Mutex Locks for Linux Thread Synchronization in C/C++: A Comprehensive Guide
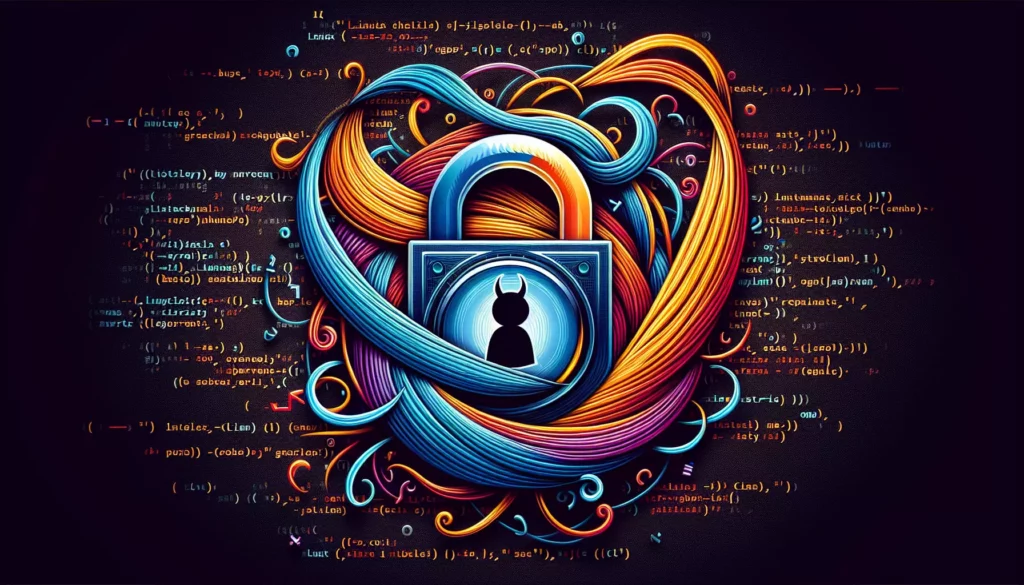
Introduction
In the world of multithreaded programming, synchronization is a crucial concept that ensures the correct execution of concurrent processes. One of the most fundamental synchronization primitives is the mutex lock. This article will dive deep into mutex locks for Linux thread synchronization in C and C++, exploring their importance, implementation, and best practices.
What is a Mutex Lock?
A mutex, short for “mutual exclusion,” is a synchronization mechanism used to protect shared resources from simultaneous access by multiple threads. It acts as a gatekeeper, allowing only one thread at a time to access a critical section of code or shared data.
The basic principle of a mutex is simple:
- When a thread wants to access a shared resource, it must first acquire the mutex lock.
- If the mutex is already locked by another thread, the requesting thread will be blocked until the mutex becomes available.
- Once the thread finishes using the shared resource, it releases the mutex, allowing other threads to acquire it.
Why Use Mutex Locks?
Mutex locks are essential in multithreaded programming for several reasons:
- Data Integrity: They prevent race conditions and ensure that shared data is not corrupted by concurrent access.
- Deadlock Prevention: Proper use of mutexes can help avoid deadlocks, where two or more threads are waiting for each other to release resources.
- Synchronization: Mutexes provide a way to synchronize the execution of multiple threads, ensuring that certain operations occur in a specific order.
- Performance: While they introduce some overhead, mutexes can improve overall performance by preventing data inconsistencies that could lead to errors and crashes.
Implementing Mutex Locks in C
In C, mutex locks are typically implemented using the POSIX threads (pthreads) library. Here’s a step-by-step guide to using mutex locks in C:
1. Include the necessary header
#include <pthread.h>
2. Declare a mutex variable
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
Alternatively, you can dynamically initialize the mutex:
pthread_mutex_t mutex;
pthread_mutex_init(&mutex, NULL);
3. Lock the mutex before entering a critical section
pthread_mutex_lock(&mutex);
4. Unlock the mutex after exiting the critical section
pthread_mutex_unlock(&mutex);
5. Destroy the mutex when it’s no longer needed
pthread_mutex_destroy(&mutex);
Complete Example in C
Here’s a complete example demonstrating the use of mutex locks in C:
#include <stdio.h>
#include <pthread.h>
#define NUM_THREADS 5
#define NUM_ITERATIONS 1000000
long long shared_counter = 0;
pthread_mutex_t mutex = PTHREAD_MUTEX_INITIALIZER;
void* increment_counter(void* arg) {
for (int i = 0; i < NUM_ITERATIONS; i++) {
pthread_mutex_lock(&mutex);
shared_counter++;
pthread_mutex_unlock(&mutex);
}
return NULL;
}
int main() {
pthread_t threads[NUM_THREADS];
for (int i = 0; i < NUM_THREADS; i++) {
pthread_create(&threads[i], NULL, increment_counter, NULL);
}
for (int i = 0; i < NUM_THREADS; i++) {
pthread_join(threads[i], NULL);
}
printf("Final counter value: %lld\n", shared_counter);
pthread_mutex_destroy(&mutex);
return 0;
}
In this example, we create multiple threads that increment a shared counter. The mutex ensures that only one thread can increment the counter at a time, preventing race conditions.
Implementing Mutex Locks in C++
C++ provides a more object-oriented approach to mutex locks through the <mutex> header, which was introduced in C++11. Here’s how to use mutex locks in C++:
1. Include the necessary header
#include <mutex>
2. Declare a mutex object
std::mutex mutex;
3. Lock the mutex
mutex.lock();
4. Unlock the mutex
mutex.unlock();
5. Use RAII with std::lock_guard or std::unique_lock
C++ provides RAII (Resource Acquisition Is Initialization) wrappers for mutexes, which automatically lock and unlock the mutex:
std::lock_guard<std::mutex> lock(mutex);
// Critical section
// lock_guard automatically unlocks when it goes out of scope
Complete Example in C++
Here’s a complete example demonstrating the use of mutex locks in C++:
#include <iostream>
#include <thread>
#include <mutex>
#include <vector>
#define NUM_THREADS 5
#define NUM_ITERATIONS 1000000
long long shared_counter = 0;
std::mutex mutex;
void increment_counter() {
for (int i = 0; i < NUM_ITERATIONS; i++) {
std::lock_guard<std::mutex> lock(mutex);
shared_counter++;
}
}
int main() {
std::vector<std::thread> threads;
for (int i = 0; i < NUM_THREADS; i++) {
threads.emplace_back(increment_counter);
}
for (auto& thread : threads) {
thread.join();
}
std::cout << "Final counter value: " << shared_counter << std::endl;
return 0;
}
This C++ example achieves the same result as the C example, but with a more modern and object-oriented approach.
Best Practices for Using Mutex Locks
To effectively use mutex locks and avoid common pitfalls, follow these best practices:
1. Keep Critical Sections Short
Minimize the amount of code inside a locked section to reduce contention and improve performance. Only lock the mutex for as long as necessary to protect the shared resource.
2. Use RAII in C++
Prefer using std::lock_guard or std::unique_lock in C++ to ensure that mutexes are always unlocked, even if an exception is thrown.
3. Avoid Nested Locks
Nesting mutex locks can lead to deadlocks. If you must use multiple locks, always acquire them in the same order across all threads.
4. Consider Using Read-Write Locks
For scenarios where you have many readers and few writers, consider using read-write locks (pthread_rwlock_t in C or std::shared_mutex in C++17) to allow multiple simultaneous readers.
5. Be Aware of Priority Inversion
Priority inversion can occur when a low-priority thread holds a lock needed by a high-priority thread. Use priority inheritance mutexes when necessary to mitigate this issue.
6. Use Condition Variables for Complex Synchronization
For more complex synchronization scenarios, combine mutexes with condition variables to implement efficient wait and signal mechanisms.
Common Pitfalls and How to Avoid Them
1. Deadlocks
Deadlocks occur when two or more threads are waiting for each other to release resources. To avoid deadlocks:
- Always acquire locks in a consistent order across all threads.
- Use std::lock or std::scoped_lock (C++17) to lock multiple mutexes atomically.
- Implement timeouts when acquiring locks to prevent indefinite waiting.
2. Priority Inversion
Priority inversion happens when a high-priority thread is indirectly preempted by a low-priority thread. To mitigate priority inversion:
- Use priority inheritance mutexes when working with real-time systems.
- Design your system to minimize the time that high-priority threads spend waiting for locks.
3. Convoy Effect
The convoy effect occurs when multiple threads of different priorities are waiting for a lock, causing lower-priority threads to delay higher-priority ones. To reduce the convoy effect:
- Keep critical sections as short as possible.
- Consider using fine-grained locking or lock-free data structures for frequently accessed resources.
4. Forgotten Unlocks
Forgetting to unlock a mutex can lead to deadlocks or resource leaks. To prevent this:
- In C++, use RAII wrappers like std::lock_guard or std::unique_lock.
- In C, use a consistent locking pattern and carefully review your code for matching lock/unlock pairs.
Advanced Mutex Techniques
1. Timed Mutex Locks
C++11 introduced timed mutex locks, which allow you to specify a timeout when trying to acquire a lock. This can be useful for avoiding deadlocks and implementing more robust error handling:
#include <mutex>
#include <chrono>
std::timed_mutex timed_mutex;
if (timed_mutex.try_lock_for(std::chrono::milliseconds(100))) {
// Lock acquired, perform operations
timed_mutex.unlock();
} else {
// Failed to acquire lock within 100ms
}
2. Recursive Mutexes
Recursive mutexes allow the same thread to lock the mutex multiple times without causing a deadlock. This can be useful in certain scenarios, but should be used carefully as it can make code harder to reason about:
#include <mutex>
std::recursive_mutex recursive_mutex;
void recursive_function(int depth) {
std::lock_guard<std::recursive_mutex> lock(recursive_mutex);
if (depth > 0) {
recursive_function(depth - 1);
}
}
3. Shared Mutexes (Read-Write Locks)
Shared mutexes, introduced in C++17, allow multiple readers to access a resource simultaneously while ensuring exclusive access for writers:
#include <shared_mutex>
std::shared_mutex shared_mutex;
// Reader
void read_data() {
std::shared_lock<std::shared_mutex> lock(shared_mutex);
// Read shared data
}
// Writer
void write_data() {
std::unique_lock<std::shared_mutex> lock(shared_mutex);
// Modify shared data
}
Mutex Alternatives and Lock-Free Programming
While mutexes are a fundamental synchronization primitive, there are scenarios where alternative approaches may be more appropriate:
1. Atomic Operations
For simple operations on basic data types, atomic operations can provide thread-safe access without the overhead of a mutex:
#include <atomic>
std::atomic<int> counter(0);
void increment() {
counter.fetch_add(1, std::memory_order_relaxed);
}
2. Lock-Free Data Structures
Lock-free data structures use atomic operations and clever algorithms to provide thread-safe access without explicit locking. These can offer better performance in high-contention scenarios:
#include <atomic>
template <typename T>
class LockFreeStack {
private:
struct Node {
T data;
Node* next;
Node(const T& data) : data(data), next(nullptr) {}
};
std::atomic<Node*> head;
public:
void push(const T& data) {
Node* new_node = new Node(data);
new_node->next = head.load(std::memory_order_relaxed);
while (!head.compare_exchange_weak(new_node->next, new_node,
std::memory_order_release,
std::memory_order_relaxed));
}
bool pop(T& result) {
Node* old_head = head.load(std::memory_order_relaxed);
do {
if (old_head == nullptr)
return false;
} while (!head.compare_exchange_weak(old_head, old_head->next,
std::memory_order_acquire,
std::memory_order_relaxed));
result = old_head->data;
delete old_head;
return true;
}
};
3. Memory Models and Ordering
C++11 introduced a standardized memory model and memory ordering options for atomic operations. Understanding these can help you write more efficient and correct lock-free code:
#include <atomic>
#include <thread>
std::atomic<bool> flag(false);
std::atomic<int> data(0);
void producer() {
data.store(42, std::memory_order_relaxed);
flag.store(true, std::memory_order_release);
}
void consumer() {
while (!flag.load(std::memory_order_acquire));
assert(data.load(std::memory_order_relaxed) == 42);
}
Conclusion
Mutex locks are a crucial tool for ensuring thread safety in multithreaded applications. By understanding how to implement and use them effectively in both C and C++, you can write robust and efficient concurrent programs. Remember to follow best practices, be aware of common pitfalls, and consider alternative synchronization techniques when appropriate.
As you gain experience with mutex locks and other synchronization primitives, you’ll develop a better intuition for designing and implementing thread-safe systems. Keep exploring advanced topics like lock-free programming and memory models to further enhance your skills in concurrent programming.
With the knowledge gained from this comprehensive guide, you’re well-equipped to tackle complex multithreading challenges and create high-performance, thread-safe applications in C and C++.