Navigating Maze and Grid-Based Coding Problems: A Comprehensive Guide
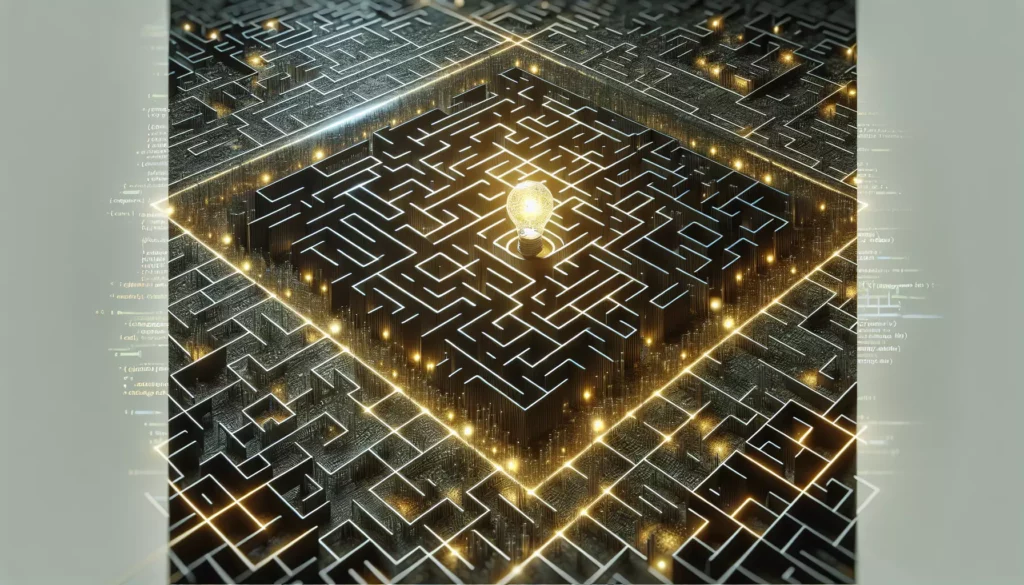
Welcome to our in-depth guide on navigating maze and grid-based coding problems. These types of challenges are common in technical interviews, especially for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). Whether you’re a beginner looking to improve your coding skills or an experienced programmer preparing for a big interview, this article will provide you with valuable insights and strategies to tackle these problems effectively.
Table of Contents
- Introduction to Maze and Grid-Based Problems
- Common Types of Maze and Grid Problems
- Representing Mazes and Grids in Code
- Basic Traversal Techniques
- Pathfinding Algorithms
- Optimization Techniques
- Advanced Concepts and Variations
- Real-World Applications
- Practice Problems and Solutions
- Conclusion
1. Introduction to Maze and Grid-Based Problems
Maze and grid-based problems are a subset of algorithmic challenges that involve navigating through a two-dimensional structure. These problems test a programmer’s ability to think spatially, implement traversal algorithms, and optimize solutions for efficiency. They are popular in coding interviews because they can be easily scaled in complexity and often have real-world applications in areas such as robotics, game development, and network routing.
The basic premise of these problems usually involves:
- A grid or maze represented as a 2D array or matrix
- A starting point and one or more goal points
- Obstacles or walls that restrict movement
- A set of rules for movement (e.g., up, down, left, right)
The objective can vary from simply finding a path to the goal, to optimizing for the shortest path, or solving more complex scenarios like collecting items or avoiding enemies.
2. Common Types of Maze and Grid Problems
Let’s explore some of the most frequently encountered maze and grid-based problem types:
2.1 Path Finding
The most basic type of problem involves finding any valid path from a start point to an end point. This can be solved using simple traversal algorithms like Depth-First Search (DFS) or Breadth-First Search (BFS).
2.2 Shortest Path
An extension of the basic path finding problem, this requires finding the path with the least number of steps or lowest cost. Algorithms like Dijkstra’s or A* are commonly used for these problems.
2.3 Maze Generation
Instead of solving a maze, these problems require creating a maze with certain properties. This tests understanding of randomization and graph theory.
2.4 Area Calculation
These problems involve calculating the area of enclosed regions within a grid. Flood fill algorithms are often used in these scenarios.
2.5 Multi-Agent Pathfinding
More complex scenarios involve finding paths for multiple agents simultaneously, often with the requirement that they don’t collide.
2.6 Dynamic Mazes
These challenging problems involve mazes that change over time, requiring algorithms that can adapt to changing conditions.
3. Representing Mazes and Grids in Code
Before we dive into solving these problems, it’s crucial to understand how to represent mazes and grids in code. The most common representations are:
3.1 2D Arrays
The simplest and most intuitive representation is using a 2D array or matrix. Each cell in the grid is represented by an element in the array. For example:
maze = [
[0, 0, 1, 0, 0],
[1, 0, 0, 0, 1],
[0, 0, 1, 0, 0],
[0, 1, 0, 0, 1],
[0, 0, 0, 1, 0]
]
In this representation, 0 might represent an open path, while 1 represents a wall or obstacle.
3.2 Graph Representation
For more complex mazes or when dealing with weighted edges, a graph representation might be more appropriate. Each cell becomes a node, and connections between cells are edges. This can be implemented using an adjacency list or adjacency matrix.
graph = {
(0,0): [(0,1), (1,0)],
(0,1): [(0,0), (0,2), (1,1)],
# ... more nodes and their connections
}
3.3 Object-Oriented Representation
For more complex scenarios, an object-oriented approach might be beneficial. Each cell can be an object with properties like position, type (wall, path, start, end), and methods for checking neighbors.
class Cell:
def __init__(self, x, y, cell_type):
self.x = x
self.y = y
self.type = cell_type
self.neighbors = []
def add_neighbor(self, neighbor):
self.neighbors.append(neighbor)
# Creating the maze
maze = [[Cell(x, y, cell_type) for y, cell_type in enumerate(row)] for x, row in enumerate(maze_layout)]
4. Basic Traversal Techniques
Once we have our maze or grid represented in code, we can start implementing traversal algorithms. The two fundamental traversal techniques are Depth-First Search (DFS) and Breadth-First Search (BFS).
4.1 Depth-First Search (DFS)
DFS explores as far as possible along each branch before backtracking. It’s implemented using a stack (or recursion) and is memory-efficient but may not find the shortest path.
def dfs(maze, start, end):
stack = [start]
visited = set()
while stack:
current = stack.pop()
if current == end:
return True # Path found
if current in visited:
continue
visited.add(current)
for neighbor in get_neighbors(maze, current):
if neighbor not in visited:
stack.append(neighbor)
return False # No path found
def get_neighbors(maze, cell):
x, y = cell
neighbors = []
for dx, dy in [(0,1), (1,0), (0,-1), (-1,0)]: # Right, Down, Left, Up
nx, ny = x + dx, y + dy
if 0 <= nx < len(maze) and 0 <= ny < len(maze[0]) and maze[nx][ny] == 0:
neighbors.append((nx, ny))
return neighbors
4.2 Breadth-First Search (BFS)
BFS explores all neighbor nodes at the present depth before moving to nodes at the next depth level. It uses a queue and is guaranteed to find the shortest path in an unweighted graph.
from collections import deque
def bfs(maze, start, end):
queue = deque([start])
visited = set([start])
while queue:
current = queue.popleft()
if current == end:
return True # Path found
for neighbor in get_neighbors(maze, current):
if neighbor not in visited:
queue.append(neighbor)
visited.add(neighbor)
return False # No path found
# get_neighbors function remains the same as in DFS
5. Pathfinding Algorithms
While DFS and BFS are great for basic traversal, more sophisticated algorithms are often needed for finding optimal paths, especially in weighted graphs or when dealing with heuristics.
5.1 Dijkstra’s Algorithm
Dijkstra’s algorithm finds the shortest path between nodes in a graph, which may represent, for example, road networks. It’s particularly useful when edges have different weights.
import heapq
def dijkstra(graph, start, end):
distances = {node: float('infinity') for node in graph}
distances[start] = 0
pq = [(0, start)]
previous = {node: None for node in graph}
while pq:
current_distance, current_node = heapq.heappop(pq)
if current_node == end:
path = []
while current_node:
path.append(current_node)
current_node = previous[current_node]
return path[::-1]
if current_distance > distances[current_node]:
continue
for neighbor, weight in graph[current_node].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
previous[neighbor] = current_node
heapq.heappush(pq, (distance, neighbor))
return None # No path found
5.2 A* Algorithm
A* is a best-first search algorithm that uses heuristics to guide its search. It’s often faster than Dijkstra’s algorithm for many problems and is widely used in pathfinding and graph traversal.
import heapq
def manhattan_distance(a, b):
return abs(b[0] - a[0]) + abs(b[1] - a[1])
def a_star(maze, start, end):
heap = [(0, start)]
g_score = {start: 0}
f_score = {start: manhattan_distance(start, end)}
came_from = {}
while heap:
current = heapq.heappop(heap)[1]
if current == end:
path = []
while current in came_from:
path.append(current)
current = came_from[current]
return path[::-1]
for neighbor in get_neighbors(maze, current):
tentative_g_score = g_score[current] + 1
if tentative_g_score < g_score.get(neighbor, float('inf')):
came_from[neighbor] = current
g_score[neighbor] = tentative_g_score
f_score[neighbor] = g_score[neighbor] + manhattan_distance(neighbor, end)
heapq.heappush(heap, (f_score[neighbor], neighbor))
return None # No path found
# get_neighbors function remains the same as before
6. Optimization Techniques
As mazes and grids grow larger, optimization becomes crucial. Here are some techniques to improve the efficiency of your algorithms:
6.1 Memoization
Memoization involves caching the results of expensive function calls and returning the cached result when the same inputs occur again. This can significantly speed up recursive algorithms.
def memoize(func):
cache = {}
def memoized_func(*args):
if args in cache:
return cache[args]
result = func(*args)
cache[args] = result
return result
return memoized_func
@memoize
def recursive_path_finder(x, y, end_x, end_y):
# Implementation here
pass
6.2 Bidirectional Search
Bidirectional search runs two simultaneous searches: one forward from the start node and one backward from the goal node, stopping when the two meet in the middle. This can be faster than a single search from start to goal.
def bidirectional_search(maze, start, end):
forward_queue = deque([start])
backward_queue = deque([end])
forward_visited = {start: None}
backward_visited = {end: None}
while forward_queue and backward_queue:
# Expand forward
current = forward_queue.popleft()
for neighbor in get_neighbors(maze, current):
if neighbor not in forward_visited:
forward_visited[neighbor] = current
forward_queue.append(neighbor)
if neighbor in backward_visited:
return reconstruct_path(forward_visited, backward_visited, neighbor)
# Expand backward
current = backward_queue.popleft()
for neighbor in get_neighbors(maze, current):
if neighbor not in backward_visited:
backward_visited[neighbor] = current
backward_queue.append(neighbor)
if neighbor in forward_visited:
return reconstruct_path(forward_visited, backward_visited, neighbor)
return None # No path found
def reconstruct_path(forward_visited, backward_visited, meeting_point):
path = []
current = meeting_point
while current:
path.append(current)
current = forward_visited[current]
path = path[::-1]
current = backward_visited[meeting_point]
while current:
path.append(current)
current = backward_visited[current]
return path
6.3 Jump Point Search
Jump Point Search is an optimization of A* for uniform-cost grids. It can significantly reduce the number of nodes expanded during the search.
7. Advanced Concepts and Variations
As you become more proficient with basic maze and grid problems, you’ll encounter more complex variations. Here are some advanced concepts to explore:
7.1 Dynamic Programming in Grid-Based Problems
Dynamic programming can be particularly useful in grid-based problems where you need to find optimal solutions or count the number of possible paths. For example, consider the problem of finding the number of unique paths from the top-left to the bottom-right of a grid, moving only right and down.
def unique_paths(m, n):
dp = [[1] * n for _ in range(m)]
for i in range(1, m):
for j in range(1, n):
dp[i][j] = dp[i-1][j] + dp[i][j-1]
return dp[m-1][n-1]
7.2 Multi-Agent Pathfinding
In multi-agent pathfinding, you need to find paths for multiple agents simultaneously, often with the constraint that they don’t collide. This is significantly more complex than single-agent pathfinding and often requires specialized algorithms like Conflict-Based Search (CBS) or M*.
7.3 Continuous Space Navigation
While most maze and grid problems deal with discrete spaces, some applications require navigation in continuous space. Techniques like Rapidly-exploring Random Trees (RRT) or Probabilistic Roadmaps (PRM) are used in these scenarios.
7.4 Maze Generation Algorithms
Creating mazes can be as interesting as solving them. Common maze generation algorithms include:
- Recursive Backtracking
- Kruskal’s Algorithm
- Prim’s Algorithm
- Eller’s Algorithm
Here’s a simple implementation of the Recursive Backtracking algorithm for maze generation:
import random
def generate_maze(width, height):
# Initialize the maze with walls
maze = [[1 for _ in range(width)] for _ in range(height)]
def carve_passages(x, y):
directions = [(0, 1), (1, 0), (0, -1), (-1, 0)]
random.shuffle(directions)
for dx, dy in directions:
nx, ny = x + dx*2, y + dy*2
if 0 <= nx < width and 0 <= ny < height and maze[ny][nx] == 1:
maze[y + dy][x + dx] = 0
maze[ny][nx] = 0
carve_passages(nx, ny)
# Start from the top-left corner
maze[1][1] = 0
carve_passages(1, 1)
return maze
# Usage
maze = generate_maze(21, 21)
for row in maze:
print(''.join('#' if cell else ' ' for cell in row))
8. Real-World Applications
Maze and grid-based problems aren’t just academic exercises; they have numerous real-world applications:
8.1 Robotics and Autonomous Navigation
Pathfinding algorithms are crucial in robotics for navigation in both known and unknown environments. Whether it’s a warehouse robot, a self-driving car, or a Mars rover, these algorithms help in planning efficient and safe routes.
8.2 Video Game Development
Many video games, especially strategy and role-playing games, use pathfinding algorithms for character movement. These algorithms ensure that game characters can navigate complex game worlds efficiently and realistically.
8.3 Network Routing
The internet itself can be thought of as a complex maze. Routing algorithms, which determine the path data packets take through the network, are essentially solving a maze problem on a massive scale.
8.4 GPS and Navigation Systems
When your GPS calculates the fastest route to your destination, it’s solving a variation of the shortest path problem, taking into account factors like traffic, road types, and user preferences.
8.5 Circuit Board Design
Routing traces on a printed circuit board (PCB) is another application of maze-solving algorithms. The goal is to connect all components while avoiding intersections and minimizing trace length.
9. Practice Problems and Solutions
To reinforce your understanding, here are some practice problems along with their solutions:
9.1 Flood Fill
Problem: Given a 2D screen, location of a pixel in the screen and a color, replace color of the given pixel and all adjacent same-colored pixels with the given color.
def flood_fill(image, sr, sc, new_color):
rows, cols = len(image), len(image[0])
color = image[sr][sc]
if color == new_color:
return image
def dfs(r, c):
if image[r][c] == color:
image[r][c] = new_color
if r >= 1: dfs(r-1, c)
if r+1 < rows: dfs(r+1, c)
if c >= 1: dfs(r, c-1)
if c+1 < cols: dfs(r, c+1)
dfs(sr, sc)
return image
# Example usage
image = [
[1,1,1],
[1,1,0],
[1,0,1]
]
print(flood_fill(image, 1, 1, 2))
9.2 Number of Islands
Problem: Given a 2D grid map of ‘1’s (land) and ‘0’s (water), count the number of islands. An island is surrounded by water and is formed by connecting adjacent lands horizontally or vertically.
def num_islands(grid):
if not grid:
return 0
count = 0
for i in range(len(grid)):
for j in range(len(grid[0])):
if grid[i][j] == '1':
dfs(grid, i, j)
count += 1
return count
def dfs(grid, i, j):
if i<0 or j<0 or i>=len(grid) or j>=len(grid[0]) or grid[i][j] != '1':
return
grid[i][j] = '#' # Mark as visited
dfs(grid, i+1, j)
dfs(grid, i-1, j)
dfs(grid, i, j+1)
dfs(grid, i, j-1)
# Example usage
grid = [
['1','1','0','0','0'],
['1','1','0','0','0'],
['0','0','1','0','0'],
['0','0','0','1','1']
]
print(num_islands(grid)) # Output: 3
9.3 Word Search
Problem: Given a 2D board and a word, find if the word exists in the grid. The word can be constructed from letters of sequentially adjacent cells, where adjacent cells are horizontally or vertically neighboring. The same letter cell may not be used more than once.
def exist(board, word):
def dfs(i, j, k):
if k == len(word):
return True
if (i < 0 or i >= len(board) or
j < 0 or j >= len(board[0]) or
word[k] != board[i][j]):
return False
tmp = board[i][j]
board[i][j] = '#' # mark as visited
res = (dfs(i+1, j, k+1) or
dfs(i-1, j, k+1) or
dfs(i, j+1, k+1) or
dfs(i, j-1, k+1))
board[i][j] = tmp
return res
for i in range(len(board)):
for j in range(len(board[0])):
if dfs(i, j, 0):
return True
return False
# Example usage
board = [
['A','B','C','E'],
['S','F','C','S'],
['A','D','E','E']
]
print(exist(board, "ABCCED")) # Output: True
print(exist(board, "SEE")) # Output: True
print(exist(board, "ABCB")) # Output: False
10. Conclusion
Maze and grid-based coding problems are a fundamental part of computer science and have wide-ranging applications in the real world. By mastering these problems, you’re not only preparing for technical interviews but also developing skills that are directly applicable to many areas of software development.
Remember, the key to excelling at these problems is practice. Start with simple maze traversals, then work your way up to more complex pathfinding algorithms. Don’t be afraid to experiment with different approaches and optimizations. As you gain experience, you’ll develop an intuition for which techniques to apply in different scenarios.
Keep in mind that while efficiency is important, clarity and correctness should always be your first priority. A working solution that’s easy to understand and maintain is often more valuable than a highly optimized but complex one.
Finally, always consider the constraints and requirements of the problem at hand. The best algorithm for a given situation depends on factors like the size of the grid, the density of obstacles, whether the environment is static or dynamic, and what exactly you’re optimizing for (speed, memory usage, optimality of solution, etc.).
With dedication and practice, you’ll find that maze and grid-based problems become not just manageable, but enjoyable challenges that showcase your problem-solving skills and algorithmic thinking. Happy coding!