Minimum Number of Platforms: Solving the Interval Overlap Problem
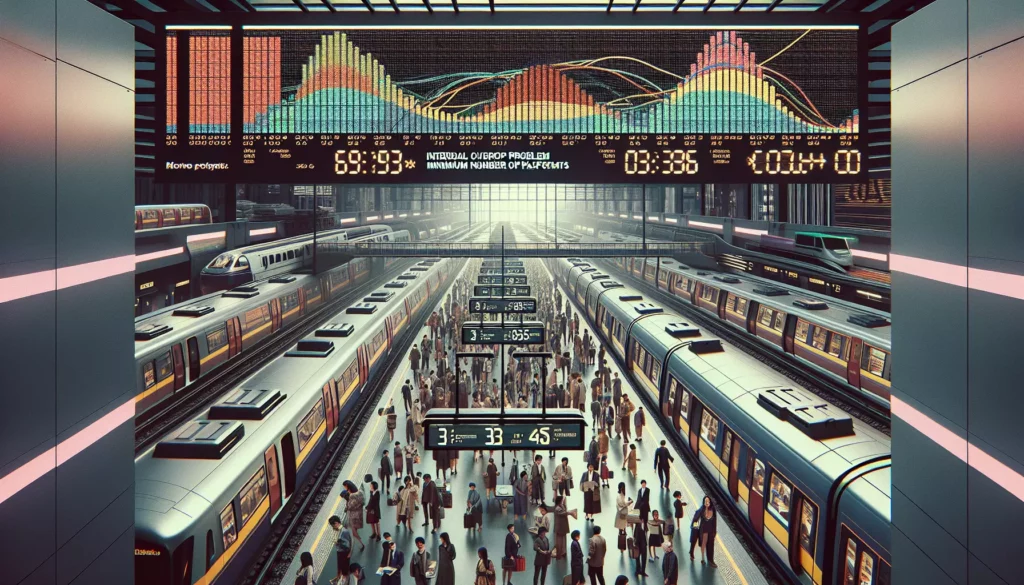
Welcome to another exciting tutorial from AlgoCademy! Today, we’re diving deep into a classic algorithmic problem that’s not only fascinating but also highly relevant to real-world scenarios. We’ll be exploring the “Minimum Number of Platforms” problem, also known as the “Interval Overlap” problem. This problem is a favorite among interviewers at top tech companies, so mastering it will definitely boost your coding skills and interview preparedness.
Understanding the Problem
Before we jump into the solution, let’s clearly define the problem:
Given a schedule of train arrivals and departures, determine the minimum number of platforms required at a railway station to accommodate all trains without any conflict.
In other words, we need to find the maximum number of trains that can be present at the station simultaneously at any given time.
Input Format
- An array of arrival times
- An array of departure times
- Both arrays are of equal length, n, where n is the number of trains
- Times are given in 24-hour format as integers (e.g., 0900 for 9:00 AM, 1730 for 5:30 PM)
Output
An integer representing the minimum number of platforms required.
Approaching the Problem
This problem is a classic example of interval scheduling, which has applications in various domains such as resource allocation, event planning, and of course, train scheduling. The key to solving this problem efficiently lies in recognizing that we don’t need to process the entire schedule chronologically. Instead, we can focus on the critical points where the number of platforms needed changes – the arrival and departure times.
Naive Approach
A straightforward but inefficient approach would be to simulate the entire day, incrementing a counter for each arrival and decrementing it for each departure. While this would work, it’s not optimal for large datasets or when the time range is extensive.
Efficient Approach
A more efficient solution involves the following steps:
- Sort the arrival and departure times separately.
- Use two pointers to iterate through the sorted arrays.
- Keep track of the platforms needed and the maximum platforms required.
Let’s implement this solution step by step.
Implementing the Solution
We’ll implement the solution in Python, but the concept can be easily translated to other programming languages.
Step 1: Sort the Arrays
First, we’ll sort both the arrival and departure arrays. This allows us to process events in chronological order.
def min_platforms(arrival, departure):
n = len(arrival)
arrival.sort()
departure.sort()
# Rest of the code will go here
Step 2: Initialize Variables
We’ll need variables to keep track of the current number of platforms in use, the maximum number of platforms needed, and indices for iterating through the arrival and departure arrays.
platforms_needed = 1
max_platforms = 1
i = 1 # pointer for arrival array
j = 0 # pointer for departure array
Step 3: Iterate Through Events
Now, we’ll iterate through the events (arrivals and departures) in chronological order. We’ll increment the platform count for arrivals and decrement it for departures.
while i < n and j < n:
if arrival[i] <= departure[j]:
platforms_needed += 1
i += 1
elif arrival[i] > departure[j]:
platforms_needed -= 1
j += 1
if platforms_needed > max_platforms:
max_platforms = platforms_needed
return max_platforms
Complete Solution
Here’s the complete Python implementation:
def min_platforms(arrival, departure):
n = len(arrival)
arrival.sort()
departure.sort()
platforms_needed = 1
max_platforms = 1
i = 1 # pointer for arrival array
j = 0 # pointer for departure array
while i < n and j < n:
if arrival[i] <= departure[j]:
platforms_needed += 1
i += 1
elif arrival[i] > departure[j]:
platforms_needed -= 1
j += 1
if platforms_needed > max_platforms:
max_platforms = platforms_needed
return max_platforms
# Example usage
arrival = [900, 940, 950, 1100, 1500, 1800]
departure = [910, 1200, 1120, 1130, 1900, 2000]
print(min_platforms(arrival, departure)) # Output: 3
Time and Space Complexity Analysis
Let’s analyze the efficiency of our solution:
Time Complexity
- Sorting the arrival and departure arrays takes O(n log n) time, where n is the number of trains.
- The while loop iterates through all events once, which takes O(n) time.
- Therefore, the overall time complexity is O(n log n), dominated by the sorting step.
Space Complexity
- We only use a constant amount of extra space for our variables.
- The sorting algorithms may use additional space depending on the implementation, but typically it’s O(log n) for in-place sorting algorithms like Quicksort.
- Thus, the space complexity is O(log n) or O(1) if we consider only the additional space used by our algorithm.
Understanding the Algorithm
To truly grasp how this algorithm works, let’s break it down step by step:
- Sorting: By sorting both arrays, we ensure that we process events in chronological order. This is crucial for keeping track of overlapping intervals.
- Two Pointers: We use two pointers, i and j, to iterate through the arrival and departure arrays respectively. This allows us to process arrivals and departures in order.
- Platform Count: We increment the platform count for each arrival and decrement it for each departure. This directly represents the number of platforms in use at any given time.
- Maximum Platforms: By keeping track of the maximum number of platforms ever needed, we ensure we find the solution to our problem.
The key insight is that we don’t need to know which specific trains are on which platforms. We only need to know how many platforms are in use at any given time.
Variations and Extensions
The “Minimum Number of Platforms” problem is a specific instance of a more general class of problems. Let’s explore some variations and extensions:
1. Meeting Rooms II
This is essentially the same problem, but instead of train platforms, we’re dealing with meeting rooms. Given a list of meeting time intervals, find the minimum number of conference rooms required.
2. Hotel Bookings Possible
Given arrival and departure times of all guests, determine if it’s possible to accommodate all guests with a given number of rooms.
3. CPU Load Balancing
Given start and end times of CPU tasks, find the maximum number of CPUs needed at any point to handle all tasks.
4. Skyline Problem
While more complex, the skyline problem uses similar concepts of processing events in order and keeping track of maximum heights.
Real-world Applications
Understanding and solving the Minimum Number of Platforms problem can be incredibly valuable in various real-world scenarios:
1. Transportation Systems
Beyond train platforms, this algorithm can be applied to optimize bus stops, airport gates, or even parking spaces in a smart parking system.
2. Resource Allocation in Cloud Computing
Cloud providers can use similar algorithms to determine the minimum number of servers needed to handle varying loads throughout the day.
3. Event Planning
Event organizers can use this approach to determine the number of venues or rooms needed for a conference with multiple parallel sessions.
4. Hospital Resource Management
Hospitals can apply this concept to optimize the number of operating rooms or specialized equipment needed based on scheduled procedures.
Common Pitfalls and How to Avoid Them
When solving the Minimum Number of Platforms problem, there are several common mistakes that programmers often make. Let’s discuss these pitfalls and how to avoid them:
1. Not Sorting the Arrays
Pitfall: Forgetting to sort the arrival and departure arrays.
Solution: Always start by sorting both arrays. This is crucial for the algorithm to work correctly.
2. Incorrect Handling of Equal Times
Pitfall: Not properly handling cases where an arrival and departure occur at the same time.
Solution: In our implementation, we process arrivals before departures when times are equal (arrival[i]
3. Off-by-One Errors
Pitfall: Incorrectly initializing or updating loop indices.
Solution: Be careful with your loop conditions and index updates. Double-check that you’re covering all events without going out of bounds.
4. Forgetting to Update Max Platforms
Pitfall: Only tracking the current number of platforms without updating the maximum.
Solution: Always update max_platforms whenever platforms_needed increases.
5. Inefficient Time Representation
Pitfall: Using a less efficient time representation, like strings or datetime objects, which can slow down sorting and comparisons.
Solution: Use integer representation for times when possible, as we did in our example (e.g., 900 for 9:00 AM).
Advanced Techniques and Optimizations
While our current solution is efficient for most cases, there are some advanced techniques and optimizations we can consider for specific scenarios or larger datasets:
1. Using a Min-Heap
For very large datasets, we can use a min-heap to keep track of departure times. This approach can be more memory-efficient and potentially faster for certain input distributions.
2. Line Sweep Algorithm
The line sweep algorithm is a more general technique that can be applied to various interval problems. It involves sorting all events (both arrivals and departures) together and then sweeping through them.
3. Parallel Processing
For extremely large datasets, we could explore parallel processing techniques to sort and process the data more quickly.
4. Custom Sorting
If the time range is limited (e.g., all times are within a 24-hour period), we could use counting sort or radix sort for O(n) sorting time.
Practice Problems
To reinforce your understanding of the Minimum Number of Platforms problem and related concepts, here are some practice problems you can try:
- Meeting Rooms II (LeetCode 253): Given an array of meeting time intervals consisting of start and end times [[s1,e1],[s2,e2],…], find the minimum number of conference rooms required.
- Car Pooling (LeetCode 1094): You are driving a car that has capacity empty seats. The vehicle only drives east (ie. it cannot turn around and drive west.) Given a list of trips, trip[i] = [num_passengers, start_location, end_location] determine if it is possible to pick up and drop off all passengers for all the given trips.
- My Calendar I (LeetCode 729): Implement a MyCalendar class to store your events. A new event can be added if adding the event will not cause a double booking.
- Maximum CPU Load: We are given a list of jobs. Each job has a Start time, an End time, and a CPU load when it is running. Our goal is to find the maximum CPU load at any time if all the jobs are running on the same machine.
Conclusion
The Minimum Number of Platforms problem is a classic example of interval scheduling and demonstrates the power of efficient algorithm design. By sorting events and using a two-pointer approach, we’ve transformed a seemingly complex problem into an elegant and efficient solution.
This problem not only appears in coding interviews but also has numerous real-world applications in resource management and scheduling. Understanding this problem and its solution will enhance your problem-solving skills and prepare you for similar interval-based challenges.
Remember, the key to mastering algorithmic problems like this is practice. Try implementing the solution yourself, experiment with different inputs, and explore the variations we discussed. Happy coding, and may your algorithms always find the optimal solution!
Keep learning and growing with AlgoCademy. Our interactive coding tutorials and AI-powered assistance are here to help you on your journey from beginner to coding expert. Whether you’re preparing for technical interviews at top tech companies or simply want to improve your programming skills, we’ve got you covered. Stay tuned for more in-depth problem-solving tutorials and coding challenges!