Pair in C++: A Comprehensive Guide to Storing and Manipulating Data Pairs
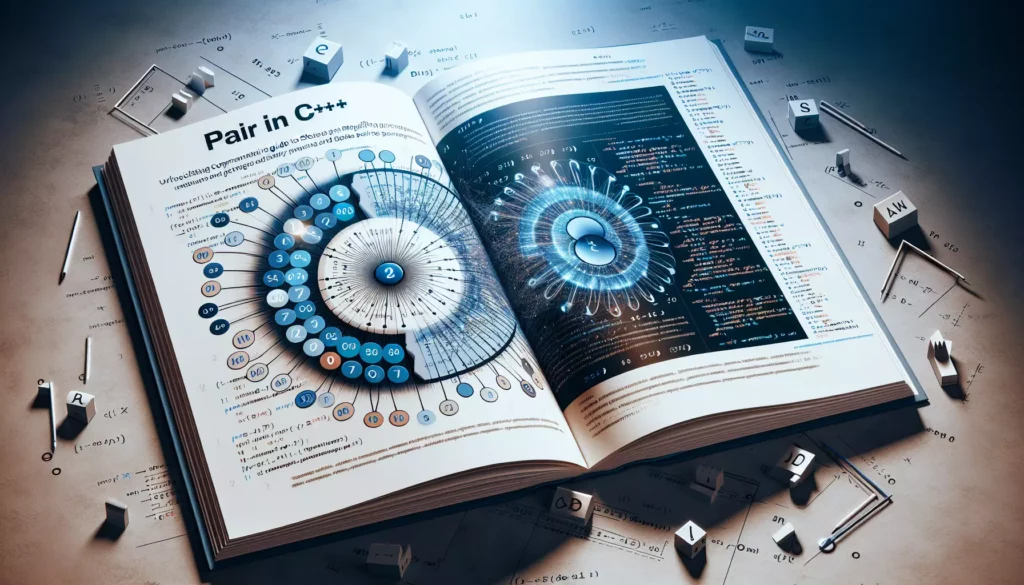
In the world of programming, it’s often necessary to work with pairs of related data. Whether you’re dealing with key-value pairs, coordinates, or any other type of paired information, having a convenient way to store and manipulate these pairs can greatly simplify your code. C++ provides a powerful template class called std::pair
that does just that. In this comprehensive guide, we’ll explore the ins and outs of using pairs in C++, from basic usage to advanced techniques.
Table of Contents
- Introduction to std::pair
- Creating and Initializing Pairs
- Accessing Pair Elements
- Modifying Pair Elements
- Comparing Pairs
- Using Pairs with STL Containers
- Pair as Function Return Type
- Advanced Techniques with Pairs
- Best Practices and Common Pitfalls
- Real-world Examples
- Conclusion
1. Introduction to std::pair
The std::pair
is a class template defined in the <utility>
header of the C++ Standard Library. It allows you to store two heterogeneous objects as a single unit. The two elements in a pair can be of different types, making it a versatile tool for various programming scenarios.
Here’s the basic structure of a pair:
template <class T1, class T2>
struct pair {
T1 first;
T2 second;
};
As you can see, a pair consists of two public members: first
and second
. These members can be of any type, including user-defined classes.
2. Creating and Initializing Pairs
There are several ways to create and initialize pairs in C++. Let’s explore the most common methods:
2.1. Using the Constructor
You can create a pair by using its constructor and specifying the values for both elements:
#include <iostream>
#include <utility>
int main() {
std::pair<int, std::string> p1(42, "Hello");
std::cout << p1.first << ", " << p1.second << std::endl;
return 0;
}
2.2. Using make_pair()
The std::make_pair()
function provides a convenient way to create pairs without explicitly specifying the types:
#include <iostream>
#include <utility>
int main() {
auto p2 = std::make_pair(3.14, 'A');
std::cout << p2.first << ", " << p2.second << std::endl;
return 0;
}
2.3. Using Uniform Initialization
C++11 introduced uniform initialization, which allows you to create pairs using curly braces:
#include <iostream>
#include <utility>
int main() {
std::pair<double, char> p3 {2.718, 'B'};
std::cout << p3.first << ", " << p3.second << std::endl;
return 0;
}
3. Accessing Pair Elements
Accessing the elements of a pair is straightforward. You can use the first
and second
member variables directly:
#include <iostream>
#include <utility>
int main() {
std::pair<int, std::string> p(10, "Hello");
std::cout << "First element: " << p.first << std::endl;
std::cout << "Second element: " << p.second << std::endl;
return 0;
}
Additionally, C++17 introduced structured bindings, which allow you to unpack the pair elements into separate variables:
#include <iostream>
#include <utility>
int main() {
std::pair<int, std::string> p(20, "World");
auto [x, y] = p;
std::cout << "x: " << x << ", y: " << y << std::endl;
return 0;
}
4. Modifying Pair Elements
You can modify the elements of a pair by directly assigning new values to the first
and second
members:
#include <iostream>
#include <utility>
int main() {
std::pair<int, std::string> p(30, "Old");
std::cout << "Before: " << p.first << ", " << p.second << std::endl;
p.first = 40;
p.second = "New";
std::cout << "After: " << p.first << ", " << p.second << std::endl;
return 0;
}
5. Comparing Pairs
C++ provides comparison operators for pairs, which perform lexicographical comparison. This means that the first
elements are compared first, and if they are equal, the second
elements are compared:
#include <iostream>
#include <utility>
int main() {
std::pair<int, char> p1(1, 'A');
std::pair<int, char> p2(1, 'B');
std::pair<int, char> p3(2, 'A');
std::cout << (p1 < p2) << std::endl; // true
std::cout << (p1 < p3) << std::endl; // true
std::cout << (p2 < p3) << std::endl; // true
std::cout << (p1 == p2) << std::endl; // false
return 0;
}
6. Using Pairs with STL Containers
Pairs are commonly used with STL containers, especially with associative containers like std::map
and std::multimap
. Here’s an example of using pairs with a vector:
#include <iostream>
#include <utility>
#include <vector>
#include <algorithm>
int main() {
std::vector<std::pair<int, std::string>> vec;
vec.push_back({3, "Three"});
vec.push_back({1, "One"});
vec.push_back({2, "Two"});
std::sort(vec.begin(), vec.end());
for (const auto& p : vec) {
std::cout << p.first << ": " << p.second << std::endl;
}
return 0;
}
7. Pair as Function Return Type
Pairs are useful for returning multiple values from a function. This can be more efficient and cleaner than using output parameters:
#include <iostream>
#include <utility>
#include <string>
std::pair<std::string, int> parse_name_age(const std::string& input) {
size_t pos = input.find(',');
std::string name = input.substr(0, pos);
int age = std::stoi(input.substr(pos + 1));
return {name, age};
}
int main() {
auto [name, age] = parse_name_age("Alice,30");
std::cout << "Name: " << name << ", Age: " << age << std::endl;
return 0;
}
8. Advanced Techniques with Pairs
8.1. Nested Pairs
You can create nested pairs to represent more complex data structures:
#include <iostream>
#include <utility>
#include <string>
int main() {
std::pair<std::string, std::pair<int, double>> person("Alice", {30, 1.75});
std::cout << "Name: " << person.first << std::endl;
std::cout << "Age: " << person.second.first << std::endl;
std::cout << "Height: " << person.second.second << std::endl;
return 0;
}
8.2. Using Pairs with Custom Types
You can use pairs with custom types, but make sure to provide appropriate comparison operators if you plan to use them in sorted containers:
#include <iostream>
#include <utility>
#include <string>
class Person {
public:
Person(std::string n, int a) : name(n), age(a) {}
std::string name;
int age;
};
bool operator<(const Person& lhs, const Person& rhs) {
return lhs.age < rhs.age;
}
int main() {
std::pair<Person, std::string> p1({"Alice", 30}, "Engineer");
std::pair<Person, std::string> p2({"Bob", 25}, "Designer");
std::cout << (p1 < p2) << std::endl; // false
return 0;
}
9. Best Practices and Common Pitfalls
9.1. Use Meaningful Names
When using pairs, try to give meaningful names to the variables or use comments to explain what each element represents:
std::pair<double, double> coordinates; // (x, y)
std::pair<std::string, int> name_age; // (name, age)
9.2. Consider Using Structs for Named Fields
If you find yourself using pairs with the same types repeatedly, consider creating a struct with named fields for better readability:
struct Person {
std::string name;
int age;
};
// Instead of:
// std::pair<std::string, int> person;
9.3. Be Careful with Auto Type Deduction
When using auto
with pairs, be aware that the deduced type might not be what you expect, especially with references:
#include <iostream>
#include <utility>
int main() {
int x = 10;
auto p1 = std::make_pair(x, 20); // std::pair<int, int>
auto p2 = std::make_pair(std::ref(x), 20); // std::pair<std::reference_wrapper<int>, int>
x = 30;
std::cout << p1.first << std::endl; // 10
std::cout << p2.first << std::endl; // 30
return 0;
}
10. Real-world Examples
10.1. Using Pairs in a Dictionary
Here’s an example of using pairs to implement a simple dictionary:
#include <iostream>
#include <utility>
#include <vector>
#include <algorithm>
#include <string>
class Dictionary {
private:
std::vector<std::pair<std::string, std::string>> entries;
public:
void add_entry(const std::string& word, const std::string& definition) {
entries.emplace_back(word, definition);
std::sort(entries.begin(), entries.end());
}
std::string lookup(const std::string& word) {
auto it = std::lower_bound(entries.begin(), entries.end(), word,
[](const auto& entry, const std::string& w) {
return entry.first < w;
});
if (it != entries.end() && it->first == word) {
return it->second;
}
return "Word not found";
}
};
int main() {
Dictionary dict;
dict.add_entry("apple", "A fruit");
dict.add_entry("car", "A vehicle");
dict.add_entry("book", "A written work");
std::cout << dict.lookup("car") << std::endl;
std::cout << dict.lookup("phone") << std::endl;
return 0;
}
10.2. Using Pairs for Graph Representation
Pairs can be used to represent edges in a graph:
#include <iostream>
#include <utility>
#include <vector>
#include <algorithm>
class Graph {
private:
int num_vertices;
std::vector<std::vector<std::pair<int, int>>> adj_list;
public:
Graph(int v) : num_vertices(v), adj_list(v) {}
void add_edge(int u, int v, int weight) {
adj_list[u].emplace_back(v, weight);
adj_list[v].emplace_back(u, weight);
}
void print_graph() {
for (int i = 0; i < num_vertices; ++i) {
std::cout << "Vertex " << i << ":";
for (const auto& edge : adj_list[i]) {
std::cout << " (" << edge.first << ", " << edge.second << ")";
}
std::cout << std::endl;
}
}
};
int main() {
Graph g(4);
g.add_edge(0, 1, 10);
g.add_edge(0, 2, 20);
g.add_edge(1, 2, 30);
g.add_edge(2, 3, 40);
g.print_graph();
return 0;
}
11. Conclusion
The std::pair
class template in C++ is a versatile and powerful tool for working with pairs of data. It provides a simple way to group two values together, which can be of different types. Pairs are widely used in the Standard Template Library, especially in associative containers, and can greatly simplify your code when dealing with related data.
In this comprehensive guide, we’ve covered the basics of creating and using pairs, as well as more advanced techniques and real-world examples. We’ve seen how pairs can be used to return multiple values from functions, represent edges in graphs, and implement simple data structures like dictionaries.
While pairs are extremely useful, it’s important to use them judiciously. For more complex data structures with more than two elements or when you need named fields, consider using structs or classes instead. Always strive for code readability and maintainability when deciding whether to use pairs or other data structures.
By mastering the use of pairs in C++, you’ll have a powerful tool at your disposal for writing cleaner, more efficient code in a wide range of programming scenarios.