Microsoft Technical Interview Prep: A Comprehensive Guide
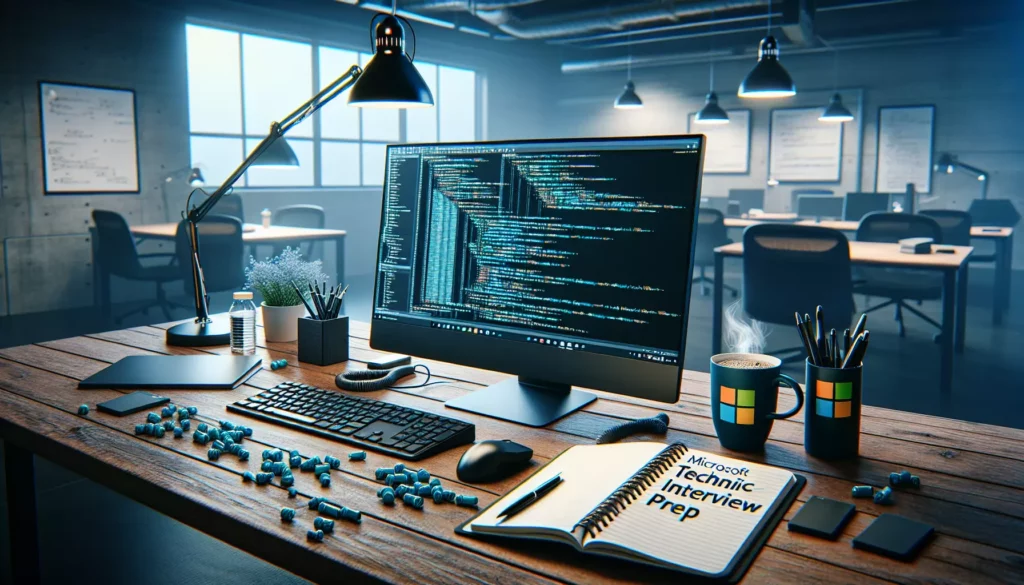
Preparing for a technical interview at Microsoft can be a daunting task, but with the right approach and resources, you can significantly increase your chances of success. In this comprehensive guide, we’ll walk you through the essential steps and strategies to help you ace your Microsoft technical interview. From understanding the interview process to mastering key coding concepts, we’ve got you covered.
Table of Contents
- Understanding the Microsoft Interview Process
- Key Areas to Focus On
- Sharpening Your Coding Skills
- Enhancing Your Problem-Solving Abilities
- Mastering Data Structures and Algorithms
- Tackling System Design Questions
- Preparing for Behavioral Questions
- Conducting Mock Interviews
- Recommended Resources and Tools
- What to Expect on the Day of the Interview
- Post-Interview Follow-up
1. Understanding the Microsoft Interview Process
Before diving into the preparation, it’s crucial to understand what the Microsoft interview process typically entails. While the exact process may vary depending on the role and team, here’s a general overview:
- Initial Screening: This usually involves a phone or video call with a recruiter to discuss your background and the role.
- Technical Phone Screen: A technical interview conducted over the phone or video call, often involving coding questions.
- On-site Interviews: If you pass the initial stages, you’ll be invited for a series of on-site interviews (or virtual equivalents due to COVID-19). This typically includes:
- 4-5 technical interviews
- 1-2 behavioral interviews
- Possibly a system design interview for more senior roles
- Final Decision: After the interviews, the hiring committee will review your performance and make a decision.
Understanding this process will help you tailor your preparation and manage your expectations throughout the journey.
2. Key Areas to Focus On
When preparing for a Microsoft technical interview, it’s essential to focus on the following key areas:
- Data Structures and Algorithms
- Problem-solving skills
- Coding proficiency (particularly in C++, C#, or Java)
- System design (for more senior positions)
- Microsoft-specific technologies and platforms (e.g., Azure, .NET)
- Behavioral competencies (e.g., teamwork, leadership, communication)
Let’s dive deeper into each of these areas in the following sections.
3. Sharpening Your Coding Skills
Proficiency in coding is a fundamental requirement for any technical role at Microsoft. Here are some tips to enhance your coding skills:
- Choose a primary language: While Microsoft uses various languages, C++, C#, and Java are commonly used in interviews. Pick one and become proficient in it.
- Practice regularly: Aim to solve at least one coding problem every day. Platforms like LeetCode, HackerRank, and CodeSignal offer a wide range of problems to practice.
- Understand time and space complexity: Be prepared to analyze and optimize your solutions for both time and space efficiency.
- Learn to write clean, readable code: Microsoft values code quality, so practice writing well-structured and commented code.
Here’s a simple example of a clean, well-commented function in C# that demonstrates good coding practices:
/// <summary>
/// Finds the maximum element in an array of integers.
/// </summary>
/// <param name="arr">The input array of integers</param>
/// <returns>The maximum element in the array</returns>
/// <exception cref="ArgumentException">Thrown when the input array is empty</exception>
public static int FindMaxElement(int[] arr)
{
// Check if the array is null or empty
if (arr == null || arr.Length == 0)
{
throw new ArgumentException("The input array cannot be null or empty.");
}
int max = arr[0]; // Initialize max with the first element
// Iterate through the array to find the maximum element
for (int i = 1; i < arr.Length; i++)
{
if (arr[i] > max)
{
max = arr[i];
}
}
return max;
}
4. Enhancing Your Problem-Solving Abilities
Problem-solving is at the core of what Microsoft engineers do. Here are some strategies to improve your problem-solving skills:
- Understand the problem: Before jumping into coding, make sure you fully understand the problem. Ask clarifying questions if needed.
- Break it down: Divide complex problems into smaller, manageable sub-problems.
- Think out loud: Practice verbalizing your thought process. This is crucial during interviews as it helps the interviewer understand your approach.
- Consider edge cases: Always think about potential edge cases and how your solution handles them.
- Optimize: Once you have a working solution, think about how you can optimize it for better time or space complexity.
Here’s an example of how you might approach a problem-solving question in a Microsoft interview:
Problem: Given a string, find the length of the longest substring without repeating characters.
Approach:
- Understand the problem: We need to find the longest contiguous sequence of unique characters in the string.
- Consider a simple approach: We could use a sliding window technique with two pointers.
- Think about optimization: We can use a HashSet to keep track of characters in the current window for O(1) lookup time.
- Implement the solution:
public class Solution {
public int LengthOfLongestSubstring(string s) {
int n = s.Length;
int maxLength = 0;
HashSet<char> charSet = new HashSet<char>();
int left = 0, right = 0;
while (right < n) {
if (!charSet.Contains(s[right])) {
charSet.Add(s[right]);
maxLength = Math.Max(maxLength, right - left + 1);
right++;
} else {
charSet.Remove(s[left]);
left++;
}
}
return maxLength;
}
}
- Explain the solution: Walk through how the sliding window moves and how the HashSet is used to efficiently check for duplicates.
- Analyze time and space complexity: This solution has a time complexity of O(n) and space complexity of O(min(m, n)), where n is the length of the string and m is the size of the character set.
- Consider edge cases: Discuss how the solution handles empty strings, strings with all unique characters, and strings with all repeated characters.
5. Mastering Data Structures and Algorithms
A solid understanding of data structures and algorithms is crucial for success in Microsoft technical interviews. Here are some key areas to focus on:
Data Structures:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees (Binary Trees, Binary Search Trees, Balanced Trees)
- Graphs
- Hash Tables
- Heaps
Algorithms:
- Sorting (Quick Sort, Merge Sort, Heap Sort)
- Searching (Binary Search, Depth-First Search, Breadth-First Search)
- Dynamic Programming
- Greedy Algorithms
- Recursion and Backtracking
- Bit Manipulation
Let’s look at an example of how you might implement a binary search tree (BST) in C#:
public class TreeNode {
public int val;
public TreeNode left;
public TreeNode right;
public TreeNode(int val=0, TreeNode left=null, TreeNode right=null) {
this.val = val;
this.left = left;
this.right = right;
}
}
public class BinarySearchTree {
private TreeNode root;
public void Insert(int value) {
root = InsertRec(root, value);
}
private TreeNode InsertRec(TreeNode root, int value) {
if (root == null) {
root = new TreeNode(value);
return root;
}
if (value < root.val)
root.left = InsertRec(root.left, value);
else if (value > root.val)
root.right = InsertRec(root.right, value);
return root;
}
public bool Search(int value) {
return SearchRec(root, value);
}
private bool SearchRec(TreeNode root, int value) {
if (root == null || root.val == value)
return root != null;
if (value < root.val)
return SearchRec(root.left, value);
return SearchRec(root.right, value);
}
}
Understanding how to implement and use data structures like this BST is crucial for many coding interview questions.
6. Tackling System Design Questions
For more senior positions or certain teams at Microsoft, you may encounter system design questions. These assess your ability to design large-scale distributed systems. Here are some tips for approaching system design questions:
- Clarify requirements: Understand the scale, features, and constraints of the system you’re designing.
- Start with a high-level design: Sketch out the main components of the system.
- Dive into details: Discuss specific technologies, data models, and algorithms you would use.
- Consider scalability: Explain how your design would handle increasing load.
- Discuss trade-offs: Be prepared to explain the pros and cons of your design choices.
Here’s a simplified example of how you might approach a system design question:
Question: Design a URL shortening service like bit.ly.
Approach:
- Clarify requirements:
- Shortening: Convert a long URL to a short URL
- Redirection: Redirect short URL to original URL
- Custom URLs: Allow users to choose custom short URLs
- Analytics: Track click rates (optional)
- Scale: Assume 100 million URLs are shortened per day
- High-level design:
- API Gateway: Handle incoming requests
- Application Servers: Process URL shortening and redirection
- Database: Store mappings between short and long URLs
- Cache: Store frequently accessed URLs for faster retrieval
- Detailed design:
- URL shortening algorithm: Use base62 encoding of an auto-incrementing ID
- Database schema: (id: bigint, short_url: varchar, long_url: text, user_id: int, created_at: timestamp)
- Caching strategy: Use Redis to cache frequently accessed URLs
- Scalability considerations:
- Database sharding: Shard based on the first character of the short URL
- Load balancing: Use a round-robin load balancer for even distribution of requests
- CDN: Use a CDN for faster redirection for users across different geographic locations
Remember, the key in system design questions is to demonstrate your thought process and ability to make and justify design decisions.
7. Preparing for Behavioral Questions
Microsoft places a high value on soft skills and cultural fit. Be prepared to answer behavioral questions that assess your teamwork, leadership, and problem-solving abilities. Use the STAR method (Situation, Task, Action, Result) to structure your responses.
Some common behavioral questions you might encounter include:
- Tell me about a time when you had to work with a difficult team member.
- Describe a situation where you had to meet a tight deadline.
- How do you handle disagreements with coworkers?
- Give an example of a time you showed initiative.
Here’s an example of how you might answer a behavioral question using the STAR method:
Question: Tell me about a time when you had to deal with a project that was behind schedule.
Answer:
- Situation: In my previous role, we were working on a critical software update for our main product. Two weeks before the deadline, we realized we were significantly behind schedule due to unexpected technical challenges.
- Task: As the lead developer, it was my responsibility to ensure we delivered the update on time without compromising on quality.
- Action: I took the following steps:
- Conducted a team meeting to reassess our priorities and identify tasks that could be postponed to a future update.
- Reorganized the team into smaller, focused sub-teams to tackle the most critical features in parallel.
- Implemented daily stand-up meetings to track progress and quickly address any blockers.
- Worked extra hours myself to support the team and maintain morale.
- Result: Through these efforts, we managed to deliver the core functionality of the update on schedule. The client was satisfied with the result, and we incorporated the postponed features into the next update, which we delivered ahead of schedule.
8. Conducting Mock Interviews
Practice makes perfect, and this is especially true for technical interviews. Conducting mock interviews can help you:
- Get comfortable with the interview format
- Improve your ability to think out loud while solving problems
- Identify areas where you need more practice
- Reduce anxiety and build confidence
Here are some ways to conduct mock interviews:
- Use online platforms: Websites like Pramp or InterviewBit allow you to practice with real people.
- Practice with peers: If you have friends in the tech industry, ask them to conduct mock interviews with you.
- Record yourself: Practice solving problems while explaining your thought process out loud, and record yourself. Review the recording to identify areas for improvement.
- Hire a coach: Consider hiring a professional interview coach who can provide personalized feedback.
9. Recommended Resources and Tools
To help you prepare for your Microsoft technical interview, here are some valuable resources:
- Books:
- “Cracking the Coding Interview” by Gayle Laakmann McDowell
- “System Design Interview” by Alex Xu
- “Clean Code” by Robert C. Martin
- Online Platforms:
- LeetCode: Offers a wide range of coding problems, including a section specifically for Microsoft interview questions.
- HackerRank: Provides coding challenges and has a specific interview preparation kit.
- AlgoExpert: Offers curated coding interview questions with video explanations.
- Courses:
- Coursera: “Data Structures and Algorithms” specialization by UC San Diego
- Udacity: “Data Structures and Algorithms in Python”
- Microsoft-specific resources:
- Microsoft Learn: Free, official learning paths and modules for Microsoft technologies
- Microsoft Developer Network (MSDN): Documentation and resources for Microsoft developers
10. What to Expect on the Day of the Interview
On the day of your Microsoft technical interview, here’s what you can typically expect:
- Arrival: If it’s an on-site interview, arrive at least 15 minutes early. For virtual interviews, test your setup well in advance.
- Introduction: Your interviewer will introduce themselves and may give you a brief overview of the interview structure.
- Technical questions: You’ll be asked to solve coding problems, often using a whiteboard or a shared coding environment.
- System design (for senior roles): You might be asked to design a large-scale system on a whiteboard.
- Behavioral questions: Expect questions about your past experiences and how you handle various situations.
- Your questions: At the end, you’ll have the opportunity to ask questions about the role and the company.
Remember these tips during the interview:
- Think out loud: Explain your thought process as you work through problems.
- Ask clarifying questions: Make sure you understand the problem before starting to solve it.
- Manage your time: If you’re stuck, consider asking for a hint or moving on to the next part of the problem.
- Stay calm: It’s normal to feel nervous, but try to stay focused and composed.
11. Post-Interview Follow-up
After your Microsoft technical interview:
- Send a thank-you email: Within 24 hours, send a brief email thanking your interviewer for their time. Reiterate your interest in the position.
- Reflect on the experience: Make notes about the questions you were asked and how you performed. This can help you improve for future interviews.
- Be patient: The hiring process at large companies like Microsoft can take time. Don’t be discouraged if you don’t hear back immediately.
- Follow up appropriately: If you haven’t heard back after a week or two, it’s okay to send a polite follow-up email to your recruiter asking about the status of your application.
Conclusion
Preparing for a Microsoft technical interview requires dedication and consistent effort. By focusing on strengthening your coding skills, mastering data structures and algorithms, practicing problem-solving, and preparing for behavioral questions, you’ll be well-equipped to tackle the interview process.
Remember, the goal of the interview is not just to test your technical skills, but also to assess how you approach problems, how you communicate your thoughts, and how you would fit into the Microsoft team culture. Stay confident, be yourself, and let your passion for technology shine through.
Good luck with your Microsoft technical interview preparation!