Oracle Technical Interview Prep: Your Comprehensive Guide to Success
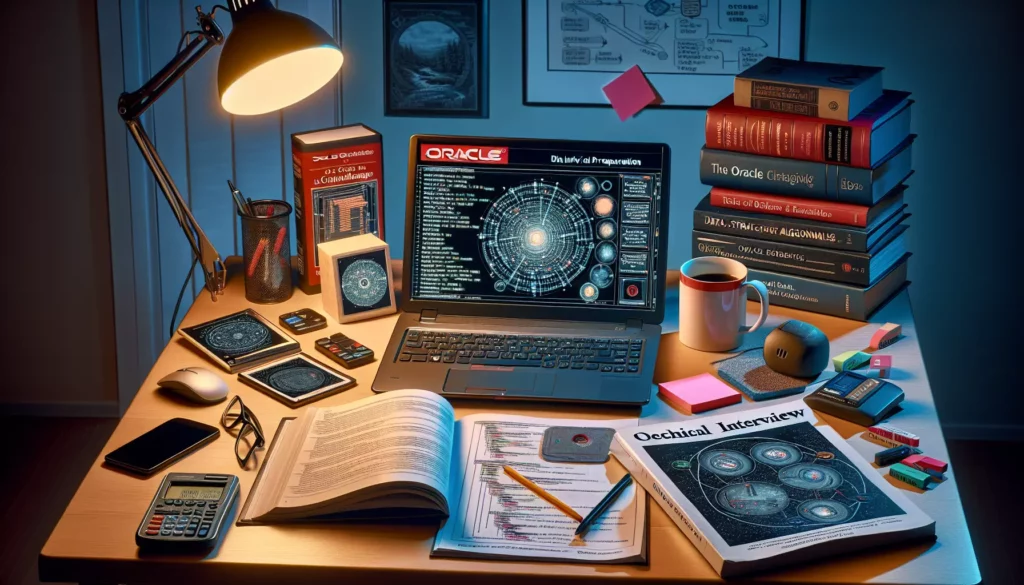
As one of the world’s largest technology companies, Oracle offers exciting career opportunities for software engineers and developers. However, landing a job at Oracle requires thorough preparation, especially for the technical interview. This comprehensive guide will walk you through the essential steps to ace your Oracle technical interview, covering everything from understanding the interview process to mastering key technical concepts.
Understanding the Oracle Interview Process
Before diving into the specifics of technical preparation, it’s crucial to understand Oracle’s interview process. Typically, it consists of several stages:
- Initial phone screening
- Technical phone interview
- On-site interviews (usually 4-5 rounds)
- HR interview
The technical interviews, both phone and on-site, are where your coding and problem-solving skills will be put to the test. These interviews usually focus on data structures, algorithms, system design, and Oracle-specific technologies.
Key Areas to Focus On
To prepare effectively for your Oracle technical interview, concentrate on the following key areas:
1. Data Structures and Algorithms
A solid understanding of data structures and algorithms is fundamental for any technical interview, including Oracle’s. Make sure you’re comfortable with:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching algorithms
- Dynamic Programming
- Recursion
Practice implementing these data structures and solving problems using them. Websites like LeetCode, HackerRank, and AlgoCademy offer a wealth of practice problems to hone your skills.
2. SQL and Database Concepts
Given Oracle’s strong focus on database technologies, a deep understanding of SQL and database concepts is crucial. Be prepared to demonstrate your knowledge of:
- Basic to advanced SQL queries
- Database design and normalization
- Indexing and query optimization
- Transactions and ACID properties
- Concurrency control
- Oracle-specific features (e.g., PL/SQL, Oracle Database architecture)
Practice writing complex SQL queries and explaining database concepts clearly and concisely.
3. Object-Oriented Programming (OOP)
Oracle uses various object-oriented programming languages in its products and services. Make sure you have a solid grasp of OOP concepts such as:
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
- Design patterns
Be prepared to discuss these concepts and demonstrate how you’ve applied them in your projects.
4. System Design
For more senior positions, system design questions are common in Oracle interviews. Familiarize yourself with:
- Scalability and performance optimization
- Distributed systems
- Microservices architecture
- Load balancing
- Caching strategies
- Database sharding
Practice designing scalable systems and be ready to explain your design choices.
5. Oracle-Specific Technologies
Depending on the position you’re applying for, you may need to demonstrate knowledge of Oracle-specific technologies such as:
- Oracle Database
- PL/SQL
- Oracle Cloud Infrastructure
- Oracle WebLogic Server
- Oracle Fusion Middleware
Research the specific technologies relevant to your desired role and brush up on your knowledge.
Coding Practice and Problem-Solving Strategies
To excel in your Oracle technical interview, consistent practice is key. Here are some strategies to improve your coding and problem-solving skills:
1. Regular Coding Practice
Set aside time each day for coding practice. Solve at least one or two problems daily, focusing on different data structures and algorithms. Websites like AlgoCademy offer a structured approach to learning and practicing, with AI-powered assistance to guide you through challenging problems.
2. Mock Interviews
Participate in mock interviews to simulate the real interview experience. Platforms like Pramp or interviewing.io allow you to practice with peers and receive feedback on your performance.
3. Time Management
Practice solving problems within a time constraint. In real interviews, you’ll typically have 30-45 minutes to solve a problem, so train yourself to work efficiently under pressure.
4. Explain Your Thought Process
Get into the habit of explaining your thought process as you solve problems. This skill is crucial during interviews, as interviewers are interested in understanding how you approach problem-solving.
5. Review and Optimize
After solving a problem, always review your solution and consider ways to optimize it. Think about time and space complexity, and be prepared to discuss trade-offs between different approaches.
Sample Oracle Interview Questions
To give you an idea of what to expect, here are some sample questions that might come up in an Oracle technical interview:
Data Structures and Algorithms:
- Implement a function to reverse a linked list.
- Find the longest common subsequence between two strings.
- Implement a binary search tree and write functions for insertion, deletion, and searching.
- Design an algorithm to find the kth largest element in an unsorted array.
SQL and Database:
- Write a SQL query to find the second highest salary in an employee table.
- Explain the difference between INNER JOIN and LEFT JOIN with examples.
- How would you optimize a slow-running query?
- Describe the ACID properties and their importance in database transactions.
Object-Oriented Programming:
- Explain the concept of polymorphism and provide an example.
- What is the difference between an abstract class and an interface?
- Describe the Singleton design pattern and when you might use it.
- How does encapsulation contribute to writing maintainable code?
System Design:
- Design a distributed cache system.
- How would you design a URL shortening service like bit.ly?
- Explain how you would design a scalable social media feed.
- Describe the architecture of a system that can handle millions of concurrent users.
Tips for Interview Success
Beyond technical preparation, keep these tips in mind to increase your chances of success in an Oracle technical interview:
1. Communicate Clearly
Clear communication is crucial. Explain your thoughts and approach clearly, even if you’re unsure about the final solution. Interviewers want to understand your problem-solving process.
2. Ask Clarifying Questions
Don’t hesitate to ask questions to clarify the problem statement or requirements. This shows that you’re thorough and want to understand the problem fully before diving into a solution.
3. Think Aloud
Verbalize your thought process as you work through problems. This gives the interviewer insight into your problem-solving approach and allows them to provide hints if you’re stuck.
4. Handle Edge Cases
Always consider and discuss edge cases in your solutions. This demonstrates attention to detail and thorough thinking.
5. Be Open to Feedback
If the interviewer provides suggestions or points out issues in your approach, be receptive to their feedback. Show that you can incorporate new ideas and improve your solution.
6. Show Enthusiasm
Demonstrate genuine interest in the role and the company. Research Oracle’s products and recent developments, and be prepared to discuss why you’re excited about the opportunity.
7. Practice Good Coding Habits
Write clean, well-organized code during the interview. Use meaningful variable names, add comments where necessary, and structure your code logically.
Conclusion
Preparing for an Oracle technical interview requires dedication, consistent practice, and a broad understanding of computer science fundamentals and Oracle-specific technologies. By focusing on the key areas outlined in this guide and following the tips for interview success, you’ll be well-equipped to showcase your skills and land that dream job at Oracle.
Remember, the key to success is not just about having the right answers, but also demonstrating your problem-solving approach, ability to learn, and passion for technology. With thorough preparation and the right mindset, you can confidently tackle your Oracle technical interview and take the next step in your career.
Good luck with your interview preparation, and don’t forget to leverage resources like AlgoCademy to enhance your coding skills and problem-solving abilities. Happy coding, and may your path to Oracle be successful!