Overcoming Perfectionism: Knowing When Your Code is Good Enough
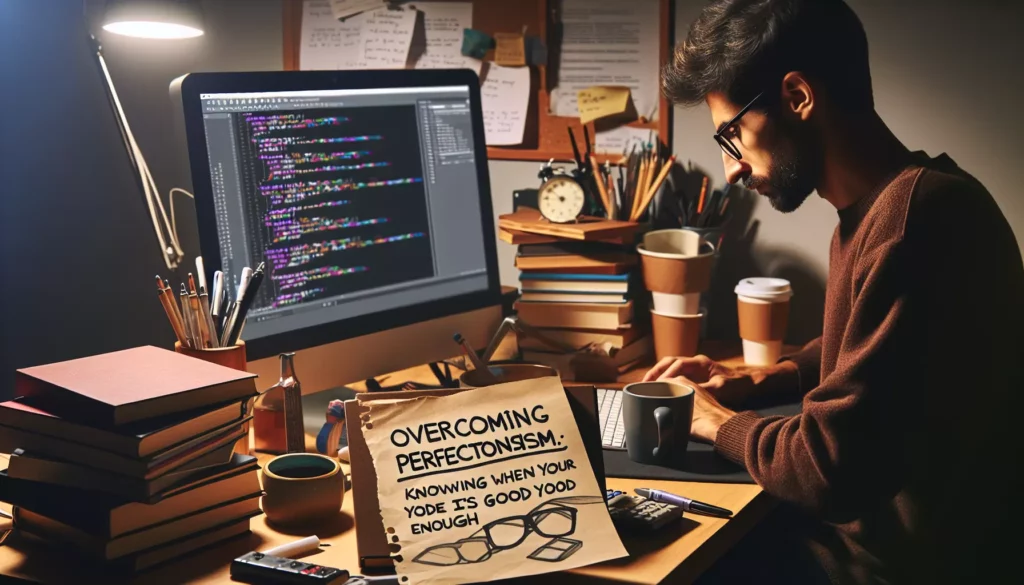
As programmers, we often strive for perfection in our code. We want our algorithms to be optimized, our functions to be elegant, and our projects to be flawless. However, this pursuit of perfection can sometimes hinder our progress and productivity. In this article, we’ll explore the concept of perfectionism in coding, its potential drawbacks, and how to strike a balance between writing high-quality code and knowing when it’s good enough.
The Perfectionist Programmer’s Dilemma
Perfectionism in programming can manifest in various ways:
- Endlessly refactoring code
- Obsessing over minor optimizations
- Postponing project completion due to perceived imperfections
- Fear of submitting code for review
- Difficulty collaborating due to overly high standards
While attention to detail and a desire for quality are admirable traits, taken to extremes, they can lead to decreased productivity, missed deadlines, and even burnout.
The Costs of Perfectionism
Let’s examine some of the negative impacts that unchecked perfectionism can have on your coding journey:
1. Reduced Productivity
When you’re constantly second-guessing your code and making minor tweaks, you may find that you’re not making significant progress on your projects. This can lead to a backlog of unfinished work and missed opportunities.
2. Increased Stress and Anxiety
The pressure to produce perfect code can be overwhelming, leading to stress and anxiety. This can negatively impact your mental health and overall well-being.
3. Difficulty Learning and Growing
Perfectionism can make it harder to learn from mistakes and embrace the iterative nature of coding. If you’re afraid to write imperfect code, you may miss out on valuable learning experiences.
4. Impaired Collaboration
In team settings, perfectionism can make it challenging to work effectively with others. You may struggle to accept others’ contributions or be hesitant to share your own work.
Strategies for Overcoming Perfectionism
Now that we’ve identified the potential pitfalls of perfectionism, let’s explore some strategies to help you overcome it and find a healthy balance in your coding practice.
1. Embrace the Concept of “Good Enough”
Recognize that there’s rarely such a thing as perfect code. Instead, aim for code that is functional, maintainable, and meets the requirements of your project. Remember the principle of YAGNI (You Ain’t Gonna Need It) – don’t add unnecessary features or optimizations just for the sake of perfection.
2. Set Realistic Goals and Deadlines
Break your projects into smaller, manageable tasks with realistic deadlines. This can help you focus on making steady progress rather than getting bogged down in details.
3. Practice Iterative Development
Adopt an iterative approach to coding. Start with a basic implementation that works, then refine and improve it over time. This allows you to make progress while still leaving room for improvements.
4. Use Version Control Effectively
Leverage version control systems like Git to your advantage. Commit your code regularly, even if it’s not perfect. This allows you to track your progress and revert changes if needed, reducing the fear of making mistakes.
5. Seek Feedback Early and Often
Don’t wait until your code is “perfect” to share it with others. Seek feedback from peers or mentors early in the development process. This can help you identify areas for improvement and gain new perspectives.
6. Focus on Learning, Not Just Performance
Shift your mindset from achieving perfection to continuous learning. Embrace mistakes as opportunities for growth and improvement.
Recognizing When Your Code is Good Enough
Determining when your code is “good enough” can be challenging, but there are several indicators you can use to guide your decision-making process:
1. Functionality
Does your code accomplish its intended purpose? If it meets the requirements and performs the necessary functions, it may be good enough for the current stage of development.
2. Readability and Maintainability
Is your code easy to read and understand? Can other developers (or your future self) maintain and modify it without excessive effort? If so, you’re on the right track.
3. Performance
Does your code perform adequately for its intended use case? While optimization is important, it’s not always necessary to achieve the absolute best performance for every piece of code.
4. Test Coverage
Have you written appropriate tests for your code? If your code passes all relevant tests and has good test coverage, it may be ready for the next stage.
5. Code Review Feedback
If you’ve received positive feedback from code reviews or your peers, it’s a good indication that your code is meeting acceptable standards.
6. Time Constraints
Consider the time constraints of your project. If you’re approaching a deadline and your code meets the essential requirements, it may be time to move forward.
Case Study: Balancing Perfectionism and Pragmatism
Let’s examine a practical example of how to balance the desire for perfect code with the need to make progress and meet deadlines.
Imagine you’re working on a function to find the nth Fibonacci number. You start with a simple recursive implementation:
def fibonacci(n):
if n <= 1:
return n
else:
return fibonacci(n-1) + fibonacci(n-2)
This function works correctly but has poor performance for large values of n due to redundant calculations. A perfectionist might be tempted to immediately optimize this function using dynamic programming or memoization. However, let’s consider a more balanced approach:
- Functionality Check: The current implementation is correct and works for small values of n.
- Test Coverage: Write unit tests to verify the function’s correctness for various inputs.
- Performance Evaluation: Benchmark the function to determine if its performance is acceptable for your current use case.
- Iterative Improvement: If performance is inadequate, implement an optimized version using dynamic programming:
def fibonacci_optimized(n):
if n <= 1:
return n
fib = [0] * (n + 1)
fib[1] = 1
for i in range(2, n + 1):
fib[i] = fib[i-1] + fib[i-2]
return fib[n]
By following this approach, you’ve created a working solution, validated it with tests, and made improvements based on actual needs rather than hypothetical perfection.
The Role of Code Reviews in Overcoming Perfectionism
Code reviews play a crucial role in helping programmers overcome perfectionism and develop a more balanced approach to coding. Here’s how code reviews can contribute to this process:
1. External Perspective
Code reviews provide an external perspective on your work, helping you identify areas that truly need improvement and those that are already sufficient.
2. Feedback and Learning
Constructive feedback from code reviews can help you learn and grow as a programmer, shifting your focus from achieving perfection to continuous improvement.
3. Confidence Building
Positive feedback from code reviews can boost your confidence and help you recognize when your code meets acceptable standards.
4. Collaborative Improvement
Code reviews foster a collaborative environment where improvements can be suggested and implemented as a team, reducing the pressure of individual perfectionism.
Tools and Techniques for Code Quality
While striving for perfection can be counterproductive, it’s still important to maintain high code quality. Here are some tools and techniques that can help you achieve this balance:
1. Linters and Static Analysis Tools
Use linters and static analysis tools to automatically check your code for potential issues and style violations. These tools can help you maintain consistency and catch common errors without obsessing over every detail.
Example of using a linter (flake8) in Python:
$ flake8 your_code.py
your_code.py:10:1: E302 expected 2 blank lines, found 1
your_code.py:15:80: E501 line too long (82 > 79 characters)
2. Automated Testing
Implement automated testing, including unit tests, integration tests, and end-to-end tests. This can give you confidence in your code’s correctness and help you identify when it’s ready for the next stage of development.
Example of a simple unit test in Python using pytest:
def test_fibonacci():
assert fibonacci(0) == 0
assert fibonacci(1) == 1
assert fibonacci(5) == 5
assert fibonacci(10) == 55
3. Continuous Integration (CI)
Set up a CI pipeline to automatically run tests, linters, and other quality checks whenever you push code to your repository. This can help catch issues early and reduce the need for manual perfectionism.
4. Code Formatting Tools
Use code formatting tools to automatically format your code according to established style guidelines. This can help maintain consistency without spending excessive time on manual formatting.
Example of using Black, a Python code formatter:
$ black your_code.py
reformatted your_code.py
All done! ✨ 🰠✨
1 file reformatted.
Balancing Perfectionism with Continuous Improvement
While it’s important to overcome debilitating perfectionism, it’s equally crucial to maintain a commitment to continuous improvement. Here are some strategies to help you strike this balance:
1. Set Incremental Goals
Instead of aiming for perfection, set incremental goals for improving your code and skills. This allows you to make steady progress without getting overwhelmed.
2. Regularly Reflect on Your Code
Schedule time to review your old code and identify areas for improvement. This retrospective approach can help you learn from past experiences and apply those lessons to future projects.
3. Stay Updated with Best Practices
Keep yourself informed about industry best practices and new developments in your programming language or framework of choice. This ongoing learning can help you write better code without falling into the perfectionism trap.
4. Embrace Refactoring
Make refactoring a regular part of your coding practice. Instead of trying to write perfect code from the start, allow yourself to improve and optimize your code over time.
5. Celebrate Small Victories
Acknowledge and celebrate your progress, no matter how small. This positive reinforcement can help shift your focus from achieving perfection to appreciating growth and improvement.
Conclusion
Overcoming perfectionism in coding is a journey that requires self-awareness, practice, and a shift in mindset. By embracing the concept of “good enough” code, setting realistic goals, and focusing on continuous improvement rather than absolute perfection, you can become a more productive and satisfied programmer.
Remember that coding is an iterative process, and there’s always room for improvement. By finding the right balance between striving for quality and accepting that your code is good enough for its current purpose, you’ll be better equipped to tackle challenges, meet deadlines, and grow as a developer.
As you continue your coding journey, whether you’re just starting out or preparing for technical interviews with major tech companies, keep in mind that progress is more important than perfection. Embrace the learning process, seek feedback, and focus on solving problems effectively rather than achieving an unattainable ideal of perfect code.
By applying the strategies and insights discussed in this article, you’ll be well on your way to becoming a more balanced, productive, and confident programmer. Remember, the goal is not to write perfect code, but to write code that solves problems and continues to evolve and improve over time.