Navigating Algorithm Complexity Classes: A Comprehensive Guide
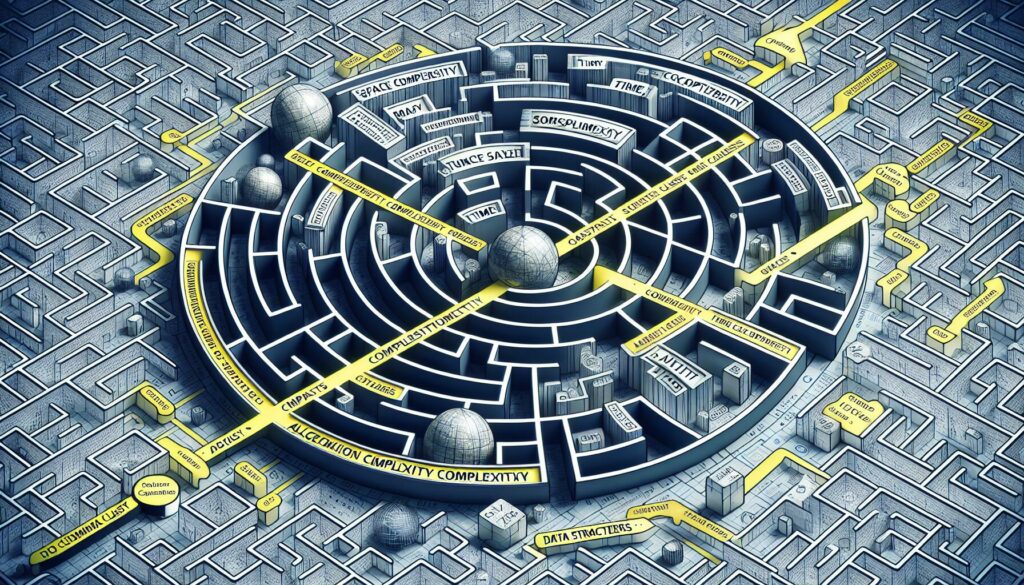
In the realm of computer science and programming, understanding algorithm complexity is crucial for developing efficient and scalable solutions. As you progress from basic coding to tackling complex problems, especially when preparing for technical interviews at major tech companies, grasping the concept of complexity classes becomes increasingly important. This comprehensive guide will walk you through the various algorithm complexity classes, their significance, and how to navigate them effectively in your coding journey.
1. Introduction to Algorithm Complexity
Before diving into specific complexity classes, it’s essential to understand what algorithm complexity means and why it matters.
1.1 What is Algorithm Complexity?
Algorithm complexity refers to the amount of resources (such as time and space) required by an algorithm to solve a problem as the input size grows. It helps us analyze and compare different algorithms’ efficiency, allowing us to choose the most suitable approach for a given problem.
1.2 Why is Algorithm Complexity Important?
Understanding algorithm complexity is crucial for several reasons:
- Performance optimization: It helps in writing more efficient code.
- Scalability: It allows you to predict how an algorithm will perform with larger inputs.
- Resource management: It aids in estimating the computational resources required.
- Interview preparation: Many technical interviews, especially at FAANG companies, focus heavily on algorithm efficiency.
2. Big O Notation
Big O notation is the most common way to express algorithm complexity. It describes the worst-case scenario or upper bound of an algorithm’s growth rate.
2.1 Understanding Big O Notation
Big O notation is expressed as O(f(n)), where f(n) represents the growth rate of the algorithm concerning the input size n. For example:
- O(1): Constant time complexity
- O(log n): Logarithmic time complexity
- O(n): Linear time complexity
- O(n log n): Linearithmic time complexity
- O(n^2): Quadratic time complexity
- O(2^n): Exponential time complexity
2.2 Rules for Calculating Big O
When calculating Big O, follow these general rules:
- Drop constants: O(2n) becomes O(n)
- Drop lower-order terms: O(n^2 + n) becomes O(n^2)
- Consider the worst-case scenario
- Different inputs should use different variables: O(a + b) instead of O(n + n)
3. Common Complexity Classes
Let’s explore the most common complexity classes you’ll encounter in algorithm analysis.
3.1 O(1) – Constant Time
Constant time algorithms perform the same number of operations regardless of input size.
Example: Accessing an array element by index.
def get_element(arr, index):
return arr[index]
3.2 O(log n) – Logarithmic Time
Logarithmic time algorithms typically divide the problem in half with each step.
Example: Binary search in a sorted array.
def binary_search(arr, target):
left, right = 0, len(arr) - 1
while left <= right:
mid = (left + right) // 2
if arr[mid] == target:
return mid
elif arr[mid] < target:
left = mid + 1
else:
right = mid - 1
return -1
3.3 O(n) – Linear Time
Linear time algorithms process each input element once.
Example: Finding the maximum element in an unsorted array.
def find_max(arr):
max_val = arr[0]
for num in arr:
if num > max_val:
max_val = num
return max_val
3.4 O(n log n) – Linearithmic Time
Linearithmic time algorithms often involve dividing the input and then processing each part.
Example: Merge sort algorithm.
def merge_sort(arr):
if len(arr) <= 1:
return arr
mid = len(arr) // 2
left = merge_sort(arr[:mid])
right = merge_sort(arr[mid:])
return merge(left, right)
def merge(left, right):
result = []
i, j = 0, 0
while i < len(left) and j < len(right):
if left[i] < right[j]:
result.append(left[i])
i += 1
else:
result.append(right[j])
j += 1
result.extend(left[i:])
result.extend(right[j:])
return result
3.5 O(n^2) – Quadratic Time
Quadratic time algorithms often involve nested iterations over the input.
Example: Bubble sort algorithm.
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n - i - 1):
if arr[j] > arr[j + 1]:
arr[j], arr[j + 1] = arr[j + 1], arr[j]
return arr
3.6 O(2^n) – Exponential Time
Exponential time algorithms have a growth rate that doubles with each addition to the input.
Example: Recursive calculation of Fibonacci numbers (without memoization).
def fibonacci(n):
if n <= 1:
return n
return fibonacci(n - 1) + fibonacci(n - 2)
4. Space Complexity
While time complexity focuses on the number of operations, space complexity deals with the amount of memory an algorithm uses relative to the input size.
4.1 Types of Space Complexity
- O(1) – Constant space: The algorithm uses a fixed amount of memory regardless of input size.
- O(n) – Linear space: Memory usage grows linearly with input size.
- O(n^2) – Quadratic space: Memory usage grows quadratically with input size.
4.2 Example: Space Complexity Analysis
Consider the following function that creates a matrix:
def create_matrix(n):
matrix = []
for i in range(n):
row = [0] * n
matrix.append(row)
return matrix
This function has a space complexity of O(n^2) because it creates an n x n matrix, where the space required grows quadratically with the input size n.
5. Amortized Analysis
Amortized analysis is a method of analyzing the time complexity of algorithms that occasionally perform expensive operations but have a lower average-case time complexity.
5.1 Understanding Amortized Analysis
Amortized analysis considers the average performance of a sequence of operations, rather than the worst-case scenario for each operation. This is particularly useful for data structures like dynamic arrays (e.g., ArrayList in Java or list in Python) that occasionally need to resize but offer constant-time access on average.
5.2 Example: Dynamic Array Resizing
Consider a dynamic array that doubles its size when it reaches capacity:
class DynamicArray:
def __init__(self):
self.array = [None] * 1
self.size = 0
def append(self, element):
if self.size == len(self.array):
self._resize()
self.array[self.size] = element
self.size += 1
def _resize(self):
new_array = [None] * (len(self.array) * 2)
for i in range(self.size):
new_array[i] = self.array[i]
self.array = new_array
While the _resize() operation takes O(n) time, it occurs infrequently. The amortized time complexity of the append() operation is O(1), as the cost of occasional resizing is distributed across many append operations.
6. NP-Completeness and Beyond
As we venture into more advanced complexity classes, it’s important to understand NP-completeness and related concepts.
6.1 P vs NP
P (Polynomial time) problems are those that can be solved in polynomial time by a deterministic Turing machine. NP (Nondeterministic Polynomial time) problems are those whose solutions can be verified in polynomial time.
6.2 NP-Complete Problems
NP-Complete problems are the hardest problems in NP. If an efficient algorithm is found for any NP-Complete problem, it would imply that all NP problems are solvable in polynomial time (P = NP).
Examples of NP-Complete problems:
- Traveling Salesman Problem
- Boolean Satisfiability Problem (SAT)
- Knapsack Problem
6.3 Coping with NP-Complete Problems
When dealing with NP-Complete problems, consider these approaches:
- Approximation algorithms: Find a solution close to optimal in polynomial time.
- Heuristics: Use problem-specific insights to find good (but not necessarily optimal) solutions quickly.
- Parameterized algorithms: Solve the problem efficiently for small values of a certain parameter.
7. Practical Tips for Navigating Complexity Classes
As you work on algorithmic problems and prepare for technical interviews, keep these tips in mind:
7.1 Recognize Common Patterns
Learn to recognize algorithmic patterns and their associated complexity classes. For example:
- Single loop over input: Often O(n)
- Nested loops: Often O(n^2)
- Divide and conquer: Often O(n log n)
- Recursive with multiple branches: Often exponential
7.2 Analyze Trade-offs
Consider the trade-offs between time and space complexity. Sometimes, you can achieve better time complexity by using more memory, or vice versa.
7.3 Use Appropriate Data Structures
Choose data structures that offer efficient operations for your specific needs. For example:
- Hash tables for O(1) average-case lookup and insertion
- Binary search trees for O(log n) search, insertion, and deletion in sorted data
- Heaps for O(log n) insertion and extraction of minimum/maximum elements
7.4 Consider Input Size and Constraints
When solving problems, especially in competitive programming or interviews, pay attention to input size constraints. This can help you determine the maximum acceptable time complexity for your solution.
7.5 Practice, Practice, Practice
Regularly solve algorithmic problems and analyze their complexity. Platforms like LeetCode, HackerRank, and AlgoCademy offer a wide range of problems to practice on.
8. Conclusion
Understanding and navigating algorithm complexity classes is a fundamental skill for any programmer aiming to write efficient code and excel in technical interviews. By mastering the concepts of time and space complexity, recognizing common patterns, and practicing regularly, you’ll be well-equipped to tackle a wide range of algorithmic challenges.
Remember that while optimizing for the best time and space complexity is important, it’s equally crucial to write clear, maintainable code. Strive for a balance between efficiency and readability in your solutions.
As you continue your journey in programming and algorithm design, keep exploring new techniques and stay updated with the latest advancements in the field. With persistence and practice, you’ll develop the skills needed to navigate even the most complex algorithmic problems with confidence.