Object-Oriented Programming: The Cornerstone of Modern Software Development
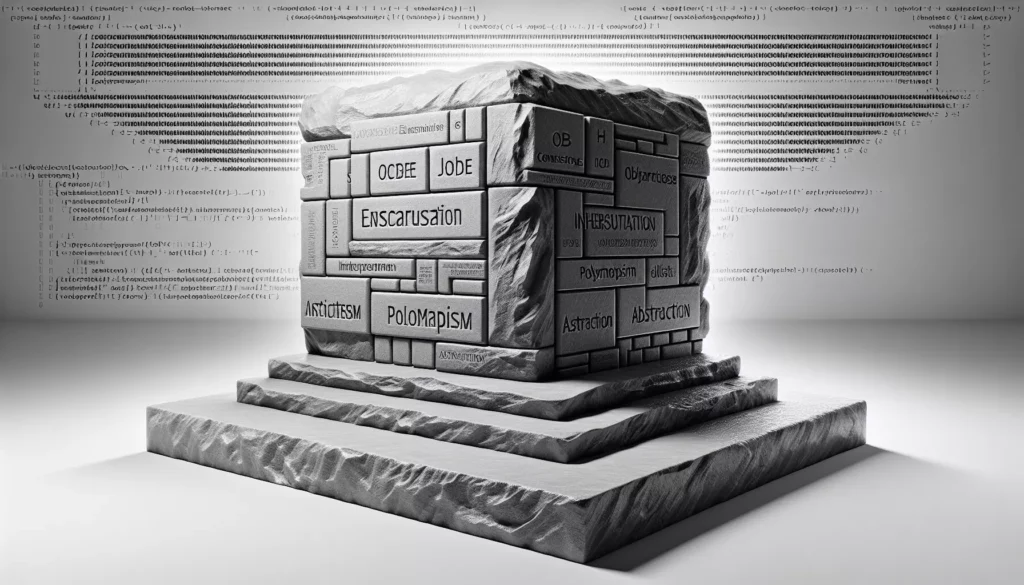
In the vast landscape of programming paradigms, Object-Oriented Programming (OOP) stands out as a pivotal approach that has revolutionized the way we design and develop software. Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at major tech companies, understanding OOP is crucial. In this comprehensive guide, we’ll delve deep into the world of Object-Oriented Programming, exploring its core concepts, benefits, and real-world applications.
What is Object-Oriented Programming?
Object-Oriented Programming is a programming paradigm that organizes software design around data, or objects, rather than functions and logic. An object is a data field that has unique attributes and behavior. OOP focuses on the objects that developers want to manipulate rather than the logic required to manipulate them.
This approach to programming is particularly well-suited to large, complex, and actively updated or maintained programs. It allows for more efficient code reuse and easier scalability compared to other programming paradigms.
The Four Pillars of OOP
To truly understand Object-Oriented Programming, it’s essential to grasp its four fundamental principles:
1. Encapsulation
Encapsulation is the bundling of data and the methods that operate on that data within a single unit or object. This concept is used to hide the internal representation, or state, of an object from the outside. This is called information hiding.
Example in Python:
class BankAccount:
def __init__(self):
self.__balance = 0 # Private attribute
def deposit(self, amount):
if amount > 0:
self.__balance += amount
return True
return False
def get_balance(self):
return self.__balance
account = BankAccount()
account.deposit(100)
print(account.get_balance()) # Output: 100
# print(account.__balance) # This would raise an AttributeError
In this example, the balance is encapsulated within the BankAccount class, and access to it is controlled through methods.
2. Abstraction
Abstraction is the process of hiding the complex reality while exposing only the necessary parts. It helps in reducing programming complexity and effort.
Example in Java:
abstract class Shape {
abstract double area();
}
class Circle extends Shape {
double radius;
Circle(double r) {
radius = r;
}
@Override
double area() {
return Math.PI * radius * radius;
}
}
class Rectangle extends Shape {
double length;
double width;
Rectangle(double l, double w) {
length = l;
width = w;
}
@Override
double area() {
return length * width;
}
}
Here, the Shape class provides an abstraction that is implemented by Circle and Rectangle.
3. Inheritance
Inheritance is a mechanism where you can to derive a class from another class for a hierarchy of classes that share a set of attributes and methods.
Example in C++:
class Animal {
public:
void eat() {
cout << "I can eat!" << endl;
}
};
class Dog : public Animal {
public:
void bark() {
cout << "I can bark! Woof woof!" << endl;
}
};
int main() {
Dog dog1;
dog1.eat();
dog1.bark();
return 0;
}
In this example, Dog inherits the eat() method from Animal and adds its own bark() method.
4. Polymorphism
Polymorphism allows objects of different classes to be treated as objects of a common super class. The most common use of polymorphism in OOP occurs when a parent class reference is used to refer to a child class object.
Example in Python:
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
def animal_sound(animal):
print(animal.speak())
dog = Dog()
cat = Cat()
animal_sound(dog) # Output: Woof!
animal_sound(cat) # Output: Meow!
Here, animal_sound() function can work with any Animal object, demonstrating polymorphism.
Why is Object-Oriented Programming Important?
OOP has become a cornerstone of modern software development for several compelling reasons:
1. Modularity
OOP allows you to break down your software into bite-sized problems that you can solve one at a time. This makes development more manageable and less error-prone.
2. Reusability
Through inheritance, you can reuse code from existing classes when you create new classes. This saves time and effort in development.
3. Flexibility and Extensibility
You can extend your application with new attributes and methods, or you can override existing ones. This makes your code more flexible and easier to maintain over time.
4. Data Security
The principle of encapsulation helps in data hiding, which enhances the security of data by restricting access to it.
5. Real-World Mapping
OOP models complex things as reproducible, simple structures. This allows for real-world situations to be reflected in code, making the design process more intuitive.
6. Collaboration
OOP’s modular structure allows different developers to work on different parts of the same program simultaneously, making it ideal for collaborative projects.
OOP in Practice: A Real-World Example
Let’s consider a practical example of how OOP might be used in a real-world scenario. Imagine we’re designing a simple library management system.
class Book:
def __init__(self, title, author, isbn):
self.title = title
self.author = author
self.isbn = isbn
self.is_checked_out = False
def check_out(self):
if not self.is_checked_out:
self.is_checked_out = True
return True
return False
def return_book(self):
if self.is_checked_out:
self.is_checked_out = False
return True
return False
class Library:
def __init__(self):
self.books = []
def add_book(self, book):
self.books.append(book)
def find_book(self, isbn):
for book in self.books:
if book.isbn == isbn:
return book
return None
class Member:
def __init__(self, name, member_id):
self.name = name
self.member_id = member_id
self.checked_out_books = []
def check_out_book(self, book):
if book.check_out():
self.checked_out_books.append(book)
return True
return False
def return_book(self, book):
if book.return_book():
self.checked_out_books.remove(book)
return True
return False
# Usage
library = Library()
book1 = Book("1984", "George Orwell", "9780451524935")
book2 = Book("To Kill a Mockingbird", "Harper Lee", "9780446310789")
library.add_book(book1)
library.add_book(book2)
member = Member("John Doe", "12345")
book_to_checkout = library.find_book("9780451524935")
if book_to_checkout:
if member.check_out_book(book_to_checkout):
print(f"{member.name} checked out {book_to_checkout.title}")
else:
print("Book is already checked out")
else:
print("Book not found")
This example demonstrates how OOP principles can be applied to model a real-world system. The Library, Book, and Member classes encapsulate related data and behavior. Inheritance could be used to create different types of books or library members. Polymorphism could be applied if we wanted to handle different types of media (books, DVDs, etc.) in a uniform way.
OOP and Technical Interviews
For those preparing for technical interviews, especially at major tech companies often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), understanding OOP is crucial. Here’s why:
1. System Design Questions
Many technical interviews include system design questions where you’re asked to design a complex system. OOP principles are invaluable for breaking down these problems and designing modular, extensible solutions.
2. Code Organization
Interviewers often look at how well you organize your code. Using OOP principles demonstrates your ability to write clean, maintainable code.
3. Problem-Solving Approach
OOP encourages a particular approach to problem-solving – breaking down complex problems into smaller, manageable parts. This approach is highly valued in technical interviews.
4. Language Proficiency
Most modern programming languages used in technical interviews (like Java, Python, C++) are object-oriented. Demonstrating a strong grasp of OOP shows proficiency in these languages.
5. Real-World Relevance
Many interview questions are designed to mimic real-world scenarios. OOP’s ability to model real-world entities makes it particularly useful for these types of questions.
Common OOP Interview Questions
Here are some common OOP-related questions you might encounter in a technical interview:
- Explain the difference between composition and inheritance.
- What is method overloading and method overriding?
- Explain the concept of abstraction in OOP.
- What is the difference between an interface and an abstract class?
- How does encapsulation contribute to data hiding?
- Explain the concept of polymorphism with an example.
- What are the SOLID principles in OOP?
- How does OOP support code reusability?
- What is the difference between static and instance methods?
- Explain the concept of coupling in OOP and why low coupling is desirable.
Challenges in OOP
While Object-Oriented Programming offers many benefits, it’s also important to be aware of its challenges:
1. Steep Learning Curve
OOP concepts can be challenging for beginners to grasp, especially when coming from a procedural programming background.
2. Program Size
OOP programs can be larger than procedural programs, as they require more lines of code to set up classes and their relationships.
3. Performance Overhead
The abstraction and encapsulation in OOP can sometimes lead to slower program execution compared to procedural code.
4. Design Complexity
Designing a good object-oriented system is challenging and requires experience. Poor design can lead to overly complex and hard-to-maintain code.
OOP vs. Other Programming Paradigms
While OOP is widely used, it’s not the only programming paradigm. Let’s briefly compare it with some others:
Procedural Programming
Procedural programming is based on the concept of procedure calls. Unlike OOP, it doesn’t bundle data and methods together. While it can be simpler for small programs, it becomes harder to manage as programs grow larger and more complex.
Functional Programming
Functional programming treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. It can lead to more predictable and easier to test code, but can be less intuitive for modeling real-world objects and processes.
Event-Driven Programming
This paradigm is based on events and triggers. It’s commonly used in graphical user interfaces and real-time systems. While it’s great for reactive systems, it can be harder to understand the flow of the program compared to OOP.
The Future of OOP
As we look to the future, Object-Oriented Programming continues to evolve and adapt to new challenges in software development:
1. Integration with Other Paradigms
Many modern programming languages and frameworks are adopting a multi-paradigm approach, combining OOP with functional programming and other paradigms to leverage the strengths of each.
2. OOP in Distributed Systems
With the rise of microservices and distributed systems, OOP principles are being adapted to work effectively in these environments.
3. OOP and AI
As artificial intelligence and machine learning become more prevalent, OOP is being used to create more intuitive and manageable AI systems.
4. OOP in Low-Code Platforms
Low-code and no-code platforms are increasingly using OOP principles to allow non-programmers to create complex applications.
Conclusion
Object-Oriented Programming is more than just a programming paradigm; it’s a way of thinking about software design and problem-solving. Its principles of encapsulation, abstraction, inheritance, and polymorphism provide a powerful toolkit for creating robust, maintainable, and scalable software systems.
For those on the journey of learning to code or preparing for technical interviews, mastering OOP is an essential step. It not only helps in writing better code but also in understanding complex systems and designing elegant solutions to real-world problems.
As you continue your coding education and skills development, remember that OOP is not just about syntax or specific languages. It’s about cultivating a mindset that approaches problems by breaking them down into manageable, interacting objects. This mindset, combined with practice and real-world application, will serve you well whether you’re building your first program or designing systems at a major tech company.
Keep exploring, keep practicing, and most importantly, keep thinking in objects!