Pair Programming in Technical Interviews: A Comprehensive Guide
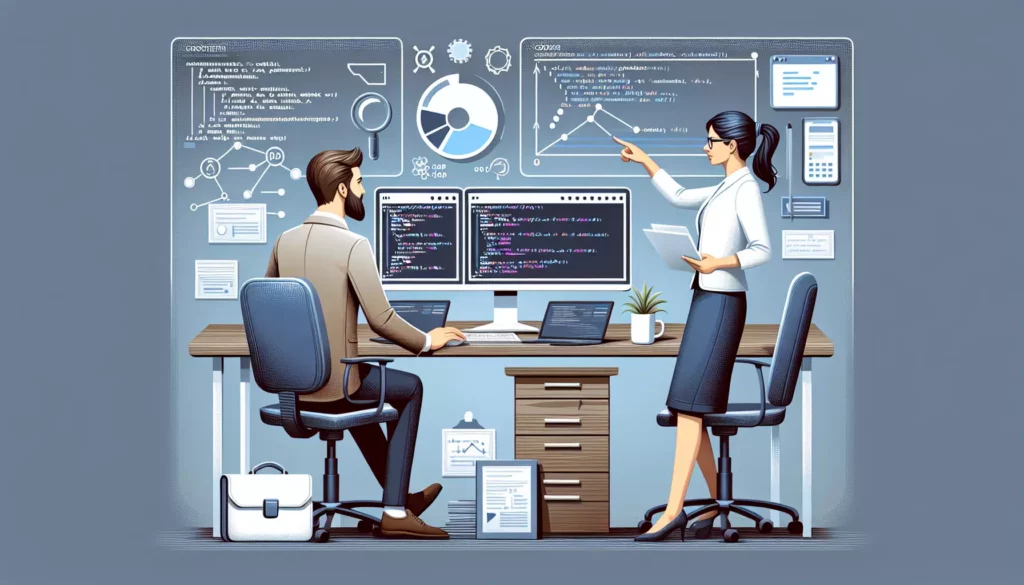
In the ever-evolving landscape of software development and technical interviews, pair programming has emerged as a powerful technique that both showcases a candidate’s skills and simulates real-world collaborative environments. This comprehensive guide will delve into the intricacies of pair programming, its application in interview settings, and how you can leverage this approach to excel in your next technical interview.
What is Pair Programming?
Pair programming is a software development technique where two programmers work together at a single workstation. One, the “driver,” writes code while the other, the “navigator,” reviews each line of code as it’s typed in. The two programmers switch roles frequently.
This collaborative approach offers several benefits:
- Improved code quality
- Better problem-solving
- Knowledge sharing
- Reduced errors
- Enhanced team communication
Pair Programming in Interview Settings
In recent years, many tech companies, including FAANG (Facebook, Amazon, Apple, Netflix, Google) and other major players, have incorporated pair programming into their interview processes. This shift reflects the industry’s growing emphasis on collaboration and real-world problem-solving skills.
During a pair programming interview, you might encounter the following scenarios:
- Collaborative Coding: You and the interviewer work together to solve a problem, taking turns as driver and navigator.
- Code Review: You review and improve existing code with the interviewer, discussing potential optimizations and best practices.
- Design Discussion: You collaborate on designing a system or feature, focusing on high-level architecture and problem-solving approaches.
Why Companies Use Pair Programming in Interviews
Companies employ pair programming in interviews for several reasons:
- Assessing Collaboration Skills: It allows interviewers to evaluate how well candidates work in a team setting.
- Simulating Real Work Environments: Many development teams use pair programming, making it an authentic test of a candidate’s abilities.
- Observing Problem-Solving Approaches: Interviewers can gain insights into a candidate’s thought process and problem-solving strategies.
- Evaluating Communication Skills: Clear communication is crucial in pair programming, making it an excellent test of a candidate’s ability to express ideas and discuss technical concepts.
- Reducing Interview Stress: The collaborative nature of pair programming can help candidates feel more at ease compared to traditional whiteboard interviews.
Preparing for a Pair Programming Interview
To excel in a pair programming interview, consider the following tips:
1. Practice Collaborative Coding
Engage in pair programming sessions with friends, colleagues, or online coding partners. Platforms like AlgoCademy offer interactive coding environments where you can practice collaborative problem-solving.
2. Enhance Your Communication Skills
Work on articulating your thoughts clearly and concisely. Practice explaining your code and reasoning to others, even when coding alone.
3. Familiarize Yourself with Common Tools
Learn to use collaborative coding tools like VS Code Live Share, CodePen, or Repl.it. Many companies use these or similar platforms during remote pair programming interviews.
4. Brush Up on Coding Best Practices
Review clean code principles, design patterns, and best practices for the programming language you’ll be using in the interview.
5. Develop Active Listening Skills
Practice active listening to ensure you fully understand the problem and your partner’s suggestions during the interview.
Common Pair Programming Interview Scenarios
Let’s explore some typical scenarios you might encounter in a pair programming interview, along with strategies to handle them effectively.
Scenario 1: Collaborative Problem Solving
In this scenario, you and the interviewer work together to solve a coding problem. Here’s an example of how it might unfold:
Interviewer: “Let’s work together to implement a function that finds the longest palindromic substring in a given string.”
You: “Certainly! Before we start coding, let’s discuss our approach. We could use a brute force method, checking every substring, or we could implement a more efficient algorithm like Manacher’s algorithm. Which approach would you prefer?”
Interviewer: “Let’s start with a simpler approach and optimize if time allows. How about we implement the brute force method first?”
You: “Sounds good. I’ll start by writing the function signature and we can build from there.”
def longest_palindrome(s: str) -> str:
if not s:
return ""
longest = s[0]
for i in range(len(s)):
for j in range(i + 1, len(s) + 1):
substring = s[i:j]
if substring == substring[::-1] and len(substring) > len(longest):
longest = substring
return longest
Interviewer: “Good start. Now, let’s think about how we can optimize this solution. Any ideas?”
You: “We could potentially use dynamic programming to reduce the time complexity. Would you like me to explain that approach?”
This scenario demonstrates your ability to collaborate, communicate clearly, and consider different approaches to problem-solving.
Scenario 2: Code Review and Refactoring
In this scenario, you’re presented with existing code and asked to review and improve it.
Interviewer: “Here’s a function that calculates the factorial of a number. Let’s review it together and discuss any improvements we can make.”
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n-1)
You: “This implementation looks correct and uses recursion effectively. However, we could make a few improvements:”
- Add type hints for better readability and to catch potential type-related errors early.
- Include error handling for negative inputs.
- Consider using an iterative approach to avoid potential stack overflow for large inputs.
Interviewer: “Great observations. Let’s implement these improvements.”
You: “Certainly. I’ll start with the modifications:”
def factorial(n: int) -> int:
if not isinstance(n, int):
raise TypeError("Input must be an integer")
if n < 0:
raise ValueError("Factorial is not defined for negative numbers")
result = 1
for i in range(1, n + 1):
result *= i
return result
Interviewer: “Excellent refactoring. Can you explain why you chose an iterative approach over recursion?”
You: “I opted for an iterative approach primarily to avoid potential stack overflow issues with large inputs. While the recursive solution is elegant, it can lead to a stack overflow for very large numbers. The iterative solution is more memory-efficient and can handle larger inputs without risk of stack overflow.”
This scenario showcases your ability to review code critically, suggest improvements, and implement those improvements while explaining your reasoning.
Scenario 3: System Design Discussion
In this scenario, you collaborate with the interviewer to design a high-level system or feature.
Interviewer: “Let’s design a basic URL shortening service together. What components do you think we’ll need?”
You: “Great question. For a URL shortening service, we’ll need several key components:”
- A web server to handle incoming requests
- An API to create short URLs and redirect requests
- A database to store the mapping between short and long URLs
- A unique ID generator for creating short URLs
Interviewer: “Good start. How would you generate the short URLs?”
You: “We have a few options for generating short URLs:”
- We could use a hash function like MD5 or SHA-256 on the original URL and take the first few characters.
- We could use a counter and convert the number to a base62 string (A-Z, a-z, 0-9).
- We could generate random strings of a fixed length.
Each approach has pros and cons. The hash function might lead to collisions, the counter is predictable but efficient, and random strings might require multiple attempts to find an unused one. For this system, I’d suggest using a counter with base62 encoding for its simplicity and efficiency.
Interviewer: “Excellent. Let’s sketch out the basic API endpoints we’d need.”
You: “Certainly. We’d need at least two main endpoints:”
POST /shorten
Request body: { "url": "https://www.example.com/very/long/url" }
Response: { "short_url": "https://short.url/abc123" }
GET /{short_code}
Redirects to the original long URL
This scenario demonstrates your ability to think at a high level about system design, consider trade-offs between different approaches, and collaborate on API design.
Best Practices for Pair Programming Interviews
To make the most of your pair programming interview, keep these best practices in mind:
- Communicate Clearly: Explain your thought process as you work. If you’re unsure about something, ask for clarification.
- Be Open to Feedback: Listen to your interviewer’s suggestions and be willing to change direction if needed.
- Stay Calm: Remember that the process is collaborative. It’s okay to make mistakes or ask for help.
- Practice Active Listening: Pay attention to what your interviewer is saying and ask follow-up questions to ensure understanding.
- Show Enthusiasm: Demonstrate your passion for problem-solving and collaboration.
- Be Mindful of Time: Keep an eye on the time and pace yourself accordingly.
- Discuss Trade-offs: When proposing solutions, discuss the pros and cons of different approaches.
- Write Clean, Readable Code: Even in a time-constrained environment, strive for clean, well-structured code.
Common Challenges in Pair Programming Interviews
While pair programming interviews offer many advantages, they can also present unique challenges. Here are some common issues you might face and strategies to overcome them:
1. Difference in Coding Styles
Challenge: Your coding style might differ from your interviewer’s preferences.
Solution: Be flexible and open to adapting your style. If you’re unsure about a particular convention, ask for clarification.
2. Keeping Up with a Fast-Paced Interviewer
Challenge: The interviewer might move quickly through problems or concepts.
Solution: Don’t hesitate to ask for clarification or to slow down if you need more time to process information.
3. Handling Disagreements
Challenge: You and your interviewer might disagree on the best approach to a problem.
Solution: Present your reasoning calmly and listen to their perspective. Be prepared to compromise or try multiple approaches if time allows.
4. Technical Difficulties
Challenge: In remote interviews, you might encounter issues with screen sharing or collaborative coding tools.
Solution: Familiarize yourself with common tools beforehand. Have a backup plan (like sharing code snippets via chat) in case of technical issues.
5. Balancing Talking and Coding
Challenge: Finding the right balance between explaining your thought process and making progress on the code.
Solution: Practice verbalizing your thoughts while coding. If you need a moment of silence to concentrate, communicate this to your interviewer.
The Future of Pair Programming in Interviews
As the tech industry continues to evolve, pair programming is likely to become an even more integral part of the interview process. Here are some trends to watch:
- Increased Use of AI: Some companies are experimenting with AI-powered pair programming partners to standardize the interview experience.
- Virtual Reality Interviews: As VR technology advances, we may see pair programming interviews conducted in virtual environments, simulating a more realistic collaborative setting.
- Focus on Soft Skills: Pair programming interviews are likely to place even greater emphasis on communication and collaboration skills alongside technical prowess.
- Diverse Problem Types: Expect to see a wider range of problems in pair programming interviews, including system design, debugging, and code optimization tasks.
Conclusion
Pair programming interviews offer a unique opportunity to showcase not only your coding skills but also your ability to collaborate, communicate, and problem-solve in real-time. By understanding the fundamentals of pair programming, preparing thoroughly, and following best practices, you can turn these interviews into a chance to shine.
Remember, the key to success in pair programming interviews lies in clear communication, openness to feedback, and a collaborative mindset. As you prepare for your next technical interview, consider using platforms like AlgoCademy to practice collaborative coding and enhance your problem-solving skills.
With the right preparation and mindset, you can approach pair programming interviews with confidence, demonstrating your technical skills and your ability to work effectively in a team environment. As the tech industry continues to value collaboration and real-world problem-solving skills, mastering pair programming can give you a significant advantage in your job search and future career.