Palindrome Partitioning: A Comprehensive Guide to Solving this Classic Algorithm Problem
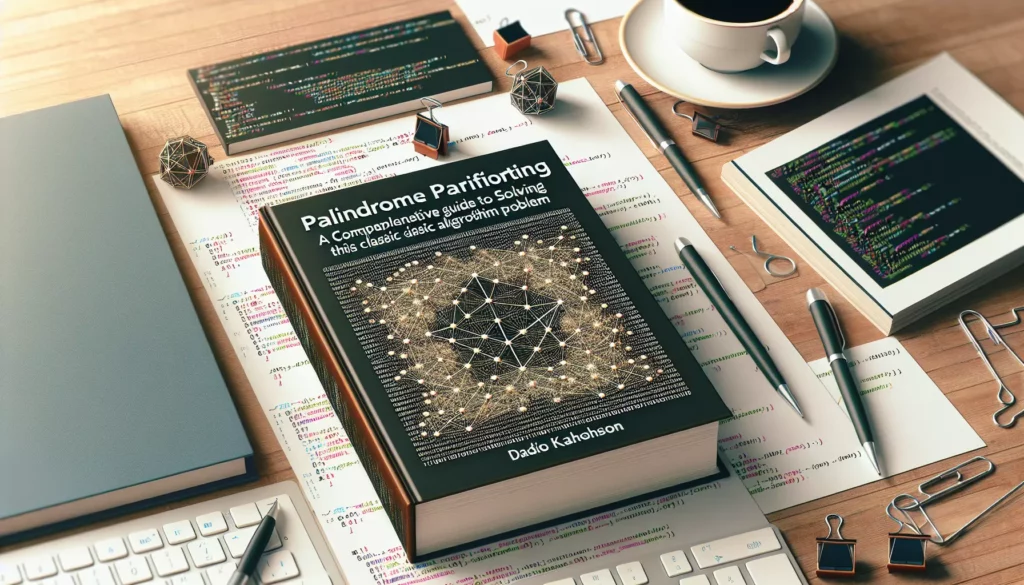
Welcome to our in-depth exploration of the Palindrome Partitioning problem, a classic algorithm challenge that often appears in technical interviews and coding competitions. This problem is an excellent example of how string manipulation, dynamic programming, and backtracking can come together to create an efficient solution. In this article, we’ll break down the problem, explore different approaches, and provide step-by-step implementations to help you master this important concept.
What is Palindrome Partitioning?
Before we dive into the problem itself, let’s refresh our understanding of palindromes. A palindrome is a word, phrase, number, or other sequence of characters that reads the same forward and backward. For example, “racecar” and “A man, a plan, a canal: Panama” are palindromes.
The Palindrome Partitioning problem asks us to find all possible ways to partition a given string into a sequence of palindromic substrings. In other words, we need to split the string into substrings such that each substring is a palindrome.
For example, given the string “aab”, the possible palindrome partitions are:
- [“a”, “a”, “b”]
- [“aa”, “b”]
This problem is particularly interesting because it combines several important concepts in computer science and algorithm design, making it a favorite among interviewers at top tech companies.
Approaching the Problem
There are several ways to approach the Palindrome Partitioning problem, each with its own trade-offs in terms of time and space complexity. We’ll explore three main approaches:
- Brute Force Approach
- Backtracking Approach
- Dynamic Programming Optimization
Let’s dive into each of these approaches in detail.
1. Brute Force Approach
The brute force approach is the most straightforward way to solve the problem. It involves generating all possible partitions of the string and then checking if each partition consists only of palindromes. While this method is simple to understand and implement, it’s not efficient for longer strings due to its high time complexity.
Here’s a step-by-step breakdown of the brute force approach:
- Generate all possible partitions of the input string.
- For each partition, check if all substrings are palindromes.
- If a partition consists only of palindromes, add it to the result list.
- Return the result list containing all valid palindrome partitions.
Let’s implement this approach in Python:
def partition(s):
def is_palindrome(substr):
return substr == substr[::-1]
def generate_partitions(s, start, partition, result):
if start == len(s):
result.append(partition[:])
return
for i in range(start, len(s)):
substr = s[start:i+1]
if is_palindrome(substr):
partition.append(substr)
generate_partitions(s, i+1, partition, result)
partition.pop()
result = []
generate_partitions(s, 0, [], result)
return result
# Example usage
s = "aab"
print(partition(s))
# Output: [['a', 'a', 'b'], ['aa', 'b']]
This implementation uses recursion to generate all possible partitions and checks each substring for palindrome property. While it works correctly, it has a time complexity of O(n * 2^n), where n is the length of the input string. This makes it impractical for longer strings.
2. Backtracking Approach
The backtracking approach is an improvement over the brute force method. It uses the same general idea but optimizes the process by avoiding unnecessary checks and backtracking when a partition is found to be invalid.
Here’s how the backtracking approach works:
- Start with an empty result list and an empty current partition.
- For each character in the string, extend the current substring.
- If the current substring is a palindrome, add it to the current partition and recursively process the rest of the string.
- If we reach the end of the string and all substrings in the current partition are palindromes, add the partition to the result list.
- Backtrack by removing the last added substring from the current partition and continue with the next character.
Let’s implement this approach in Python:
def partition(s):
def is_palindrome(start, end):
while start < end:
if s[start] != s[end]:
return False
start += 1
end -= 1
return True
def backtrack(start, partition):
if start == len(s):
result.append(partition[:])
return
for end in range(start, len(s)):
if is_palindrome(start, end):
partition.append(s[start:end+1])
backtrack(end + 1, partition)
partition.pop()
result = []
backtrack(0, [])
return result
# Example usage
s = "aab"
print(partition(s))
# Output: [['a', 'a', 'b'], ['aa', 'b']]
This backtracking approach is more efficient than the brute force method because it avoids generating invalid partitions. However, its time complexity is still O(n * 2^n) in the worst case, where n is the length of the input string. The space complexity is O(n) for the recursion stack.
3. Dynamic Programming Optimization
To further optimize our solution, we can use dynamic programming to precompute palindrome information. This approach significantly reduces the time complexity by avoiding redundant palindrome checks.
Here’s how we can implement the dynamic programming optimization:
- Create a 2D boolean array
dp
wheredp[i][j]
is true if the substrings[i:j+1]
is a palindrome. - Fill the
dp
array using a bottom-up approach. - Use the precomputed palindrome information in the backtracking process.
Let’s implement this optimized approach in Python:
def partition(s):
n = len(s)
dp = [[False] * n for _ in range(n)]
# Precompute palindrome information
for i in range(n - 1, -1, -1):
for j in range(i, n):
if s[i] == s[j] and (j - i <= 2 or dp[i + 1][j - 1]):
dp[i][j] = True
def backtrack(start, partition):
if start == n:
result.append(partition[:])
return
for end in range(start, n):
if dp[start][end]:
partition.append(s[start:end+1])
backtrack(end + 1, partition)
partition.pop()
result = []
backtrack(0, [])
return result
# Example usage
s = "aab"
print(partition(s))
# Output: [['a', 'a', 'b'], ['aa', 'b']]
This dynamic programming optimization reduces the time complexity to O(n^2) for palindrome precomputation and O(2^n) for generating all partitions. The space complexity is O(n^2) for the dp
array and O(n) for the recursion stack.
Time and Space Complexity Analysis
Let’s compare the time and space complexities of the three approaches we’ve discussed:
Approach | Time Complexity | Space Complexity |
---|---|---|
Brute Force | O(n * 2^n) | O(n) |
Backtracking | O(n * 2^n) | O(n) |
Dynamic Programming | O(n^2) + O(2^n) | O(n^2) |
While the dynamic programming approach has a higher space complexity due to the dp
array, it significantly reduces the time complexity for palindrome checks, making it the most efficient solution for larger inputs.
Practical Applications and Variations
The Palindrome Partitioning problem and its variations have several practical applications in computer science and beyond:
- Text Processing: In natural language processing, palindrome partitioning can be used to identify meaningful palindromic phrases or sentences within a larger text.
- Bioinformatics: In DNA sequence analysis, palindromic sequences play a crucial role. Efficient palindrome partitioning algorithms can help identify these important structures.
- Cryptography: Some encryption techniques use palindromes as part of their algorithms. Understanding palindrome partitioning can be useful in cryptanalysis.
- Puzzle Solving: Many word puzzles and games involve finding palindromes or palindromic structures, making this algorithm relevant for game development and AI.
Variations of the Palindrome Partitioning problem include:
- Palindrome Partitioning II: Find the minimum number of cuts needed to partition a string into palindromic substrings.
- Longest Palindromic Subsequence: Find the length of the longest subsequence in a string that is also a palindrome.
- Palindrome Pairs: Given a list of words, find all pairs of distinct indices (i, j) such that the concatenation of the two words is a palindrome.
Interview Tips and Tricks
If you encounter the Palindrome Partitioning problem or a similar challenge in a technical interview, keep these tips in mind:
- Clarify the Problem: Make sure you understand the input constraints and expected output format. Ask about edge cases, such as empty strings or strings with only one character.
- Start Simple: Begin with a brute force approach to demonstrate your problem-solving skills. Then, discuss potential optimizations.
- Optimize Step-by-Step: Explain how you can improve the solution using techniques like backtracking and dynamic programming. This shows your ability to analyze and optimize algorithms.
- Consider Space-Time Trade-offs: Discuss the trade-offs between time and space complexity for different approaches. This demonstrates your understanding of algorithm efficiency.
- Test Your Solution: Write test cases to cover various scenarios, including edge cases. This shows attention to detail and thoroughness.
- Analyze Complexity: Provide a clear explanation of the time and space complexity of your final solution.
- Discuss Real-world Applications: If time allows, mention some practical applications of palindrome partitioning to showcase your broader understanding of computer science concepts.
Conclusion
The Palindrome Partitioning problem is a fascinating algorithm challenge that combines string manipulation, recursion, backtracking, and dynamic programming. By understanding and implementing different approaches to this problem, you’ll gain valuable insights into algorithm design and optimization techniques.
As you continue your journey in coding education and programming skills development, remember that problems like Palindrome Partitioning are excellent opportunities to improve your algorithmic thinking and problem-solving abilities. Practice implementing these solutions, experiment with variations, and don’t hesitate to explore more advanced topics in string algorithms and dynamic programming.
Keep coding, keep learning, and best of luck in your technical interviews and coding competitions!