Maximal Rectangle in Binary Matrix: A Comprehensive Guide
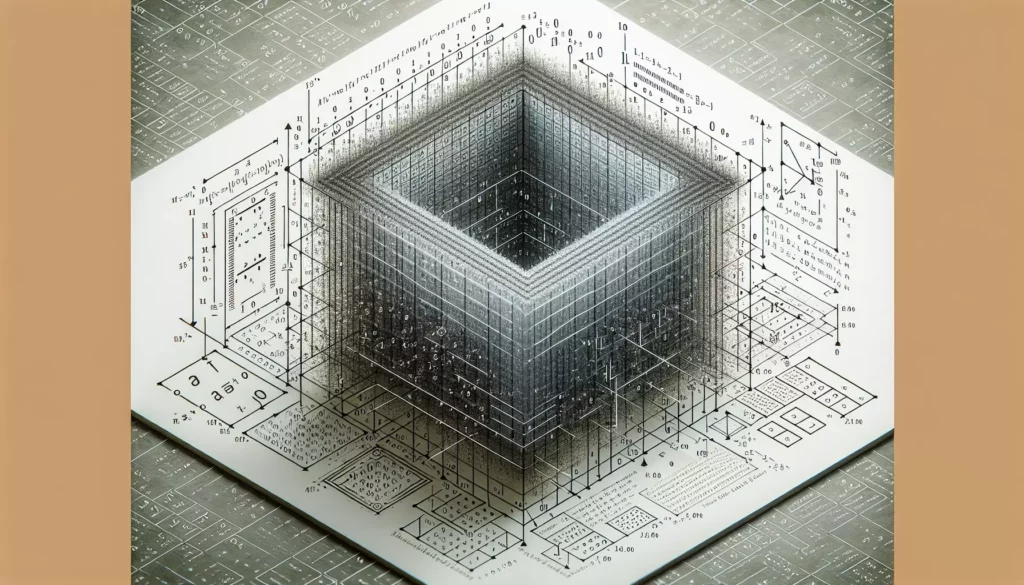
Welcome to another deep dive into algorithmic problem-solving! Today, we’re tackling a classic problem that often appears in technical interviews and coding challenges: finding the maximal rectangle in a binary matrix. This problem is not only intellectually stimulating but also has practical applications in image processing, data analysis, and more. Let’s unravel this complex problem step by step, providing you with the knowledge and skills to ace your next coding interview.
Understanding the Problem
Before we dive into the solution, let’s clearly define the problem:
Given a 2D binary matrix filled with 0’s and 1’s, find the largest rectangle containing only 1’s and return its area.
For example, consider the following matrix:
1 0 1 0 0
1 0 1 1 1
1 1 1 1 1
1 0 0 1 0
The largest rectangle containing only 1’s has an area of 6 (2×3 rectangle).
Approaching the Problem
At first glance, this problem might seem daunting. However, we can break it down into smaller, more manageable steps. The key insight is to leverage the solution to a related problem: the “Largest Rectangle in Histogram” problem.
Here’s our approach:
- We’ll treat each row of the matrix as a histogram.
- For each row, we’ll calculate the maximum rectangle area considering all rows up to and including the current row.
- We’ll use the “Largest Rectangle in Histogram” algorithm as a subroutine.
- We’ll keep track of the maximum area found across all rows.
The “Largest Rectangle in Histogram” Problem
Before we tackle our main problem, let’s first understand and solve the “Largest Rectangle in Histogram” problem. This will be a crucial component of our final solution.
Problem Statement
Given an array of integers heights representing the histogram’s bar height where the width of each bar is 1, return the area of the largest rectangle in the histogram.
Solution Using a Stack
We can solve this problem efficiently using a stack. The key idea is to maintain a stack of indices such that the corresponding heights are in ascending order. When we encounter a bar shorter than the one on top of the stack, we know we’ve found the right boundary of a rectangle. The left boundary is the bar just before the one we’re popping from the stack.
Here’s the implementation in Python:
def largestRectangleArea(heights):
stack = [-1]
heights.append(0)
max_area = 0
for i in range(len(heights)):
while heights[i] < heights[stack[-1]]:
h = heights[stack.pop()]
w = i - stack[-1] - 1
max_area = max(max_area, h * w)
stack.append(i)
return max_area
Solving the Maximal Rectangle Problem
Now that we have a solution for the “Largest Rectangle in Histogram” problem, we can use it to solve our main problem. Here’s how we’ll do it:
- Initialize a histogram array with the same width as the matrix, filled with zeros.
- Iterate through each row of the matrix:
- For each cell in the row:
- If the cell is 1, increment the corresponding histogram bar.
- If the cell is 0, reset the corresponding histogram bar to 0.
- Calculate the largest rectangle area for the current histogram.
- Update the maximum area if necessary.
Here’s the implementation in Python:
def maximalRectangle(matrix):
if not matrix or not matrix[0]:
return 0
n = len(matrix[0])
heights = [0] * n
max_area = 0
for row in matrix:
for i in range(n):
if row[i] == '1':
heights[i] += 1
else:
heights[i] = 0
max_area = max(max_area, largestRectangleArea(heights))
return max_area
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our solution:
Time Complexity
The time complexity of this solution is O(R * C), where R is the number of rows and C is the number of columns in the matrix. Here’s why:
- We iterate through each cell in the matrix once, which takes O(R * C) time.
- For each row, we call largestRectangleArea, which takes O(C) time.
- We do this for each of the R rows, so the total time is O(R * C).
Space Complexity
The space complexity is O(C), where C is the number of columns in the matrix. This is because:
- We use an additional array heights of size C to store the histogram heights.
- The stack used in largestRectangleArea can grow up to size C in the worst case.
Optimizations and Variations
While our current solution is efficient, there are a few optimizations and variations worth considering:
1. In-place Histogram
Instead of using a separate heights array, we could modify the input matrix in-place to store the histogram heights. This would reduce our space complexity to O(1) if we’re allowed to modify the input. However, this approach may not be suitable if we need to preserve the original matrix.
2. Dynamic Programming Approach
There’s a dynamic programming solution to this problem that runs in O(R * C) time and O(C) space. It involves maintaining three arrays: height, left, and right. This approach can be more intuitive for some and might be slightly faster in practice due to better cache performance.
3. Bit Manipulation for Binary Matrices
If we know that our matrix is truly binary (contains only 0s and 1s) and the number of columns is small (say, less than 64), we can use bit manipulation techniques to optimize the solution further. Each row can be represented as a single integer, allowing for very fast operations.
Real-world Applications
The Maximal Rectangle problem isn’t just an academic exercise. It has several practical applications:
1. Image Processing
In image processing and computer vision, this algorithm can be used to find the largest rectangular region of interest in a binary image. This could be useful in tasks like object detection or document analysis.
2. Data Mining
In data mining, this algorithm can be applied to find the largest submatrix of all 1s in a binary dataset, which could represent the most significant cluster of related data points.
3. Architectural Design
In architectural or urban planning software, this algorithm could be used to find the largest buildable area in a plot of land, given certain constraints represented as a binary matrix.
4. Game Development
In game development, particularly for games with grid-based maps, this algorithm could be used to find the largest open area for placing objects or characters.
Common Pitfalls and How to Avoid Them
When implementing this solution, there are a few common pitfalls to watch out for:
1. Off-by-One Errors
Be careful with indexing, especially when calculating widths in the largestRectangleArea function. It’s easy to be off by one when dealing with stack indices.
2. Integer Overflow
If dealing with very large matrices, be mindful of potential integer overflow when calculating areas. In languages like Java or C++, you might need to use long instead of int.
3. Handling Empty Inputs
Don’t forget to handle edge cases like empty matrices or matrices with zero rows or columns.
4. Stack Underflow
In the largestRectangleArea function, ensure that the stack always has at least one element (we use -1 as a sentinel value) to avoid stack underflow errors.
Practice and Further Learning
To truly master this problem and similar algorithmic challenges, practice is key. Here are some suggestions:
- Implement the solution in your preferred programming language.
- Try solving the problem using the dynamic programming approach mentioned earlier.
- Solve related problems like “Maximal Square” (leetcode.com/problems/maximal-square/) to reinforce your understanding of dynamic programming on matrices.
- Explore variations of the problem, such as finding the maximal rectangle with a sum less than or equal to a given value.
- Practice explaining your solution as if you were in a technical interview. Being able to clearly communicate your thought process is just as important as coding the solution.
Conclusion
The Maximal Rectangle in Binary Matrix problem is a challenging yet rewarding algorithmic puzzle. By breaking it down into smaller subproblems and leveraging our knowledge of the “Largest Rectangle in Histogram” problem, we’ve developed an efficient solution.
Remember, the key to mastering these types of problems is not just about memorizing solutions, but understanding the underlying principles and problem-solving techniques. As you continue your journey in algorithmic problem-solving, you’ll find that the skills you’ve developed here – like using stacks efficiently, breaking down complex problems, and optimizing for time and space complexity – will serve you well in many other challenges.
Keep practicing, stay curious, and never stop learning. Happy coding!