Pair Programming: Enhancing Collaboration and Code Quality in Software Development
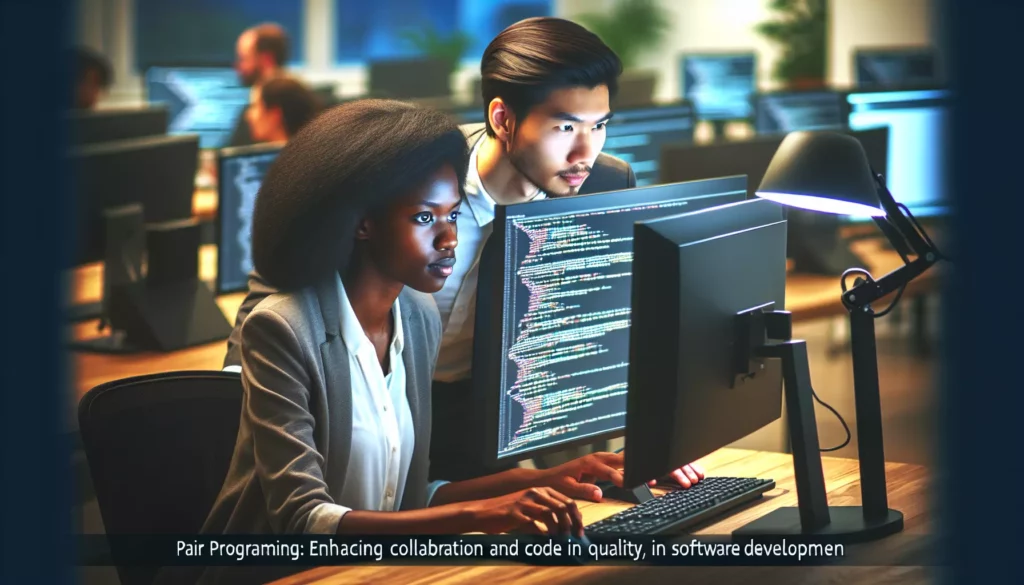
In the ever-evolving landscape of software development, various methodologies and practices have emerged to improve code quality, enhance productivity, and foster collaboration among developers. One such practice that has gained significant traction in recent years is pair programming. This collaborative approach to coding has proven to be a powerful tool in the arsenal of modern software development teams. In this comprehensive guide, we’ll explore what pair programming is, its numerous benefits, and how it can be effectively implemented in your development process.
What is Pair Programming?
Pair programming is a software development technique in which two programmers work together at a single workstation. This collaborative approach involves two roles:
- The Driver: The programmer who actively writes the code and controls the keyboard and mouse.
- The Navigator: The programmer who observes, reviews, and provides guidance to the driver in real-time.
These roles are typically swapped frequently, allowing both programmers to take turns in each role. The idea behind pair programming is that two heads are better than one, especially when it comes to solving complex problems and writing high-quality code.
The Benefits of Pair Programming
Pair programming offers a wide array of benefits that can significantly improve the software development process. Let’s dive into some of the key advantages:
1. Improved Code Quality
One of the primary benefits of pair programming is the improvement in code quality. When two developers work together, they can catch errors and bugs more quickly than a single programmer working alone. The navigator can spot mistakes in real-time, leading to fewer bugs and cleaner code. This real-time code review process helps in:
- Identifying logical errors
- Catching syntax mistakes
- Ensuring adherence to coding standards and best practices
- Improving overall code readability and maintainability
2. Knowledge Sharing and Skill Development
Pair programming serves as an excellent platform for knowledge sharing and skill development. When two developers with different skill sets and experiences work together, they can learn from each other’s strengths and perspectives. This collaborative environment fosters:
- Rapid knowledge transfer between team members
- Exposure to different problem-solving approaches
- Learning of new tools, techniques, and best practices
- Mentoring opportunities for senior developers to guide junior team members
3. Enhanced Problem-Solving Capabilities
When faced with complex problems, two minds working together can often find solutions more quickly and efficiently than a single developer. Pair programming encourages:
- Brainstorming and discussing alternative approaches
- Challenging assumptions and preconceptions
- Leveraging diverse experiences and perspectives
- Breaking down complex problems into manageable parts
4. Increased Focus and Productivity
While it might seem counterintuitive to have two developers working on the same task, pair programming can actually lead to increased productivity. The constant interaction and collaboration help in:
- Maintaining focus and reducing distractions
- Keeping developers accountable and on-task
- Encouraging a steady work pace
- Reducing time spent on debugging and fixing errors
5. Better Code Design and Architecture
The collaborative nature of pair programming often leads to better overall code design and architecture. With two developers constantly discussing and refining ideas, the resulting code tends to be:
- More modular and easier to maintain
- Better structured and organized
- More aligned with design patterns and architectural best practices
- More scalable and adaptable to future changes
6. Improved Team Cohesion and Communication
Pair programming fosters stronger relationships among team members and improves overall team dynamics. This collaborative approach encourages:
- Better communication skills
- Increased empathy and understanding among team members
- Shared ownership of code and project outcomes
- A more cohesive and supportive team environment
7. Reduced Bus Factor
The “bus factor” refers to the risk associated with knowledge being concentrated in a single team member. Pair programming helps mitigate this risk by:
- Spreading knowledge across multiple team members
- Ensuring that more than one person is familiar with each part of the codebase
- Reducing dependency on any single individual
- Improving project continuity in case of team member turnover
Implementing Pair Programming Effectively
While the benefits of pair programming are numerous, implementing this practice effectively requires careful consideration and planning. Here are some tips for successfully incorporating pair programming into your development process:
1. Choose the Right Pairs
Not all developer pairings will be equally effective. Consider the following when forming pairs:
- Mix skill levels: Pairing a senior developer with a junior developer can be highly beneficial for mentoring and knowledge transfer.
- Rotate pairs regularly: This ensures that knowledge is spread across the team and prevents the formation of information silos.
- Consider personality compatibility: While not always possible, try to pair developers who work well together and have complementary communication styles.
2. Set Clear Goals and Expectations
Before starting a pair programming session, it’s important to:
- Define the objectives of the session
- Agree on the roles (driver and navigator) and how often to switch
- Establish ground rules for communication and collaboration
- Set time limits to maintain focus and prevent fatigue
3. Create a Comfortable Work Environment
The physical setup can greatly impact the effectiveness of pair programming. Consider:
- Using a large monitor or dual monitors to ensure both developers can see the code clearly
- Providing comfortable seating arrangements that allow for easy communication
- Ensuring a quiet workspace to minimize distractions
- Using collaboration tools for remote pair programming when necessary
4. Encourage Active Participation
Both the driver and navigator should be actively engaged throughout the session:
- The navigator should provide timely feedback and suggestions
- The driver should vocalize their thought process and explain their actions
- Encourage open discussion and debate about different approaches
- Ensure both partners have equal opportunities to contribute ideas
5. Practice Effective Communication
Good communication is crucial for successful pair programming:
- Use clear and concise language
- Ask questions and seek clarification when needed
- Provide constructive feedback in a respectful manner
- Be open to different ideas and perspectives
6. Regular Breaks and Reflection
To maintain productivity and prevent burnout:
- Take regular breaks to rest and recharge
- Reflect on the session’s progress and adjust as needed
- Discuss what worked well and areas for improvement
- Celebrate successes and learning moments
Pair Programming in Practice: A Simple Example
To illustrate how pair programming works in practice, let’s consider a simple example of two developers, Alice and Bob, working together to implement a function that checks if a given string is a palindrome.
Alice takes on the role of the driver, while Bob acts as the navigator. Here’s how their interaction might unfold:
Alice (Driver): "Okay, let's start by defining our function. We'll call it 'isPalindrome' and it'll take a string as input."
Bob (Navigator): "Sounds good. Don't forget to add a comment explaining what the function does."
Alice: "Right, thanks for reminding me." (Types the following code)
// Function to check if a given string is a palindrome
function isPalindrome(str) {
// TODO: Implement palindrome check
}
Bob: "Great. Now, what's our approach going to be?"
Alice: "I'm thinking we could convert the string to lowercase, remove non-alphanumeric characters, and then compare it with its reverse."
Bob: "That's a solid approach. Let's start by converting to lowercase and removing non-alphanumeric characters."
Alice: "Okay, here goes." (Types the following code)
function isPalindrome(str) {
// Convert to lowercase and remove non-alphanumeric characters
const cleanStr = str.toLowerCase().replace(/[^a-z0-9]/g, '');
// TODO: Compare with reverse
}
Bob: "Looks good. Now we need to compare it with its reverse. Remember, we can use the split, reverse, and join methods to reverse the string."
Alice: "Right, I'll add that now." (Adds the following code)
function isPalindrome(str) {
// Convert to lowercase and remove non-alphanumeric characters
const cleanStr = str.toLowerCase().replace(/[^a-z0-9]/g, '');
// Compare with reverse
return cleanStr === cleanStr.split('').reverse().join('');
}
Bob: "Perfect! Let's add a few test cases to make sure it works correctly."
Alice: "Good idea." (Adds test cases)
console.log(isPalindrome("A man, a plan, a canal: Panama")); // Should return true
console.log(isPalindrome("race a car")); // Should return false
console.log(isPalindrome("Was it a car or a cat I saw?")); // Should return true
Bob: "Great job! The function looks correct and the test cases cover different scenarios. Shall we run it to verify?"
Alice: "Definitely. Let's see the results." (Runs the code)
// Output:
// true
// false
// true
Bob: "Excellent! The function is working as expected. Is there anything else we should consider?"
Alice: "We could add some error handling, like checking if the input is a string."
Bob: "Good point. Let's add that check at the beginning of the function."
This example demonstrates how pair programming facilitates real-time collaboration, knowledge sharing, and immediate feedback. Alice and Bob work together to implement the function, with Bob providing suggestions and catching potential issues while Alice writes the code. This collaborative approach results in a well-structured, tested, and robust solution.
Overcoming Challenges in Pair Programming
While pair programming offers numerous benefits, it’s not without its challenges. Here are some common issues that teams might face when implementing pair programming, along with strategies to overcome them:
1. Resistance to Change
Challenge: Some developers may be resistant to pair programming, preferring to work independently.
Solution:
- Educate the team about the benefits of pair programming
- Start with short, focused pairing sessions and gradually increase duration
- Encourage feedback and address concerns promptly
- Lead by example, with managers and senior developers participating in pair programming
2. Personality Conflicts
Challenge: Differences in personality or working styles can lead to tension or ineffective collaboration.
Solution:
- Rotate pairs regularly to prevent prolonged conflicts
- Provide training on effective communication and conflict resolution
- Encourage open dialogue about working preferences and styles
- Mediate conflicts when necessary and adjust pairings accordingly
3. Skill Level Disparities
Challenge: Significant differences in skill levels between pairs can lead to frustration or inefficiency.
Solution:
- Frame skill disparities as learning opportunities for both partners
- Encourage more experienced developers to practice patience and mentoring
- Set clear expectations for knowledge sharing and skill development
- Balance pairs to include a mix of skill levels across the team
4. Maintaining Focus and Energy
Challenge: Pair programming can be mentally taxing, leading to fatigue and decreased productivity.
Solution:
- Schedule regular breaks and switch roles frequently
- Limit pair programming sessions to manageable durations (e.g., 2-4 hours)
- Encourage pairs to take short walks or engage in brief physical activities between sessions
- Provide a comfortable and ergonomic work environment
5. Measuring Individual Contributions
Challenge: Traditional metrics for individual productivity may not apply in pair programming scenarios.
Solution:
- Focus on team productivity and code quality metrics rather than individual output
- Implement peer evaluations that consider collaboration and knowledge sharing
- Recognize and reward effective pairing and mentoring behaviors
- Educate stakeholders about the value and metrics of pair programming
Pair Programming in Different Development Contexts
Pair programming can be adapted to various development contexts and methodologies. Here’s how it can be effectively integrated into different scenarios:
1. Agile Development
Pair programming aligns well with Agile principles, emphasizing collaboration, adaptability, and continuous improvement. In Agile environments:
- Use pair programming for complex user stories or critical features
- Incorporate pairing into daily stand-ups and sprint planning
- Rotate pairs between sprints to spread knowledge across the team
- Use pair programming for code reviews and refactoring tasks
2. Remote Teams
With the rise of remote work, virtual pair programming has become increasingly common:
- Utilize screen sharing and collaborative coding tools (e.g., VS Code Live Share, Tuple)
- Establish clear communication protocols for remote pairing
- Schedule regular video check-ins to maintain personal connection
- Be mindful of time zone differences when scheduling pairing sessions
3. Large-Scale Projects
In large projects with multiple teams or components:
- Use pair programming to onboard new team members quickly
- Implement cross-team pairing to improve overall system understanding
- Use pairing for integrating components or resolving cross-module issues
- Rotate pairs to ensure knowledge of different parts of the system
4. Startups and Small Teams
In startup environments or small teams:
- Use pair programming to rapidly prototype and iterate on ideas
- Leverage pairing to cross-train team members on different aspects of the product
- Use mob programming (multiple developers working on the same task) for critical decisions or complex problems
- Implement pair programming selectively based on task complexity and available resources
Conclusion: Embracing Pair Programming for Enhanced Development
Pair programming is a powerful technique that can significantly improve code quality, foster knowledge sharing, and enhance team collaboration in software development. By bringing two minds together to tackle coding challenges, it offers a unique approach to problem-solving and skill development.
While implementing pair programming effectively may require some adjustment and overcoming initial challenges, the long-term benefits to both individual developers and the overall team are substantial. From improved code quality and reduced bugs to enhanced learning opportunities and stronger team cohesion, pair programming can be a game-changer for development teams of all sizes and across various contexts.
As with any development practice, the key to success with pair programming lies in thoughtful implementation, continuous refinement, and a commitment to open communication within the team. By embracing pair programming and adapting it to fit your team’s unique needs and workflow, you can unlock new levels of productivity, creativity, and collaboration in your software development process.
Whether you’re a seasoned developer looking to improve your skills, a team leader aiming to boost your team’s performance, or a newcomer to the world of coding, pair programming offers valuable opportunities for growth and improvement. So why not give it a try? You might just find that two heads are indeed better than one when it comes to writing great code and building amazing software.