MongoDB Aggregation: Unleashing the Power of Data Processing
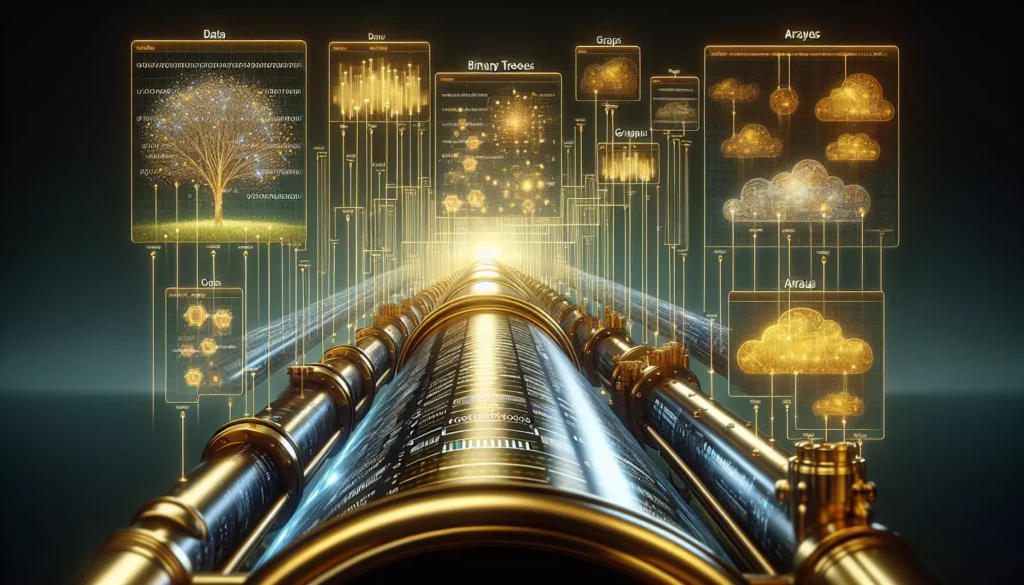
In the world of database management and data processing, MongoDB has emerged as a powerful player, especially when it comes to handling large volumes of unstructured or semi-structured data. One of the most potent features of MongoDB is its aggregation framework, which allows developers to perform complex data analysis and transformation operations with ease. In this comprehensive guide, we’ll dive deep into MongoDB aggregation, exploring its concepts, use cases, and best practices.
Table of Contents
- What is MongoDB Aggregation?
- The Aggregation Pipeline
- Common Aggregation Stages
- Aggregation Operators
- Real-World Use Cases
- Performance Optimization
- Comparison with SQL
- Best Practices
- Conclusion
What is MongoDB Aggregation?
MongoDB aggregation is a powerful framework that allows you to process and analyze data within the database itself. It provides a way to perform complex operations on collections of documents, transforming them into aggregated results. The aggregation framework is designed to handle large datasets efficiently, making it an excellent choice for data analysis and reporting tasks.
At its core, MongoDB aggregation is built around the concept of a pipeline. This pipeline consists of multiple stages, each performing a specific operation on the input documents. The output of one stage becomes the input for the next, allowing you to create sophisticated data processing workflows.
The Aggregation Pipeline
The aggregation pipeline is the heart of MongoDB’s aggregation framework. It consists of one or more stages that process documents sequentially. Each stage performs a specific operation on the input documents and passes the results to the next stage. This pipeline approach allows you to break down complex queries into smaller, more manageable steps.
Here’s a simple example of an aggregation pipeline:
db.orders.aggregate([
{ $match: { status: "completed" } },
{ $group: { _id: "$customer_id", total: { $sum: "$amount" } } },
{ $sort: { total: -1 } }
])
In this example, we have three stages:
- $match: Filters the documents to include only completed orders.
- $group: Groups the documents by customer_id and calculates the total amount for each customer.
- $sort: Sorts the results by total amount in descending order.
The power of the aggregation pipeline lies in its flexibility and the ability to combine multiple stages to perform complex data transformations and analysis.
Common Aggregation Stages
MongoDB provides a wide range of aggregation stages to help you process and analyze your data. Here are some of the most commonly used stages:
1. $match
The $match stage filters the documents to pass only those that match the specified condition(s). It’s often used as the first stage in a pipeline to reduce the number of documents to process.
{ $match: { age: { $gte: 18 } } }
2. $group
The $group stage groups documents by a specified expression and can perform various aggregations on the grouped data.
{ $group: { _id: "$category", avgPrice: { $avg: "$price" } } }
3. $sort
The $sort stage sorts the documents based on the specified field(s) in ascending or descending order.
{ $sort: { score: -1 } }
4. $project
The $project stage reshapes documents by specifying which fields to include, exclude, or add.
{ $project: { name: 1, age: 1, _id: 0 } }
5. $limit and $skip
These stages are used for pagination. $limit restricts the number of documents passed to the next stage, while $skip skips a specified number of documents.
{ $skip: 10 },
{ $limit: 5 }
6. $unwind
The $unwind stage deconstructs an array field from the input documents to output a document for each element.
{ $unwind: "$tags" }
7. $lookup
The $lookup stage performs a left outer join to another collection in the same database.
{
$lookup: {
from: "inventory",
localField: "item",
foreignField: "sku",
as: "inventory_docs"
}
}
Aggregation Operators
In addition to stages, MongoDB aggregation framework provides a rich set of operators that can be used within the stages to perform various operations on the data. Here are some important categories of operators:
1. Arithmetic Operators
These operators perform mathematical operations on numeric values.
- $add: Adds numbers together or adds numbers and a date.
- $subtract: Subtracts two numbers or a date and a number of milliseconds.
- $multiply: Multiplies numbers together.
- $divide: Divides one number by another.
2. Array Operators
These operators work with array fields in documents.
- $size: Returns the number of elements in an array.
- $filter: Selects a subset of an array based on the specified condition.
- $map: Applies an expression to each element in an array and returns the results.
3. Comparison Operators
These operators compare values and return boolean results.
- $eq: Matches values that are equal to a specified value.
- $gt, $gte: Matches values greater than (or equal to) a specified value.
- $lt, $lte: Matches values less than (or equal to) a specified value.
- $ne: Matches values that are not equal to a specified value.
4. Conditional Operators
These operators add conditional logic to the aggregation pipeline.
- $cond: A ternary operator that returns one of two expressions based on a condition.
- $ifNull: Returns an alternative value if the specified expression evaluates to null.
5. Date Operators
These operators work with date fields.
- $year, $month, $dayOfMonth: Extract the respective parts from a date.
- $dateToString: Converts a date to a formatted string.
Real-World Use Cases
MongoDB aggregation is versatile and can be applied to various real-world scenarios. Here are some common use cases:
1. Sales Analytics
Aggregation can be used to analyze sales data, calculate total revenue by product category, identify top-selling items, or compute average order values.
db.sales.aggregate([
{ $group: {
_id: "$product_category",
totalRevenue: { $sum: "$amount" },
averageOrderValue: { $avg: "$amount" }
}
},
{ $sort: { totalRevenue: -1 } }
])
2. User Behavior Analysis
For applications tracking user interactions, aggregation can help analyze user behavior patterns, session durations, or feature usage frequencies.
db.user_actions.aggregate([
{ $group: {
_id: "$user_id",
totalSessions: { $sum: 1 },
avgSessionDuration: { $avg: "$session_duration" }
}
},
{ $sort: { totalSessions: -1 } },
{ $limit: 10 }
])
3. Content Recommendation
Aggregation can be used to build recommendation systems by analyzing user preferences and content interactions.
db.user_preferences.aggregate([
{ $match: { user_id: "user123" } },
{ $unwind: "$liked_categories" },
{ $group: {
_id: "$liked_categories",
count: { $sum: 1 }
}
},
{ $sort: { count: -1 } },
{ $limit: 5 }
])
4. Geospatial Analysis
MongoDB’s geospatial features can be combined with aggregation for location-based analysis.
db.restaurants.aggregate([
{ $geoNear: {
near: { type: "Point", coordinates: [-73.93414657, 40.82302903] },
distanceField: "distance",
maxDistance: 1000,
spherical: true
}
},
{ $group: {
_id: "$cuisine",
count: { $sum: 1 }
}
},
{ $sort: { count: -1 } }
])
Performance Optimization
While MongoDB’s aggregation framework is powerful, it’s important to optimize your queries for better performance, especially when dealing with large datasets. Here are some tips to improve aggregation performance:
1. Use Indexes
Ensure that the fields used in $match and $sort stages are properly indexed. This can significantly speed up the filtering and sorting operations.
2. Limit Documents Early
Use $match stages early in your pipeline to reduce the number of documents processed in subsequent stages.
3. Avoid Unnecessary Stages
Combine operations where possible to reduce the number of stages in your pipeline.
4. Use Aggregation Pipeline Operators
Prefer using built-in pipeline operators over custom JavaScript functions, as they are optimized for performance.
5. Consider using $limit and $skip for Pagination
When implementing pagination, use $limit and $skip judiciously to avoid processing unnecessary documents.
6. Monitor and Profile
Use MongoDB’s profiling tools to identify slow queries and optimize them.
Comparison with SQL
For those familiar with SQL, it can be helpful to draw parallels between MongoDB aggregation and SQL operations. Here’s a quick comparison:
MongoDB Aggregation | SQL Equivalent |
---|---|
$match | WHERE |
$group | GROUP BY |
$sort | ORDER BY |
$limit | LIMIT |
$skip | OFFSET |
$project | SELECT |
$lookup | LEFT OUTER JOIN |
While these comparisons provide a general idea, it’s important to note that MongoDB’s aggregation framework is more flexible and powerful in many ways, especially when dealing with nested documents and arrays.
Best Practices
To make the most of MongoDB aggregation, consider following these best practices:
1. Plan Your Pipeline
Before writing your aggregation query, plan out the stages and operations you need. This can help you optimize the pipeline and avoid unnecessary stages.
2. Use Appropriate Data Types
Ensure that your data is stored using appropriate data types. This can improve query performance and make aggregations more straightforward.
3. Leverage Atlas Features
If you’re using MongoDB Atlas, take advantage of features like Atlas Search for full-text search capabilities within your aggregation pipelines.
4. Test with Representative Data
Always test your aggregation queries with a dataset that represents your production data in terms of size and complexity.
5. Use Aggregation Expressions
Utilize MongoDB’s rich set of aggregation expressions to perform complex calculations and transformations within your pipeline.
6. Consider Memory Limitations
Be aware of the 100MB memory limit for aggregation operations. For operations that may exceed this limit, consider using the $out stage to output results to a new collection.
7. Document Your Aggregations
Complex aggregation pipelines can be difficult to understand at a glance. Add comments to your code and maintain documentation for your aggregation queries.
Conclusion
MongoDB’s aggregation framework is a powerful tool for data processing and analysis. It offers a flexible and efficient way to transform and analyze large volumes of data directly within the database. By understanding the various stages, operators, and best practices, you can leverage MongoDB aggregation to build sophisticated data processing pipelines and extract valuable insights from your data.
As you continue to work with MongoDB aggregation, you’ll discover even more ways to optimize and enhance your data operations. Remember that practice and experimentation are key to mastering this powerful feature. Whether you’re building a complex analytics system or simply need to generate reports from your MongoDB data, the aggregation framework provides the tools you need to get the job done efficiently and effectively.
Keep exploring, keep learning, and don’t hesitate to dive deep into MongoDB’s documentation for more advanced features and optimizations. Happy aggregating!