Mind Maps: Visualizing Coding Concepts for Enhanced Learning
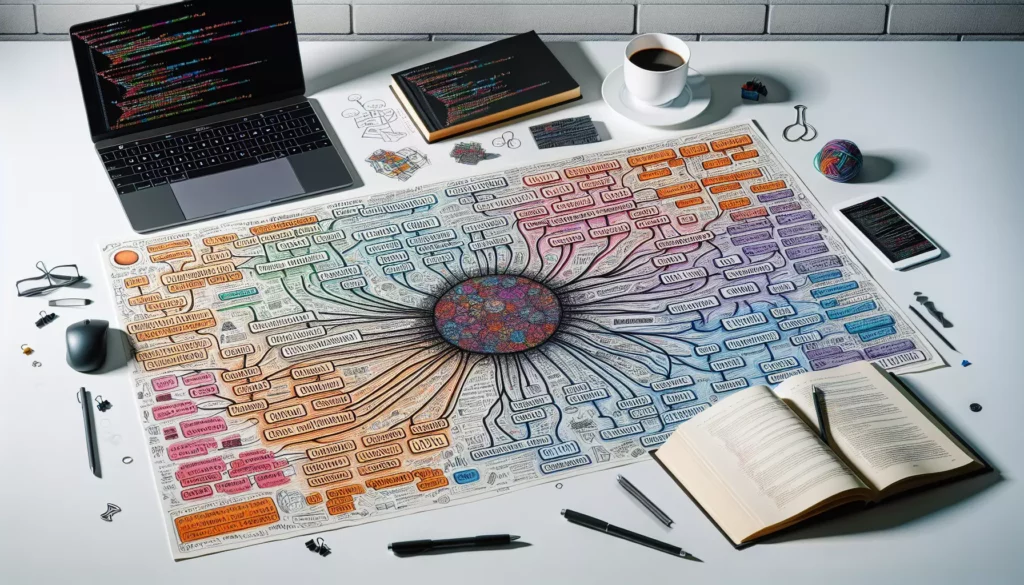
In the fast-paced world of coding education and programming skills development, finding effective ways to learn and retain complex information is crucial. One powerful technique that has gained popularity among developers and coding enthusiasts is the use of mind maps. These visual tools can revolutionize how we approach coding concepts, making them more accessible and easier to understand. In this comprehensive guide, we’ll explore how mind maps can be leveraged to visualize coding concepts, enhance learning, and boost your programming skills.
What Are Mind Maps?
Before diving into their application in coding, let’s first understand what mind maps are. A mind map is a diagram used to visually organize information. It typically starts with a central concept or idea, from which related ideas, words, and concepts branch out. This radial structure allows for a natural flow of thoughts and helps in creating connections between different elements.
Mind maps were popularized by British author Tony Buzan in the 1970s as a note-taking and brainstorming technique. Since then, they have been adopted across various fields, including education, business, and personal development. In the context of coding and programming, mind maps serve as an excellent tool for breaking down complex concepts, visualizing algorithms, and planning project structures.
The Benefits of Using Mind Maps in Coding Education
Incorporating mind maps into your coding education journey can offer numerous advantages:
- Visual Learning: Many people are visual learners, and mind maps cater to this learning style by presenting information in a graphical format.
- Improved Retention: The visual nature of mind maps can help in better retention of information compared to linear note-taking methods.
- Holistic Understanding: Mind maps allow you to see the big picture while also focusing on individual components, promoting a more comprehensive understanding of coding concepts.
- Creativity Boost: The free-flowing structure of mind maps encourages creative thinking and can lead to new insights and problem-solving approaches.
- Efficient Organization: Mind maps help in organizing complex information in a logical and easy-to-follow manner.
- Quick Review: Once created, mind maps serve as excellent review tools, allowing you to quickly refresh your memory on various coding topics.
How to Create Effective Mind Maps for Coding Concepts
Creating mind maps for coding concepts is a skill that can be developed with practice. Here are some steps to get you started:
- Choose a Central Topic: Begin with the main concept you want to explore. This could be a programming language, a specific algorithm, or a broader coding principle.
- Use Keywords: Instead of long sentences, use concise keywords or phrases to represent ideas. This makes the mind map easier to read and remember.
- Create Branches: From your central topic, create main branches for major subtopics. For example, if your central topic is “Python,” main branches could include “Data Types,” “Control Structures,” “Functions,” etc.
- Add Sub-branches: Expand on each main branch with relevant sub-topics. For instance, under “Data Types,” you might have sub-branches for “Strings,” “Lists,” “Dictionaries,” and so on.
- Use Colors and Images: Incorporate different colors for various branches and add relevant images or icons to make the mind map more visually appealing and memorable.
- Connect Related Concepts: Draw lines or arrows to connect related ideas across different branches. This helps in understanding relationships between various coding concepts.
- Keep It Clear and Uncluttered: While it’s tempting to include everything, try to maintain clarity by not overcrowding your mind map. You can always create separate, more detailed mind maps for complex subtopics.
Tools for Creating Coding Mind Maps
While you can create mind maps using pen and paper, there are numerous digital tools available that can enhance your mind mapping experience:
- XMind: A popular mind mapping software with a clean interface and support for various diagram types.
- MindMeister: A web-based tool that allows for real-time collaboration, making it great for team projects.
- Coggle: A simple and intuitive mind mapping tool with a free tier that’s sufficient for most users.
- FreeMind: An open-source mind mapping software that’s feature-rich and completely free.
- MindNode: A visually appealing mind mapping app for Apple devices, known for its ease of use.
These tools often come with templates and pre-designed elements that can be particularly useful for creating coding-related mind maps.
Applying Mind Maps to Different Aspects of Coding
Mind maps can be applied to various aspects of coding education and programming skills development. Let’s explore some specific applications:
1. Learning Programming Languages
When learning a new programming language, creating a mind map can help you visualize its structure and key components. For example, a mind map for Python might look like this:
- Central Topic: Python
- Syntax
- Indentation
- Comments
- Variables
- Data Types
- Numeric (int, float)
- String
- List
- Tuple
- Dictionary
- Control Structures
- If-else statements
- Loops (for, while)
- Functions
- Definition
- Parameters
- Return values
- Object-Oriented Programming
- Classes
- Objects
- Inheritance
- Syntax
This structure provides a clear overview of the language’s key elements, making it easier to understand how different components relate to each other.
2. Visualizing Algorithms
Mind maps can be particularly useful for understanding and remembering complex algorithms. For instance, a mind map for the QuickSort algorithm might include:
- Central Topic: QuickSort
- Concept
- Divide and Conquer
- Partitioning
- Steps
- Choose pivot
- Partition array
- Recursively sort subarrays
- Time Complexity
- Best Case: O(n log n)
- Average Case: O(n log n)
- Worst Case: O(n^2)
- Space Complexity
- O(log n) to O(n)
- Advantages
- Efficient for large datasets
- In-place sorting
- Disadvantages
- Unstable sort
- Poor performance on small arrays
- Concept
This visual representation helps in grasping the algorithm’s key components and characteristics at a glance.
3. Planning Project Structures
When starting a new coding project, a mind map can help in organizing your thoughts and planning the project structure. For example, a mind map for a web application might look like this:
- Central Topic: E-commerce Web App
- Frontend
- User Interface (React.js)
- Product Listing
- Shopping Cart
- Checkout Process
- State Management (Redux)
- Routing (React Router)
- User Interface (React.js)
- Backend
- API (Node.js/Express)
- User Authentication
- Product Management
- Order Processing
- Database (MongoDB)
- User Data
- Product Catalog
- Order History
- API (Node.js/Express)
- DevOps
- Version Control (Git)
- CI/CD (Jenkins)
- Hosting (AWS)
- Testing
- Unit Tests
- Integration Tests
- End-to-End Tests
- Frontend
This mind map provides a clear overview of the project’s components and their relationships, helping in planning and task allocation.
4. Preparing for Technical Interviews
Mind maps can be an excellent tool for organizing your knowledge and preparing for technical interviews, especially for positions at major tech companies. A mind map for interview preparation might include:
- Central Topic: Technical Interview Prep
- Data Structures
- Arrays
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Algorithms
- Sorting
- Searching
- Dynamic Programming
- Graph Algorithms
- System Design
- Scalability
- Load Balancing
- Caching
- Database Design
- Programming Languages
- Java
- Python
- JavaScript
- C++
- Behavioral Questions
- Past Projects
- Teamwork Examples
- Problem-solving Scenarios
- Data Structures
This comprehensive mind map can serve as a roadmap for your interview preparation, ensuring you cover all crucial areas.
Integrating Mind Maps with Coding Practice
While mind maps are excellent for visualizing concepts, they are most effective when integrated with hands-on coding practice. Here are some ways to combine mind mapping with practical coding exercises:
1. Code Snippets in Mind Maps
Include small code snippets directly in your mind maps to illustrate specific concepts. For example, in a Python mind map, you might include:
# Under the "List Comprehension" branch
squares = [x**2 for x in range(10)]
# Under the "Lambda Functions" branch
multiply = lambda x, y: x * y
These snippets provide concrete examples of the concepts, making them easier to remember and apply.
2. Problem-Solving Strategies
Create mind maps that outline problem-solving strategies for different types of coding challenges. For instance:
- Central Topic: Array Problem Solving
- Two-Pointer Technique
- Example: Finding pair with given sum
- Code snippet
- Sliding Window
- Example: Maximum sum subarray of size k
- Code snippet
- Divide and Conquer
- Example: Merge Sort
- Code snippet
- Two-Pointer Technique
This approach helps in recognizing patterns in problems and applying appropriate strategies.
3. Project Workflow Mind Maps
For larger coding projects, create mind maps that outline the development workflow. This can include:
- Central Topic: Project Workflow
- Planning Phase
- Requirements Gathering
- System Design
- Task Breakdown
- Development Phase
- Coding Standards
- Version Control Practices
- Code Review Process
- Testing Phase
- Unit Testing
- Integration Testing
- User Acceptance Testing
- Deployment
- CI/CD Pipeline
- Staging Environment
- Production Rollout
- Planning Phase
This type of mind map helps in understanding and managing the entire software development lifecycle.
Challenges and Solutions in Using Mind Maps for Coding
While mind maps are powerful tools for visualizing coding concepts, they come with their own set of challenges. Here are some common issues and solutions:
1. Overcomplexity
Challenge: As you delve deeper into a topic, mind maps can become overly complex and cluttered.
Solution: Use hierarchical mind maps or create separate, linked mind maps for subtopics. Many digital mind mapping tools allow for collapsible branches, which can help manage complexity.
2. Balancing Detail and Overview
Challenge: It can be difficult to decide how much detail to include without losing the big picture.
Solution: Start with a high-level overview and gradually add details. Use the concept of “progressive disclosure” where you reveal more information as you zoom in or expand branches.
3. Representing Code Structure
Challenge: Representing actual code structure in a mind map can be challenging due to the linear nature of code.
Solution: Focus on conceptual representation rather than trying to mimic code structure exactly. Use code snippets sparingly to illustrate key points.
4. Keeping Mind Maps Updated
Challenge: In the rapidly evolving field of programming, keeping mind maps up-to-date can be time-consuming.
Solution: Use digital mind mapping tools that allow for easy updates and version control. Regularly review and update your mind maps as part of your learning routine.
Enhancing Learning with AI-Powered Assistance
As we explore the use of mind maps in coding education, it’s worth noting how AI-powered assistance can further enhance this learning approach. Platforms like AlgoCademy are leveraging AI to provide personalized learning experiences and step-by-step guidance in coding education.
Here’s how AI can complement mind mapping techniques:
1. Personalized Mind Map Suggestions
AI algorithms can analyze your learning progress and suggest personalized mind map structures tailored to your current knowledge level and learning goals. This can help in creating more effective and relevant mind maps.
2. Interactive Mind Maps
AI can power interactive mind maps where clicking on a node provides additional information, code examples, or even launches a coding environment to practice the concept in real-time.
3. Adaptive Learning Paths
By combining mind maps with AI-driven adaptive learning, the system can guide you through different branches of your mind map based on your performance and areas that need more focus.
4. Automated Mind Map Generation
Advanced AI systems could potentially generate initial mind maps based on coding topics or even analyze your code to create custom mind maps reflecting your project structure or algorithmic approach.
Conclusion: Empowering Your Coding Journey with Visual Learning
Mind maps are powerful tools for visualizing coding concepts, enhancing understanding, and boosting retention of complex programming ideas. By incorporating mind mapping techniques into your coding education and combining them with hands-on practice and AI-powered assistance, you can create a robust learning strategy that caters to different learning styles and cognitive processes.
Whether you’re a beginner just starting your coding journey or an experienced developer preparing for technical interviews at top tech companies, mind maps can provide valuable support. They offer a flexible, creative approach to organizing knowledge, planning projects, and tackling complex algorithms.
As you continue to develop your programming skills, experiment with different mind mapping techniques and tools. Find the approach that works best for you, and don’t hesitate to adapt and evolve your mind maps as your understanding grows. Remember, the goal is not just to create beautiful diagrams, but to build a deep, interconnected understanding of coding concepts that will serve you well throughout your career in software development.
By mastering the art of mind mapping for coding, you’re not just learning to code – you’re learning how to learn more effectively, a skill that will prove invaluable in the ever-evolving world of technology.