Mobile Security and Privacy: Protecting Your Apps from Threats
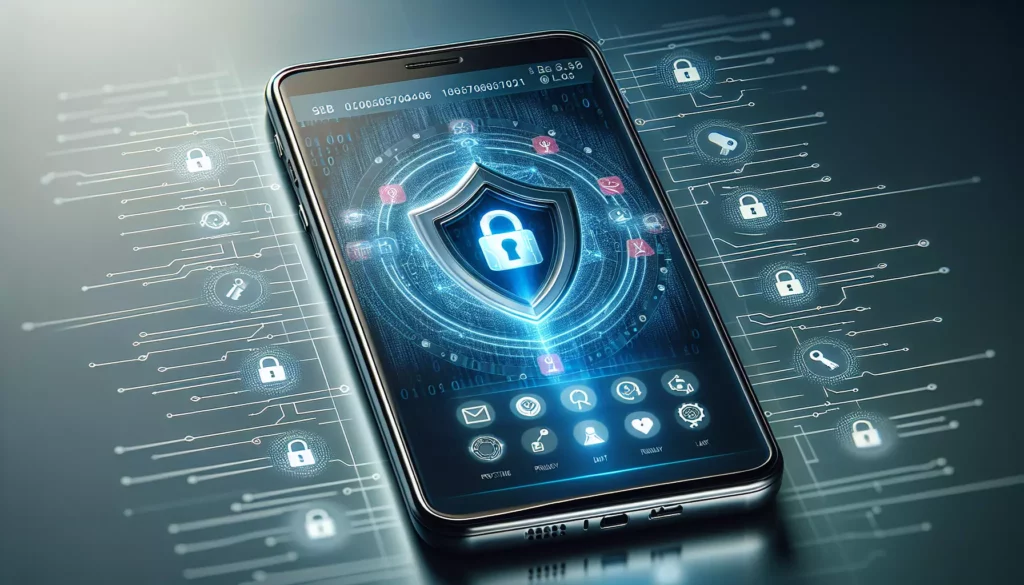
In today’s digital age, mobile devices have become an integral part of our lives. We use them for communication, entertainment, work, and countless other purposes. With the increasing reliance on mobile applications, it’s crucial to prioritize mobile security and privacy. This comprehensive guide will explore the various aspects of mobile security, common threats, and strategies to protect your mobile applications from potential security breaches.
Table of Contents
- Understanding Mobile Security
- Common Mobile Security Threats
- Best Practices for Mobile App Security
- Secure Coding Techniques
- Data Encryption and Protection
- Secure Authentication and Authorization
- Network Security
- Third-party Libraries and APIs
- Security Testing and Auditing
- Privacy Considerations
- Staying Updated on Security Trends
- Conclusion
1. Understanding Mobile Security
Mobile security refers to the measures taken to protect mobile devices, such as smartphones and tablets, as well as the data stored on them from various threats and vulnerabilities. It encompasses a wide range of practices, technologies, and strategies aimed at safeguarding both the hardware and software aspects of mobile devices.
The importance of mobile security cannot be overstated, especially in the context of mobile application development. As developers, it’s our responsibility to ensure that the apps we create are not only functional but also secure and respectful of user privacy. This involves implementing robust security measures, following best practices, and staying informed about the latest security threats and mitigation techniques.
2. Common Mobile Security Threats
Before diving into protection strategies, it’s essential to understand the various threats that mobile applications face. Here are some of the most common mobile security threats:
- Malware: Malicious software designed to infiltrate and damage mobile devices, including viruses, trojans, and spyware.
- Phishing: Attempts to trick users into revealing sensitive information through fake websites or messages.
- Data Leakage: Unauthorized access or transmission of sensitive data from mobile devices.
- Insecure Network Connections: Vulnerabilities in Wi-Fi or cellular networks that can be exploited by attackers.
- Device Loss or Theft: Physical loss of mobile devices, potentially exposing stored data.
- Unsecured APIs: Poorly implemented or unprotected application programming interfaces that can be exploited.
- Reverse Engineering: The process of analyzing and modifying an app’s code to understand its functionality or introduce malicious changes.
- Man-in-the-Middle (MitM) Attacks: Interception of communication between two parties to eavesdrop or manipulate data.
3. Best Practices for Mobile App Security
To protect mobile applications from security threats, developers should adhere to the following best practices:
- Implement Secure Communication: Use HTTPS for all network communications and implement certificate pinning to prevent MitM attacks.
- Secure Data Storage: Encrypt sensitive data stored on the device and use secure storage mechanisms provided by the operating system.
- Input Validation: Validate and sanitize all user inputs to prevent injection attacks and other vulnerabilities.
- Implement Proper Authentication: Use strong authentication mechanisms, such as multi-factor authentication, and implement secure session management.
- Code Obfuscation: Obfuscate your app’s code to make it more difficult for attackers to reverse engineer and understand its functionality.
- Secure Backend APIs: Implement proper authentication and authorization for all backend APIs used by your mobile app.
- Regular Updates: Keep your app updated with the latest security patches and improvements.
- Minimize Permissions: Request only the necessary permissions for your app to function properly.
- Implement Secure Logout: Ensure that all sensitive data and tokens are properly cleared when a user logs out of the app.
- Use Secure Third-party Libraries: Carefully vet and regularly update any third-party libraries used in your app.
4. Secure Coding Techniques
Implementing secure coding techniques is crucial for developing robust and secure mobile applications. Here are some key practices to follow:
4.1. Input Validation and Sanitization
Always validate and sanitize user inputs to prevent injection attacks and other security vulnerabilities. Here’s an example of input validation in Java:
public boolean isValidEmail(String email) {
String emailRegex = "^[A-Za-z0-9+_.-]+@[A-Za-z0-9.-]+$";
Pattern pattern = Pattern.compile(emailRegex);
if (email == null) {
return false;
}
return pattern.matcher(email).matches();
}
4.2. Avoid Hardcoding Sensitive Information
Never hardcode sensitive information such as API keys, passwords, or encryption keys directly in your code. Instead, use secure storage mechanisms or environment variables. Here’s an example of how to retrieve a sensitive value from secure storage in Android:
private String getApiKey() {
KeyStore keyStore = KeyStore.getInstance("AndroidKeyStore");
keyStore.load(null);
KeyStore.SecretKeyEntry secretKeyEntry = (KeyStore.SecretKeyEntry) keyStore.getEntry("api_key", null);
byte[] encodedKey = secretKeyEntry.getSecretKey().getEncoded();
return Base64.encodeToString(encodedKey, Base64.DEFAULT);
}
4.3. Use Parameterized Queries
When working with databases, always use parameterized queries to prevent SQL injection attacks. Here’s an example using SQLite in Android:
public void insertUser(String username, String password) {
SQLiteDatabase db = dbHelper.getWritableDatabase();
ContentValues values = new ContentValues();
values.put("username", username);
values.put("password", password);
db.insert("users", null, values);
db.close();
}
4.4. Implement Proper Error Handling
Implement proper error handling to prevent sensitive information from being exposed in error messages. Here’s an example of how to handle exceptions securely:
try {
// Perform some operation
} catch (Exception e) {
Log.e("SecureApp", "An error occurred", e);
// Display a generic error message to the user
showErrorDialog("An unexpected error occurred. Please try again later.");
}
5. Data Encryption and Protection
Protecting sensitive data through encryption is a crucial aspect of mobile app security. Here are some key considerations and techniques:
5.1. Encryption at Rest
Encrypt sensitive data stored on the device using strong encryption algorithms. Here’s an example of how to encrypt data using AES encryption in Java:
public static byte[] encrypt(byte[] key, byte[] data) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key, "AES");
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
return cipher.doFinal(data);
}
5.2. Encryption in Transit
Always use HTTPS for network communications to ensure data is encrypted during transmission. Here’s an example of how to configure an OkHttpClient to use HTTPS in Android:
OkHttpClient client = new OkHttpClient.Builder()
.connectTimeout(10, TimeUnit.SECONDS)
.writeTimeout(10, TimeUnit.SECONDS)
.readTimeout(30, TimeUnit.SECONDS)
.followRedirects(true)
.followSslRedirects(true)
.retryOnConnectionFailure(true)
.cache(null)
.build();
5.3. Key Management
Implement proper key management practices to securely store and handle encryption keys. Use the Android Keystore System or iOS Keychain to store cryptographic keys securely.
6. Secure Authentication and Authorization
Implementing secure authentication and authorization mechanisms is crucial for protecting user accounts and sensitive data. Consider the following practices:
6.1. Multi-factor Authentication
Implement multi-factor authentication to add an extra layer of security. This can include something the user knows (password), something they have (device or token), or something they are (biometric).
6.2. Secure Password Storage
Never store passwords in plain text. Instead, use strong hashing algorithms like bcrypt or Argon2 to securely store password hashes. Here’s an example of how to hash a password using bcrypt in Java:
import org.mindrot.jbcrypt.BCrypt;
public String hashPassword(String password) {
return BCrypt.hashpw(password, BCrypt.gensalt());
}
public boolean checkPassword(String password, String hashedPassword) {
return BCrypt.checkpw(password, hashedPassword);
}
6.3. JWT for Authorization
Use JSON Web Tokens (JWT) for secure authorization. Here’s an example of how to create and verify JWTs using the jjwt library in Java:
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.SignatureAlgorithm;
import io.jsonwebtoken.security.Keys;
private static final Key key = Keys.secretKeyFor(SignatureAlgorithm.HS256);
public String createJWT(String subject) {
return Jwts.builder()
.setSubject(subject)
.signWith(key)
.compact();
}
public boolean verifyJWT(String jwt) {
try {
Jwts.parserBuilder().setSigningKey(key).build().parseClaimsJws(jwt);
return true;
} catch (Exception e) {
return false;
}
}
7. Network Security
Ensuring the security of network communications is vital for protecting mobile applications from various attacks. Consider the following practices:
7.1. Certificate Pinning
Implement certificate pinning to prevent man-in-the-middle attacks by ensuring your app only communicates with trusted servers. Here’s an example of how to implement certificate pinning using OkHttp in Android:
private static final String HOSTNAME = "api.example.com";
private static final int CERTIFICATE_PIN = 0x1234abcd; // SHA-256 hash of the certificate's public key
private OkHttpClient createPinnedClient() {
CertificatePinner certificatePinner = new CertificatePinner.Builder()
.add(HOSTNAME, "sha256/" + Integer.toHexString(CERTIFICATE_PIN))
.build();
return new OkHttpClient.Builder()
.certificatePinner(certificatePinner)
.build();
}
7.2. Secure WebView Implementation
When using WebViews in your mobile app, ensure they are configured securely to prevent potential vulnerabilities. Here’s an example of how to configure a secure WebView in Android:
WebView webView = findViewById(R.id.webView);
WebSettings webSettings = webView.getSettings();
webSettings.setJavaScriptEnabled(true);
webSettings.setAllowFileAccess(false);
webSettings.setAllowContentAccess(false);
webSettings.setAllowFileAccessFromFileURLs(false);
webSettings.setAllowUniversalAccessFromFileURLs(false);
webView.setWebViewClient(new WebViewClient() {
@Override
public boolean shouldOverrideUrlLoading(WebView view, String url) {
// Implement custom URL handling logic
return true;
}
});
8. Third-party Libraries and APIs
When integrating third-party libraries and APIs into your mobile application, it’s crucial to ensure they don’t introduce security vulnerabilities. Follow these best practices:
8.1. Vetting Third-party Libraries
Carefully evaluate third-party libraries before integrating them into your app. Consider the following factors:
- Reputation and community support
- Regular updates and maintenance
- Known security vulnerabilities
- Licensing and compliance requirements
8.2. Keeping Dependencies Updated
Regularly update your app’s dependencies to ensure you have the latest security patches. Use dependency management tools like Gradle for Android or CocoaPods for iOS to manage and update your dependencies efficiently.
8.3. Secure API Integration
When integrating external APIs, implement proper authentication and authorization mechanisms. Use API keys, OAuth, or other secure authentication methods provided by the API. Here’s an example of how to securely integrate an API using Retrofit in Android:
public interface ApiService {
@GET("users")
Call<List<User>> getUsers(@Header("Authorization") String authToken);
}
OkHttpClient client = new OkHttpClient.Builder()
.addInterceptor(new Interceptor() {
@Override
public Response intercept(Chain chain) throws IOException {
Request original = chain.request();
Request request = original.newBuilder()
.header("Authorization", "Bearer " + getAuthToken())
.method(original.method(), original.body())
.build();
return chain.proceed(request);
}
})
.build();
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://api.example.com/")
.addConverterFactory(GsonConverterFactory.create())
.client(client)
.build();
ApiService apiService = retrofit.create(ApiService.class);
9. Security Testing and Auditing
Regular security testing and auditing are essential to identify and address potential vulnerabilities in your mobile application. Consider the following approaches:
9.1. Static Analysis
Use static analysis tools to scan your codebase for potential security issues. Tools like SonarQube, FindBugs, or Android Lint can help identify common security vulnerabilities.
9.2. Dynamic Analysis
Perform dynamic analysis by testing your app in a running state. Use tools like OWASP ZAP or Burp Suite to identify runtime vulnerabilities and security issues.
9.3. Penetration Testing
Conduct regular penetration testing to simulate real-world attacks on your mobile application. This can help identify vulnerabilities that may not be apparent through other testing methods.
9.4. Automated Security Testing
Integrate automated security testing into your CI/CD pipeline to catch potential security issues early in the development process. Here’s an example of how to integrate security testing using the OWASP Dependency-Check tool in a Gradle build file:
plugins {
id "org.owasp.dependencycheck" version "6.2.2"
}
apply plugin: "org.owasp.dependencycheck"
dependencyCheck {
failBuildOnCVSS = 7
formats = ["HTML", "JSON"]
outputDirectory = "$buildDir/reports/dependency-check"
suppressionFile = "$projectDir/dependency-check-suppressions.xml"
}
10. Privacy Considerations
Respecting user privacy is not only a legal requirement in many jurisdictions but also crucial for building trust with your users. Consider the following privacy best practices:
10.1. Data Minimization
Collect and store only the minimum amount of user data necessary for your app to function. Avoid collecting unnecessary personal information.
10.2. Transparent Privacy Policies
Clearly communicate your app’s data collection and usage practices through a comprehensive privacy policy. Make sure users can easily access and understand this information.
10.3. User Consent
Obtain explicit user consent before collecting or processing sensitive data. Implement proper consent management mechanisms in your app. Here’s an example of how to request user consent in Android:
private void requestConsent() {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setTitle("Data Collection Consent");
builder.setMessage("We collect usage data to improve our app. Do you consent to this data collection?");
builder.setPositiveButton("I Consent", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// User consented, save the consent status
saveConsentStatus(true);
}
});
builder.setNegativeButton("I Do Not Consent", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
// User did not consent, save the consent status
saveConsentStatus(false);
}
});
builder.show();
}
private void saveConsentStatus(boolean consented) {
SharedPreferences prefs = getSharedPreferences("AppPrefs", MODE_PRIVATE);
SharedPreferences.Editor editor = prefs.edit();
editor.putBoolean("user_consented", consented);
editor.apply();
}
10.4. Data Retention and Deletion
Implement proper data retention policies and provide users with the ability to delete their data from your app. Ensure that data is securely and completely removed when requested.
11. Staying Updated on Security Trends
The field of mobile security is constantly evolving, with new threats and vulnerabilities emerging regularly. To stay ahead of potential security risks, consider the following practices:
11.1. Follow Security Blogs and Publications
Stay informed about the latest security trends, vulnerabilities, and best practices by following reputable security blogs and publications. Some recommended resources include:
- OWASP Mobile Security Project
- Google’s Android Security Blog
- Apple’s Security Updates page
- NowSecure Blog
- Mobile Security Framework (MobSF) GitHub repository
11.2. Attend Security Conferences and Webinars
Participate in security conferences, webinars, and workshops to learn from industry experts and stay up-to-date with the latest security trends and techniques.
11.3. Join Security Communities
Engage with other mobile security professionals by joining online communities and forums. This can provide valuable insights, allow you to share experiences, and stay informed about emerging threats.
11.4. Continuous Learning and Skill Development
Invest in continuous learning and skill development to enhance your mobile security expertise. Consider obtaining relevant certifications, such as:
- GIAC Mobile Device Security Analyst (GMOB)
- EC-Council Certified Mobile Application Security Tester (CMAST)
- SANS SEC575: Mobile Device Security and Ethical Hacking
12. Conclusion
Mobile security and privacy are critical aspects of developing robust and trustworthy mobile applications. By implementing the best practices and techniques discussed in this guide, you can significantly enhance the security posture of your mobile apps and protect your users from potential threats.
Remember that mobile security is an ongoing process that requires constant vigilance and adaptation to new threats and vulnerabilities. Stay informed about the latest security trends, regularly update your apps and dependencies, and conduct thorough security testing to ensure your mobile applications remain secure and respect user privacy.
By prioritizing mobile security and privacy in your development process, you not only protect your users but also build trust and credibility for your mobile applications in an increasingly security-conscious digital landscape.