Palantir Technical Interview Prep: A Comprehensive Guide
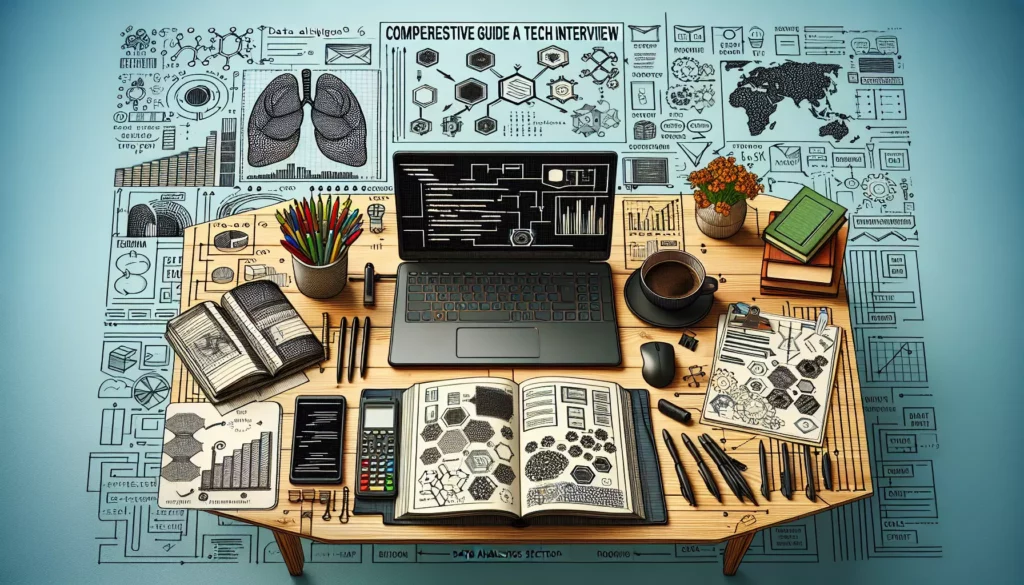
Preparing for a technical interview at Palantir can be a daunting task, but with the right approach and resources, you can significantly increase your chances of success. In this comprehensive guide, we’ll walk you through everything you need to know to ace your Palantir technical interview, from understanding the company’s unique interview process to mastering the key skills and knowledge areas they prioritize.
Table of Contents
- Understanding Palantir
- The Palantir Interview Process
- Key Technical Skills to Master
- Common Coding Challenges and How to Approach Them
- System Design and Architecture
- Behavioral Questions and Company Culture
- Effective Preparation Strategies
- What to Expect on Interview Day
- Post-Interview Follow-up and Next Steps
- Additional Resources for Palantir Interview Prep
1. Understanding Palantir
Before diving into the technical aspects of the interview, it’s crucial to understand what Palantir does and why they’re unique in the tech industry. Palantir Technologies is a software company that specializes in big data analytics. They work with government agencies, financial institutions, and other organizations to help them make sense of large, complex datasets.
Key points to know about Palantir:
- Founded in 2003 by Peter Thiel, Nathan Gettings, Joe Lonsdale, Stephen Cohen, and Alex Karp
- Focuses on data integration, information management, and quantitative analytics
- Known for their two main software platforms: Palantir Gotham and Palantir Foundry
- Works on high-stakes, mission-critical projects often involving national security and financial crimes
- Values problem-solving skills, analytical thinking, and the ability to work with complex systems
Understanding Palantir’s mission and values will not only help you during the interview process but also allow you to assess if the company aligns with your career goals and interests.
2. The Palantir Interview Process
Palantir’s interview process is known for being rigorous and comprehensive. It typically consists of several stages:
- Resume Screening: Your application and resume are reviewed by the hiring team.
- Phone Screen: A brief call with a recruiter to discuss your background and interest in Palantir.
- Technical Phone Interview: A 45-60 minute interview focusing on coding and problem-solving skills.
- On-site Interviews: If you pass the previous stages, you’ll be invited for a series of on-site interviews, which typically include:
- Coding interviews
- System design interviews
- Behavioral interviews
- Decomposition interview (unique to Palantir)
- Final Decision: After the on-site interviews, the hiring committee will make a decision.
The entire process can take several weeks to a couple of months, depending on the position and the company’s hiring needs.
3. Key Technical Skills to Master
To succeed in a Palantir technical interview, you should be well-versed in the following areas:
Data Structures and Algorithms
This is the foundation of any technical interview. Make sure you’re comfortable with:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
- Dynamic Programming
- Sorting and Searching algorithms
Programming Languages
While Palantir doesn’t require expertise in any specific language, it’s best to be proficient in at least one of the following:
- Java
- Python
- C++
- JavaScript
Database Knowledge
Given Palantir’s focus on data, understanding database concepts is crucial:
- SQL fundamentals
- Indexing and query optimization
- ACID properties
- NoSQL databases
Distributed Systems
Palantir deals with large-scale systems, so knowledge in this area is valuable:
- Consistency and consensus algorithms
- Distributed caching
- Load balancing
- Fault tolerance and disaster recovery
Software Design Patterns
Familiarity with common design patterns can demonstrate your ability to write clean, maintainable code:
- Singleton
- Factory
- Observer
- Strategy
- Decorator
4. Common Coding Challenges and How to Approach Them
Palantir’s coding interviews often focus on problem-solving skills and the ability to write clean, efficient code. Here are some types of questions you might encounter:
String Manipulation
Example: Implement a function to reverse words in a string while preserving the order of the words.
def reverse_words(s):
# Split the string into words
words = s.split()
# Reverse each word
reversed_words = [word[::-1] for word in words]
# Join the reversed words back into a string
return ' '.join(reversed_words)
# Test the function
print(reverse_words("Hello World")) # Output: "olleH dlroW"
Array Processing
Example: Find the maximum subarray sum in a given array of integers.
def max_subarray_sum(arr):
max_sum = current_sum = arr[0]
for num in arr[1:]:
current_sum = max(num, current_sum + num)
max_sum = max(max_sum, current_sum)
return max_sum
# Test the function
print(max_subarray_sum([-2, 1, -3, 4, -1, 2, 1, -5, 4])) # Output: 6
Graph Traversal
Example: Implement a function to find the shortest path between two nodes in an unweighted graph.
from collections import deque
def shortest_path(graph, start, end):
queue = deque([[start]])
visited = set([start])
while queue:
path = queue.popleft()
node = path[-1]
if node == end:
return path
for neighbor in graph[node]:
if neighbor not in visited:
visited.add(neighbor)
new_path = list(path)
new_path.append(neighbor)
queue.append(new_path)
return None
# Test the function
graph = {
'A': ['B', 'C'],
'B': ['A', 'D', 'E'],
'C': ['A', 'F'],
'D': ['B'],
'E': ['B', 'F'],
'F': ['C', 'E']
}
print(shortest_path(graph, 'A', 'F')) # Output: ['A', 'C', 'F']
Tips for Approaching Coding Challenges
- Clarify the problem: Ask questions to ensure you understand the requirements and constraints.
- Think out loud: Explain your thought process as you work through the problem.
- Start with a brute force solution: It’s okay to begin with a less efficient solution and optimize later.
- Test your code: Write test cases to verify your solution works correctly.
- Optimize: Look for ways to improve the time and space complexity of your solution.
- Handle edge cases: Consider and address potential edge cases in your implementation.
5. System Design and Architecture
System design interviews are a crucial part of the Palantir interview process, especially for more senior positions. These interviews assess your ability to design large-scale distributed systems. Here are some key areas to focus on:
Scalability
Understand how to design systems that can handle increasing loads:
- Horizontal vs. Vertical scaling
- Load balancing techniques
- Database sharding
- Caching strategies
Availability and Reliability
Know how to design systems that are highly available and can recover from failures:
- Redundancy and replication
- Fault tolerance mechanisms
- Disaster recovery planning
Data Storage
Understand different data storage solutions and when to use them:
- Relational vs. NoSQL databases
- Data warehousing
- Big data technologies (e.g., Hadoop, Spark)
API Design
Be able to design clean, efficient APIs:
- RESTful principles
- Authentication and authorization
- Rate limiting and throttling
Example System Design Question
“Design a system to store and query large amounts of time-series data, such as stock prices or sensor readings.”
Approach:
- Clarify requirements: Data volume, query patterns, latency requirements, etc.
- High-level design: Sketch out the main components (data ingestion, storage, query service)
- Data model: Decide on how to structure the time-series data (e.g., using a NoSQL database like Cassandra)
- Scalability: Discuss sharding strategies for distributing data across multiple nodes
- Query optimization: Explain how to handle different types of queries efficiently (e.g., using pre-aggregation for summary queries)
- Data retention: Discuss strategies for managing old data (e.g., downsampling, archiving)
6. Behavioral Questions and Company Culture
While technical skills are crucial, Palantir also places a high value on cultural fit and soft skills. Be prepared to answer behavioral questions that assess your problem-solving abilities, teamwork, and alignment with Palantir’s values.
Common Behavioral Questions
- “Tell me about a time when you had to solve a complex problem.”
- “How do you handle disagreements with team members?”
- “Describe a situation where you had to learn a new technology quickly.”
- “How do you prioritize tasks when working on multiple projects?”
- “Tell me about a time when you failed and what you learned from it.”
Palantir’s Core Values
Familiarize yourself with Palantir’s core values and be prepared to discuss how they align with your own:
- Make the right decision, not the easy one
- Optimize for the mission, not for ourselves
- Empower our partners
- Be radically honest and transparent
- Check your ego at the door
Tips for Behavioral Interviews
- Use the STAR method (Situation, Task, Action, Result) to structure your responses
- Provide specific examples from your past experiences
- Highlight your problem-solving skills and ability to work in teams
- Show enthusiasm for tackling complex challenges
- Demonstrate your ability to learn quickly and adapt to new situations
7. Effective Preparation Strategies
To maximize your chances of success in the Palantir interview process, consider the following preparation strategies:
Create a Study Plan
Develop a structured study plan that covers all the key areas:
- Allocate time for each topic (data structures, algorithms, system design, etc.)
- Set specific goals for each study session
- Track your progress and adjust your plan as needed
Practice Coding Regularly
Consistent practice is key to improving your coding skills:
- Solve problems on platforms like LeetCode, HackerRank, or CodeSignal
- Aim to solve at least 2-3 problems per day
- Focus on medium to hard difficulty problems
- Time yourself to simulate interview conditions
Mock Interviews
Conduct mock interviews to get comfortable with the interview format:
- Practice with friends or use platforms like Pramp or interviewing.io
- Get feedback on your communication and problem-solving approach
- Work on explaining your thought process clearly
Review Palantir-Specific Resources
Familiarize yourself with Palantir’s technology and interview process:
- Read Palantir’s engineering blog
- Watch tech talks and presentations by Palantir engineers
- Review interview experiences shared on platforms like Glassdoor or Blind
Brush Up on Computer Science Fundamentals
Ensure you have a solid understanding of core CS concepts:
- Review operating systems principles
- Understand networking basics
- Refresh your knowledge of database systems
8. What to Expect on Interview Day
Being well-prepared for the actual interview day can help you feel more confident and perform better. Here’s what you can expect:
Before the Interview
- Get a good night’s sleep and eat a healthy meal
- Dress professionally, even for virtual interviews
- Test your equipment if it’s a remote interview
- Review your resume and be prepared to discuss any project or experience listed
During the Interview
- Be punctual – arrive or log in 5-10 minutes early
- Bring a notebook and pen for taking notes
- Listen carefully to the questions and ask for clarification if needed
- Think out loud and communicate your thought process
- Stay calm and composed, even if you encounter difficult questions
The Decomposition Interview
This is a unique interview format used by Palantir. In this interview, you’ll be presented with a complex, open-ended problem and asked to break it down into smaller, manageable components. The key is to demonstrate your ability to:
- Analyze and structure ambiguous problems
- Identify key components and dependencies
- Prioritize tasks and create a plan of action
- Communicate your thoughts clearly and logically
After Each Interview
- Thank the interviewer for their time
- Ask thoughtful questions about the role or the company
- Request the interviewer’s contact information for follow-up
9. Post-Interview Follow-up and Next Steps
After completing your interviews, there are several steps you can take to leave a positive impression and increase your chances of success:
Send Thank-You Notes
- Email each interviewer within 24 hours of your interview
- Express your appreciation for their time and insights
- Reiterate your interest in the position and the company
- Briefly mention a specific point from your conversation to make the note more personal
Reflect on Your Performance
- Write down the questions you were asked while they’re fresh in your mind
- Analyze your responses and identify areas for improvement
- Consider any feedback you received during the interviews
Follow Up with the Recruiter
- If you haven’t heard back within the timeframe they provided, politely follow up
- Express your continued interest in the position
- Ask about the next steps in the process
Continue Your Preparation
- Keep practicing coding problems and system design
- Stay updated on Palantir’s latest news and developments
- Prepare for potential additional rounds or follow-up interviews
10. Additional Resources for Palantir Interview Prep
To further enhance your preparation for the Palantir technical interview, consider using these resources:
Books
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “System Design Interview” by Alex Xu
- “Designing Data-Intensive Applications” by Martin Kleppmann
Online Courses
- AlgoExpert – for algorithm and data structure practice
- Educative.io’s “Grokking the System Design Interview”
- MIT OpenCourseWare’s “Introduction to Algorithms”
Websites and Platforms
- LeetCode – for coding practice and interview preparation
- HackerRank – for coding challenges and contests
- GeeksforGeeks – for computer science theory and practice problems
Palantir-Specific Resources
- Palantir’s official website and careers page
- Palantir’s GitHub repositories
- Palantir’s engineering blog
YouTube Channels
- Back To Back SWE – for algorithm explanations
- System Design Interview – for system design concepts and examples
- Tech Dummies – for system design and architecture discussions
Remember, the key to success in the Palantir technical interview is not just about memorizing solutions or concepts, but truly understanding them and being able to apply that knowledge to solve complex problems. Use these resources to deepen your understanding and broaden your skill set.
With thorough preparation, a solid grasp of fundamental concepts, and a demonstration of your problem-solving abilities, you’ll be well-equipped to tackle the Palantir technical interview. Good luck with your preparation, and may you succeed in your journey to join one of the most innovative companies in the tech industry!