.
- Problem-solving: It helps in breaking down complex problems into manageable parts.
- Code optimization: Understanding patterns leads to writing more efficient and elegant code.
- Learning and growth: It accelerates your learning curve and enhances your problem-solving skills.
Common Coding Patterns
Let’s explore some of the most common coding patterns that you’ll encounter in your programming journey:
1. Two-Pointer Technique
The two-pointer technique is a simple and effective way to solve problems that involve searching, reversing, or manipulating arrays or linked lists. It involves using two pointers that either move towards each other or in the same direction at different speeds.
Example problem: Reverse a string in-place.
def reverse_string(s):
left, right = 0, len(s) - 1
while left < right:
s[left], s[right] = s[right], s[left]
left += 1
right -= 1
return s
# Usage
print(reverse_string(['h', 'e', 'l', 'l', 'o'])) # Output: ['o', 'l', 'l', 'e', 'h']
2. Sliding Window
The sliding window technique is used to perform operations on a specific window size of an array or string. It’s particularly useful for problems involving subarrays or substrings.
Example problem: Find the maximum sum subarray of size k.
def max_sum_subarray(arr, k):
n = len(arr)
if n < k:
return None
window_sum = sum(arr[:k])
max_sum = window_sum
for i in range(n - k):
window_sum = window_sum - arr[i] + arr[i + k]
max_sum = max(max_sum, window_sum)
return max_sum
# Usage
print(max_sum_subarray([1, 4, 2, 10, 23, 3, 1, 0, 20], 4)) # Output: 39
3. Fast and Slow Pointers (Floyd’s Cycle-Finding Algorithm)
This pattern is often used to detect cycles in linked lists or arrays. It uses two pointers moving at different speeds to eventually meet if a cycle exists.
Example problem: Detect a cycle in a linked list.
class ListNode:
def __init__(self, x):
self.val = x
self.next = None
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
# Usage
# Create a linked list with a cycle
head = ListNode(3)
head.next = ListNode(2)
head.next.next = ListNode(0)
head.next.next.next = ListNode(-4)
head.next.next.next.next = head.next # Create cycle
print(has_cycle(head)) # Output: True
4. Merge Intervals
This pattern is used to deal with overlapping intervals. It’s commonly applied in scheduling problems or when you need to merge overlapping time periods.
Example problem: Merge overlapping intervals.
def merge_intervals(intervals):
if not intervals:
return []
# Sort intervals based on start time
intervals.sort(key=lambda x: x[0])
merged = [intervals[0]]
for interval in intervals[1:]:
# If current interval overlaps with the previous, merge them
if interval[0] <= merged[-1][1]:
merged[-1][1] = max(merged[-1][1], interval[1])
else:
merged.append(interval)
return merged
# Usage
intervals = [[1,3],[2,6],[8,10],[15,18]]
print(merge_intervals(intervals)) # Output: [[1,6],[8,10],[15,18]]
5. Binary Search
Binary search is a highly efficient search algorithm used on sorted arrays. It repeatedly divides the search interval in half, significantly reducing the search space.
Example problem: Find the index of a target value in a sorted array.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1 # Target not found
# Usage
arr = [1, 3, 5, 7, 9, 11, 13, 15]
target = 7
print(binary_search(arr, target)) # Output: 3
6. Dynamic Programming
Dynamic programming is a method for solving complex problems by breaking them down into simpler subproblems. It’s often used for optimization problems and can significantly improve the time complexity of naive recursive solutions.
Example problem: Calculate the nth Fibonacci number.
def fibonacci(n):
if n <= 1:
return n
dp = [0] * (n + 1)
dp[1] = 1
for i in range(2, n + 1):
dp[i] = dp[i-1] + dp[i-2]
return dp[n]
# Usage
print(fibonacci(10)) # Output: 55
7. Depth-First Search (DFS)
DFS is a graph traversal algorithm that explores as far as possible along each branch before backtracking. It’s commonly used for problems involving trees, graphs, and maze-like structures.
Example problem: Implement DFS for a binary tree.
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def dfs(root):
if not root:
return
print(root.val, end=' ') # Process current node
dfs(root.left) # Traverse left subtree
dfs(root.right) # Traverse right subtree
# Usage
root = TreeNode(1)
root.left = TreeNode(2)
root.right = TreeNode(3)
root.left.left = TreeNode(4)
root.left.right = TreeNode(5)
dfs(root) # Output: 1 2 4 5 3
8. Breadth-First Search (BFS)
BFS is another graph traversal algorithm that explores all the vertices of a graph in breadth-first order. It’s often used for finding the shortest path in unweighted graphs and for level-order traversals.
Example problem: Implement level-order traversal of a binary tree.
from collections import deque
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def level_order_traversal(root):
if not root:
return []
result = []
queue = deque([root])
while queue:
level_size = len(queue)
current_level = []
for _ in range(level_size):
node = queue.popleft()
current_level.append(node.val)
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
result.append(current_level)
return result
# Usage
root = TreeNode(3)
root.left = TreeNode(9)
root.right = TreeNode(20)
root.right.left = TreeNode(15)
root.right.right = TreeNode(7)
print(level_order_traversal(root)) # Output: [[3], [9, 20], [15, 7]]
Developing Pattern Recognition Skills
Improving your pattern recognition skills in coding is a gradual process that requires practice and exposure to various problems. Here are some strategies to enhance your abilities:
1. Solve Diverse Problems
Expose yourself to a wide range of coding problems. Platforms like LeetCode, HackerRank, and CodeSignal offer a variety of problems categorized by difficulty and topic.
2. Analyze Solutions
After solving a problem, take time to study different solutions. Understanding various approaches to the same problem will help you recognize patterns more easily in the future.
3. Categorize Problems
As you solve problems, try to categorize them based on the underlying patterns or techniques used. This mental organization will help you quickly identify similar problems in the future.
4. Practice Regularly
Consistent practice is key to improving pattern recognition. Set aside time regularly to solve coding problems, even if it’s just for a short period each day.
5. Review and Reflect
Periodically review the problems you’ve solved and reflect on the patterns you’ve encountered. This reinforcement will help solidify your understanding and recognition of these patterns.
6. Teach Others
Explaining problem-solving techniques and patterns to others can deepen your own understanding and help you articulate the underlying principles more clearly.
Advanced Pattern Recognition Techniques
As you become more proficient in recognizing basic patterns, you can start to explore more advanced techniques:
1. Combining Patterns
Many complex problems require the application of multiple patterns. Learning to recognize when and how to combine different patterns is a valuable skill.
2. Pattern Adaptation
Sometimes, a problem may not exactly match a known pattern but can be solved by adapting or modifying an existing pattern. Developing this flexibility in your thinking is crucial for tackling novel problems.
3. Creating New Patterns
As you gain experience, you may start to recognize new patterns that aren’t commonly discussed. Don’t be afraid to create and document your own patterns based on your observations.
4. Time and Space Complexity Analysis
Understanding the time and space complexity of different patterns helps in choosing the most efficient solution for a given problem. Practice analyzing the complexity of various algorithms and patterns.
Common Pitfalls in Pattern Recognition
While developing your pattern recognition skills, be aware of these common pitfalls:
1. Over-reliance on Memorization
Memorizing solutions without understanding the underlying principles can lead to difficulties when faced with slightly modified problems. Focus on understanding the why and how of each pattern.
2. Forcing Patterns
Not every problem fits neatly into a known pattern. Be cautious about forcing a familiar pattern onto a problem where it doesn’t apply. Sometimes, a unique approach is required.
3. Neglecting Edge Cases
When applying a pattern, don’t forget to consider edge cases. Many patterns have specific conditions or assumptions that need to be checked.
4. Ignoring Problem Constraints
Always pay attention to the specific constraints of each problem. A pattern that works in general may not be suitable if it violates time or space complexity requirements.
Conclusion
Pattern recognition is a powerful skill that can significantly enhance your problem-solving abilities in coding. By familiarizing yourself with common patterns, practicing regularly, and developing a systematic approach to problem analysis, you can train your mind to quickly identify and apply the most appropriate solutions.
Remember that becoming proficient in pattern recognition is a journey that requires patience and persistence. As you continue to practice and expand your knowledge, you’ll find yourself solving increasingly complex problems with greater ease and confidence. The ability to recognize patterns and apply them effectively is what often distinguishes experienced developers from novices.
Keep challenging yourself with diverse problems, stay curious about different approaches, and don’t be discouraged by difficulties. With time and practice, you’ll develop the intuition to solve many coding problems on first sight, making you a more efficient and valuable programmer.