Message Brokers: Powering Modern Distributed Systems
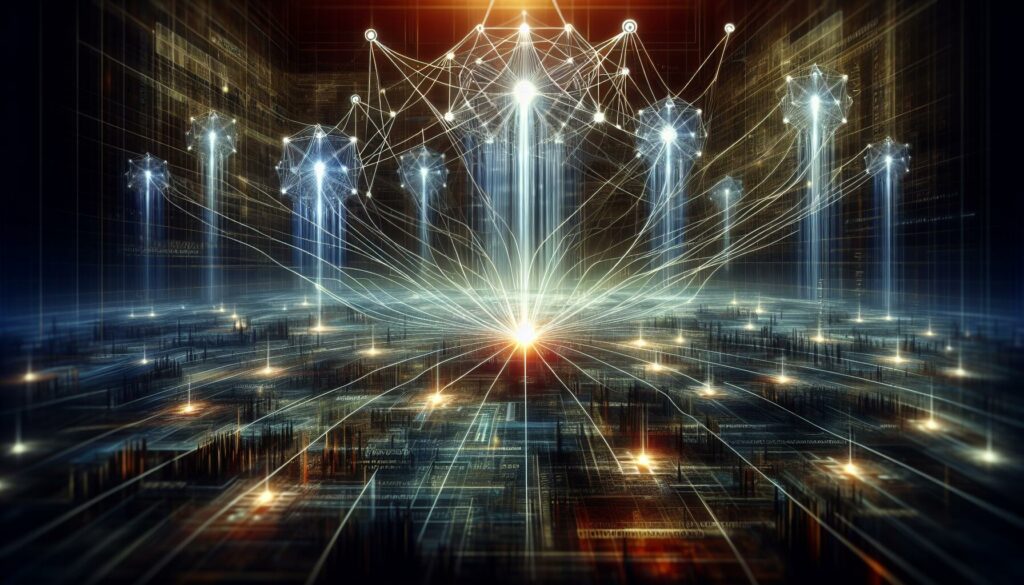
In the ever-evolving landscape of software development, distributed systems have become increasingly prevalent. As applications grow in complexity and scale, the need for efficient communication between different components of a system becomes crucial. This is where message brokers come into play, serving as the backbone of many modern distributed architectures. In this comprehensive guide, we’ll dive deep into the world of message brokers, exploring their role, benefits, and implementation in today’s software ecosystem.
Table of Contents
- What Are Message Brokers?
- How Message Brokers Work
- Benefits of Using Message Brokers
- Common Message Broker Patterns
- Popular Message Broker Solutions
- Implementing a Message Broker
- Best Practices and Considerations
- Message Brokers in Microservices Architecture
- The Future of Message Brokers
- Conclusion
1. What Are Message Brokers?
Message brokers are intermediary software components that facilitate communication between different parts of a distributed system. They act as a central hub for messages, enabling various applications, services, or systems to exchange information without direct connections. This decoupling of communication allows for greater flexibility, scalability, and reliability in complex software architectures.
At its core, a message broker receives messages from publishers (or producers) and routes them to the appropriate subscribers (or consumers). This publish-subscribe model forms the foundation of many event-driven architectures and is crucial for building responsive, real-time systems.
2. How Message Brokers Work
To understand how message brokers function, let’s break down their operation into key components:
2.1. Message Production
The process begins when a producer creates a message. This message typically contains data or an event notification that needs to be communicated to other parts of the system. The producer sends this message to the message broker.
2.2. Message Queuing
Upon receiving a message, the broker stores it in a queue. Queues are ordered lists of messages that follow a first-in-first-out (FIFO) principle. Messages remain in the queue until they are consumed or expire.
2.3. Message Routing
The broker is responsible for determining where each message should be sent. This routing can be based on various criteria, such as topic subscriptions, message content, or predefined rules.
2.4. Message Consumption
Consumers subscribe to specific queues or topics. When a message is available and matches a consumer’s subscription, the broker delivers the message to that consumer.
2.5. Acknowledgment and Persistence
After successful consumption, the consumer sends an acknowledgment to the broker. This ensures that messages are not lost and can be redelivered if necessary. Many brokers also offer persistence mechanisms to store messages on disk, providing durability in case of system failures.
3. Benefits of Using Message Brokers
Incorporating message brokers into your system architecture offers numerous advantages:
3.1. Decoupling
Message brokers allow different parts of a system to communicate without direct dependencies. This decoupling makes it easier to modify, scale, or replace individual components without affecting the entire system.
3.2. Scalability
By acting as an intermediary, message brokers can handle varying loads and distribute messages across multiple consumers. This enables systems to scale horizontally by adding more consumers as needed.
3.3. Reliability
Message persistence and guaranteed delivery mechanisms ensure that messages are not lost, even in the event of system failures or network issues.
3.4. Asynchronous Communication
Message brokers enable asynchronous communication, allowing producers and consumers to operate independently and at their own pace. This is particularly useful for handling time-consuming tasks or dealing with slow consumers.
3.5. Load Balancing
Brokers can distribute messages across multiple consumers, effectively balancing the workload and preventing any single component from becoming a bottleneck.
3.6. Fault Tolerance
With features like message persistence and redelivery, message brokers can help systems recover from failures and ensure that no data is lost during outages.
4. Common Message Broker Patterns
Message brokers support various communication patterns to suit different use cases:
4.1. Publish-Subscribe (Pub/Sub)
In this pattern, messages are published to topics, and all subscribers to those topics receive the messages. This is useful for broadcasting events or updates to multiple interested parties.
4.2. Point-to-Point
Messages are sent to a specific queue and consumed by a single consumer. This pattern ensures that each message is processed only once, which is ideal for task distribution.
4.3. Request-Response
A two-way communication pattern where a client sends a request message and expects a response. This can be implemented using temporary response queues or correlation IDs.
4.4. Competing Consumers
Multiple consumers subscribe to the same queue, competing to process messages. This pattern is excellent for load balancing and parallel processing.
5. Popular Message Broker Solutions
Several message broker implementations are widely used in the industry. Let’s explore some of the most popular options:
5.1. Apache Kafka
Kafka is a distributed streaming platform that combines messaging, storage, and stream processing. It’s known for its high throughput and scalability, making it popular for big data and real-time analytics use cases.
5.2. RabbitMQ
RabbitMQ is a lightweight, easy-to-deploy message broker that supports multiple messaging protocols. It’s versatile and well-suited for a wide range of applications, from simple queuing to complex routing scenarios.
5.3. Apache ActiveMQ
ActiveMQ is a flexible, multi-protocol message broker that supports enterprise integration patterns. It offers features like clustering, security, and persistence, making it suitable for enterprise environments.
5.4. Redis
While primarily known as an in-memory data store, Redis also provides pub/sub functionality, making it a lightweight option for simple messaging needs.
5.5. Amazon SQS and SNS
Amazon Simple Queue Service (SQS) and Simple Notification Service (SNS) are managed message queue and pub/sub services, respectively. They integrate well with other AWS services and provide a scalable, cloud-native messaging solution.
6. Implementing a Message Broker
To illustrate how to work with a message broker, let’s implement a simple producer and consumer using RabbitMQ and Python. This example will demonstrate the basics of publishing and consuming messages.
6.1. Setting Up RabbitMQ
First, ensure that RabbitMQ is installed and running on your system. You can install it using package managers or Docker.
6.2. Installing Dependencies
Install the pika library, which is a Python client for RabbitMQ:
pip install pika
6.3. Implementing the Producer
Here’s a simple producer that sends messages to a queue:
import pika
# Establish a connection to RabbitMQ
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
# Declare a queue
channel.queue_declare(queue='hello')
# Publish a message
message = "Hello, World!"
channel.basic_publish(exchange='',
routing_key='hello',
body=message)
print(f" [x] Sent '{message}'")
# Close the connection
connection.close()
6.4. Implementing the Consumer
Now, let’s create a consumer that receives messages from the queue:
import pika
# Establish a connection to RabbitMQ
connection = pika.BlockingConnection(pika.ConnectionParameters('localhost'))
channel = connection.channel()
# Declare the same queue as the producer
channel.queue_declare(queue='hello')
# Callback function to process received messages
def callback(ch, method, properties, body):
print(f" [x] Received {body}")
# Set up the consumer
channel.basic_consume(queue='hello',
auto_ack=True,
on_message_callback=callback)
print(' [*] Waiting for messages. To exit press CTRL+C')
channel.start_consuming()
This simple example demonstrates the basic concepts of publishing and consuming messages using a message broker. In a real-world scenario, you’d likely have multiple producers and consumers, possibly using different queues or topics, and implementing more complex routing and processing logic.
7. Best Practices and Considerations
When working with message brokers, keep these best practices in mind:
7.1. Message Durability
Configure your queues and messages for durability to prevent data loss in case of broker restarts or crashes.
7.2. Idempotent Consumers
Design your consumers to handle duplicate messages gracefully, as message brokers may sometimes deliver messages more than once.
7.3. Error Handling
Implement robust error handling and dead-letter queues to manage messages that cannot be processed.
7.4. Monitoring and Alerting
Set up comprehensive monitoring for your message broker to track queue sizes, message rates, and consumer health.
7.5. Security
Implement authentication, authorization, and encryption to secure your message broker and the data it handles.
7.6. Performance Tuning
Optimize your broker configuration, message sizes, and consumer processing to achieve the best performance for your specific use case.
8. Message Brokers in Microservices Architecture
Message brokers play a crucial role in microservices architectures, facilitating communication between independent services. They enable:
8.1. Event-Driven Architecture
Services can publish events when their state changes, allowing other services to react accordingly.
8.2. Service Decoupling
Microservices can communicate without direct dependencies, making it easier to evolve and scale individual services.
8.3. Data Consistency
Message brokers can help implement eventual consistency patterns across distributed data stores.
8.4. Asynchronous Processing
Long-running tasks can be offloaded to background workers, improving the responsiveness of user-facing services.
9. The Future of Message Brokers
As distributed systems continue to evolve, message brokers are adapting to meet new challenges and requirements:
9.1. Cloud-Native Solutions
Managed message broker services and cloud-native implementations are becoming increasingly popular, offering easier scaling and management.
9.2. Serverless Integration
Message brokers are being integrated with serverless platforms, enabling event-driven, serverless architectures.
9.3. Edge Computing
As edge computing grows, message brokers are being adapted to work efficiently in distributed edge environments.
9.4. AI and Machine Learning
Message brokers are being used to stream data for real-time AI and ML applications, enabling more intelligent and responsive systems.
10. Conclusion
Message brokers are a fundamental component of modern distributed systems, enabling scalable, reliable, and decoupled communication between different parts of an application. By facilitating asynchronous messaging, load balancing, and fault tolerance, they play a crucial role in building robust and responsive software architectures.
As you continue your journey in software development and system design, understanding message brokers will be invaluable. They are not just a tool for large-scale distributed systems but can also simplify and improve the architecture of smaller applications. By mastering message brokers, you’ll be better equipped to design and implement efficient, scalable, and maintainable software solutions.
Remember that choosing the right message broker and implementing it correctly requires careful consideration of your specific use case, scalability requirements, and overall system architecture. As with any technology, it’s essential to weigh the benefits against the added complexity and ensure that a message broker is the right solution for your needs.
Whether you’re building a microservices architecture, implementing an event-driven system, or simply looking to decouple components in your application, message brokers offer a powerful set of tools to achieve your goals. As you apply these concepts in your projects, you’ll gain practical experience that will deepen your understanding and help you leverage the full potential of message brokers in your software designs.