Navigating Follow-Up Questions: How to Handle Variations and Extensions to Your Solution
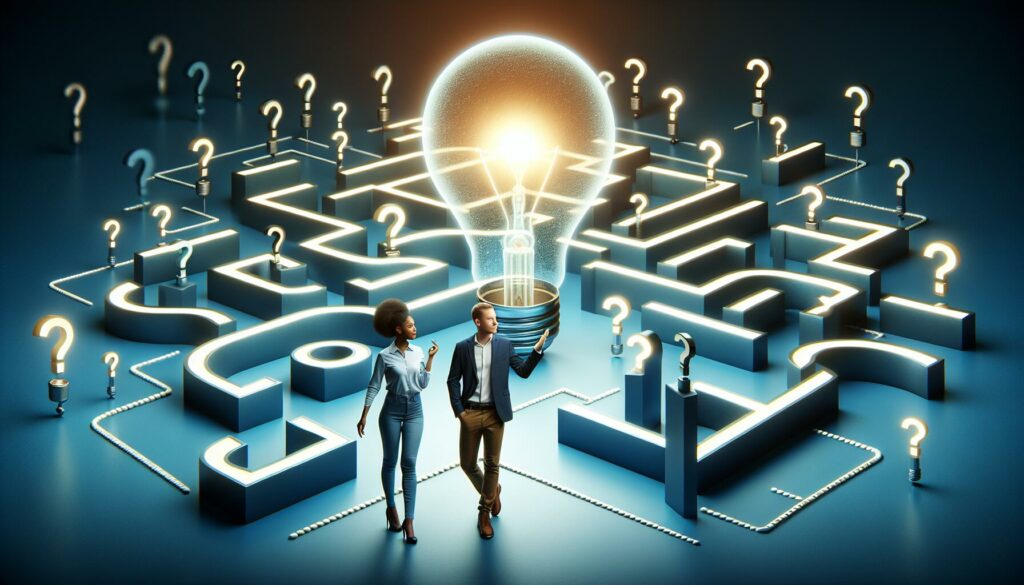
In the world of coding interviews and technical assessments, successfully solving the initial problem is only half the battle. What often separates exceptional candidates from the rest is their ability to handle follow-up questions, variations, and extensions to their original solution. This skill demonstrates not only your technical prowess but also your adaptability, critical thinking, and deep understanding of the problem at hand. In this comprehensive guide, we’ll explore strategies for navigating these follow-up questions effectively, helping you showcase your problem-solving skills and stand out in technical interviews.
Understanding the Nature of Follow-Up Questions
Before diving into strategies, it’s crucial to understand why interviewers ask follow-up questions and what they’re typically looking for. Follow-up questions serve several purposes:
- To test the depth of your understanding
- To assess your ability to think on your feet
- To evaluate how you handle more complex scenarios
- To gauge your problem-solving approach when faced with new constraints
- To see how you optimize solutions for better performance
These questions often fall into a few common categories:
- Variations on input or constraints
- Performance optimizations
- Scaling the solution
- Handling edge cases
- Applying the solution to a related problem
Strategies for Handling Follow-Up Questions
1. Stay Calm and Collected
The first and most crucial step is to remain calm. Follow-up questions are not meant to trick you or catch you off guard; they’re an opportunity to showcase your adaptability and deeper understanding. Take a deep breath and approach the new challenge with confidence.
2. Clarify the New Requirements
Before jumping into modifications or new solutions, make sure you fully understand the new requirements or constraints. Don’t hesitate to ask clarifying questions such as:
- “Can you provide an example of the new input format?”
- “Are there any additional constraints I should be aware of?”
- “What’s the expected outcome with these new conditions?”
This step ensures you’re solving the right problem and demonstrates your attention to detail.
3. Think Aloud
As you process the new information and consider your approach, think aloud. This gives the interviewer insight into your problem-solving process and allows them to provide hints if you’re heading in the wrong direction. You might say something like:
“Given this new constraint, I’m thinking we might need to modify our data structure to accommodate faster lookups. Let me think through a few options…”
4. Start with Your Existing Solution
Often, the best approach is to start with your existing solution and consider how it can be adapted or extended. This shows that you can build upon your work and demonstrates efficiency in problem-solving. Consider:
- Can the current algorithm be tweaked to handle the new requirements?
- Is there a way to preprocess the data to fit your existing solution?
- Can you add a step before or after your current solution to address the new constraints?
5. Consider Time and Space Complexity
When asked about optimizations or scaling, always consider both time and space complexity. Be prepared to discuss trade-offs between the two. For example:
“We could improve the time complexity from O(n^2) to O(n log n) by using a heap, but this would increase our space complexity from O(1) to O(n). The trade-off might be worth it for larger datasets.”
6. Draw from Your Knowledge Base
Don’t be afraid to apply concepts or techniques from other problems you’ve encountered. This shows breadth of knowledge and ability to make connections. You might say:
“This new requirement reminds me of the sliding window technique we could apply here to optimize our solution.”
7. Break Down Complex Extensions
If the follow-up significantly increases the complexity, break it down into smaller, manageable parts. This demonstrates your ability to tackle complex problems methodically. For instance:
“To handle this multi-dimensional aspect, let’s first consider how we can adapt our solution for 2D, then we’ll think about extending it to 3D or n-dimensions.”
8. Be Honest About Limitations
If you’re unsure about a particular optimization or extension, it’s okay to admit it. Honesty coupled with a willingness to learn is often appreciated. You could say:
“I’m not immediately sure how to optimize this for distributed systems, but I’d approach it by researching distributed algorithms and possibly consulting with colleagues who have experience in this area.”
9. Propose Multiple Approaches
When faced with a new constraint or optimization request, try to come up with multiple approaches if possible. This showcases your ability to see problems from different angles. You might say:
“We could approach this optimization in two ways: either by using a more efficient data structure like a balanced BST, or by preprocessing the data to allow for faster queries. Let’s explore the trade-offs of each.”
10. Use Analogies and Visual Aids
Sometimes, explaining your thought process for complex extensions can be challenging. Use analogies or draw diagrams to illustrate your ideas. This demonstrates communication skills and can help clarify your thoughts. For example:
“Think of this new requirement like a multi-level cache system. We’ll handle frequently accessed items in our fastest cache, while less common items can be stored in slower, larger caches.”
Common Types of Follow-Up Questions and How to Handle Them
1. Scaling the Solution
Often, interviewers will ask how your solution would scale with larger inputs or in distributed systems. To handle this:
- Consider parallel processing possibilities
- Think about data partitioning strategies
- Discuss potential bottlenecks and how to mitigate them
- Consider caching strategies for frequently accessed data
Example response:
“To scale this solution for much larger datasets, we could implement a MapReduce approach. We’d partition the data across multiple machines, apply our algorithm to each partition in parallel, then combine the results. We’d need to consider load balancing and fault tolerance in this distributed setup.”
2. Optimizing for Time or Space Complexity
Improving the efficiency of your solution is a common follow-up. Approach this by:
- Analyzing the current bottlenecks in your solution
- Considering more efficient data structures
- Looking for redundant operations that can be eliminated
- Thinking about precomputation or caching strategies
Example response:
“To optimize our current O(n^2) solution, we could use a hash map to store previously computed values, reducing repeated calculations. This would bring our time complexity down to O(n) at the cost of O(n) space complexity.”
3. Handling Edge Cases
Interviewers often ask about how your solution handles edge cases. To address this:
- Systematically consider extreme inputs (empty, very large, negative numbers, etc.)
- Think about invalid inputs and how to handle them gracefully
- Consider boundary conditions in your algorithm
Example response:
“Let’s consider some edge cases. For an empty input, we should return []. For inputs with all identical elements, our current solution works correctly. If we receive negative numbers, we might need to modify our comparison logic to handle them properly.”
4. Applying the Solution to a Related Problem
Sometimes, you’ll be asked to adapt your solution to a slightly different problem. Here’s how to approach this:
- Identify the similarities and differences between the problems
- Consider which parts of your current solution can be reused
- Think about what new components or modifications are needed
Example response:
“To adapt our solution from finding the maximum subarray to finding the maximum submatrix in a 2D array, we could apply our 1D solution to each row, then use a similar approach to combine the results vertically. This would increase our time complexity from O(n) to O(n^3), but we could optimize further using techniques like Kadane’s algorithm in both dimensions.”
5. Handling Constraints on Input or Output
New constraints might be introduced to challenge your solution. Address these by:
- Carefully considering how the new constraints affect each step of your algorithm
- Thinking about preprocessing steps to handle the constraints
- Considering if a completely different approach might be more suitable
Example response:
“If we’re now constrained to O(1) space complexity, we can’t use our hash map approach. Instead, we could implement Floyd’s cycle-finding algorithm, also known as the “tortoise and hare” algorithm, which allows us to detect cycles using only two pointers, thus satisfying the O(1) space requirement.”
Demonstrating Adaptability in Your Responses
Adaptability is key when handling follow-up questions. Here are some ways to showcase this skill:
1. Quickly Acknowledge the New Challenge
Show that you’re engaged and ready to tackle the new aspect of the problem. You might say:
“That’s an interesting twist on the problem. Let me think about how we can adapt our current approach to handle this new requirement.”
2. Draw Parallels to Known Problems
Demonstrate your ability to connect different concepts by relating the new challenge to problems you’re familiar with. For example:
“This new constraint reminds me of the producer-consumer problem. We might be able to adapt some of those synchronization techniques to our current solution.”
3. Propose Incremental Changes
Instead of completely scrapping your original solution, try to build upon it incrementally. This shows that you can evolve your thinking. You could say:
“Let’s start by modifying our input processing step to handle the new data format, then we’ll see if we need to adjust our core algorithm.”
4. Consider Multiple Perspectives
Show that you can approach the problem from different angles. For instance:
“We could tackle this either from a top-down perspective using recursion with memoization, or from a bottom-up approach using dynamic programming. Let’s explore the trade-offs of each.”
5. Discuss Trade-offs Openly
Demonstrate your understanding of the implications of different approaches. You might say:
“While this modification would improve our time complexity, it would increase our space usage. In a real-world scenario, we’d need to consider factors like available memory and the size of our dataset to decide if this trade-off is worthwhile.”
Practical Examples of Handling Follow-Up Questions
Let’s walk through a couple of examples to illustrate how these strategies can be applied in practice.
Example 1: Two Sum Problem
Initial Problem: Given an array of integers and a target sum, return indices of two numbers such that they add up to the target.
Initial Solution:
def two_sum(nums, target):
seen = {}
for i, num in enumerate(nums):
complement = target - num
if complement in seen:
return [seen[complement], i]
seen[num] = i
return []
Follow-up Question: “How would you modify your solution if the array was sorted?”
Approach to Answer:
- Acknowledge the new information: “Interesting, the fact that the array is sorted gives us new possibilities to optimize our solution.”
- Consider how it changes the problem: “With a sorted array, we can use a two-pointer approach, which could potentially reduce our space complexity.”
- Propose a new solution: “Let’s use two pointers, one at the start and one at the end of the array, and move them based on the sum we calculate.”
- Implement the new solution:
def two_sum_sorted(nums, target):
left, right = 0, len(nums) - 1
while left < right:
current_sum = nums[left] + nums[right]
if current_sum == target:
return [left, right]
elif current_sum < target:
left += 1
else:
right -= 1
return []
Explain the benefits: “This new solution has a time complexity of O(n) and a space complexity of O(1), which is an improvement over our original O(n) space complexity. It takes advantage of the sorted nature of the array to efficiently narrow down the search space.”
Example 2: Fibonacci Sequence
Initial Problem: Write a function to generate the nth Fibonacci number.
Initial Solution (Recursive):
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n-1) + fibonacci(n-2)
Follow-up Question: “This solution is not very efficient for large n. Can you optimize it?”
Approach to Answer:
- Acknowledge the issue: “You’re right, this recursive solution has exponential time complexity, which is inefficient for large n.”
- Propose optimization techniques: “We can significantly improve this using dynamic programming or even achieve O(1) space complexity with iterative calculation.”
- Implement an optimized solution:
def fibonacci_optimized(n):
if n <= 1:
return n
a, b = 0, 1
for _ in range(2, n + 1):
a, b = b, a + b
return b
Explain the optimization: “This solution uses constant space and has a linear time complexity of O(n). It iteratively calculates Fibonacci numbers up to n, storing only the two most recent numbers at any time.”
Further extension: “If we needed even faster computation for very large n, we could discuss matrix exponentiation methods or closed-form solutions like Binet’s formula, though we’d need to consider issues of numerical precision with the latter.”
Conclusion
Mastering the art of handling follow-up questions is crucial for success in coding interviews and demonstrates your ability to think critically and adapt to new challenges. By staying calm, clarifying requirements, thinking aloud, and systematically approaching variations and optimizations, you can showcase not just your coding skills, but your problem-solving abilities and technical depth.
Remember, the goal of these follow-up questions is not to trip you up, but to explore the depths of your understanding and your ability to apply your knowledge in different contexts. Embrace these questions as opportunities to demonstrate your skills, and you’ll be well on your way to impressing interviewers and advancing your coding career.
Practice regularly with varied problems, anticipate potential follow-ups, and always be ready to explain your thought process. With time and experience, you’ll develop the confidence and skills to navigate even the most challenging interview scenarios.