Optimizing Algorithms for Mobile Platforms: Enhancing Performance and User Experience
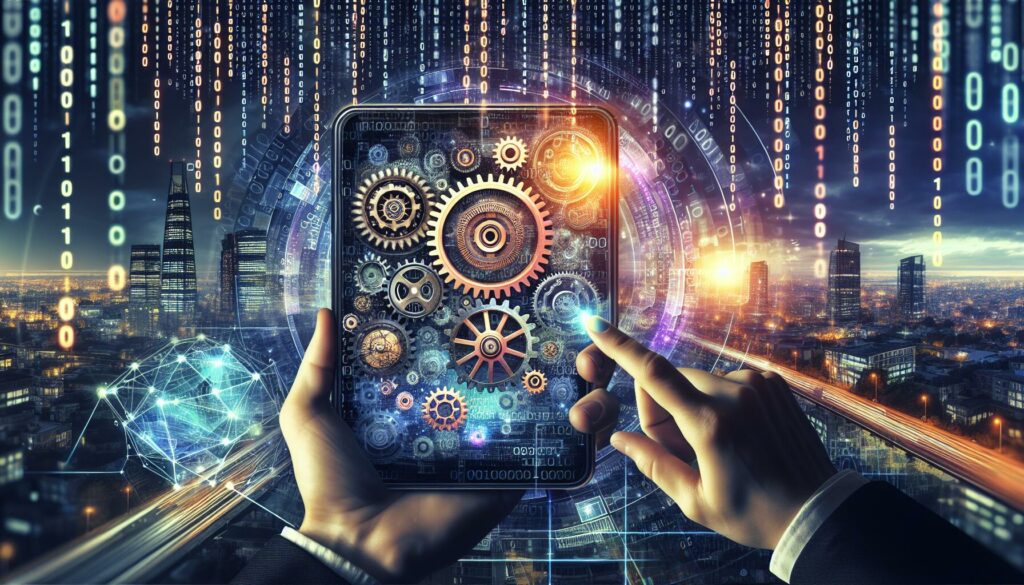
In today’s fast-paced digital world, mobile devices have become an integral part of our daily lives. With the increasing reliance on smartphones and tablets, it’s crucial for developers to optimize their algorithms for mobile platforms. This optimization not only enhances the performance of mobile applications but also significantly improves the user experience. In this comprehensive guide, we’ll explore various strategies and techniques for optimizing algorithms specifically for mobile platforms.
Understanding the Mobile Environment
Before diving into optimization techniques, it’s essential to understand the unique challenges and constraints of the mobile environment:
- Limited processing power compared to desktop computers
- Restricted memory and storage capacity
- Battery life considerations
- Varied network conditions (3G, 4G, 5G, Wi-Fi)
- Diverse screen sizes and resolutions
- Touch-based input mechanisms
These factors significantly influence how we approach algorithm optimization for mobile platforms. Let’s explore some key strategies to address these challenges.
1. Efficient Data Structures
Choosing the right data structures is crucial for optimizing algorithms on mobile platforms. The goal is to minimize memory usage while maintaining fast access times. Here are some considerations:
Use Compact Data Structures
Opt for data structures that have a small memory footprint. For example, use arrays instead of linked lists when possible, as arrays have better cache locality and require less memory overhead.
Implement Custom Data Structures
Sometimes, creating custom data structures tailored to your specific needs can lead to better performance. For instance, if you frequently need to search for elements, consider implementing a hash table or a balanced binary search tree.
Utilize Memory-Efficient Collections
Many programming languages offer specialized collections designed for memory efficiency. In Java, for example, you can use ArrayList
instead of LinkedList
for most scenarios, as it generally performs better on mobile devices.
2. Algorithmic Complexity Optimization
Reducing the time and space complexity of your algorithms is crucial for mobile optimization. Here are some strategies to consider:
Analyze and Improve Time Complexity
Always strive for the lowest possible time complexity. For example, if you’re implementing a sorting algorithm, consider using QuickSort (average-case O(n log n)) instead of Bubble Sort (O(n^2)) for larger datasets.
Space-Time Tradeoffs
In some cases, you may need to balance between time and space complexity. On mobile devices, it’s often preferable to use slightly more memory if it significantly reduces execution time, as long as the memory usage remains within reasonable limits.
Avoid Nested Loops When Possible
Nested loops can quickly lead to poor performance, especially on mobile devices. Look for ways to reduce the number of nested loops or replace them with more efficient algorithms.
3. Caching and Memoization
Implementing caching and memoization techniques can significantly improve the performance of mobile applications, especially for computationally intensive tasks.
Implement Local Caching
Store frequently accessed data or computation results in memory to avoid redundant calculations or network requests. This is particularly useful for data that doesn’t change often.
Use Memoization for Recursive Algorithms
Memoization can dramatically improve the performance of recursive algorithms by storing the results of expensive function calls and returning the cached result when the same inputs occur again. Here’s a simple example in Python:
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
Implement an LRU Cache
For scenarios where you need to limit the amount of cached data, consider implementing a Least Recently Used (LRU) cache. This ensures that the most frequently accessed items are kept in memory while less used items are discarded.
4. Asynchronous Programming and Threading
Leveraging asynchronous programming and multi-threading can significantly improve the responsiveness of mobile applications.
Use Asynchronous Operations
Perform time-consuming operations asynchronously to prevent blocking the main thread. This is especially important for I/O operations, network requests, and database queries. Here’s an example using JavaScript’s async/await:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching data:', error);
}
}
Implement Multi-threading Carefully
While multi-threading can improve performance, it should be used judiciously on mobile devices due to their limited resources. Use thread pools to manage and reuse threads efficiently.
Prioritize Tasks
Implement a task prioritization system to ensure that critical operations are executed first, while less important tasks are deferred or executed in the background.
5. Memory Management
Efficient memory management is crucial for mobile applications, as mobile devices have limited memory resources.
Implement Proper Object Lifecycle Management
Ensure that objects are properly deallocated when they’re no longer needed. In languages with manual memory management, like C++, be diligent about freeing memory. In garbage-collected languages, nullify references to large objects when they’re no longer needed.
Use Weak References
In scenarios where you want to cache objects but allow them to be garbage collected if memory is low, consider using weak references. This is particularly useful in mobile environments where memory constraints are common.
Optimize Image Handling
Images can consume a significant amount of memory. Implement efficient image loading and caching mechanisms, and consider using libraries that can load images at appropriate resolutions for the device’s screen size.
6. Network Optimization
Mobile applications often rely heavily on network operations. Optimizing these operations can significantly improve performance and user experience.
Minimize Network Requests
Reduce the number of network requests by batching operations when possible. This helps conserve battery life and improves performance, especially in areas with poor network connectivity.
Implement Efficient Data Serialization
Use lightweight data formats like JSON or Protocol Buffers for data serialization. These formats are efficient in terms of both processing time and data size.
Implement Offline Functionality
Design your algorithms to work offline when possible. Implement local storage solutions and synchronization mechanisms to allow users to work with the app even when they don’t have an internet connection.
7. Platform-Specific Optimizations
Different mobile platforms (iOS, Android, etc.) have their own unique characteristics and optimization techniques.
Utilize Platform-Specific APIs
Take advantage of platform-specific APIs that are optimized for performance. For example, on iOS, use Grand Central Dispatch (GCD) for efficient concurrent programming:
DispatchQueue.global(qos: .background).async {
// Perform background task
DispatchQueue.main.async {
// Update UI on main thread
}
}
Optimize for Different Screen Sizes
Ensure that your UI algorithms are efficient across various screen sizes and resolutions. Use responsive design techniques and avoid expensive layout calculations when possible.
Leverage Hardware Acceleration
Take advantage of hardware acceleration features provided by mobile platforms. For example, use GPU-accelerated animations and graphics rendering when appropriate.
8. Profiling and Performance Monitoring
Regularly profiling your mobile application is crucial for identifying performance bottlenecks and optimizing algorithms effectively.
Use Platform-Specific Profiling Tools
Utilize profiling tools provided by mobile platforms, such as Xcode Instruments for iOS or Android Profiler for Android. These tools can help you identify memory leaks, CPU usage spikes, and other performance issues.
Implement Custom Performance Metrics
Integrate custom performance tracking into your application to monitor key metrics specific to your app’s functionality. This can help you identify areas that need optimization in real-world usage scenarios.
A/B Testing for Algorithm Variants
When optimizing critical algorithms, consider implementing A/B testing to compare different algorithm variants in real-world conditions. This can help you make data-driven decisions about which optimizations are most effective.
9. Battery Usage Optimization
Optimizing algorithms for battery efficiency is crucial for mobile applications, as users expect their devices to last throughout the day.
Minimize Background Processing
Limit the amount of processing done in the background. Use mobile platform features like background fetch or push notifications to update data efficiently without constantly running processes in the background.
Optimize Location Services Usage
If your app uses location services, implement algorithms that minimize GPS usage. For example, use geofencing instead of continuous location tracking when possible.
Implement Efficient Polling Mechanisms
When your app needs to periodically check for updates, implement efficient polling mechanisms. Use exponential backoff algorithms to reduce the frequency of checks when updates are less likely.
10. Code Optimization Techniques
In addition to algorithmic optimizations, consider these code-level optimization techniques:
Use Compiler Optimizations
Enable compiler optimizations when building your mobile application. Most modern compilers offer various optimization levels that can significantly improve performance.
Implement Lazy Loading
Use lazy loading techniques to defer the initialization of objects until they are needed. This can help reduce memory usage and improve startup time.
Optimize String Operations
String operations can be expensive, especially on mobile devices. Use efficient string manipulation techniques, such as StringBuilder in Java or string interpolation in Swift:
// Swift example of efficient string interpolation
let name = "Alice"
let age = 30
let message = "Hello, my name is \(name) and I'm \(age) years old."
Conclusion
Optimizing algorithms for mobile platforms is a complex but crucial task in today’s mobile-centric world. By focusing on efficient data structures, algorithmic complexity, caching, asynchronous programming, memory management, and platform-specific optimizations, developers can create mobile applications that are not only functional but also performant and user-friendly.
Remember that optimization is an ongoing process. As mobile devices and platforms evolve, so too should your optimization strategies. Regularly profile your application, stay updated with the latest mobile development best practices, and always consider the unique constraints and opportunities presented by mobile platforms.
By applying these optimization techniques, you’ll be well-equipped to create mobile applications that stand out in terms of performance, responsiveness, and user satisfaction. Happy coding!