Navigating Graph Algorithms in Coding Interviews
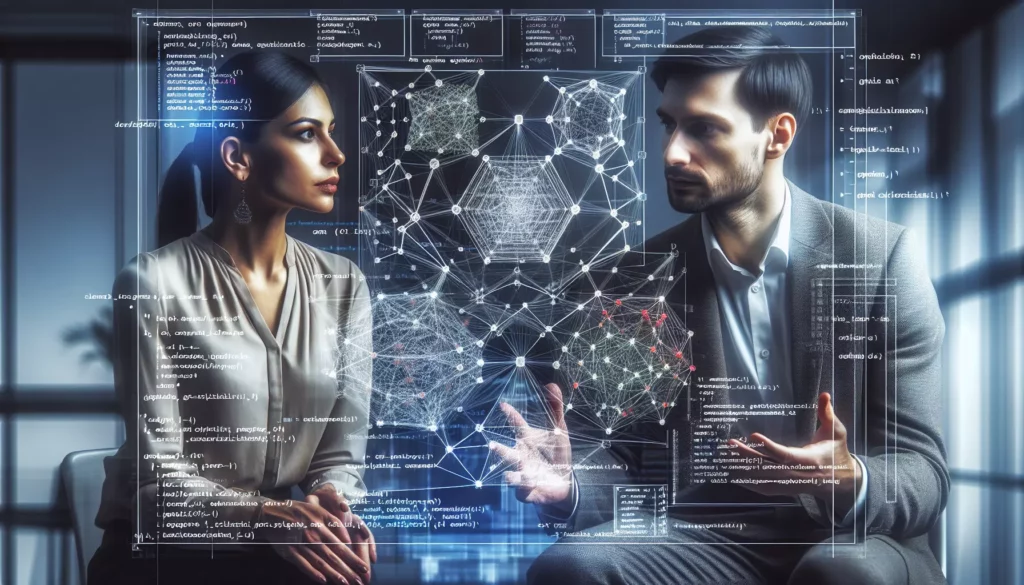
Graph algorithms are a crucial component of computer science and play a significant role in coding interviews, especially for positions at major tech companies. Understanding and implementing these algorithms effectively can be the key to success in technical interviews and real-world programming challenges. In this comprehensive guide, we’ll explore various graph algorithms, their applications, and strategies to tackle graph-related problems in coding interviews.
1. Introduction to Graphs
Before diving into specific algorithms, it’s essential to understand what graphs are and why they’re important in computer science.
1.1 What is a Graph?
A graph is a data structure consisting of a finite set of vertices (also called nodes) and a set of edges that connect these vertices. Graphs are used to represent relationships between different entities and are incredibly versatile in modeling various real-world scenarios.
1.2 Types of Graphs
- Directed Graphs (Digraphs): Edges have a direction, indicating a one-way relationship between vertices.
- Undirected Graphs: Edges have no direction, representing a two-way relationship between vertices.
- Weighted Graphs: Edges have associated weights or costs.
- Cyclic Graphs: Contain at least one cycle (a path that starts and ends at the same vertex).
- Acyclic Graphs: Contain no cycles.
1.3 Graph Representations
Graphs can be represented in various ways in code. The two most common representations are:
- Adjacency Matrix: A 2D array where matrix[i][j] represents the edge between vertices i and j.
- Adjacency List: An array of lists, where each list contains the neighbors of a vertex.
Here’s an example of how to represent a graph using an adjacency list in Python:
class Graph:
def __init__(self, vertices):
self.V = vertices
self.graph = [[] for _ in range(vertices)]
def add_edge(self, u, v):
self.graph[u].append(v)
self.graph[v].append(u) # For undirected graph
2. Depth-First Search (DFS)
Depth-First Search is a fundamental graph traversal algorithm that explores as far as possible along each branch before backtracking.
2.1 How DFS Works
- Start at a chosen vertex.
- Mark the current vertex as visited.
- Recursively visit all adjacent unvisited vertices.
- Backtrack when all adjacent vertices have been visited.
2.2 DFS Implementation
Here’s a Python implementation of DFS:
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start, end=' ')
for neighbor in graph[start]:
if neighbor not in visited:
dfs(graph, neighbor, visited)
2.3 Applications of DFS
- Detecting cycles in a graph
- Topological sorting
- Solving maze problems
- Finding connected components
3. Breadth-First Search (BFS)
Breadth-First Search is another crucial graph traversal algorithm that explores all vertices at the present depth before moving to vertices at the next depth level.
3.1 How BFS Works
- Start at a chosen vertex and enqueue it.
- Dequeue a vertex and mark it as visited.
- Enqueue all adjacent unvisited vertices.
- Repeat steps 2-3 until the queue is empty.
3.2 BFS Implementation
Here’s a Python implementation of BFS:
from collections import deque
def bfs(graph, start):
visited = set()
queue = deque([start])
visited.add(start)
while queue:
vertex = queue.popleft()
print(vertex, end=' ')
for neighbor in graph[vertex]:
if neighbor not in visited:
visited.add(neighbor)
queue.append(neighbor)
3.3 Applications of BFS
- Finding the shortest path in an unweighted graph
- Web crawling
- Social network analysis
- GPS navigation systems
4. Dijkstra’s Algorithm
Dijkstra’s algorithm is used to find the shortest path between nodes in a graph, which may represent, for example, road networks.
4.1 How Dijkstra’s Algorithm Works
- Initialize distances to all vertices as infinite and distance to the source as 0.
- Create a set of unvisited vertices.
- For the current vertex, consider all its unvisited neighbors and calculate their tentative distances.
- When we’re done considering all neighbors of the current vertex, mark it as visited and remove it from the unvisited set.
- If the destination vertex has been marked visited, we’re done.
- Otherwise, select the unvisited vertex with the smallest tentative distance, and repeat from step 3.
4.2 Dijkstra’s Algorithm Implementation
Here’s a Python implementation of Dijkstra’s algorithm:
import heapq
def dijkstra(graph, start):
distances = {vertex: float('infinity') for vertex in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_vertex = heapq.heappop(pq)
if current_distance > distances[current_vertex]:
continue
for neighbor, weight in graph[current_vertex].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return distances
4.3 Applications of Dijkstra’s Algorithm
- GPS and mapping services
- Network routing protocols
- Finding the shortest path in social networks
- Robotics and pathfinding in games
5. Bellman-Ford Algorithm
The Bellman-Ford algorithm is used to find the shortest paths from a single source vertex to all other vertices in a weighted graph. Unlike Dijkstra’s algorithm, it can handle graphs with negative edge weights.
5.1 How Bellman-Ford Algorithm Works
- Initialize distances from the source to all vertices as infinite and distance to the source itself as 0.
- Relax all edges |V| – 1 times, where |V| is the number of vertices in the graph.
- Check for negative-weight cycles. If we can still relax an edge, then there is a negative-weight cycle.
5.2 Bellman-Ford Algorithm Implementation
Here’s a Python implementation of the Bellman-Ford algorithm:
def bellman_ford(graph, source):
distance = {vertex: float('infinity') for vertex in graph}
distance[source] = 0
for _ in range(len(graph) - 1):
for vertex in graph:
for neighbor, weight in graph[vertex].items():
if distance[vertex] + weight < distance[neighbor]:
distance[neighbor] = distance[vertex] + weight
# Check for negative-weight cycles
for vertex in graph:
for neighbor, weight in graph[vertex].items():
if distance[vertex] + weight < distance[neighbor]:
print("Graph contains a negative-weight cycle")
return None
return distance
5.3 Applications of Bellman-Ford Algorithm
- Detecting negative cycles in graphs
- Routing in networking protocols (like RIP)
- Arbitrage detection in currency exchange
6. Minimum Spanning Tree Algorithms
A Minimum Spanning Tree (MST) is a subset of the edges of a connected, edge-weighted graph that connects all the vertices together, without any cycles and with the minimum possible total edge weight.
6.1 Kruskal’s Algorithm
Kruskal’s algorithm builds the MST by adding edges one by one into a growing spanning tree.
6.1.1 How Kruskal’s Algorithm Works
- Sort all edges in non-decreasing order of their weight.
- Pick the smallest edge. Check if it forms a cycle with the spanning tree formed so far. If not, include this edge. Else, discard it.
- Repeat step 2 until there are (V-1) edges in the spanning tree.
6.1.2 Kruskal’s Algorithm Implementation
Here’s a Python implementation of Kruskal’s algorithm:
class DisjointSet:
def __init__(self, vertices):
self.parent = {v: v for v in vertices}
self.rank = {v: 0 for v in vertices}
def find(self, item):
if self.parent[item] != item:
self.parent[item] = self.find(self.parent[item])
return self.parent[item]
def union(self, x, y):
xroot = self.find(x)
yroot = self.find(y)
if self.rank[xroot] < self.rank[yroot]:
self.parent[xroot] = yroot
elif self.rank[xroot] > self.rank[yroot]:
self.parent[yroot] = xroot
else:
self.parent[yroot] = xroot
self.rank[xroot] += 1
def kruskal_mst(graph):
edges = [(w, u, v) for u in graph for v, w in graph[u].items()]
edges.sort()
vertices = list(graph.keys())
ds = DisjointSet(vertices)
mst = []
for w, u, v in edges:
if ds.find(u) != ds.find(v):
ds.union(u, v)
mst.append((u, v, w))
return mst
6.2 Prim’s Algorithm
Prim’s algorithm builds the MST by adding vertices one by one to the growing spanning tree.
6.2.1 How Prim’s Algorithm Works
- Initialize a tree with a single vertex, chosen arbitrarily from the graph.
- Grow the tree by one edge: of the edges that connect the tree to vertices not yet in the tree, find the minimum-weight edge, and transfer it to the tree.
- Repeat step 2 until all vertices are in the tree.
6.2.2 Prim’s Algorithm Implementation
Here’s a Python implementation of Prim’s algorithm:
import heapq
def prim_mst(graph):
start_vertex = next(iter(graph))
mst = []
visited = set([start_vertex])
edges = [(w, start_vertex, v) for v, w in graph[start_vertex].items()]
heapq.heapify(edges)
while edges:
w, u, v = heapq.heappop(edges)
if v not in visited:
visited.add(v)
mst.append((u, v, w))
for next_v, next_w in graph[v].items():
if next_v not in visited:
heapq.heappush(edges, (next_w, v, next_v))
return mst
6.3 Applications of MST Algorithms
- Network design (e.g., laying cable for computer networks)
- Approximation algorithms for NP-hard problems
- Cluster analysis in data mining
- Image segmentation in computer vision
7. Topological Sorting
Topological sorting is used for ordering tasks with dependencies. It’s applicable only to Directed Acyclic Graphs (DAGs).
7.1 How Topological Sorting Works
- Choose a vertex with no incoming edges.
- Add it to the result list and remove it from the graph.
- Repeat steps 1-2 until all vertices are processed.
7.2 Topological Sorting Implementation
Here’s a Python implementation of topological sorting using DFS:
def topological_sort(graph):
visited = set()
stack = []
def dfs(v):
visited.add(v)
for neighbor in graph[v]:
if neighbor not in visited:
dfs(neighbor)
stack.append(v)
for vertex in graph:
if vertex not in visited:
dfs(vertex)
return stack[::-1]
7.3 Applications of Topological Sorting
- Scheduling jobs or tasks
- Determining the order of compilation tasks
- Resolving symbol dependencies in linkers
8. Strongly Connected Components
A strongly connected component (SCC) of a directed graph is a maximal set of vertices such that for every pair of vertices u and v, there is a path from u to v and a path from v to u.
8.1 Kosaraju’s Algorithm
Kosaraju’s algorithm is used to find strongly connected components in a graph.
8.1.1 How Kosaraju’s Algorithm Works
- Perform a DFS on the original graph, keeping track of the finish times of vertices.
- Create the transpose of the graph (reverse all edge directions).
- Perform a DFS on the transposed graph, in the order of decreasing finish times from step 1.
8.1.2 Kosaraju’s Algorithm Implementation
Here’s a Python implementation of Kosaraju’s algorithm:
def kosaraju(graph):
def dfs_first(v):
visited.add(v)
for neighbor in graph[v]:
if neighbor not in visited:
dfs_first(neighbor)
finish_time.append(v)
def dfs_second(v):
visited.add(v)
component.append(v)
for neighbor in transpose[v]:
if neighbor not in visited:
dfs_second(neighbor)
visited = set()
finish_time = []
for vertex in graph:
if vertex not in visited:
dfs_first(vertex)
transpose = {v: [] for v in graph}
for v in graph:
for neighbor in graph[v]:
transpose[neighbor].append(v)
visited.clear()
sccs = []
for vertex in reversed(finish_time):
if vertex not in visited:
component = []
dfs_second(vertex)
sccs.append(component)
return sccs
8.2 Applications of Strongly Connected Components
- Social network analysis
- Bioinformatics (analyzing metabolic networks)
- Solving 2-satisfiability problems
9. Graph Coloring
Graph coloring is the assignment of labels (colors) to elements of a graph subject to certain constraints. The most common type is vertex coloring, where adjacent vertices must have different colors.
9.1 Greedy Coloring Algorithm
While not always optimal, the greedy approach is simple and often effective for graph coloring.
9.1.1 How Greedy Coloring Works
- Order the vertices in some way.
- Assign the first color to the first vertex.
- For the remaining vertices, assign the lowest numbered color that hasn’t been used on any adjacent vertices.
9.1.2 Greedy Coloring Implementation
Here’s a Python implementation of the greedy coloring algorithm:
def greedy_coloring(graph):
colors = {}
for vertex in graph:
used_colors = set(colors.get(neighbor) for neighbor in graph[vertex] if neighbor in colors)
colors[vertex] = next(color for color in range(len(graph)) if color not in used_colors)
return colors
9.2 Applications of Graph Coloring
- Scheduling problems
- Register allocation in compilers
- Map coloring
- Solving Sudoku puzzles
10. Tips for Tackling Graph Problems in Interviews
- Understand the problem: Clearly identify if the problem is related to graphs and what type of graph you’re dealing with (directed, undirected, weighted, etc.).
- Choose the right representation: Decide whether an adjacency matrix or adjacency list is more suitable for the problem at hand.
- Identify the appropriate algorithm: Based on the problem requirements, choose the most suitable algorithm (DFS, BFS, Dijkstra’s, etc.).
- Consider edge cases: Think about scenarios like disconnected graphs, self-loops, or negative edge weights.
- Optimize for space and time: Be prepared to discuss the time and space complexity of your solution.
- Practice implementation: Familiarize yourself with implementing these algorithms efficiently in your preferred programming language.
- Explain your approach: Clearly communicate your thought process and the rationale behind your chosen algorithm.
Conclusion
Graph algorithms are a fundamental part of computer science and play a crucial role in many real-world applications. By mastering these algorithms and understanding their applications, you’ll be well-prepared to tackle a wide range of problems in coding interviews and beyond. Remember, the key to success lies not just in memorizing these algorithms, but in understanding the underlying principles and being able to apply them to solve novel problems.
As you continue your journey in coding education and programming skills development, platforms like AlgoCademy can provide valuable resources and interactive coding tutorials to help you practice and refine your graph algorithm skills. With consistent practice and a solid understanding of these concepts, you’ll be well-equipped to navigate the challenges of technical interviews and excel in your programming career.