Matrix Traversal: Mastering Spiral, Diagonal, and Zigzag Patterns
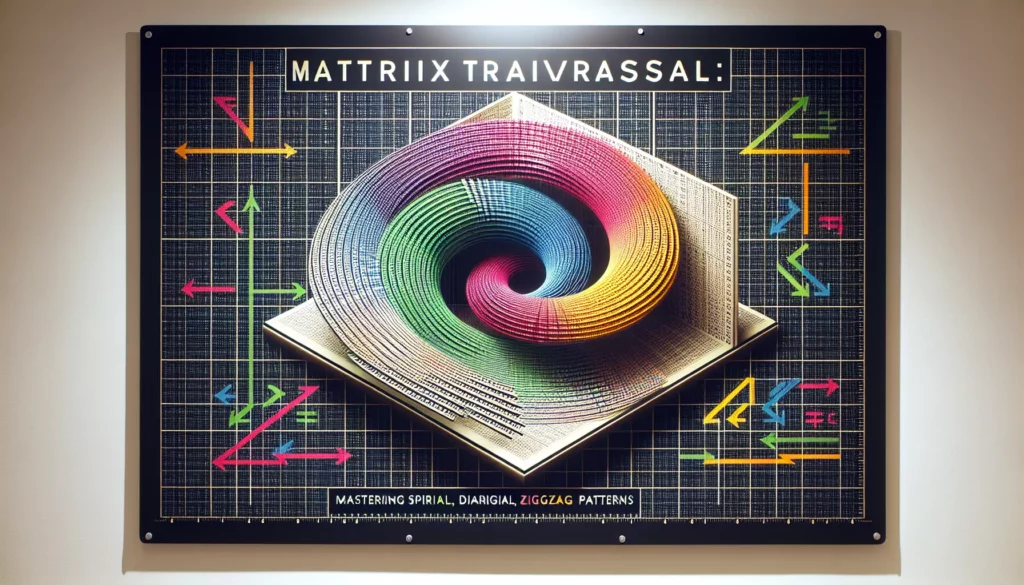
Matrix traversal is a fundamental concept in computer science and programming that involves systematically visiting every element in a two-dimensional array or grid. This skill is crucial for solving various problems in areas such as image processing, game development, and data analysis. In this comprehensive guide, we’ll explore three popular matrix traversal patterns: spiral, diagonal, and zigzag. We’ll dive deep into each pattern, providing clear explanations, step-by-step algorithms, and practical code examples to help you master these essential techniques.
1. Spiral Matrix Traversal
Spiral matrix traversal is a method of visiting all elements in a matrix by following a spiral path from the outer elements towards the center. This pattern is often used in problems related to image processing, data compression, and even in some game algorithms.
1.1 Understanding the Spiral Pattern
The spiral pattern typically starts from the top-left corner of the matrix and moves in a clockwise direction. The traversal follows these steps:
- Move right along the top row
- Move down along the right column
- Move left along the bottom row
- Move up along the left column
- Repeat steps 1-4 for inner layers until all elements are visited
1.2 Algorithm for Spiral Matrix Traversal
Here’s a step-by-step algorithm to perform spiral matrix traversal:
- Initialize four variables to keep track of the boundaries:
top
,bottom
,left
, andright
- While
top <= bottom
andleft <= right
:- Traverse from left to right along the top row
- Increment
top
- Traverse from top to bottom along the right column
- Decrement
right
- If
top <= bottom
:- Traverse from right to left along the bottom row
- Decrement
bottom
- If
left <= right
:- Traverse from bottom to top along the left column
- Increment
left
1.3 Python Implementation of Spiral Matrix Traversal
Let’s implement the spiral matrix traversal algorithm in Python:
def spiral_traverse(matrix):
if not matrix:
return []
result = []
top, bottom = 0, len(matrix) - 1
left, right = 0, len(matrix[0]) - 1
while top <= bottom and left <= right:
# Traverse top row
for col in range(left, right + 1):
result.append(matrix[top][col])
top += 1
# Traverse right column
for row in range(top, bottom + 1):
result.append(matrix[row][right])
right -= 1
if top <= bottom:
# Traverse bottom row
for col in range(right, left - 1, -1):
result.append(matrix[bottom][col])
bottom -= 1
if left <= right:
# Traverse left column
for row in range(bottom, top - 1, -1):
result.append(matrix[row][left])
left += 1
return result
# Example usage
matrix = [
[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12],
[13, 14, 15, 16]
]
print(spiral_traverse(matrix))
# Output: [1, 2, 3, 4, 8, 12, 16, 15, 14, 13, 9, 5, 6, 7, 11, 10]
This implementation efficiently traverses the matrix in a spiral order, handling both square and rectangular matrices.
2. Diagonal Matrix Traversal
Diagonal matrix traversal involves visiting elements along the diagonals of a matrix. This pattern is useful in various applications, including image processing and certain mathematical computations.
2.1 Understanding the Diagonal Pattern
There are two main types of diagonal traversals:
- Main diagonal traversal: Moving from top-left to bottom-right
- Anti-diagonal traversal: Moving from top-right to bottom-left
We’ll focus on the anti-diagonal traversal, as it’s slightly more challenging and can be easily adapted for main diagonal traversal.
2.2 Algorithm for Diagonal Matrix Traversal
Here’s a step-by-step algorithm for anti-diagonal matrix traversal:
- Start with the top-right element of the matrix
- For each diagonal:
- Initialize row to 0 and column to the current diagonal
- While row is less than the number of rows and column is greater than or equal to 0:
- Visit the element at (row, column)
- Increment row and decrement column
- Repeat step 2 for all diagonals, moving the starting point down the right edge and then along the bottom edge
2.3 Python Implementation of Diagonal Matrix Traversal
Let’s implement the diagonal matrix traversal algorithm in Python:
def diagonal_traverse(matrix):
if not matrix or not matrix[0]:
return []
m, n = len(matrix), len(matrix[0])
result = []
# Traverse the top-right half of the matrix
for d in range(n):
row, col = 0, d
while row < m and col >= 0:
result.append(matrix[row][col])
row += 1
col -= 1
# Traverse the bottom-left half of the matrix
for d in range(1, m):
row, col = d, n - 1
while row < m and col >= 0:
result.append(matrix[row][col])
row += 1
col -= 1
return result
# Example usage
matrix = [
[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12],
[13, 14, 15, 16]
]
print(diagonal_traverse(matrix))
# Output: [4, 3, 8, 2, 7, 12, 1, 6, 11, 16, 5, 10, 15, 9, 14, 13]
This implementation efficiently traverses the matrix diagonally, starting from the top-right corner and moving towards the bottom-left corner.
3. Zigzag Matrix Traversal
Zigzag matrix traversal, also known as wave traversal, involves visiting matrix elements in a zigzag pattern. This traversal method is often used in image processing and data compression algorithms.
3.1 Understanding the Zigzag Pattern
The zigzag pattern typically follows these rules:
- Start from the top-left corner of the matrix
- Move diagonally up and right until reaching the top row or right column
- Move down or right (depending on which boundary was reached)
- Move diagonally down and left until reaching the bottom row or left column
- Move right or down (depending on which boundary was reached)
- Repeat steps 2-5 until all elements are visited
3.2 Algorithm for Zigzag Matrix Traversal
Here’s a step-by-step algorithm for zigzag matrix traversal:
- Initialize variables for row and column (starting at 0, 0)
- While not all elements have been visited:
- Move up and right diagonally while possible:
- Visit the current element
- If at the top row or right column, break
- Move up and right
- Move to the next element (right if possible, else down)
- Move down and left diagonally while possible:
- Visit the current element
- If at the bottom row or left column, break
- Move down and left
- Move to the next element (down if possible, else right)
- Move up and right diagonally while possible:
3.3 Python Implementation of Zigzag Matrix Traversal
Let’s implement the zigzag matrix traversal algorithm in Python:
def zigzag_traverse(matrix):
if not matrix or not matrix[0]:
return []
m, n = len(matrix), len(matrix[0])
result = []
row, col = 0, 0
going_up = True
while len(result) < m * n:
result.append(matrix[row][col])
if going_up:
if row > 0 and col < n - 1:
row -= 1
col += 1
else:
going_up = False
if col == n - 1:
row += 1
else:
col += 1
else:
if row < m - 1 and col > 0:
row += 1
col -= 1
else:
going_up = True
if row == m - 1:
col += 1
else:
row += 1
return result
# Example usage
matrix = [
[1, 2, 3, 4],
[5, 6, 7, 8],
[9, 10, 11, 12],
[13, 14, 15, 16]
]
print(zigzag_traverse(matrix))
# Output: [1, 2, 5, 9, 6, 3, 4, 7, 10, 13, 14, 11, 8, 12, 15, 16]
This implementation efficiently traverses the matrix in a zigzag pattern, handling both square and rectangular matrices.
4. Time and Space Complexity Analysis
Let’s analyze the time and space complexity of each traversal method:
4.1 Spiral Matrix Traversal
- Time Complexity: O(m * n), where m is the number of rows and n is the number of columns. We visit each element exactly once.
- Space Complexity: O(1) if we don’t count the space used to store the result. If we include the result array, it becomes O(m * n).
4.2 Diagonal Matrix Traversal
- Time Complexity: O(m * n), as we visit each element once.
- Space Complexity: O(1) excluding the result array, or O(m * n) including it.
4.3 Zigzag Matrix Traversal
- Time Complexity: O(m * n), since we visit each element once.
- Space Complexity: O(1) not counting the result array, or O(m * n) with it.
All three traversal methods have the same time and space complexity in the worst case. The choice between them depends on the specific problem requirements and the desired output pattern.
5. Practical Applications and Interview Tips
Matrix traversal problems are popular in coding interviews, especially for positions at major tech companies. Here are some tips and applications to keep in mind:
5.1 Applications
- Image Processing: Matrix traversals are used in various image processing algorithms, such as image rotation, compression, and filtering.
- Game Development: In game programming, these traversals can be used for map generation, pathfinding, and implementing game mechanics.
- Data Analysis: When working with 2D data sets, different traversal methods can reveal patterns or help in data transformation.
- Cryptography: Some encryption algorithms use matrix traversals to scramble or unscramble data.
5.2 Interview Tips
- Practice Implementation: Implement each traversal method from scratch multiple times to build muscle memory.
- Understand the Patterns: Be able to visualize and explain each traversal pattern clearly.
- Edge Cases: Always consider edge cases, such as empty matrices or matrices with only one row or column.
- Optimization: Think about ways to optimize your solution, such as reducing the number of boundary checks or improving space efficiency.
- Variations: Be prepared for variations of these problems, such as traversing only the border elements or traversing in a counter-clockwise spiral.
- Real-world Applications: Discuss potential real-world applications of matrix traversals to demonstrate your understanding of their practical use.
6. Conclusion
Matrix traversal techniques are fundamental skills for any programmer, especially those preparing for technical interviews at top tech companies. By mastering spiral, diagonal, and zigzag traversals, you’ll be well-equipped to tackle a wide range of matrix-related problems.
Remember that the key to success in coding interviews is not just knowing the algorithms, but understanding when and how to apply them. Practice these traversal methods regularly, and try to come up with your own variations and applications. With time and effort, you’ll develop the confidence and skills needed to excel in your coding interviews and real-world programming challenges.
Keep exploring, keep coding, and never stop learning. Happy matrix traversing!