Meta Technical Interview Prep: Your Ultimate Guide to Success
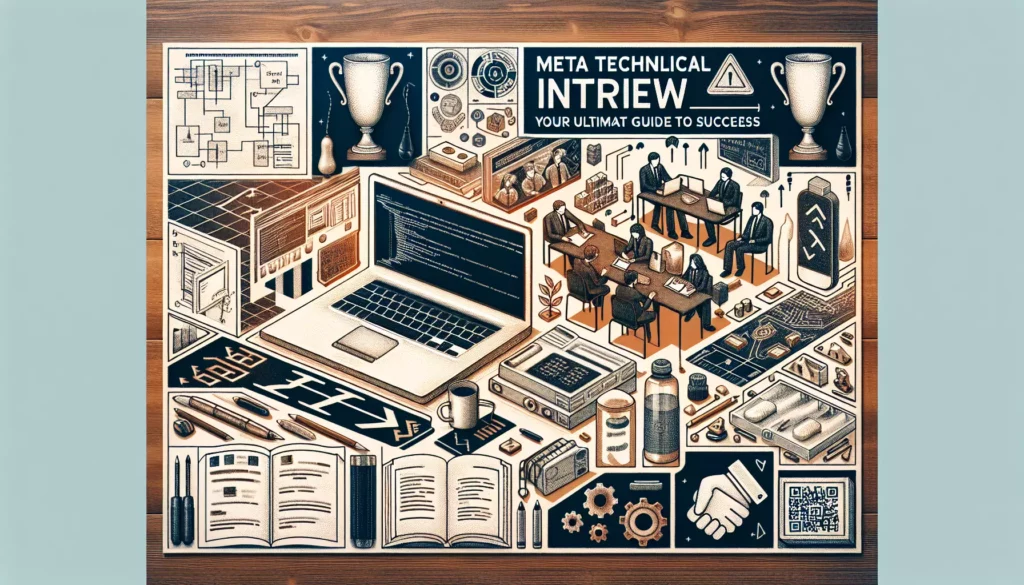
Preparing for a technical interview at Meta (formerly Facebook) can be an exciting yet daunting experience. As one of the world’s leading tech companies, Meta is known for its rigorous interview process that tests candidates on various aspects of computer science and programming. In this comprehensive guide, we’ll walk you through everything you need to know to ace your Meta technical interview, from understanding the interview structure to mastering key concepts and practicing effectively.
Understanding the Meta Technical Interview Process
Before diving into the preparation strategies, it’s crucial to understand the structure of Meta’s technical interview process. Typically, it consists of several stages:
- Initial Phone Screen: A brief conversation with a recruiter to assess your background and interest.
- Technical Phone Interview: A 45-60 minute coding interview conducted remotely.
- On-site Interviews: Usually 4-5 interviews, including coding, system design, and behavioral questions.
For this guide, we’ll focus primarily on preparing for the coding and system design aspects of the interview process.
Key Areas to Focus On
Meta’s technical interviews cover a wide range of topics, but some key areas consistently appear:
1. Data Structures and Algorithms
A solid understanding of fundamental data structures and algorithms is crucial. Focus on:
- Arrays and Strings
- Linked Lists
- Trees and Graphs
- Stacks and Queues
- Hash Tables
- Heaps
- Dynamic Programming
- Sorting and Searching Algorithms
2. Problem-Solving Skills
Meta values candidates who can approach problems systematically. Practice:
- Breaking down complex problems into smaller, manageable parts
- Analyzing time and space complexity
- Optimizing solutions
- Communicating your thought process clearly
3. System Design
For more experienced roles, system design questions are common. Familiarize yourself with:
- Scalability concepts
- Database design
- Caching mechanisms
- Load balancing
- Microservices architecture
4. Coding Proficiency
You should be comfortable coding in at least one programming language. Popular choices include:
- Python
- Java
- C++
- JavaScript
Preparing for the Coding Interview
The coding portion of the interview is where you’ll demonstrate your problem-solving skills and coding proficiency. Here’s how to prepare effectively:
1. Practice Coding Problems
Regularly solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal. Focus on Meta-specific problem sets if available. Aim to solve at least 2-3 problems daily, gradually increasing difficulty.
2. Mock Interviews
Conduct mock interviews with friends, mentors, or through online platforms. This helps simulate the pressure of a real interview and improves your ability to communicate your thought process.
3. Time Management
Practice solving problems within time constraints. In a real interview, you’ll typically have 30-45 minutes per coding question.
4. Review and Optimize
After solving a problem, always review your solution. Look for ways to optimize it in terms of time and space complexity.
5. Learn Common Patterns
Recognize and learn common problem-solving patterns. For example:
- Two-pointer technique
- Sliding window
- Depth-First Search (DFS) and Breadth-First Search (BFS)
- Binary search
- Backtracking
Sample Coding Problem: Two Sum
Let’s walk through a common coding problem you might encounter in a Meta interview:
Problem: Given an array of integers nums
and an integer target
, return indices of the two numbers such that they add up to target
.
Example:
Input: nums = [2,7,11,15], target = 9
Output: [0,1]
Explanation: Because nums[0] + nums[1] == 9, we return [0, 1].
Solution:
def twoSum(nums, target):
num_map = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_map:
return [num_map[complement], i]
num_map[num] = i
return [] # No solution found
Explanation:
- We use a hash map to store each number and its index as we iterate through the array.
- For each number, we calculate its complement (target – number).
- If the complement exists in our hash map, we’ve found our pair and return their indices.
- If not, we add the current number and its index to the hash map and continue.
This solution has a time complexity of O(n) and space complexity of O(n), which is optimal for this problem.
Preparing for the System Design Interview
System design questions assess your ability to design large-scale systems. Here’s how to prepare:
1. Study Fundamental Concepts
Familiarize yourself with key concepts such as:
- Load balancing
- Caching
- Sharding
- Replication
- CAP theorem
- Consistency models
2. Analyze Existing Systems
Study the architecture of popular systems and services. Understand how they handle scale, reliability, and performance.
3. Practice Designing Systems
Attempt to design systems like:
- A URL shortener
- A social media feed
- A distributed cache
- A ride-sharing application
4. Follow a Structured Approach
When answering system design questions, follow these steps:
- Clarify requirements and constraints
- Estimate scale (users, data volume, etc.)
- Define API endpoints
- Design high-level architecture
- Dive into component details
- Discuss trade-offs and potential improvements
Sample System Design Question: Design a News Feed System
Let’s walk through a simplified version of designing a news feed system similar to Facebook’s:
1. Requirements Clarification
- Users can create posts (text, images, videos)
- Users can follow other users
- The feed should show recent posts from followed users
- The system should handle millions of users and posts
2. Scale Estimation
- Assume 100 million daily active users
- Each user views their feed 10 times a day
- Each user creates 2 posts per day
3. High-Level Design
+---------+ +-------------+ +-----------+
Users -> | Web/App | -> | Application | -> | Database |
| Servers | | Servers | | |
+---------+ +-------------+ +-----------+
|
+------------+
| Cache (Redis) |
+------------+
4. Component Details
- Post Storage: Use a NoSQL database like Cassandra for scalability.
- User Graph: Store user relationships in a graph database like Neo4j.
- Feed Generation: Use a fan-out approach, pre-computing feeds for users.
- Caching: Implement Redis to cache recent feed items and reduce database load.
- Load Balancing: Use consistent hashing to distribute load across servers.
5. Optimizations and Trade-offs
- Implement a hybrid approach of push and pull for feed updates.
- Use content delivery networks (CDNs) for faster media delivery.
- Implement rate limiting to prevent system abuse.
- Consider eventual consistency for better performance at scale.
This is a simplified design, but it covers the main components and considerations for a news feed system.
Behavioral Interview Preparation
While technical skills are crucial, Meta also values cultural fit and soft skills. Prepare for behavioral questions by:
1. Reflecting on Past Experiences
Prepare stories that demonstrate:
- Leadership and teamwork
- Problem-solving in challenging situations
- Innovation and creativity
- Handling conflicts or failures
2. Understanding Meta’s Culture and Values
Familiarize yourself with Meta’s core values:
- Move Fast
- Be Bold
- Focus on Impact
- Be Open
- Build Social Value
3. Preparing Questions for Interviewers
Have thoughtful questions ready about Meta’s products, culture, and your potential role.
Final Tips for Success
- Stay Calm: Remember to breathe and stay composed during the interview.
- Communicate Clearly: Explain your thought process as you work through problems.
- Ask for Clarification: Don’t hesitate to ask questions if you need more information.
- Practice Whiteboarding: Get comfortable explaining your solutions visually.
- Be Yourself: Show your passion for technology and problem-solving.
Conclusion
Preparing for a Meta technical interview requires dedication, practice, and a well-rounded approach. By focusing on strong fundamentals in data structures and algorithms, honing your problem-solving skills, and understanding system design principles, you’ll be well-equipped to tackle the challenges of the interview process.
Remember, the goal is not just to memorize solutions but to develop a deep understanding of computer science concepts and their practical applications. With consistent practice and the right mindset, you can approach your Meta interview with confidence and showcase your skills effectively.
Good luck with your preparation, and may your journey to joining Meta be successful!