Mastering Wix: Fetch – A Comprehensive Guide to Data Retrieval in Velo
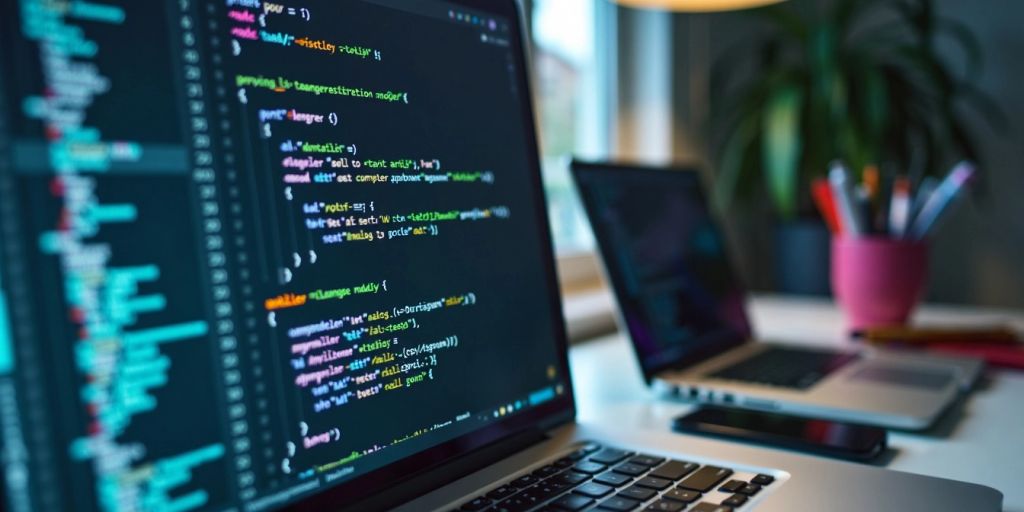
In the world of web development, efficiently retrieving data is crucial for creating dynamic and responsive websites. If you’re building on the Wix platform using Velo (formerly Corvid), understanding how to properly implement fetch operations can significantly enhance your site’s functionality and user experience. This comprehensive guide will walk you through everything you need to know about data retrieval in Velo, from basic concepts to advanced techniques.
Understanding Fetch in Velo
Fetch is a modern API that provides an interface for making HTTP requests to servers, APIs, and other web resources. In Velo, the fetch API allows you to retrieve data from various sources, making it possible to create dynamic content on your Wix site. Before diving into implementation details, let’s understand what makes fetch so powerful:
- It uses Promises, providing a cleaner way to handle asynchronous operations
- It offers more flexibility than older methods like XMLHttpRequest
- It integrates seamlessly with Velo’s JavaScript environment
- It allows for more precise control over request headers and response handling
Basic Fetch Syntax in Velo
The basic syntax for using fetch in Velo is straightforward. Here’s a simple example:
import { fetch } from 'wix-fetch';
export function getDataFromAPI() {
return fetch('https://api.example.com/data')
.then((response) => {
return response.json();
})
.then((json) => {
return json;
})
.catch((error) => {
console.error(error);
});
}
This simple pattern forms the foundation of data retrieval in Velo. Let’s break down what’s happening:
- We import the fetch function from the wix-fetch module
- We call fetch with a URL to retrieve data from
- The first .then() converts the response to JSON format
- The second .then() processes the JSON data
- The .catch() handles any errors that might occur
HTTP Methods with Fetch
While GET is the default method when using fetch, you can specify different HTTP methods to interact with APIs in various ways:
import { fetch } from 'wix-fetch';
// GET request (default)
export function getData() {
return fetch('https://api.example.com/data');
}
// POST request
export function postData(data) {
return fetch('https://api.example.com/data', {
method: 'POST',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
});
}
// PUT request
export function updateData(id, data) {
return fetch(`https://api.example.com/data/${id}`, {
method: 'PUT',
headers: {
'Content-Type': 'application/json'
},
body: JSON.stringify(data)
});
}
// DELETE request
export function deleteData(id) {
return fetch(`https://api.example.com/data/${id}`, {
method: 'DELETE'
});
}
Working with Headers
Headers allow you to send additional information with your HTTP requests. This is particularly important when working with APIs that require authentication or specific content types:
import { fetch } from 'wix-fetch';
export function fetchWithHeaders() {
return fetch('https://api.example.com/secured-data', {
headers: {
'Authorization': 'Bearer YOUR_API_KEY',
'Content-Type': 'application/json',
'Accept': 'application/json',
'Custom-Header': 'custom-value'
}
})
.then(response => response.json());
}
You can also read headers from the response:
import { fetch } from 'wix-fetch';
export function checkResponseHeaders() {
return fetch('https://api.example.com/data')
.then(response => {
// Log all headers
for (let [key, value] of response.headers.entries()) {
console.log(`${key}: ${value}`);
}
// Get a specific header
const contentType = response.headers.get('content-type');
console.log('Content type:', contentType);
return response.json();
});
}
Handling Different Response Types
APIs can return data in various formats. Here’s how to handle different response types:
import { fetch } from 'wix-fetch';
// JSON response (most common)
export function getJsonData() {
return fetch('https://api.example.com/json-data')
.then(response => response.json());
}
// Text response
export function getTextData() {
return fetch('https://api.example.com/text-data')
.then(response => response.text());
}
// Binary data (like images)
export function getBinaryData() {
return fetch('https://api.example.com/binary-data')
.then(response => response.arrayBuffer());
}
// Form data
export function getFormData() {
return fetch('https://api.example.com/form-data')
.then(response => response.formData());
}
Error Handling
Proper error handling is crucial when working with external data sources. Here’s a robust approach to handling errors with fetch:
import { fetch } from 'wix-fetch';
export function fetchWithErrorHandling() {
return fetch('https://api.example.com/data')
.then(response => {
// Check if the response is successful (status code 200-299)
if (!response.ok) {
// Create an error with the status text
throw new Error(`HTTP error! Status: ${response.status} ${response.statusText}`);
}
return response.json();
})
.then(data => {
// Process the data
return {
success: true,
data: data
};
})
.catch(error => {
// Handle network errors or errors thrown from the above code
console.error('Fetch error:', error.message);
return {
success: false,
error: error.message
};
});
}
Timeout and Request Cancellation
Sometimes you need to set timeouts or cancel requests. While the fetch API itself doesn’t directly support timeouts, you can implement them using Promises:
import { fetch } from 'wix-fetch';
export function fetchWithTimeout(url, options = {}, timeout = 5000) {
// Create a promise that rejects after the specified timeout
const timeoutPromise = new Promise((_, reject) => {
setTimeout(() => {
reject(new Error('Request timed out'));
}, timeout);
});
// Race the fetch request against the timeout
return Promise.race([
fetch(url, options),
timeoutPromise
]).then(response => response.json());
}
Using Async/Await with Fetch
The async/await syntax provides a cleaner way to work with Promises, making your fetch code more readable and maintainable:
import { fetch } from 'wix-fetch';
export async function getDataAsync() {
try {
const response = await fetch('https://api.example.com/data');
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
return data;
} catch (error) {
console.error('Error fetching data:', error);
throw error; // Re-throw to allow handling by the caller
}
}
This approach makes the code more linear and easier to follow, especially when dealing with complex data retrieval operations.
Fetching Data from Wix Collections
While the standard fetch API is great for external resources, Velo provides specialized modules for working with Wix Collections. Here’s how to retrieve data from your site’s database:
import wixData from 'wix-data';
export function getItemsFromCollection() {
return wixData.query('MyCollection')
.limit(10)
.find()
.then((results) => {
return results.items;
})
.catch((error) => {
console.error(error);
});
}
You can also use async/await with wixData:
import wixData from 'wix-data';
export async function getItemById(id) {
try {
const item = await wixData.get('MyCollection', id);
return item;
} catch (error) {
console.error('Error retrieving item:', error);
throw error;
}
}
Integrating External APIs with Wix
One of the most powerful uses of fetch in Velo is integrating third-party APIs. Here’s an example of fetching weather data:
import { fetch } from 'wix-fetch';
export function getWeatherData(city) {
const apiKey = 'YOUR_WEATHER_API_KEY';
const url = `https://api.weatherapi.com/v1/current.json?key=${apiKey}&q=${city}`;
return fetch(url)
.then(response => {
if (!response.ok) {
throw new Error(`Weather API error: ${response.status}`);
}
return response.json();
})
.then(data => {
// Extract and format the relevant weather information
return {
location: data.location.name,
country: data.location.country,
temperature: data.current.temp_c,
condition: data.current.condition.text,
humidity: data.current.humidity,
windSpeed: data.current.wind_kph
};
})
.catch(error => {
console.error('Error fetching weather data:', error);
return {
error: true,
message: error.message
};
});
}
Handling CORS Issues
Cross-Origin Resource Sharing (CORS) can sometimes cause issues when fetching data from external APIs. If you encounter CORS errors, you have several options:
- Use APIs that support CORS
- Create a Wix HTTP Function to act as a proxy
- Use a third-party CORS proxy (though this isn’t recommended for production sites)
Here’s an example of creating a Wix HTTP Function to bypass CORS restrictions:
// In the backend/http-functions.js file
import { fetch } from 'wix-fetch';
import { ok, serverError } from 'wix-http-functions';
export async function get_fetchExternalAPI(request) {
const url = request.query.url;
try {
const response = await fetch(url);
const data = await response.json();
return ok({
headers: {
'Content-Type': 'application/json'
},
body: data
});
} catch (error) {
return serverError({
body: {
error: error.message
}
});
}
}
// In your frontend code
import { fetch } from 'wix-fetch';
export function getExternalData() {
const apiUrl = encodeURIComponent('https://api.example.com/data');
return fetch(`/_functions/fetchExternalAPI?url=${apiUrl}`)
.then(response => response.json());
}
Caching Fetch Results
To improve performance and reduce unnecessary API calls, you can implement a simple caching mechanism:
import { fetch } from 'wix-fetch';
import { session } from 'wix-storage';
export async function fetchWithCache(url, cacheKey, cacheTimeInMinutes = 10) {
// Check if we have cached data
const cachedData = session.getItem(cacheKey);
if (cachedData) {
const { timestamp, data } = JSON.parse(cachedData);
const cacheExpiryTime = timestamp + (cacheTimeInMinutes * 60 * 1000);
// If the cache hasn't expired, return the cached data
if (Date.now() < cacheExpiryTime) {
console.log('Returning cached data for:', url);
return data;
}
}
// If no valid cache exists, fetch fresh data
try {
const response = await fetch(url);
const data = await response.json();
// Store in cache with current timestamp
const cacheObject = {
timestamp: Date.now(),
data: data
};
session.setItem(cacheKey, JSON.stringify(cacheObject));
return data;
} catch (error) {
console.error('Error fetching data:', error);
throw error;
}
}
Batching Multiple Fetch Requests
Sometimes you need to fetch data from multiple sources. Promise.all is perfect for this scenario:
import { fetch } from 'wix-fetch';
export function batchFetchData() {
const urls = [
'https://api.example.com/products',
'https://api.example.com/categories',
'https://api.example.com/users'
];
const fetchPromises = urls.map(url =>
fetch(url)
.then(response => {
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
return response.json();
})
);
return Promise.all(fetchPromises)
.then(([products, categories, users]) => {
// Now you have all three datasets
return {
products,
categories,
users
};
})
.catch(error => {
console.error('Error in batch fetch:', error);
throw error;
});
}
Sequential Fetch Operations
In some cases, you might need to perform sequential fetches where each request depends on the result of the previous one:
import { fetch } from 'wix-fetch';
export async function sequentialFetch() {
try {
// First fetch to get a user
const userResponse = await fetch('https://api.example.com/users/current');
const user = await userResponse.json();
// Second fetch using the user's ID
const ordersResponse = await fetch(`https://api.example.com/orders?userId=${user.id}`);
const orders = await ordersResponse.json();
// Third fetch using an order ID from the previous result
const latestOrderId = orders[0]?.id;
if (latestOrderId) {
const orderDetailsResponse = await fetch(`https://api.example.com/orders/${latestOrderId}/details`);
const orderDetails = await orderDetailsResponse.json();
return {
user,
orders,
latestOrderDetails: orderDetails
};
}
return {
user,
orders,
latestOrderDetails: null
};
} catch (error) {
console.error('Error in sequential fetch:', error);
throw error;
}
}
Real-time Data with Fetch and Intervals
For creating real-time or near-real-time updates on your Wix site, you can combine fetch with setInterval:
import { fetch } from 'wix-fetch';
let intervalId;
// Start polling an API
export function startRealTimeUpdates(callback, intervalMs = 5000) {
// Clear any existing interval
if (intervalId) {
clearInterval(intervalId);
}
// Function to fetch the latest data
const fetchLatestData = () => {
fetch('https://api.example.com/live-data')
.then(response => response.json())
.then(data => {
// Pass the data to the callback function
callback(data);
})
.catch(error => {
console.error('Error fetching real-time data:', error);
});
};
// Fetch immediately
fetchLatestData();
// Then set up the interval
intervalId = setInterval(fetchLatestData, intervalMs);
return intervalId;
}
// Stop polling
export function stopRealTimeUpdates() {
if (intervalId) {
clearInterval(intervalId);
intervalId = null;
return true;
}
return false;
}
Integrating Fetch with Wix UI Components
A common use case is to populate Wix UI components with data from an API. Here's how to fetch data and display it in a repeater:
import { fetch } from 'wix-fetch';
import wixWindow from 'wix-window';
$w.onReady(function () {
loadProductData();
});
function loadProductData() {
$w('#loadingIndicator').show();
$w('#errorMessage').hide();
fetch('https://api.example.com/products')
.then(response => {
if (!response.ok) {
throw new Error('Failed to load products');
}
return response.json();
})
.then(products => {
// Set the repeater data
$w('#productsRepeater').data = products;
// Set up the repeater item event
$w('#productsRepeater').onItemReady(($item, product) => {
$item('#productName').text = product.name;
$item('#productPrice').text = `$${product.price.toFixed(2)}`;
$item('#productImage').src = product.imageUrl;
$item('#viewButton').onClick(() => {
wixWindow.openLightbox('ProductDetails', { productId: product.id });
});
});
$w('#loadingIndicator').hide();
})
.catch(error => {
console.error('Error loading products:', error);
$w('#loadingIndicator').hide();
$w('#errorMessage').show();
$w('#errorMessage').text = 'Failed to load products. Please try again later.';
});
}
Implementing Search with Fetch
You can create dynamic search functionality using fetch to query an API based on user input:
import { fetch } from 'wix-fetch';
import { debounce } from 'lodash';
$w.onReady(function () {
// Set up the search input with debounce to prevent too many requests
const debouncedSearch = debounce(searchProducts, 300);
$w('#searchInput').onChange(() => {
debouncedSearch($w('#searchInput').value);
});
});
function searchProducts(query) {
if (!query || query.length < 2) {
// Clear results if query is too short
$w('#searchResults').data = [];
$w('#noResultsMessage').hide();
return;
}
$w('#searchSpinner').show();
$w('#noResultsMessage').hide();
fetch(`https://api.example.com/products/search?q=${encodeURIComponent(query)}`)
.then(response => response.json())
.then(results => {
$w('#searchSpinner').hide();
if (results.length === 0) {
$w('#noResultsMessage').show();
} else {
$w('#searchResults').data = results;
}
})
.catch(error => {
console.error('Search error:', error);
$w('#searchSpinner').hide();
$w('#noResultsMessage').text = 'An error occurred during search';
$w('#noResultsMessage').show();
});
}
Uploading Files with Fetch
Fetch can also be used to upload files to external services:
import { fetch } from 'wix-fetch';
export function uploadFile(file, uploadUrl) {
const formData = new FormData();
formData.append('file', file);
return fetch(uploadUrl, {
method: 'POST',
body: formData
})
.then(response => {
if (!response.ok) {
throw new Error(`Upload failed with status: ${response.status}`);
}
return response.json();
})
.then(data => {
// Return the information about the uploaded file
return {
success: true,
fileUrl: data.fileUrl,
fileId: data.fileId
};
})
.catch(error => {
console.error('File upload error:', error);
return {
success: false,
error: error.message
};
});
}
Security Considerations
When working with fetch in Velo, keep these security considerations in mind:
- Never expose API keys in frontend code. Use backend HTTP functions for sensitive operations.
- Validate and sanitize all user inputs before sending them in fetch requests.
- Use HTTPS URLs exclusively for fetch operations.
- Implement proper error handling to prevent exposing sensitive information.
- Be cautious about which third-party APIs you integrate with.
Here's an example of secure API key usage with a backend function:
// In backend/http-functions.js
import { fetch } from 'wix-fetch';
import { ok, serverError } from 'wix-http-functions';
// API key stored securely on the backend
const API_KEY = 'your_secret_api_key';
export async function get_secureApiCall(request) {
const query = request.query.q;
try {
const response = await fetch(`https://api.example.com/data?q=${encodeURIComponent(query)}&key=${API_KEY}`);
const data = await response.json();
return ok({
headers: {
'Content-Type': 'application/json'
},
body: data
});
} catch (error) {
console.error('Secure API call error:', error);
return serverError({
body: {
error: 'An error occurred while processing your request'
}
});
}
}
// In frontend code
import { fetch } from 'wix-fetch';
export function searchSecurely(query) {
return fetch(`/_functions/secureApiCall?q=${encodeURIComponent(query)}`)
.then(response => response.json());
}
Performance Optimization
To ensure your fetch operations are as efficient as possible, consider these optimization techniques:
- Implement caching for frequently accessed data
- Use pagination when fetching large datasets
- Limit the fields returned by APIs when possible
- Implement request throttling and debouncing for user-initiated fetches
- Consider using IndexedDB for client-side storage of larger datasets
Debugging Fetch Operations
When your fetch operations aren't working as expected, try these debugging approaches:
import { fetch } from 'wix-fetch';
export function debugFetch(url) {
console.log('Starting fetch request to:', url);
return fetch(url)
.then(response => {
console.log('Response status:', response.status);
console.log('Response headers:', Object.fromEntries(response.headers.entries()));
return response.text(); // Get raw text first for debugging
})
.then(text => {
console.log('Raw response body:', text);
// Try to parse as JSON if applicable
try {
return JSON.parse(text);
} catch (e) {
console.log('Response is not valid JSON');
return text;
}
})
.catch(error => {
console.error('Fetch error:', error);
throw error;
});
}
Best Practices for Fetch in Velo
To wrap up, here are some best practices to follow when using fetch in your Velo projects:
- Always handle errors - Never leave fetch operations without proper error handling.
- Use async/await - It makes your code more readable and easier to maintain.
- Cache responsibly - Implement caching for frequently accessed data that doesn't change often.
- Secure sensitive operations - Use backend HTTP functions for operations requiring API keys or authentication.
- Optimize payload size - Only fetch the data you need, using query parameters to filter server-side when possible.
- Implement loading states - Always show users when data is being loaded or when errors occur.
- Use meaningful function names - Name your fetch functions based on what they retrieve, not how they retrieve it.
- Test with realistic conditions - Test your fetch operations with network throttling to simulate mobile conditions.
Conclusion
Mastering fetch in Velo opens up endless possibilities for creating dynamic, data-driven Wix websites. From basic GET requests to complex data orchestration, the techniques covered in this guide provide you with the tools to elevate your Wix site's functionality.
Remember that effective data retrieval is not just about getting data—it's about getting the right data at the right time, in the right format, while maintaining performance and security. By following the patterns and practices outlined here, you'll be well-equipped to implement sophisticated data retrieval operations in your Velo projects.
Whether you're building a simple blog, an e-commerce site, or a complex web application on Wix, these fetch techniques will help you create more dynamic, responsive, and user-friendly experiences for your visitors.