Mental Models for Problem-Solving: A Cheat Sheet for Cracking Coding Interviews
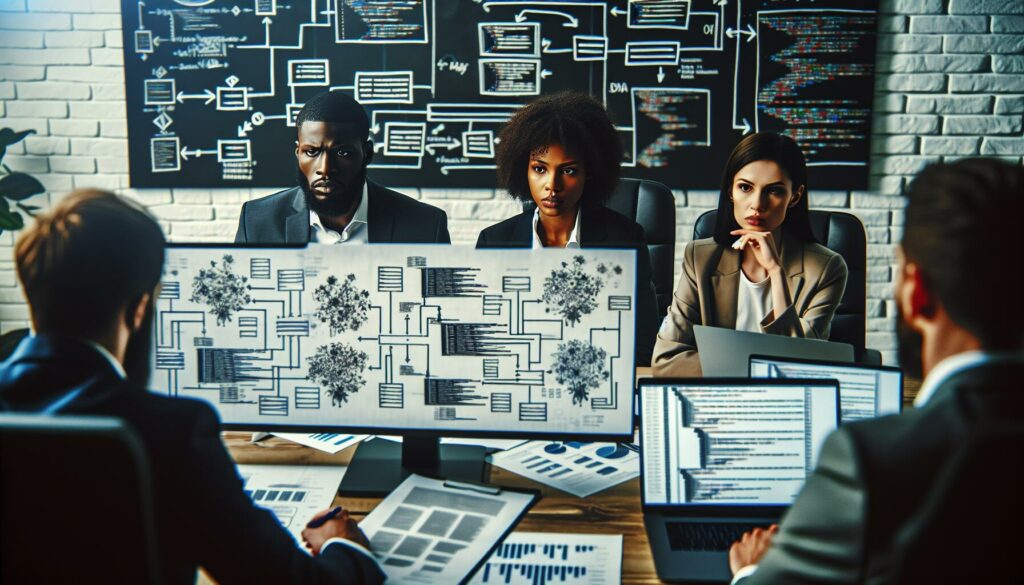
In the world of coding interviews, success often hinges on more than just knowing algorithms and data structures. It’s about having the right mental models to approach problems efficiently and effectively. These mental models serve as powerful tools that help you visualize complex problems, break them down into manageable pieces, and devise optimal solutions. In this comprehensive guide, we’ll explore various mental models that can significantly boost your problem-solving skills and give you an edge in coding interviews.
1. Recursion Trees: Visualizing Recursive Problems
Recursion is a fundamental concept in programming, and mastering it can be a game-changer in coding interviews. Recursion trees provide a visual representation of how recursive algorithms work, making it easier to understand their flow and complexity.
How to Use Recursion Trees
- Start with the initial problem at the root of the tree.
- Break down the problem into smaller subproblems, representing each as a child node.
- Continue this process until you reach the base cases (leaf nodes).
- Analyze the tree to understand the algorithm’s behavior and complexity.
Example: Fibonacci Sequence
Let’s visualize the recursive calculation of the 5th Fibonacci number:
fib(5)
/ \
fib(4) fib(3)
/ \ / \
fib(3) fib(2) fib(2) fib(1)
/ \
fib(2) fib(1)
This tree helps us see the overlapping subproblems and understand why a simple recursive implementation of Fibonacci is inefficient (O(2^n) time complexity).
Benefits of Recursion Trees
- Helps identify overlapping subproblems, leading to optimization opportunities (e.g., dynamic programming).
- Provides a clear visual of the recursive call stack, aiding in debugging and optimization.
- Assists in analyzing time and space complexity of recursive algorithms.
2. Dynamic Programming Tables: Optimizing Overlapping Subproblems
Dynamic Programming (DP) is a powerful technique for solving optimization problems by breaking them down into simpler subproblems. DP tables provide a structured way to visualize and solve these problems efficiently.
How to Use DP Tables
- Identify the problem’s dimensions (usually 1D or 2D).
- Create a table to store solutions to subproblems.
- Define the base cases and fill in the corresponding cells.
- Iterate through the table, filling each cell based on previously computed values.
- The final cell(s) will contain the solution to the original problem.
Example: Longest Common Subsequence (LCS)
Let’s visualize solving the LCS problem for sequences “ABCDGH” and “AEDFHR”:
A E D F H R
0 0 0 0 0 0 0
A 0 1 1 1 1 1 1
B 0 1 1 1 1 1 1
C 0 1 1 1 1 1 1
D 0 1 1 2 2 2 2
G 0 1 1 2 2 2 2
H 0 1 1 2 2 3 3
This table shows how we build up the solution, with the bottom-right cell giving us the length of the LCS (3).
Benefits of DP Tables
- Provides a systematic approach to solving complex optimization problems.
- Helps in identifying the optimal substructure of a problem.
- Enables efficient memoization, reducing time complexity from exponential to polynomial in many cases.
3. Backtracking Paths: Exploring All Possibilities
Backtracking is a general algorithm for finding all (or some) solutions to computational problems, particularly those that involve making a sequence of decisions. Visualizing backtracking paths can greatly aid in understanding and implementing these algorithms.
How to Use Backtracking Paths
- Start with an empty solution and a set of choices.
- Make a choice and add it to the current solution.
- Recursively explore further choices.
- If the current path doesn’t lead to a valid solution, backtrack (undo the last choice).
- Continue until all possibilities are explored.
Example: N-Queens Problem
Let’s visualize the backtracking process for placing 4 queens on a 4×4 chessboard:
Level 1: Q _ _ _ Q _ _ _ Q _ _ _ Q _ _ _
_ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _
_ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _
_ _ _ _ _ _ _ _ _ _ _ _ _ _ _ _
Level 2: Q _ _ _ Q _ _ _ Q _ _ _
_ _ Q _ _ _ _ Q _ Q _ _
_ _ _ _ _ _ _ _ _ _ _ _
_ _ _ _ _ _ _ _ _ _ _ _
Level 3: Q _ _ _ Q _ _ _
_ _ Q _ _ _ _ Q
_ _ _ Q _ Q _ _
_ _ _ _ _ _ _ _
Level 4: Q _ _ _
_ _ Q _
_ _ _ Q
_ Q _ _
This visualization shows how we explore different queen placements, backtracking when we reach an invalid configuration.
Benefits of Backtracking Paths
- Helps in understanding the decision-making process in combinatorial problems.
- Aids in identifying pruning opportunities to optimize the algorithm.
- Provides a clear picture of the search space, helping in complexity analysis.
4. Sliding Window: Optimizing Subarray Problems
The sliding window technique is a powerful mental model for solving problems involving subarrays or substrings. It’s particularly useful for optimizing brute force approaches in array or string manipulation problems.
How to Use the Sliding Window Model
- Define a window with start and end pointers.
- Expand the window by moving the end pointer to include new elements.
- Contract the window by moving the start pointer when certain conditions are met.
- Process the elements within the current window.
- Repeat steps 2-4 until the end of the array/string is reached.
Example: Maximum Sum Subarray of Size K
Let’s visualize finding the maximum sum subarray of size 3 in the array [1, 4, 2, 10, 23, 3, 1, 0, 20]:
Step 1: [1, 4, 2], 10, 23, 3, 1, 0, 20 Sum = 7
Step 2: 1, [4, 2, 10], 23, 3, 1, 0, 20 Sum = 16
Step 3: 1, 4, [2, 10, 23], 3, 1, 0, 20 Sum = 35
Step 4: 1, 4, 2, [10, 23, 3], 1, 0, 20 Sum = 36
Step 5: 1, 4, 2, 10, [23, 3, 1], 0, 20 Sum = 27
Step 6: 1, 4, 2, 10, 23, [3, 1, 0], 20 Sum = 4
Step 7: 1, 4, 2, 10, 23, 3, [1, 0, 20] Sum = 21
Maximum Sum = 36
Benefits of the Sliding Window Model
- Reduces time complexity from O(n^2) to O(n) for many subarray problems.
- Provides an intuitive way to process consecutive elements in arrays or strings.
- Helps in solving problems related to subarrays, substrings, and pattern matching efficiently.
5. Two Pointers: Navigating Arrays and Linked Lists
The two-pointer technique is a simple yet powerful mental model for solving problems involving arrays, linked lists, or strings. It involves using two pointers that move through the data structure in a coordinated manner.
How to Use the Two-Pointer Model
- Initialize two pointers, often at different positions in the data structure.
- Move the pointers based on certain conditions or rules.
- Process elements at the pointer positions.
- Continue until a specific condition is met or the entire structure is traversed.
Example: Reverse a String
Let’s visualize reversing the string “hello” using two pointers:
Step 1: h e l l o left = 0, right = 4
^ ^
Step 2: o e l l h left = 1, right = 3
^ ^
Step 3: o l l e h left = 2, right = 2
^
Benefits of the Two-Pointer Model
- Efficient for problems involving searching, reversing, or comparing elements in linear data structures.
- Often reduces time complexity from O(n^2) to O(n).
- Useful for in-place operations, saving space complexity.
6. Graph Traversal: Visualizing Paths and Connections
Graph traversal is a fundamental technique in computer science, used to explore and analyze interconnected data. Two primary mental models for graph traversal are Depth-First Search (DFS) and Breadth-First Search (BFS).
How to Use Graph Traversal Models
Depth-First Search (DFS)
- Start at a chosen node.
- Explore as far as possible along each branch before backtracking.
- Use a stack (or recursion) to keep track of nodes to visit.
Breadth-First Search (BFS)
- Start at a chosen node.
- Explore all neighbor nodes at the present depth before moving to nodes at the next depth level.
- Use a queue to keep track of nodes to visit.
Example: Graph Traversal
Consider the following graph:
1 --- 2 --- 5
| |
3 --- 4
DFS traversal (starting from 1): 1, 2, 5, 4, 3
BFS traversal (starting from 1): 1, 2, 3, 5, 4
Benefits of Graph Traversal Models
- Essential for solving problems involving networks, paths, and connections.
- DFS is useful for exploring paths, cycle detection, and topological sorting.
- BFS is ideal for finding shortest paths in unweighted graphs and level-order traversals.
7. Binary Search: Divide and Conquer
Binary search is a powerful mental model for efficiently searching sorted arrays or solving problems with a monotonic search space. It’s based on the divide-and-conquer paradigm.
How to Use the Binary Search Model
- Define the search space (usually an array or a range of values).
- Find the middle element of the search space.
- Compare the middle element with the target value.
- Eliminate half of the search space based on the comparison.
- Repeat steps 2-4 until the target is found or the search space is exhausted.
Example: Finding a Number in a Sorted Array
Let’s visualize finding the number 23 in the sorted array [2, 5, 8, 12, 16, 23, 38, 56, 72, 91]:
Step 1: [2, 5, 8, 12, 16, 23, 38, 56, 72, 91] mid = 16 < 23
^
Step 2: [23, 38, 56, 72, 91] mid = 56 > 23
^
Step 3: [23, 38] mid = 23 = 23 (Found!)
^
Benefits of the Binary Search Model
- Reduces time complexity from O(n) to O(log n) for searching in sorted arrays.
- Applicable to various problem types beyond simple searching (e.g., finding peak elements, square roots).
- Useful for optimizing solutions in problems with monotonic properties.
8. State Machines: Modeling Complex Systems
State machines provide a powerful mental model for solving problems that involve transitions between different states or conditions. They’re particularly useful in parsing, game logic, and workflow management.
How to Use the State Machine Model
- Identify the possible states of the system.
- Define the transitions between states and the conditions that trigger them.
- Implement the logic for each state and transition.
- Process input by moving through states according to the defined transitions.
Example: Parsing a Simple Mathematical Expression
Let’s visualize a state machine for parsing expressions like “3 + 4 * 2”:
[Start] --> (Number) --> (Operator) --> (Number) --> [End]
^ | ^ |
| | | |
+---------+ +---------+
Benefits of the State Machine Model
- Provides a clear structure for complex logic with multiple conditions.
- Helps in breaking down complex problems into manageable states and transitions.
- Useful for implementing parsers, validators, and systems with well-defined rules.
Conclusion: Leveraging Mental Models for Success
Mastering these mental models is crucial for excelling in coding interviews and becoming a proficient problem solver. Each model provides a unique perspective and approach to tackling different types of problems:
- Recursion Trees help visualize and optimize recursive algorithms.
- Dynamic Programming Tables enable efficient solutions to optimization problems.
- Backtracking Paths guide through exhaustive search problems.
- Sliding Window optimizes subarray and substring problems.
- Two Pointers efficiently navigate linear data structures.
- Graph Traversal explores interconnected data and networks.
- Binary Search efficiently searches and optimizes in sorted or monotonic spaces.
- State Machines model complex systems with distinct states and transitions.
By internalizing these models, you’ll be better equipped to:
- Quickly identify the type of problem you’re facing.
- Choose the most appropriate approach for solving it.
- Visualize the problem-solving process, making it easier to implement and explain your solution.
- Optimize your solutions by leveraging the inherent efficiencies of these models.
Remember, the key to mastering these mental models is practice. Regularly solve problems that employ these techniques, and try to visualize your approach using these models. Over time, you’ll develop an intuition for which model to apply to different problem types, significantly boosting your problem-solving skills and interview performance.
As you prepare for coding interviews, don’t just memorize algorithms and data structures. Instead, focus on understanding and applying these mental models. They will not only help you solve problems more effectively but also demonstrate to interviewers your ability to think critically and approach complex problems systematically.
With these powerful mental models in your toolkit, you’ll be well-prepared to tackle a wide range of coding challenges and excel in your technical interviews. Happy coding, and best of luck in your interview preparations!