Memory Management for Beginners: What’s Going on Under the Hood
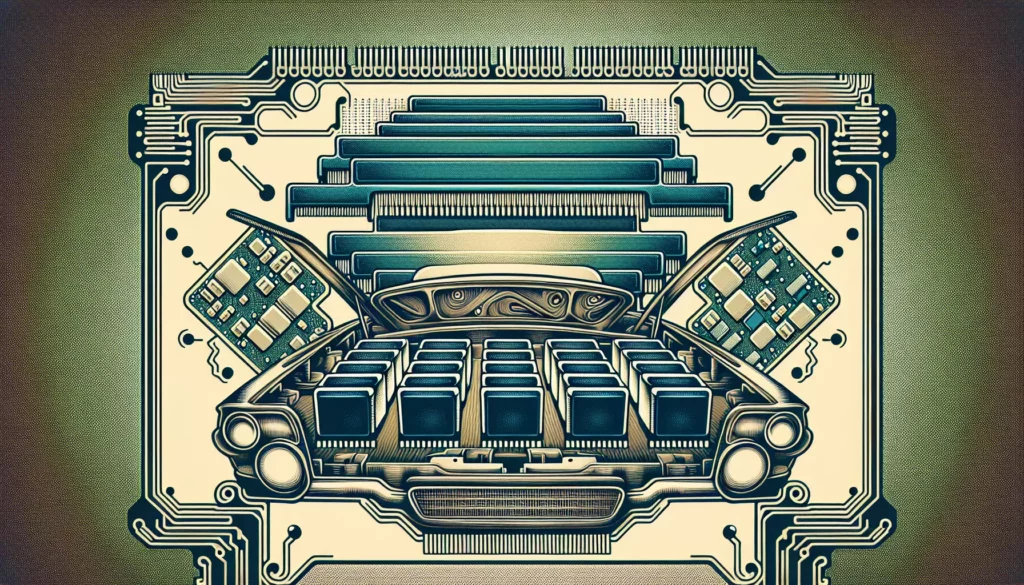
As you embark on your journey to become a proficient programmer, understanding memory management is crucial. It’s one of those fundamental concepts that separates novice coders from seasoned developers. In this comprehensive guide, we’ll dive deep into the world of memory management, exploring what happens behind the scenes when you run your code. By the end of this article, you’ll have a solid grasp of how computers handle memory, why it matters, and how you can write more efficient code.
Table of Contents
- What is Memory Management?
- Types of Memory
- Stack vs. Heap
- Memory Allocation
- Garbage Collection
- Memory Leaks
- Best Practices for Efficient Memory Management
- Language-Specific Memory Management
- Tools for Memory Management
- Conclusion
What is Memory Management?
Memory management is the process of controlling and coordinating how a computer program accesses computer memory. It involves allocating portions of memory to various programs and processes, ensuring that each has enough memory to execute efficiently, and freeing up memory that is no longer needed.
Effective memory management is crucial for several reasons:
- It ensures that your program runs smoothly without crashing due to memory-related issues.
- It improves the overall performance of your application by efficiently utilizing available resources.
- It prevents memory leaks, which can cause your program to consume more and more memory over time.
- It allows multiple programs to run concurrently without interfering with each other’s memory space.
Types of Memory
Before we dive deeper into memory management, it’s essential to understand the different types of memory in a computer system:
1. RAM (Random Access Memory)
RAM is the primary memory of a computer. It’s volatile, meaning it loses its contents when the power is turned off. RAM is used to store data and instructions that are actively being used or processed by the CPU.
2. ROM (Read-Only Memory)
ROM is non-volatile memory that retains its contents even when the power is turned off. It typically stores firmware or essential instructions that the computer needs to boot up.
3. Cache Memory
Cache is a small amount of high-speed memory that stores frequently accessed data and instructions. It helps bridge the speed gap between the CPU and main memory.
4. Virtual Memory
Virtual memory is a memory management technique that uses both RAM and hard disk space to give programs the impression that they have more memory available than the physical RAM installed in the computer.
Stack vs. Heap
When it comes to memory management in programming, two key areas of memory are the stack and the heap. Understanding the difference between these two is crucial for writing efficient code.
The Stack
The stack is a region of memory that follows a Last-In-First-Out (LIFO) structure. It’s used for storing local variables, function parameters, and return addresses. Key characteristics of the stack include:
- Fast allocation and deallocation
- Limited in size (typically a few megabytes)
- Automatically managed by the compiler
- Used for static memory allocation
Here’s a simple example of how the stack works in C++:
void exampleFunction() {
int x = 5; // Allocated on the stack
int y = 10; // Also on the stack
// x and y are automatically deallocated when the function ends
}
The Heap
The heap is a region of memory used for dynamic memory allocation. It’s more flexible than the stack but slower to allocate and deallocate. Key characteristics of the heap include:
- Larger size (limited by available system memory)
- Manually managed in languages without garbage collection
- Used for dynamic memory allocation
- More prone to fragmentation
Here’s an example of heap allocation in C++:
int* dynamicInt = new int; // Allocates memory on the heap
*dynamicInt = 42;
// ... use the dynamicInt ...
delete dynamicInt; // Manually free the allocated memory
Memory Allocation
Memory allocation is the process of reserving a portion of computer memory for a specific purpose. There are two main types of memory allocation:
1. Static Memory Allocation
Static memory allocation occurs at compile-time. The compiler allocates a fixed amount of memory for variables that have a predetermined size. This type of allocation is typically used for global variables and local variables with a fixed size.
Example of static allocation in C:
int staticArray[10]; // Statically allocated array of 10 integers
2. Dynamic Memory Allocation
Dynamic memory allocation occurs at runtime. It allows programs to request memory as needed during execution. This is particularly useful when the amount of memory needed is not known in advance or when working with data structures that can grow or shrink.
Example of dynamic allocation in C:
int* dynamicArray = (int*)malloc(10 * sizeof(int)); // Dynamically allocated array of 10 integers
// Use the array...
free(dynamicArray); // Don't forget to free the memory when done!
In languages with automatic memory management, like Java or Python, you don’t need to explicitly free memory, as the garbage collector handles this for you.
Garbage Collection
Garbage collection is an automatic memory management feature in many modern programming languages. It’s responsible for identifying and removing objects that are no longer needed by the program, freeing up memory for reuse.
The basic process of garbage collection involves:
- Marking: The garbage collector identifies which objects in memory are still in use.
- Sweeping: It removes the objects that are no longer needed.
- Compacting: Optionally, it may reorganize the remaining objects to reduce fragmentation.
While garbage collection relieves programmers from manual memory management, it’s not without drawbacks. Garbage collection can introduce performance overhead and unpredictable pauses in program execution.
Here’s a simple example in Java, where garbage collection happens automatically:
public class GCExample {
public static void main(String[] args) {
for (int i = 0; i < 1000000; i++) {
String s = new String("Hello, World!"); // Creates many objects
}
// The garbage collector will automatically clean up unused String objects
}
}
Memory Leaks
A memory leak occurs when a program fails to release memory that is no longer needed. Over time, these leaks can cause a program to consume more and more memory, potentially leading to performance issues or crashes.
Common causes of memory leaks include:
- Failing to free dynamically allocated memory
- Keeping references to objects that are no longer needed
- Circular references in languages with reference counting
Here’s an example of a potential memory leak in C++:
void leakyFunction() {
int* ptr = new int[1000]; // Allocate memory
// ... do something with ptr ...
// Oops! We forgot to delete[] ptr
}
int main() {
while(true) {
leakyFunction(); // This will continuously allocate memory without freeing it
}
return 0;
}
To prevent memory leaks, always ensure that you free any dynamically allocated memory when it’s no longer needed. In languages with garbage collection, be mindful of holding onto references to large objects unnecessarily.
Best Practices for Efficient Memory Management
Regardless of whether you’re working with a language that has manual or automatic memory management, following these best practices can help you write more efficient and reliable code:
- Understand your language’s memory model: Each programming language handles memory differently. Familiarize yourself with how your chosen language manages memory.
- Use appropriate data structures: Choose data structures that are suitable for your needs. For example, use a linked list instead of an array if you need frequent insertions and deletions.
- Avoid unnecessary object creation: Creating objects can be expensive. Reuse objects when possible, especially in performance-critical sections of your code.
- Release resources promptly: In languages with manual memory management, always free memory when you’re done with it. In garbage-collected languages, set objects to null when you’re finished with them to help the garbage collector.
- Use smart pointers: In C++, smart pointers can help manage memory automatically and prevent common pitfalls like memory leaks.
- Be cautious with global variables: Global variables persist throughout the lifetime of the program and can unnecessarily occupy memory.
- Profile your code: Use profiling tools to identify memory-intensive parts of your program and optimize them.
- Implement proper error handling: Ensure that resources are properly released even when exceptions occur.
- Use memory pools for frequent allocations: If your program frequently allocates and deallocates objects of the same size, consider using a memory pool to improve performance.
- Be mindful of closures and callbacks: These can inadvertently keep objects alive longer than necessary in garbage-collected languages.
Language-Specific Memory Management
Different programming languages handle memory management in various ways. Let’s look at a few popular languages and their approaches:
C and C++
C and C++ provide low-level control over memory management. Developers are responsible for allocating and freeing memory manually.
// C example
int* arr = (int*)malloc(10 * sizeof(int));
// Use the array...
free(arr);
// C++ example
int* arr = new int[10];
// Use the array...
delete[] arr;
Java
Java uses automatic memory management with garbage collection. Developers create objects, and the JVM handles memory allocation and deallocation.
List<String> list = new ArrayList<>();
list.add("Hello");
list.add("World");
// No need to manually free memory
Python
Python also uses garbage collection for memory management. It employs reference counting along with generational garbage collection.
def create_list():
my_list = [1, 2, 3, 4, 5]
# The list will be automatically garbage collected when it goes out of scope
create_list()
Rust
Rust uses a unique ownership system with borrowing rules, allowing for memory safety without a garbage collector.
fn main() {
let s1 = String::from("hello");
let s2 = s1; // s1 is moved here and can no longer be used
println!("{}", s2);
}
Tools for Memory Management
Several tools can help you analyze and optimize your program’s memory usage:
- Valgrind: A powerful tool suite for debugging and profiling, particularly useful for C and C++ programs.
- JProfiler: A Java profiler that helps you analyze memory usage, detect memory leaks, and optimize your Java applications.
- Memory Profiler: A .NET memory profiler for tracking memory usage in .NET applications.
- Python Memory Profiler: A module for monitoring memory consumption of a Python program.
- Chrome DevTools: Provides memory profiling capabilities for JavaScript in web applications.
Using these tools can help you identify memory leaks, understand memory usage patterns, and optimize your code for better performance.
Conclusion
Memory management is a crucial aspect of programming that directly impacts the performance, efficiency, and reliability of your software. Whether you’re working with a language that requires manual memory management or one with automatic garbage collection, understanding what’s happening under the hood is invaluable.
As you continue your journey in programming, keep these key points in mind:
- Understand the difference between stack and heap memory
- Be aware of how your chosen language handles memory allocation and deallocation
- Practice good memory management habits to prevent leaks and improve performance
- Utilize appropriate tools to profile and optimize your code’s memory usage
By mastering memory management, you’ll be better equipped to write efficient, robust, and scalable code – skills that are highly valued in the tech industry, especially when preparing for technical interviews at major companies.
Remember, like any programming concept, proficiency in memory management comes with practice. Keep coding, experimenting, and analyzing your programs, and you’ll soon find yourself confidently handling even the most complex memory-related challenges.