Object-Oriented Design in Coding Interviews: A Comprehensive Guide
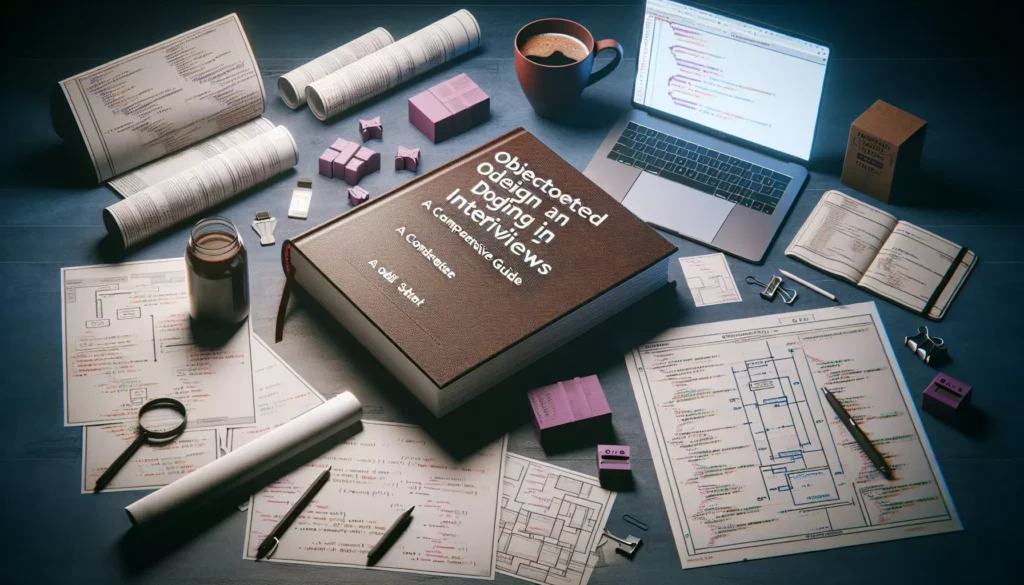
In the world of software development, object-oriented design (OOD) is a fundamental concept that plays a crucial role in creating robust, scalable, and maintainable code. As you prepare for coding interviews, particularly those at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google), understanding and applying OOD principles can significantly boost your chances of success. This comprehensive guide will delve into the intricacies of object-oriented design in the context of coding interviews, providing you with the knowledge and skills needed to excel in this critical area.
What is Object-Oriented Design?
Object-Oriented Design is a programming paradigm that organizes software design around data, or objects, rather than functions and logic. It focuses on the objects that developers want to manipulate rather than the logic required to manipulate them. This approach to software design has several key advantages:
- Modularity: OOD allows for the separation of a program into distinct, independent modules.
- Reusability: Objects can be reused across different parts of a program or even in different projects.
- Scalability: OOD makes it easier to scale applications by adding new objects without disrupting existing code.
- Maintainability: The modular nature of OOD makes it easier to maintain and update code over time.
Core Principles of Object-Oriented Design
To effectively apply OOD in coding interviews, it’s essential to understand and internalize its core principles. These principles, often referred to as the SOLID principles, provide a foundation for creating flexible and maintainable software designs:
1. Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. In other words, a class should have a single, well-defined responsibility. This principle promotes modularity and makes code easier to understand and maintain.
Example:
// Bad design
class User {
public void saveUser() { /* ... */ }
public void sendEmail() { /* ... */ }
}
// Good design
class User {
public void saveUser() { /* ... */ }
}
class EmailService {
public void sendEmail() { /* ... */ }
}
2. Open-Closed Principle (OCP)
The Open-Closed Principle states that software entities (classes, modules, functions, etc.) should be open for extension but closed for modification. This means you should be able to extend a class’s behavior without modifying its existing code.
Example:
// Bad design
class Rectangle {
public double width;
public double height;
}
class AreaCalculator {
public double calculateArea(Rectangle rectangle) {
return rectangle.width * rectangle.height;
}
}
// Good design
interface Shape {
double calculateArea();
}
class Rectangle implements Shape {
private double width;
private double height;
public double calculateArea() {
return width * height;
}
}
class Circle implements Shape {
private double radius;
public double calculateArea() {
return Math.PI * radius * radius;
}
}
3. Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program. In other words, derived classes must be substitutable for their base classes.
Example:
// Bad design
class Bird {
public void fly() { /* ... */ }
}
class Penguin extends Bird {
@Override
public void fly() {
throw new UnsupportedOperationException("Penguins can't fly");
}
}
// Good design
interface FlyingBird {
void fly();
}
class Sparrow implements FlyingBird {
public void fly() { /* ... */ }
}
class Penguin {
// Penguin doesn't implement FlyingBird
}
4. Interface Segregation Principle (ISP)
The Interface Segregation Principle states that clients should not be forced to depend on interfaces they do not use. It’s better to have many smaller, specific interfaces rather than a few large, general-purpose ones.
Example:
// Bad design
interface Worker {
void work();
void eat();
}
// Good design
interface Workable {
void work();
}
interface Eatable {
void eat();
}
class Human implements Workable, Eatable {
public void work() { /* ... */ }
public void eat() { /* ... */ }
}
class Robot implements Workable {
public void work() { /* ... */ }
}
5. Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules; both should depend on abstractions. Abstractions should not depend on details; details should depend on abstractions.
Example:
// Bad design
class LightBulb {
public void turnOn() { /* ... */ }
public void turnOff() { /* ... */ }
}
class Switch {
private LightBulb bulb;
public Switch() {
bulb = new LightBulb();
}
public void operate() {
// ...
}
}
// Good design
interface Switchable {
void turnOn();
void turnOff();
}
class LightBulb implements Switchable {
public void turnOn() { /* ... */ }
public void turnOff() { /* ... */ }
}
class Switch {
private Switchable device;
public Switch(Switchable device) {
this.device = device;
}
public void operate() {
// ...
}
}
Applying OOD Principles in Coding Interviews
When faced with an object-oriented design question in a coding interview, it’s crucial to approach the problem systematically. Here’s a step-by-step guide to help you tackle OOD problems effectively:
1. Clarify Requirements
Begin by asking questions to clarify the requirements and constraints of the problem. This will help you understand the scope of the design and identify any potential edge cases or special considerations.
2. Identify Key Objects and Relationships
Analyze the problem statement and identify the main objects or entities involved. Determine how these objects relate to each other and what responsibilities each object should have.
3. Define Class Hierarchies and Interfaces
Based on the identified objects and their relationships, define appropriate class hierarchies and interfaces. Consider using inheritance, composition, and interfaces to create a flexible and extensible design.
4. Apply SOLID Principles
As you develop your design, consciously apply the SOLID principles to ensure your solution is modular, extensible, and maintainable. Be prepared to explain how your design adheres to these principles.
5. Consider Design Patterns
Evaluate whether any common design patterns can be applied to solve specific aspects of the problem. Familiarity with design patterns can help you quickly identify and implement proven solutions to common design challenges.
6. Implement Core Functionality
Start implementing the core functionality of your design. Focus on the most critical components first, and be prepared to explain your implementation choices.
7. Discuss Trade-offs and Alternatives
As you present your solution, be prepared to discuss any trade-offs in your design and potential alternative approaches. This demonstrates your ability to think critically about design decisions and consider different perspectives.
Common OOD Interview Questions and Examples
To help you prepare for object-oriented design questions in coding interviews, let’s explore some common types of problems you might encounter, along with example solutions:
1. Design a Parking Lot
This is a classic OOD interview question that tests your ability to model real-world systems using object-oriented principles.
Key considerations:
- Different types of vehicles (cars, motorcycles, buses)
- Multiple levels in the parking lot
- Payment system
- Capacity management
Example solution outline:
interface Vehicle {
String getType();
int getSize();
}
class Car implements Vehicle {
// Implementation
}
class Motorcycle implements Vehicle {
// Implementation
}
class Bus implements Vehicle {
// Implementation
}
class ParkingSpot {
private Vehicle vehicle;
private int spotNumber;
private boolean isOccupied;
// Methods to park and remove vehicles
}
class ParkingLevel {
private List<ParkingSpot> spots;
private int levelNumber;
// Methods to find available spots, park vehicles, etc.
}
class ParkingLot {
private List<ParkingLevel> levels;
private PaymentSystem paymentSystem;
// Methods to manage parking operations
}
class PaymentSystem {
// Methods to handle payments
}
2. Design a Library Management System
This question tests your ability to model a system with multiple user types, resources, and operations.
Key considerations:
- Different types of library items (books, magazines, DVDs)
- User management (members, librarians)
- Borrowing and returning processes
- Search functionality
Example solution outline:
interface LibraryItem {
String getTitle();
String getUniqueId();
boolean isAvailable();
}
class Book implements LibraryItem {
// Implementation
}
class Magazine implements LibraryItem {
// Implementation
}
class DVD implements LibraryItem {
// Implementation
}
abstract class User {
protected String name;
protected String id;
// Common user methods
}
class Member extends User {
private List<LibraryItem> borrowedItems;
// Methods for borrowing, returning items
}
class Librarian extends User {
// Methods for managing library operations
}
class Library {
private List<LibraryItem> items;
private List<User> users;
// Methods for search, user management, etc.
}
class BorrowingSystem {
// Methods to handle borrowing and returning processes
}
class SearchCatalog {
// Methods to search for library items
}
3. Design a Restaurant Ordering System
This question tests your ability to design a system with multiple interacting components and workflows.
Key considerations:
- Menu management
- Order processing
- Table management
- Payment handling
Example solution outline:
class MenuItem {
private String name;
private double price;
private String category;
// Getters and setters
}
class Menu {
private List<MenuItem> items;
// Methods to add, remove, and update menu items
}
class Order {
private List<MenuItem> orderedItems;
private Table table;
private OrderStatus status;
// Methods to add items, calculate total, etc.
}
enum OrderStatus {
PLACED, PREPARING, READY, SERVED, PAID
}
class Table {
private int tableNumber;
private int capacity;
private boolean isOccupied;
// Methods to manage table status
}
class Restaurant {
private Menu menu;
private List<Table> tables;
private List<Order> activeOrders;
// Methods to manage restaurant operations
}
class PaymentSystem {
// Methods to handle payments
}
class KitchenManagement {
// Methods to manage food preparation
}
Best Practices for OOD in Coding Interviews
To excel in object-oriented design questions during coding interviews, keep these best practices in mind:
- Start with a high-level overview: Begin by sketching out the main components and their relationships before diving into implementation details.
- Use appropriate abstractions: Leverage interfaces and abstract classes to create flexible and extensible designs.
- Follow the SOLID principles: Continuously evaluate your design against the SOLID principles and be prepared to explain how your solution adheres to them.
- Consider scalability: Design your solution with future growth in mind, making it easy to add new features or modify existing ones.
- Prioritize readability: Use clear naming conventions and organize your code logically to enhance readability and maintainability.
- Be prepared to iterate: Be open to feedback and ready to refine your design based on new requirements or constraints introduced by the interviewer.
- Discuss trade-offs: Acknowledge the pros and cons of your design decisions and be prepared to discuss alternative approaches.
- Practice, practice, practice: Regularly work on OOD problems to sharpen your skills and become more comfortable with different types of design challenges.
Conclusion
Object-oriented design is a crucial skill for software developers, particularly when preparing for coding interviews at top tech companies. By understanding and applying the principles of OOD, you can create flexible, maintainable, and scalable software designs that will impress interviewers and set you apart from other candidates.
Remember that mastering OOD is an ongoing process that requires practice and continuous learning. As you prepare for your coding interviews, make sure to work on a variety of OOD problems, study common design patterns, and stay up-to-date with best practices in software design. With dedication and consistent effort, you’ll be well-equipped to tackle even the most challenging object-oriented design questions in your upcoming interviews.
Good luck with your interview preparation, and may your object-oriented designs be as elegant and efficient as the code you write!