Node.js for Back-End Development: A Comprehensive Guide
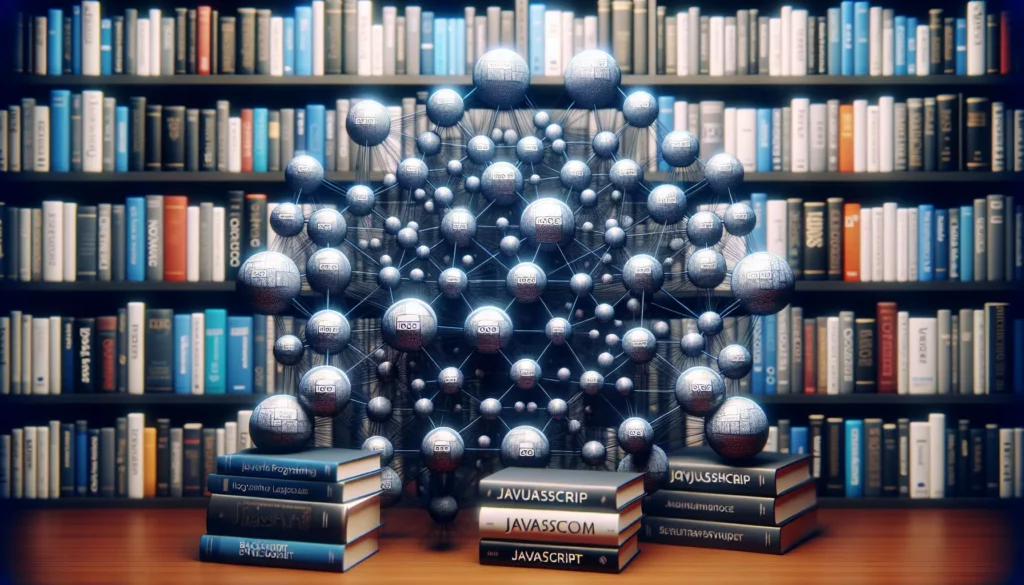
In the ever-evolving landscape of web development, Node.js has emerged as a powerful tool for building scalable and efficient back-end applications. Whether you’re a beginner looking to expand your coding skills or an experienced developer aiming to diversify your tech stack, understanding Node.js is crucial in today’s programming world. This comprehensive guide will walk you through what Node.js is, its key features, and how to use it effectively for back-end development.
What is Node.js?
Node.js is an open-source, cross-platform JavaScript runtime environment that executes JavaScript code outside of a web browser. It was created by Ryan Dahl in 2009 and has since become one of the most popular tools for server-side programming.
At its core, Node.js uses the V8 JavaScript engine, the same engine that powers Google Chrome. This allows developers to use JavaScript for both client-side and server-side scripting, creating a unified language experience across the full stack.
Key Features of Node.js
- Asynchronous and Event-Driven: Node.js uses non-blocking, event-driven architecture, making it efficient and lightweight.
- Fast Execution: The V8 engine compiles JavaScript into native machine code, ensuring high performance.
- Single-Threaded but Highly Scalable: It can handle a large number of simultaneous connections with high throughput.
- NPM (Node Package Manager): A vast ecosystem of open-source libraries and tools.
- Cross-Platform: Runs on various platforms (Windows, Linux, Unix, Mac OS X, etc.).
Why Use Node.js for Back-End Development?
Node.js has several advantages that make it an excellent choice for back-end development:
- JavaScript Everywhere: Use the same language on both front-end and back-end, simplifying development and maintenance.
- High Performance: Non-blocking I/O and event-driven architecture make it ideal for real-time applications.
- Large and Active Community: Extensive support, frequent updates, and a wealth of resources.
- Rich Ecosystem: NPM provides access to hundreds of thousands of reusable packages.
- Microservices Architecture: Well-suited for building scalable microservices.
- Corporate Backing: Supported by companies like Microsoft, IBM, and Netflix.
Getting Started with Node.js
1. Installation
To begin using Node.js, you first need to install it on your system. Visit the official Node.js website (https://nodejs.org/) and download the version suitable for your operating system. The installation process is straightforward:
- For Windows and macOS: Download the installer and follow the installation wizard.
- For Linux: Use your distribution’s package manager or follow the instructions on the Node.js website.
After installation, you can verify it by opening a terminal or command prompt and typing:
node --version
npm --version
These commands should display the installed versions of Node.js and npm respectively.
2. Creating Your First Node.js Application
Let’s create a simple “Hello, World!” application to get started:
- Create a new file named
app.js
. - Open the file in your preferred text editor and add the following code:
console.log("Hello, World!");
- Save the file and run it using Node.js by opening a terminal in the same directory and typing:
node app.js
You should see “Hello, World!” printed in the console.
3. Understanding Node.js Modules
Node.js uses a modular system to organize and reuse code. There are three types of modules:
- Core Modules: Built-in modules that come with Node.js installation.
- Local Modules: Modules you create for your application.
- Third-party Modules: Modules installed via npm.
Here’s an example of using a core module (the ‘http’ module) to create a simple web server:
const http = require('http');
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello, World!');
});
server.listen(3000, 'localhost', () => {
console.log('Server running at http://localhost:3000/');
});
Save this code in a file (e.g., server.js
) and run it with Node.js. You’ll have a web server running on http://localhost:3000
.
Node.js for Back-End Development: Key Concepts
1. Asynchronous Programming
One of Node.js’s core strengths is its asynchronous, non-blocking nature. This is achieved through callbacks, promises, and async/await syntax.
Callbacks:
const fs = require('fs');
fs.readFile('example.txt', 'utf8', (err, data) => {
if (err) {
console.error('Error reading file:', err);
return;
}
console.log(data);
});
Promises:
const fs = require('fs').promises;
fs.readFile('example.txt', 'utf8')
.then(data => console.log(data))
.catch(err => console.error('Error reading file:', err));
Async/Await:
const fs = require('fs').promises;
async function readFile() {
try {
const data = await fs.readFile('example.txt', 'utf8');
console.log(data);
} catch (err) {
console.error('Error reading file:', err);
}
}
readFile();
2. Event-Driven Programming
Node.js uses an event-driven architecture, which is central to how it handles asynchronous operations. The EventEmitter class is at the core of this model:
const EventEmitter = require('events');
class MyEmitter extends EventEmitter {}
const myEmitter = new MyEmitter();
myEmitter.on('event', () => {
console.log('An event occurred!');
});
myEmitter.emit('event');
3. Streams
Streams are a powerful feature in Node.js for handling reading/writing files, network communications, or any kind of end-to-end information exchange efficiently.
const fs = require('fs');
const server = require('http').createServer();
server.on('request', (req, res) => {
const src = fs.createReadStream('./big.file');
src.pipe(res);
});
server.listen(8000);
This example creates a server that streams a large file to the client, which is much more memory-efficient than reading the entire file into memory.
4. Express.js: A Popular Node.js Framework
While Node.js provides a solid foundation for back-end development, many developers use frameworks to streamline their work. Express.js is one of the most popular:
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(port, () => {
console.log(`Example app listening at http://localhost:${port}`);
});
This creates a simple Express server that responds with “Hello World!” when you visit the root URL.
Building RESTful APIs with Node.js
RESTful APIs are a common use case for Node.js back-end development. Here’s a basic example using Express:
const express = require('express');
const app = express();
const port = 3000;
// Middleware to parse JSON bodies
app.use(express.json());
let users = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Doe' }
];
// GET all users
app.get('/users', (req, res) => {
res.json(users);
});
// GET a specific user
app.get('/users/:id', (req, res) => {
const user = users.find(u => u.id === parseInt(req.params.id));
if (!user) return res.status(404).send('User not found');
res.json(user);
});
// POST a new user
app.post('/users', (req, res) => {
const user = {
id: users.length + 1,
name: req.body.name
};
users.push(user);
res.status(201).json(user);
});
app.listen(port, () => {
console.log(`API server listening at http://localhost:${port}`);
});
This example sets up a basic CRUD (Create, Read, Update, Delete) API for a user resource.
Database Integration
Most back-end applications require database integration. Node.js can work with various databases, both SQL and NoSQL. Here’s an example using MongoDB with the Mongoose ODM:
const mongoose = require('mongoose');
const express = require('express');
const app = express();
mongoose.connect('mongodb://localhost/myapp', { useNewUrlParser: true, useUnifiedTopology: true });
const User = mongoose.model('User', {
name: String,
email: String
});
app.use(express.json());
app.post('/users', async (req, res) => {
const user = new User(req.body);
await user.save();
res.status(201).json(user);
});
app.get('/users', async (req, res) => {
const users = await User.find();
res.json(users);
});
app.listen(3000, () => console.log('Server started'));
This example demonstrates how to connect to a MongoDB database, define a model, and perform basic CRUD operations.
Testing in Node.js
Testing is crucial in back-end development. Node.js has several testing frameworks available, with Jest and Mocha being popular choices. Here’s a simple example using Jest:
// math.js
function add(a, b) {
return a + b;
}
module.exports = { add };
// math.test.js
const { add } = require('./math');
test('adds 1 + 2 to equal 3', () => {
expect(add(1, 2)).toBe(3);
});
To run this test, you would typically add a script to your package.json
:
{
"scripts": {
"test": "jest"
}
}
Then run npm test
in your terminal.
Deployment and Scaling
When it comes to deploying Node.js applications, there are several options:
- Platform as a Service (PaaS): Services like Heroku, Google App Engine, or Azure App Service make deployment simple.
- Container Orchestration: Docker and Kubernetes are popular for containerizing and managing Node.js applications.
- Serverless: Platforms like AWS Lambda or Google Cloud Functions allow you to run Node.js code without managing servers.
For scaling Node.js applications, consider:
- Load Balancing: Distribute incoming network traffic across multiple servers.
- Clustering: Use the Node.js cluster module to run multiple instances of your application on a single server.
- Caching: Implement caching strategies to reduce database load and improve response times.
- Database Optimization: Use database indexing, query optimization, and possibly sharding for large-scale applications.
Security Considerations
When developing back-end applications with Node.js, security should be a top priority. Some key security practices include:
- Input Validation: Always validate and sanitize user inputs to prevent injection attacks.
- Authentication and Authorization: Implement robust user authentication and proper authorization checks.
- HTTPS: Use HTTPS to encrypt data in transit.
- Dependencies Management: Regularly update your dependencies and use tools like npm audit to check for vulnerabilities.
- Environment Variables: Use environment variables for sensitive information like API keys and database credentials.
- Rate Limiting: Implement rate limiting to prevent abuse of your API.
Conclusion
Node.js has revolutionized back-end development by allowing developers to use JavaScript across the entire stack. Its event-driven, non-blocking I/O model makes it particularly well-suited for building scalable network applications.
As you continue your journey with Node.js, remember that practice is key. Start with small projects and gradually build up to more complex applications. Explore the vast ecosystem of npm packages, but also take the time to understand core Node.js concepts.
Whether you’re building a simple API or a complex microservices architecture, Node.js provides the tools and flexibility to bring your ideas to life. As you grow more comfortable with Node.js, you’ll find it opens up a world of possibilities in back-end development.
Keep learning, stay curious, and happy coding!