Maximum Square Submatrix: A Deep Dive into Dynamic Programming
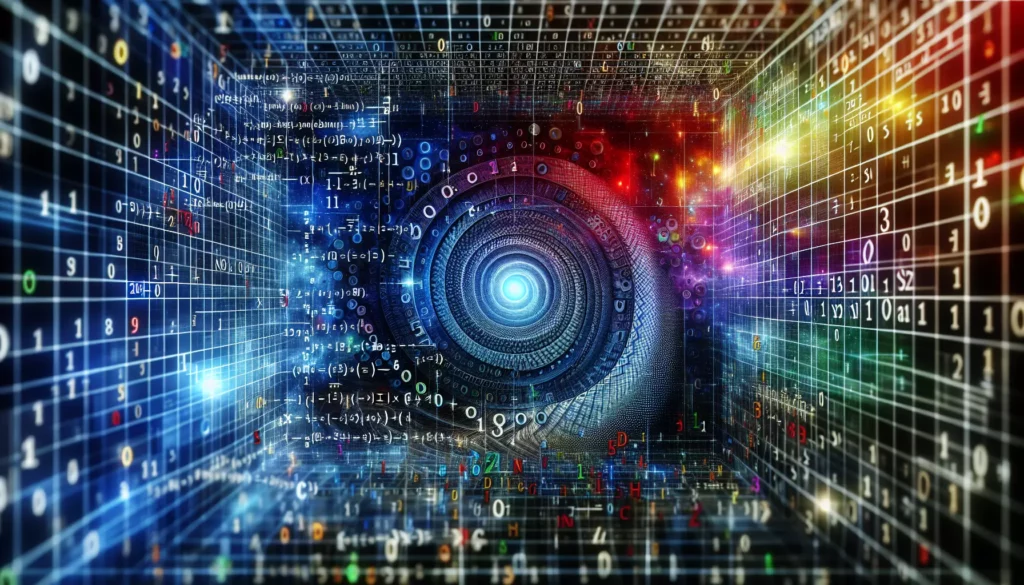
Welcome to another exciting exploration of algorithmic problem-solving! Today, we’re going to tackle a classic dynamic programming problem: finding the maximum square submatrix in a binary matrix. This problem is not only a favorite in coding interviews but also a great way to sharpen your skills in dynamic programming and matrix manipulation.
Understanding the Problem
Before we dive into the solution, let’s clearly define what we’re trying to achieve:
Problem Statement: Given a binary matrix (a matrix containing only 0s and 1s), find the largest square submatrix of 1s and return its area.
For example, consider the following matrix:
1 0 1 0 0
1 0 1 1 1
1 1 1 1 1
1 0 0 1 0
The largest square submatrix of 1s in this matrix is 3×3, so the answer would be 9 (the area of the submatrix).
Approaching the Problem
At first glance, this problem might seem daunting. You might be tempted to try a brute force approach, checking every possible square submatrix. However, that would be highly inefficient, especially for large matrices. Instead, we’ll use dynamic programming to solve this problem efficiently.
The Dynamic Programming Insight
The key insight for this problem is that we can build our solution incrementally. We can start from the top-left corner of the matrix and work our way to the bottom-right, keeping track of the largest square submatrix that ends at each cell.
Here’s the crucial observation: For any cell (i, j), the size of the largest square submatrix ending at (i, j) depends on three adjacent cells:
- (i-1, j) (the cell above)
- (i, j-1) (the cell to the left)
- (i-1, j-1) (the cell diagonally up and to the left)
If the current cell (i, j) contains a 1, then the size of the largest square submatrix ending at (i, j) is the minimum of these three adjacent cells, plus 1.
Implementing the Solution
Now that we understand the approach, let’s implement it in Python:
def maximal_square(matrix):
if not matrix or not matrix[0]:
return 0
rows, cols = len(matrix), len(matrix[0])
dp = [[0] * (cols + 1) for _ in range(rows + 1)]
max_side = 0
for i in range(1, rows + 1):
for j in range(1, cols + 1):
if matrix[i-1][j-1] == '1':
dp[i][j] = min(dp[i-1][j], dp[i][j-1], dp[i-1][j-1]) + 1
max_side = max(max_side, dp[i][j])
return max_side * max_side
Let’s break down this implementation:
- We first check if the matrix is empty. If it is, we return 0.
- We create a 2D DP table with an extra row and column to handle edge cases smoothly.
- We iterate through each cell in the matrix.
- For each cell that contains a ‘1’, we update our DP table based on the minimum of the three adjacent cells, plus 1.
- We keep track of the maximum side length we’ve seen so far.
- Finally, we return the area of the largest square submatrix, which is the square of the maximum side length.
Time and Space Complexity
Let’s analyze the time and space complexity of our solution:
- Time Complexity: O(m * n), where m is the number of rows and n is the number of columns in the matrix. We iterate through each cell once.
- Space Complexity: O(m * n) as well, because we create a DP table of the same size as the input matrix (plus one extra row and column).
Optimizing Space Complexity
While our current solution is efficient in terms of time complexity, we can optimize the space complexity. Notice that for each cell, we only need information from the current row and the previous row. This means we can reduce our space complexity to O(n) by only keeping track of two rows at a time.
Here’s the optimized version:
def maximal_square_optimized(matrix):
if not matrix or not matrix[0]:
return 0
rows, cols = len(matrix), len(matrix[0])
dp = [0] * (cols + 1)
max_side = 0
prev = 0
for i in range(1, rows + 1):
for j in range(1, cols + 1):
temp = dp[j]
if matrix[i-1][j-1] == '1':
dp[j] = min(dp[j], dp[j-1], prev) + 1
max_side = max(max_side, dp[j])
else:
dp[j] = 0
prev = temp
return max_side * max_side
In this optimized version:
- We use a 1D array
dp
instead of a 2D array. - We use
prev
to keep track of the value diagonally up and to the left. - We update
dp[j]
in place, usingtemp
to store the old value before updating.
This optimized version has a space complexity of O(n) while maintaining the same time complexity of O(m * n).
Variations and Related Problems
The Maximum Square Submatrix problem is a great foundation for understanding dynamic programming on 2D grids. Here are some related problems that you might encounter:
- Maximal Rectangle: Instead of finding the largest square, find the largest rectangle of 1s in the matrix. This is a more challenging variation of our problem.
- Count Square Submatrices with All Ones: Count the total number of square submatrices filled with 1s. This problem uses a very similar DP approach.
- Largest Plus Sign: Find the largest plus sign (+) of 1s in a binary matrix. This problem also uses DP but requires thinking about the problem from four directions.
- Maximal Square II: Similar to our problem, but allows at most one 0 in the square submatrix.
Real-World Applications
While finding the largest square submatrix might seem like a purely academic exercise, it has several practical applications:
- Image Processing: In image analysis, this algorithm can be used to find the largest homogeneous square region in an image.
- Data Mining: In data mining applications, it can be used to find the largest consistent subset of data in a binary dataset.
- Urban Planning: In city planning, a variation of this algorithm could be used to find the largest square area satisfying certain criteria (e.g., zoning requirements).
- Computer Graphics: In rendering algorithms, identifying large square regions can help optimize drawing operations.
Common Mistakes and Pitfalls
When solving the Maximum Square Submatrix problem, there are a few common mistakes to watch out for:
- Off-by-One Errors: Be careful with your indexing, especially when dealing with the DP table and the original matrix.
- Forgetting to Square the Result: Remember that we’re returning the area, not the side length of the square.
- Not Handling Empty Matrices: Always check for empty input at the beginning of your function.
- Confusion with String vs Integer Input: Some versions of this problem provide the matrix as strings (‘0’ and ‘1’) rather than integers. Make sure you’re comparing with the correct type.
Tips for Solving Similar Problems
Dynamic programming problems like the Maximum Square Submatrix can be challenging. Here are some tips to help you approach similar problems:
- Identify the Subproblem: In DP problems, try to identify how you can break down the problem into smaller, similar subproblems.
- Define the State: Clearly define what each entry in your DP table represents.
- Find the Recurrence Relation: Determine how the solution to a larger problem relates to the solutions of smaller problems.
- Consider the Base Cases: Make sure you handle the smallest possible inputs correctly.
- Think About Optimization: Once you have a working solution, consider if you can optimize space or time complexity.
- Practice, Practice, Practice: The more DP problems you solve, the better you’ll become at recognizing patterns and applying DP techniques.
Conclusion
The Maximum Square Submatrix problem is a classic example of how dynamic programming can turn a seemingly complex problem into an elegant and efficient solution. By breaking down the problem into smaller subproblems and building our solution incrementally, we’ve created an algorithm that can solve the problem in linear time relative to the size of the input matrix.
As you continue your journey in algorithmic problem-solving, you’ll find that the principles we’ve discussed here – identifying subproblems, defining states, and finding recurrence relations – will serve you well in tackling a wide variety of challenges. Dynamic programming is a powerful tool in any programmer’s toolkit, and mastering problems like this one is a great step towards becoming proficient in this technique.
Remember, the key to getting better at these types of problems is practice. Try implementing the solution yourself, play around with different inputs, and see if you can solve some of the variations we mentioned. Happy coding!