Overcoming the Blank Mind Syndrome: How to Start Solving a Problem When You Have No Idea
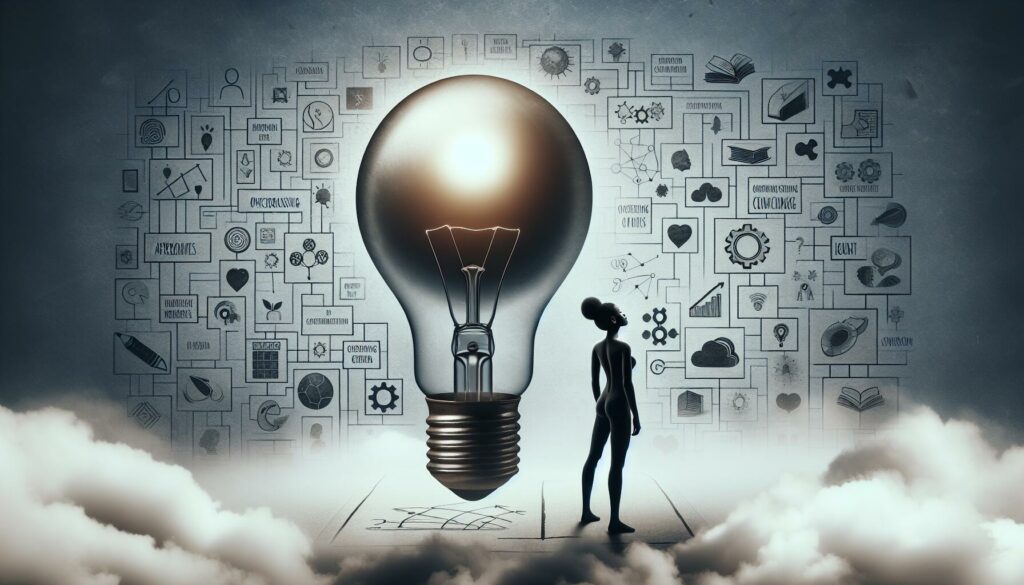
Have you ever found yourself staring at a coding problem, your mind as blank as the untouched snow? You’re not alone. This phenomenon, often referred to as “Blank Mind Syndrome,” is a common hurdle for programmers of all levels, from beginners to seasoned professionals. It’s that moment when you’re faced with a complex problem, and your brain seems to shut down, leaving you without a clue on how to begin.
In the world of coding and algorithmic problem-solving, getting started is often the most challenging part. But fear not! This comprehensive guide will equip you with powerful techniques to overcome this mental block and kick-start your problem-solving process. We’ll explore methods like problem restatement, hypothesis generation, and simplifying assumptions, providing you with a toolkit to tackle even the most daunting coding challenges.
Understanding Blank Mind Syndrome
Before we dive into the solutions, let’s take a moment to understand what Blank Mind Syndrome is and why it occurs. This psychological phenomenon is not unique to programming; it can happen in any situation where we’re faced with a complex task or problem that seems overwhelming at first glance.
In the context of coding and algorithmic problem-solving, Blank Mind Syndrome often manifests when:
- You’re presented with a problem that seems too complex or abstract
- You’re under time pressure, such as during a coding interview or exam
- You’re dealing with unfamiliar concepts or technologies
- You’re experiencing fatigue or stress
- You’re overthinking and trying to solve the entire problem at once
Recognizing when you’re experiencing Blank Mind Syndrome is the first step towards overcoming it. Now, let’s explore some effective techniques to break through this mental barrier and start solving problems with confidence.
Technique 1: Problem Restatement
One of the most powerful techniques to overcome Blank Mind Syndrome is problem restatement. This method involves rephrasing the problem in your own words, which can help you gain a fresh perspective and uncover hidden insights.
How to Use Problem Restatement:
- Read the problem carefully: Make sure you understand all the given information and requirements.
- Identify key components: Break down the problem into its core elements.
- Rephrase in your own words: Rewrite the problem statement using different terminology or from a different angle.
- Focus on the outcome: Clearly state what the solution should achieve.
- Compare your restatement: Ensure your rephrased version aligns with the original problem.
Example: Two Sum Problem
Let’s apply problem restatement to a classic coding problem: the Two Sum problem.
Original problem statement: Given an array of integers nums and an integer target, return indices of the two numbers such that they add up to target.
Restated problem: Find two different positions in a list of numbers where the numbers at those positions add up to a specific total. Return the positions, not the numbers themselves.
By restating the problem, we’ve clarified a few key points:
- We’re looking for positions (indices), not the actual numbers
- The two numbers must be at different positions
- We need to return the positions that satisfy the condition
This restatement can help trigger ideas for potential solutions, such as using a hash table to store seen numbers and their indices.
Technique 2: Hypothesis Generation
Hypothesis generation is a powerful technique that involves proposing potential solutions or approaches to the problem, even if you’re not sure they’ll work. This method can help jumpstart your thinking process and lead you towards the correct solution.
How to Use Hypothesis Generation:
- Brainstorm possible approaches: Don’t worry about correctness at this stage; just generate ideas.
- Consider different data structures: Think about how various data structures might be applied to the problem.
- Explore algorithm categories: Consider if the problem fits into common algorithm categories (e.g., sorting, searching, dynamic programming).
- Think about similar problems: Recall solutions to problems you’ve solved before that might be relevant.
- Evaluate your hypotheses: Assess the pros and cons of each approach you’ve generated.
Example: Finding the Maximum Subarray Sum
Let’s apply hypothesis generation to the problem of finding the maximum subarray sum.
Problem: Given an array of integers, find the contiguous subarray with the largest sum and return that sum.
Hypotheses:
- Brute Force: Try all possible subarrays and keep track of the maximum sum.
- Divide and Conquer: Split the array and solve for smaller subarrays, then combine the results.
- Dynamic Programming: Build up the solution by considering one element at a time and maintaining a running maximum.
- Kadane’s Algorithm: A specific form of dynamic programming that keeps track of the best sum ending at each position.
By generating these hypotheses, we’ve given ourselves several starting points to explore. We can now evaluate each approach, considering factors like time complexity and implementation difficulty, to choose the most suitable solution.
Technique 3: Simplifying Assumptions
When faced with a complex problem, making simplifying assumptions can help you break it down into more manageable pieces. This technique involves temporarily ignoring certain aspects of the problem or assuming ideal conditions to get started.
How to Use Simplifying Assumptions:
- Identify complex aspects: Pinpoint the parts of the problem that are making it difficult to approach.
- Make simplifying assumptions: Temporarily ignore or simplify these aspects.
- Solve the simplified version: Develop a solution for the simplified problem.
- Gradually add complexity: Reintroduce the ignored aspects one by one, adjusting your solution as needed.
- Verify the final solution: Ensure your solution works for the original, unsimplified problem.
Example: Implementing a Cache with a Limited Size
Let’s apply simplifying assumptions to the problem of implementing a cache with a limited size.
Original problem: Implement a cache that can store a limited number of key-value pairs. When the cache is full, the least recently used item should be evicted to make room for a new item.
Simplifying assumptions:
- Assume the cache has unlimited size (remove the size constraint).
- Ignore the requirement for evicting the least recently used item.
- Assume all keys and values are strings.
With these simplifying assumptions, we can start by implementing a basic key-value store using a hash map:
class SimpleCache:
def __init__(self):
self.cache = {}
def get(self, key):
return self.cache.get(key)
def put(self, key, value):
self.cache[key] = value
Now, we can gradually add complexity:
- Add a size limit and basic eviction:
class LimitedCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = {}
def get(self, key):
return self.cache.get(key)
def put(self, key, value):
if len(self.cache) >= self.capacity and key not in self.cache:
self.cache.pop(next(iter(self.cache)))
self.cache[key] = value
- Implement least recently used (LRU) eviction:
from collections import OrderedDict
class LRUCache:
def __init__(self, capacity):
self.capacity = capacity
self.cache = OrderedDict()
def get(self, key):
if key not in self.cache:
return None
self.cache.move_to_end(key)
return self.cache[key]
def put(self, key, value):
if key in self.cache:
self.cache.move_to_end(key)
elif len(self.cache) >= self.capacity:
self.cache.popitem(last=False)
self.cache[key] = value
By using simplifying assumptions, we were able to approach the problem step by step, gradually increasing the complexity until we arrived at a full solution.
Applying These Techniques in Real-World Scenarios
Now that we’ve explored these powerful techniques for overcoming Blank Mind Syndrome, let’s look at how they can be applied in real-world coding scenarios, particularly in the context of technical interviews and complex programming tasks.
Scenario 1: Technical Interview – Graph Traversal
Problem: Given a graph representing a social network, find the shortest path between two users.
Application of techniques:
- Problem Restatement: Find the minimum number of friendship connections needed to link two people in a social network.
- Hypothesis Generation:
- Use Depth-First Search (DFS) to explore all possible paths
- Use Breadth-First Search (BFS) to find the shortest path
- Apply Dijkstra’s algorithm if the connections have weights
- Simplifying Assumptions:
- Assume the graph is undirected (friendships are mutual)
- Start with a small, simple graph to test the algorithm
- Initially ignore edge cases like disconnected users
By applying these techniques, you can quickly formulate an approach (likely using BFS for the unweighted shortest path) and start implementing the solution, even if you initially felt stuck.
Scenario 2: System Design – Implementing a URL Shortener
Problem: Design a system that shortens long URLs into unique, short aliases.
Application of techniques:
- Problem Restatement: Create a service that maps long strings (URLs) to short, unique identifiers, and vice versa.
- Hypothesis Generation:
- Use a hash function to generate short codes
- Implement a counter-based system for sequential short codes
- Use a combination of timestamp and random characters
- Simplifying Assumptions:
- Start with a single-server architecture
- Initially ignore scalability concerns
- Assume a fixed length for the short codes
These techniques help break down the complex system design problem into manageable components, allowing you to start with a basic design and then expand on it.
Scenario 3: Algorithm Optimization – Improving Search Performance
Problem: Optimize the search function for a large dataset of product information.
Application of techniques:
- Problem Restatement: Reduce the time it takes to find specific products in a vast collection of data.
- Hypothesis Generation:
- Implement indexing for faster lookups
- Use a more efficient data structure (e.g., hash table, B-tree)
- Apply caching mechanisms for frequently searched items
- Simplifying Assumptions:
- Start by optimizing for a single search criterion
- Initially focus on in-memory optimization before considering database-level improvements
- Assume a static dataset to begin with, before handling real-time updates
By breaking down the optimization problem and considering various approaches, you can systematically improve the search performance, even if the initial complexity seemed overwhelming.
Integrating These Techniques into Your Problem-Solving Routine
Overcoming Blank Mind Syndrome is not just about knowing the techniques; it’s about integrating them into your regular problem-solving routine. Here are some tips to help you make these methods a natural part of your approach to coding challenges:
- Practice regularly: Like any skill, problem-solving improves with practice. Regularly tackle coding challenges, even when you’re not preparing for interviews or working on specific projects.
- Start with the techniques: When faced with a new problem, make it a habit to begin by applying these techniques before diving into coding.
- Keep a problem-solving journal: Document how you apply these techniques to different problems. This can help you reflect on your process and improve over time.
- Collaborate and discuss: Share your problem-solving approaches with peers or in coding communities. Explaining your thought process can reinforce your understanding and expose you to new perspectives.
- Embrace the struggle: Remember that feeling stuck is a normal part of the problem-solving process. Use it as a cue to apply these techniques rather than a sign of failure.
- Review and refine: After solving a problem, review how you applied these techniques and consider how you might improve your approach next time.
Conclusion: Empowering Your Problem-Solving Skills
Blank Mind Syndrome can be a frustrating experience for any programmer, but it doesn’t have to be a roadblock in your coding journey. By mastering techniques like problem restatement, hypothesis generation, and simplifying assumptions, you can equip yourself with powerful tools to overcome mental blocks and approach even the most challenging problems with confidence.
Remember, the goal is not just to solve individual problems, but to develop a robust problem-solving mindset that will serve you throughout your programming career. Whether you’re preparing for technical interviews, working on complex projects, or simply honing your skills, these techniques will help you break through the initial paralysis and start making progress.
As you continue to grow as a programmer, keep exploring new problem-solving strategies and refining your approach. The ability to tackle unfamiliar and complex problems is what sets great programmers apart, and with consistent practice and the right techniques, you can cultivate this valuable skill.
So the next time you find yourself staring at a blank screen, mind empty of ideas, remember these techniques. Take a deep breath, restate the problem, generate some hypotheses, make simplifying assumptions, and watch as the path forward begins to reveal itself. Happy coding, and may your mind never stay blank for long!