Mesh Networks and P2P Programming: Revolutionizing Decentralized Networking
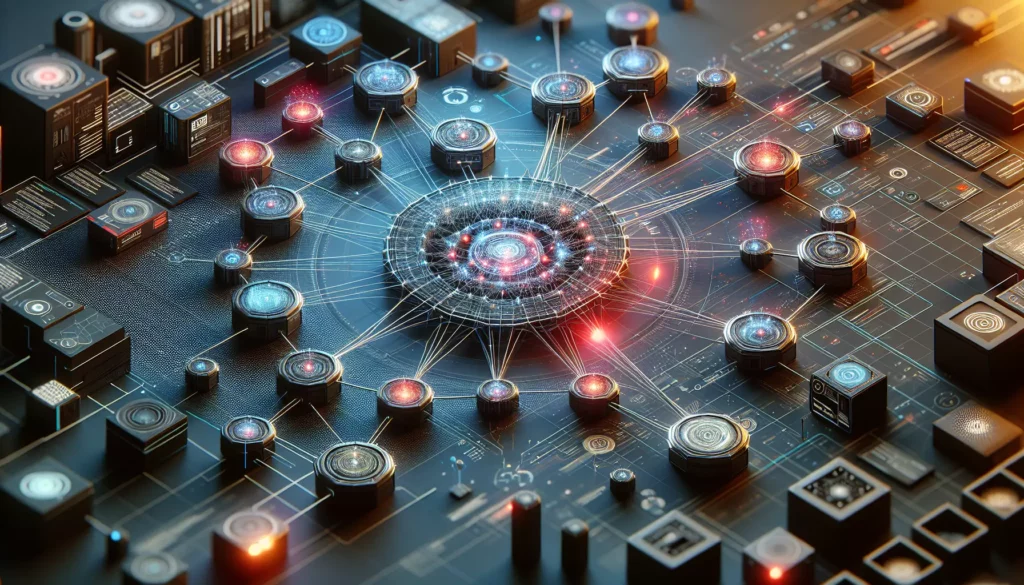
In the ever-evolving landscape of technology, decentralized networking has emerged as a powerful paradigm shift, challenging traditional centralized models. At the forefront of this revolution are mesh networks and peer-to-peer (P2P) programming. These technologies are reshaping how we think about connectivity, data sharing, and application development. In this comprehensive guide, we’ll delve deep into the world of mesh networks and P2P programming, exploring their principles, applications, and the impact they’re having on the digital ecosystem.
Understanding Mesh Networks
Mesh networks represent a radical departure from conventional network topologies. Unlike traditional networks that rely on a centralized infrastructure, mesh networks distribute the responsibility of data transmission across multiple nodes, creating a resilient and scalable network architecture.
Key Characteristics of Mesh Networks
- Decentralization: No single point of failure or control
- Self-healing: Ability to route around failed nodes
- Scalability: Easy to expand by adding new nodes
- Redundancy: Multiple paths for data transmission
- Flexibility: Adaptable to various environments and use cases
How Mesh Networks Work
In a mesh network, each device (or node) acts as both a user of the network and a relay for other nodes. This distributed approach allows data to hop from node to node until it reaches its destination. The network dynamically determines the best path for data transmission, considering factors like distance, signal strength, and node availability.
function meshRoutingAlgorithm(source, destination, nodes) {
let path = [];
let currentNode = source;
while (currentNode !== destination) {
let nextNode = findBestNextHop(currentNode, destination, nodes);
path.push(nextNode);
currentNode = nextNode;
}
return path;
}
function findBestNextHop(current, destination, nodes) {
// Implementation of routing logic
// Consider factors like signal strength, hop count, etc.
}
This simplified pseudocode demonstrates the basic concept of routing in a mesh network. The actual implementation would be much more complex, taking into account various network conditions and optimizations.
Applications of Mesh Networks
Mesh networks find applications in various domains, including:
- Disaster Recovery: Providing communication in areas where traditional infrastructure is damaged or unavailable
- Smart Cities: Connecting IoT devices for efficient urban management
- Military Operations: Ensuring robust communication in challenging environments
- Community Networks: Enabling internet access in underserved areas
- Industrial IoT: Connecting sensors and devices in large industrial settings
Diving into P2P Programming
Peer-to-peer (P2P) programming is a paradigm that complements mesh networks by enabling direct communication between devices without the need for a central server. This approach forms the backbone of many decentralized applications and services.
Core Concepts of P2P Programming
- Distributed Hash Tables (DHTs): A mechanism for storing and retrieving data in a decentralized network
- NAT Traversal: Techniques for establishing direct connections between peers behind firewalls or NATs
- Peer Discovery: Methods for finding and connecting to other peers in the network
- Data Replication: Strategies for ensuring data availability and redundancy
- Consensus Algorithms: Mechanisms for achieving agreement in a decentralized system
Implementing a Basic P2P Network
Let’s look at a simplified example of how to implement a basic P2P network using JavaScript and WebRTC:
class Peer {
constructor(id) {
this.id = id;
this.connections = new Map();
}
connect(peerId, peerConnection) {
this.connections.set(peerId, peerConnection);
}
send(peerId, message) {
const connection = this.connections.get(peerId);
if (connection) {
connection.send(JSON.stringify(message));
}
}
onMessage(callback) {
this.connections.forEach((connection) => {
connection.on('data', (data) => {
const message = JSON.parse(data);
callback(message);
});
});
}
}
// Usage
const peer1 = new Peer('peer1');
const peer2 = new Peer('peer2');
// Establish connection (simplified, actual WebRTC setup would be more complex)
const connection = new RTCPeerConnection();
peer1.connect(peer2.id, connection);
peer2.connect(peer1.id, connection);
peer1.send(peer2.id, { type: 'hello', content: 'Hello from Peer 1!' });
peer2.onMessage((message) => {
console.log('Peer 2 received:', message);
});
This example demonstrates the basic structure of a P2P network, where peers can connect directly and exchange messages. In a real-world scenario, you’d need to handle peer discovery, NAT traversal, and other complexities.
P2P Applications and Use Cases
P2P programming enables a wide range of applications, including:
- File Sharing: Decentralized platforms for sharing files without a central server
- Cryptocurrency: The backbone of blockchain networks like Bitcoin
- Distributed Computing: Harnessing the power of multiple devices for complex computations
- Decentralized Social Networks: Platforms that give users control over their data and connections
- Content Delivery Networks (CDNs): Efficient distribution of content across a network of peers
Challenges and Considerations
While mesh networks and P2P programming offer numerous advantages, they also come with their own set of challenges:
Security Concerns
Decentralized networks can be vulnerable to various attacks, including:
- Sybil attacks: Where an attacker creates multiple fake identities to gain influence
- Eclipse attacks: Isolating a node from the rest of the network
- Man-in-the-middle attacks: Intercepting and potentially altering communications
To mitigate these risks, developers must implement robust security measures such as:
function validatePeer(peer) {
// Implement peer validation logic
if (!isValidPeerSignature(peer.signature)) {
throw new Error('Invalid peer signature');
}
if (isBlacklisted(peer.id)) {
throw new Error('Peer is blacklisted');
}
// Additional validation checks...
}
function encryptMessage(message, recipientPublicKey) {
// Implement end-to-end encryption
return encryptWithPublicKey(message, recipientPublicKey);
}
Scalability and Performance
As networks grow, ensuring efficient routing and data distribution becomes increasingly challenging. Developers must consider:
- Optimizing routing algorithms for large-scale networks
- Implementing efficient data replication strategies
- Balancing network load to prevent bottlenecks
Regulatory and Legal Issues
The decentralized nature of mesh networks and P2P systems can sometimes conflict with existing regulations, particularly in areas such as:
- Data protection and privacy laws
- Content distribution rights
- Telecommunications regulations
Developers and network operators must navigate these legal landscapes carefully to ensure compliance while preserving the benefits of decentralization.
The Future of Decentralized Networking
As we look to the future, several emerging trends and technologies are set to further transform the landscape of mesh networks and P2P programming:
5G and Beyond
The rollout of 5G networks and the development of 6G technologies will enable faster, more reliable connections between devices. This will enhance the capabilities of mesh networks, allowing for more complex and data-intensive applications.
Integration with Artificial Intelligence
AI and machine learning algorithms can be leveraged to optimize network routing, predict node failures, and enhance security in decentralized networks. For example:
class AIEnhancedMeshNode extends MeshNode {
constructor(id, aiModel) {
super(id);
this.aiModel = aiModel;
}
predictOptimalRoute(destination) {
return this.aiModel.predict({
source: this.id,
destination: destination,
networkState: this.getCurrentNetworkState()
});
}
detectAnomalies() {
const anomalies = this.aiModel.detectAnomalies(this.getTrafficPatterns());
if (anomalies.length > 0) {
this.raiseAlert(anomalies);
}
}
}
Quantum Networking
The advent of quantum computing and quantum communication technologies could revolutionize P2P networks, offering unprecedented levels of security through quantum key distribution and enabling new forms of distributed computing.
Interplanetary Mesh Networks
As space exploration advances, the concept of mesh networks could extend beyond Earth, creating interplanetary communication networks that could support future space colonies and deep space missions.
Practical Steps for Getting Started
For developers looking to dive into mesh networks and P2P programming, here are some practical steps to get started:
- Learn the Fundamentals: Study the core concepts of distributed systems, network protocols, and cryptography.
- Explore Existing Projects: Familiarize yourself with open-source projects like IPFS (InterPlanetary File System), libp2p, and Ethereum’s p2p network.
- Start Small: Begin by building simple P2P applications, such as a distributed chat system or a file-sharing tool.
- Experiment with Simulators: Use network simulators to test and visualize mesh network behaviors without deploying physical hardware.
- Join the Community: Participate in forums, attend conferences, and contribute to open-source projects to learn from experienced developers and stay updated on the latest trends.
Sample Project: Building a Simple P2P Chat Application
Here’s a basic example of how you might start building a P2P chat application using JavaScript and WebRTC:
class P2PChat {
constructor(peerId) {
this.peerId = peerId;
this.peers = new Map();
this.dataChannel = null;
}
async initializePeerConnection(remotePeerId) {
const peerConnection = new RTCPeerConnection();
this.dataChannel = peerConnection.createDataChannel('chat');
this.dataChannel.onmessage = (event) => {
console.log(`Received message from ${remotePeerId}: ${event.data}`);
};
const offer = await peerConnection.createOffer();
await peerConnection.setLocalDescription(offer);
// Send offer to remote peer (implementation depends on signaling method)
this.sendSignalingMessage(remotePeerId, { type: 'offer', sdp: offer });
this.peers.set(remotePeerId, peerConnection);
}
async handleIncomingSignalingMessage(message, fromPeerId) {
if (message.type === 'offer') {
const peerConnection = new RTCPeerConnection();
await peerConnection.setRemoteDescription(new RTCSessionDescription(message.sdp));
const answer = await peerConnection.createAnswer();
await peerConnection.setLocalDescription(answer);
// Send answer back to offering peer
this.sendSignalingMessage(fromPeerId, { type: 'answer', sdp: answer });
this.peers.set(fromPeerId, peerConnection);
} else if (message.type === 'answer') {
const peerConnection = this.peers.get(fromPeerId);
await peerConnection.setRemoteDescription(new RTCSessionDescription(message.sdp));
}
}
sendMessage(peerId, message) {
const dataChannel = this.peers.get(peerId).dataChannel;
if (dataChannel && dataChannel.readyState === 'open') {
dataChannel.send(message);
} else {
console.error('Data channel not ready');
}
}
sendSignalingMessage(peerId, message) {
// Implementation depends on your signaling server or method
console.log(`Sending signaling message to ${peerId}:`, message);
}
}
// Usage
const chat = new P2PChat('myPeerId');
chat.initializePeerConnection('remotePeerId');
// Later, after connection is established:
chat.sendMessage('remotePeerId', 'Hello, this is a P2P chat message!');
This example provides a basic structure for a P2P chat application. In a real-world scenario, you’d need to implement a signaling mechanism, handle ICE candidates, and add error handling and reconnection logic.
Conclusion
Mesh networks and P2P programming represent a paradigm shift in how we approach networking and distributed systems. By embracing decentralization, these technologies offer increased resilience, scalability, and user empowerment. As we continue to push the boundaries of what’s possible in the digital realm, mesh networks and P2P systems will play an increasingly crucial role in shaping the future of the internet and beyond.
For developers, mastering these technologies opens up a world of possibilities, from creating resilient communication systems to building the next generation of decentralized applications. As you embark on your journey into mesh networks and P2P programming, remember that you’re not just learning a new set of tools—you’re participating in a movement that’s reshaping the digital landscape.
The road ahead is filled with challenges, but also with immense opportunities for innovation and impact. By understanding the principles, tackling the challenges, and staying abreast of emerging trends, you’ll be well-equipped to contribute to this exciting field and help shape the decentralized future of technology.