Navigating Ambiguity: How to Handle Open-Ended Problems in a Coding Interview
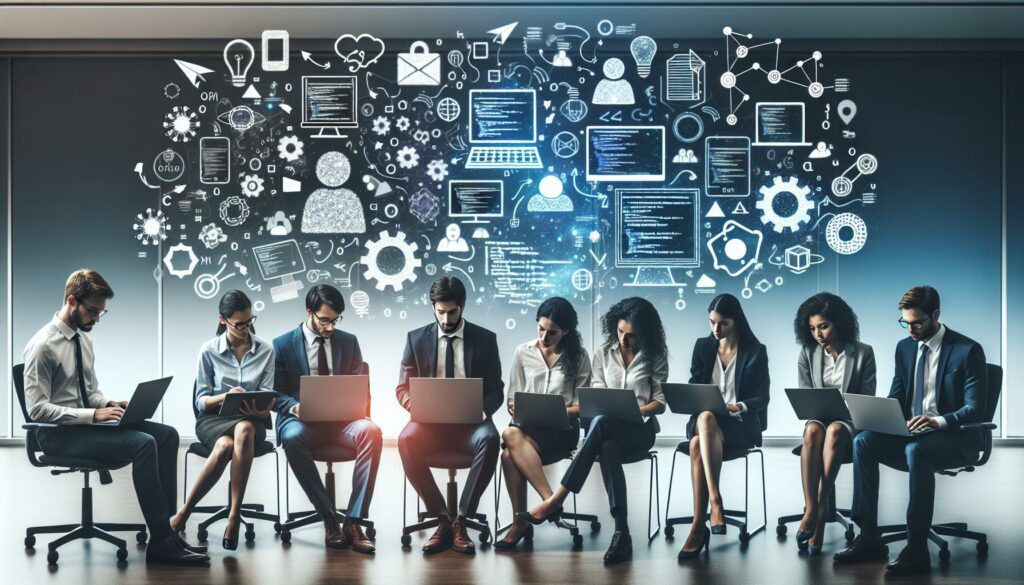
In the world of software engineering, problem-solving skills are paramount. This is especially true during coding interviews, where candidates are often presented with complex, open-ended problems that don’t have clear-cut solutions. These ambiguous questions are designed to test not just your coding abilities, but also your approach to problem-solving, your communication skills, and your ability to think on your feet.
In this comprehensive guide, we’ll explore strategies for tackling ambiguous or open-ended interview questions, providing you with the tools you need to navigate these challenging scenarios with confidence. We’ll discuss how to frame the problem, make reasonable assumptions, and validate those assumptions with your interviewer, all while showcasing your problem-solving prowess.
Understanding the Purpose of Open-Ended Interview Questions
Before we dive into strategies, it’s important to understand why interviewers ask open-ended questions in the first place. These types of questions serve several purposes:
- To assess your problem-solving approach
- To evaluate your communication skills
- To gauge your ability to handle ambiguity
- To see how you think through complex issues
- To observe how you collaborate and respond to feedback
By understanding these objectives, you can tailor your approach to not only solve the problem but also demonstrate the skills and qualities that interviewers are looking for.
Strategies for Approaching Open-Ended Problems
1. Take a Moment to Gather Your Thoughts
When presented with an ambiguous problem, it’s perfectly acceptable—and often advisable—to take a moment to collect your thoughts. Don’t feel pressured to start coding or even talking immediately. Instead, consider saying something like:
“That’s an interesting problem. Would you mind if I take a minute to think about it before we discuss further?”
This shows the interviewer that you’re thoughtful and deliberate in your approach, rather than rushing into a solution without proper consideration.
2. Ask Clarifying Questions
One of the most critical steps in handling open-ended problems is to ask clarifying questions. This serves multiple purposes:
- It helps you understand the problem better
- It demonstrates your analytical thinking
- It shows that you’re not afraid to seek clarification when needed
- It can reveal important details that weren’t initially provided
Some examples of clarifying questions might include:
- “What are the expected inputs and outputs for this problem?”
- “Are there any specific constraints or requirements we need to consider?”
- “Can you provide an example of how this might be used in a real-world scenario?”
- “Are there any performance considerations we should keep in mind?”
3. Frame the Problem
Once you’ve gathered more information through clarifying questions, try to frame the problem in your own words. This accomplishes several things:
- It ensures that you and the interviewer are on the same page
- It helps you organize your thoughts
- It allows the interviewer to correct any misunderstandings
You might say something like:
“Based on our discussion, it seems like we’re trying to design a system that [describe the main goal]. The key challenges appear to be [list main challenges]. Is that an accurate summary of the problem?”
4. Make Reasonable Assumptions
In many cases, you’ll need to make assumptions to move forward with the problem. The key is to make these assumptions explicit and reasonable. Here’s how:
- State your assumption clearly
- Explain why you’re making this assumption
- Ask if the assumption is valid
For example:
“For this problem, I’m assuming that the input data will always be in a valid format. This simplifies our error handling for now. Is it okay to make this assumption, or should we consider invalid input scenarios as well?”
5. Break Down the Problem
Large, open-ended problems can be overwhelming. A effective strategy is to break the problem down into smaller, more manageable components. This approach has several benefits:
- It makes the problem less daunting
- It allows you to tackle one piece at a time
- It helps identify potential sub-problems or edge cases
- It demonstrates your ability to handle complex systems
You might say something like:
“To approach this problem, I think we can break it down into the following components: [list components]. Let’s start by focusing on [specific component]. Does this breakdown make sense to you?”
6. Consider Multiple Approaches
Open-ended problems often have multiple valid solutions. Instead of immediately jumping to implement the first solution that comes to mind, consider and discuss multiple approaches. This shows:
- Your ability to think creatively
- Your knowledge of different algorithms and data structures
- Your understanding of trade-offs between different solutions
You might say:
“I can think of a few different ways we could approach this. We could use [approach 1], which has the advantage of [benefit] but might [drawback]. Alternatively, we could use [approach 2], which [describe]. Would you like me to elaborate on one of these approaches, or do you have a preference for which direction we should go?”
7. Start with a Simple Solution
When dealing with complex problems, it’s often beneficial to start with a simple solution and then iterate. This approach, sometimes called the “naive solution,” has several advantages:
- It gets you started quickly
- It provides a baseline for optimization
- It helps identify edge cases or potential issues
- It demonstrates your ability to write working code
You might say:
“Let’s start with a simple solution to get the basic functionality working. We can then identify areas for optimization. Here’s what I’m thinking…”
8. Think Aloud
As you work through the problem, make sure to vocalize your thought process. This is crucial in a coding interview because:
- It allows the interviewer to follow your reasoning
- It demonstrates your problem-solving approach
- It gives the interviewer opportunities to provide hints or guidance
- It shows your ability to communicate technical concepts
Don’t be afraid to think out loud, even if you’re not sure about something. You might say:
“I’m considering using a hash map here because it would give us O(1) lookup time, but I’m wondering if the space complexity might be an issue. What do you think?”
9. Be Open to Feedback and Hints
Remember, the interview is not just about getting to the right answer—it’s also about demonstrating your ability to collaborate and learn. Be open to feedback and hints from the interviewer. If they suggest a different approach or point out a potential issue, engage with their ideas constructively. This shows:
- Your ability to take feedback
- Your willingness to learn and adapt
- Your collaborative nature
You might respond to feedback like this:
“That’s a great point. I hadn’t considered that edge case. Let’s modify our approach to handle that scenario.”
Practical Example: Designing a URL Shortener
Let’s walk through a practical example of how to apply these strategies to an open-ended interview question. Suppose the interviewer asks:
“Design a URL shortening service like bit.ly.”
This is a classic system design question that’s intentionally vague. Here’s how you might approach it:
1. Take a Moment and Ask Clarifying Questions
After taking a brief moment to consider the question, you might ask:
- “What’s the expected scale of this service? How many URLs might we need to handle?”
- “Do we need to consider analytics, like tracking the number of clicks?”
- “Should the shortened URLs be randomly generated or can users customize them?”
- “Do we need to handle URL expiration?”
2. Frame the Problem
Based on the answers, you might frame the problem like this:
“It seems we’re designing a URL shortening service that can handle millions of URLs, with basic analytics for tracking clicks. We’ll generate random short URLs, and we don’t need to worry about expiration for now. Is that correct?”
3. Make Assumptions and Break Down the Problem
You might continue:
“I’m going to assume that we want our shortened URLs to be as short as possible while still being unique. Let’s break this down into a few key components:
1. A service to generate unique short codes
2. A database to store the mapping between short codes and original URLs
3. A web server to handle redirects and track clicks
Does this sound reasonable?”
4. Consider Multiple Approaches and Start Simple
For the URL generation, you might say:
“For generating unique codes, we could use a simple incremental ID and convert it to base62 to keep it short. Alternatively, we could use a hash function on the original URL. Let’s start with the incremental ID approach as it’s simpler and gives us more control over the length of the short URL.”
5. Think Aloud and Be Open to Feedback
As you start to design the system, continue to vocalize your thought process:
“For the database, I’m thinking we could use a NoSQL database like Cassandra for its scalability. We’d have two main operations: inserting new mappings and retrieving original URLs. The schema might look something like this:”
short_code (primary key) | original_url | creation_date | click_count
-------------------------|--------------|---------------|------------
abc123 | http://... | 2023-06-01 | 42
If the interviewer suggests considering SQL databases as well, you might respond:
“That’s a good point. A SQL database could work well too, especially if we need to do more complex queries on the data in the future. We could use MySQL or PostgreSQL, which would allow for easy joins if we decide to add user accounts or more detailed analytics later.”
Common Pitfalls to Avoid
When tackling open-ended problems in coding interviews, there are several common pitfalls that candidates often fall into. Being aware of these can help you navigate the interview more successfully:
1. Rushing to Code
One of the biggest mistakes is jumping straight into coding without fully understanding the problem or considering different approaches. Remember, the interviewer is often more interested in your problem-solving process than in seeing a complete implementation.
2. Failing to Communicate
Silence can be uncomfortable, but it’s worse to leave the interviewer in the dark about your thought process. Even if you’re stuck, verbalize what you’re thinking and what’s causing you difficulty.
3. Ignoring Constraints
Make sure you’ve understood and are addressing all the constraints of the problem. If you’re unsure about a constraint, ask for clarification rather than making assumptions.
4. Not Considering Edge Cases
Open-ended problems often have numerous edge cases. Take time to consider these and discuss how your solution would handle them.
5. Sticking to One Approach
If your initial approach isn’t working, be willing to take a step back and consider alternatives. Demonstrating flexibility and the ability to pivot is valuable.
6. Overlooking Scalability
Especially for system design questions, consider how your solution would scale. Discuss potential bottlenecks and how you might address them.
7. Neglecting to Test
Even if you don’t have time to write formal unit tests, talk through how you would test your solution. Consider various inputs, including edge cases.
Practicing Open-Ended Problem Solving
Improving your ability to handle open-ended problems takes practice. Here are some ways you can hone this skill:
1. Mock Interviews
Practice with a friend or use online platforms that offer mock interview services. This can help you get comfortable with the pressure of thinking on your feet.
2. System Design Questions
Study and practice system design questions, which are often open-ended. Resources like “System Design Interview” by Alex Xu can be helpful.
3. Solve Real-World Problems
Try to solve real-world problems you encounter in your daily life. How would you design a traffic light system? Or a restaurant reservation system? Approaching these problems systematically can improve your problem-solving skills.
4. Contribute to Open Source
Contributing to open-source projects can expose you to complex, real-world problems and collaborative problem-solving.
5. Read Code
Reading and understanding others’ code can help you recognize different approaches to solving problems.
6. Teach Others
Explaining concepts or walking others through problem-solving can deepen your own understanding and improve your communication skills.
Conclusion
Navigating ambiguity in coding interviews is a skill that can be developed with practice and the right approach. By following the strategies outlined in this guide—taking time to think, asking clarifying questions, framing the problem, making and validating assumptions, breaking down the problem, considering multiple approaches, starting simple, thinking aloud, and being open to feedback—you’ll be well-equipped to handle open-ended problems.
Remember, the goal isn’t just to solve the problem, but to demonstrate your problem-solving process, communication skills, and ability to handle complex, ambiguous situations. These are all valuable skills in the real world of software development, where problems are often not as well-defined as in textbooks or coding challenges.
As you prepare for your coding interviews, practice applying these strategies to a variety of problems. Over time, you’ll find that your ability to navigate ambiguity improves, making you not just a stronger interview candidate, but a more effective problem solver and software engineer overall.
Keep in mind that every interview and every problem is an opportunity to learn and grow. Even if you don’t arrive at the perfect solution, your approach to the problem and your ability to communicate your thoughts clearly can leave a strong positive impression on your interviewer.
Good luck with your coding interviews, and remember: embrace the ambiguity. It’s not just a challenge—it’s an opportunity to showcase your unique problem-solving abilities.