Mastering Whiteboard Interviews: Best Practices for Success
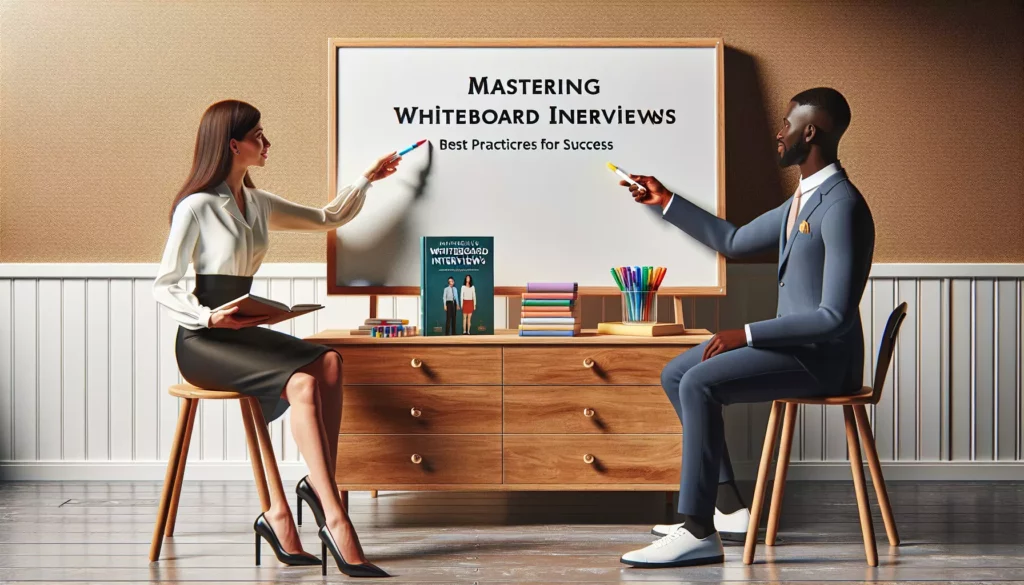
Whiteboard interviews have become a staple in the tech industry, especially for software engineering positions. These interviews can be intimidating, but with the right preparation and mindset, you can excel and showcase your problem-solving skills. In this comprehensive guide, we’ll explore the best practices for whiteboard interviews, helping you navigate this crucial step in your journey to landing your dream job at top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google).
Understanding Whiteboard Interviews
Before diving into the best practices, it’s essential to understand what whiteboard interviews are and why companies use them. Whiteboard interviews are technical interviews where candidates are asked to solve coding problems or design systems on a whiteboard. The primary goal is to assess a candidate’s problem-solving skills, algorithmic thinking, and ability to communicate technical concepts effectively.
These interviews typically involve the following elements:
- Problem presentation by the interviewer
- Clarification of requirements and constraints
- Brainstorming and discussing potential solutions
- Writing code or designing systems on a whiteboard
- Explaining your thought process and approach
- Analyzing time and space complexity
- Answering follow-up questions and discussing optimizations
Best Practices for Whiteboard Interviews
1. Practice, Practice, Practice
The old adage “practice makes perfect” holds true for whiteboard interviews. Regular practice is crucial to building confidence and improving your problem-solving skills. Here are some ways to practice effectively:
- Solve coding problems on platforms like AlgoCademy, LeetCode, or HackerRank
- Participate in mock interviews with friends or mentors
- Join coding communities and attend coding meetups
- Use a physical whiteboard or digital whiteboard tool to simulate the interview environment
Remember, the goal is not just to solve problems but to improve your ability to explain your thought process clearly and concisely.
2. Master the Problem-Solving Framework
Having a structured approach to problem-solving can help you stay organized and confident during the interview. Follow this framework:
- Understand the problem: Carefully read or listen to the problem statement. Ask clarifying questions to ensure you fully understand the requirements and constraints.
- Analyze the input and output: Identify the expected input format and the desired output. Consider edge cases and potential limitations.
- Brainstorm solutions: Think of multiple approaches to solve the problem. Consider trade-offs between different solutions.
- Choose an approach: Select the most appropriate solution based on time complexity, space complexity, and any specific requirements mentioned by the interviewer.
- Plan your solution: Outline the steps of your chosen approach before writing any code. This helps organize your thoughts and catch potential issues early.
- Implement the solution: Write clean, readable code on the whiteboard. Use proper indentation and follow coding best practices.
- Test and debug: Walk through your code with sample inputs, including edge cases. Identify and fix any bugs or logical errors.
- Analyze time and space complexity: Discuss the efficiency of your solution in terms of Big O notation.
- Optimize (if necessary): If time allows, discuss potential optimizations or alternative approaches.
3. Communicate Effectively
Clear communication is crucial in whiteboard interviews. Here are some tips to improve your communication:
- Think out loud: Share your thought process as you work through the problem.
- Use proper terminology: Demonstrate your technical knowledge by using appropriate programming terms and concepts.
- Ask for feedback: Check with the interviewer if your approach makes sense before diving into implementation.
- Be open to hints: If you’re stuck, don’t be afraid to ask for hints or clarification.
- Explain your code: As you write code, explain what each section does and why you’re making certain decisions.
4. Manage Your Time Wisely
Time management is crucial in whiteboard interviews. Here’s how to make the most of your time:
- Ask about time constraints at the beginning of the interview
- Allocate time for each step of the problem-solving process
- If you’re spending too much time on one aspect, consider moving on and coming back to it later
- Practice solving problems within time limits to improve your pacing
5. Write Clean and Readable Code
Even though you’re writing on a whiteboard, it’s essential to maintain good coding practices:
- Use consistent indentation and spacing
- Write legibly and organize your code neatly
- Use meaningful variable and function names
- Include comments to explain complex logic or assumptions
- Follow the coding style conventions of the language you’re using
6. Handle Edge Cases and Error Scenarios
Demonstrating your ability to consider and handle edge cases is a key aspect of whiteboard interviews:
- Identify potential edge cases (e.g., empty inputs, negative numbers, extremely large values)
- Discuss how your solution handles these cases
- Implement error checking and exception handling where appropriate
- Show that you’re thinking about the robustness of your solution
7. Analyze Time and Space Complexity
Understanding and articulating the efficiency of your solution is crucial:
- Analyze the time complexity of your algorithm using Big O notation
- Discuss the space complexity, including any additional data structures used
- Be prepared to explain trade-offs between time and space complexity
- If asked, suggest potential optimizations or alternative approaches with different complexity characteristics
8. Stay Calm and Positive
Maintaining a positive attitude can significantly impact your performance:
- Take deep breaths and stay relaxed
- If you make a mistake, acknowledge it and move on
- View challenging questions as opportunities to showcase your problem-solving skills
- Remember that the interviewer wants to see how you think and approach problems, not just the final solution
9. Use Pseudocode When Appropriate
Sometimes, using pseudocode can be beneficial:
- Use pseudocode to outline your approach before writing actual code
- If you’re unsure about specific syntax, write pseudocode and explain that you’d look up the exact implementation
- Pseudocode can help you communicate your ideas more quickly and focus on the overall logic
10. Practice Common Algorithms and Data Structures
Familiarize yourself with frequently used algorithms and data structures:
- Sorting algorithms (e.g., quicksort, mergesort)
- Searching algorithms (e.g., binary search)
- Graph algorithms (e.g., DFS, BFS, Dijkstra’s)
- Dynamic programming
- Common data structures (e.g., arrays, linked lists, trees, hash tables, heaps)
11. Learn to Optimize Your Solutions
Being able to optimize your initial solution demonstrates advanced problem-solving skills:
- Start with a brute-force approach if necessary, but always look for ways to improve
- Consider using appropriate data structures to optimize time or space complexity
- Look for opportunities to use techniques like memoization or dynamic programming
- Discuss trade-offs between different optimizations
12. Prepare for System Design Questions
For more senior positions, system design questions are common in whiteboard interviews:
- Study common system design patterns and architectures
- Practice designing scalable systems on a whiteboard
- Be prepared to discuss trade-offs in system design decisions
- Understand concepts like load balancing, caching, and database sharding
13. Use the Whiteboard Effectively
Make the most of the whiteboard space:
- Organize your work neatly on the whiteboard
- Use different colors if available to highlight important parts
- Leave space for potential additions or modifications
- Erase and rewrite if necessary to keep your work clean and readable
14. Ask Thoughtful Questions
Demonstrate your interest and engagement by asking relevant questions:
- Inquire about specific requirements or constraints not mentioned in the problem statement
- Ask about the scale of the problem (e.g., size of input data) if it affects your approach
- If time allows, ask about the real-world applications of the problem
15. Follow Up and Reflect
After the interview:
- Send a thank-you note to your interviewer
- Reflect on your performance and identify areas for improvement
- If you didn’t get the job, ask for feedback to help you prepare for future interviews
Common Whiteboard Interview Questions
To help you prepare, here are some common types of questions you might encounter in a whiteboard interview:
- String manipulation (e.g., reversing a string, finding palindromes)
- Array operations (e.g., finding duplicates, merging sorted arrays)
- Linked list problems (e.g., detecting cycles, reversing a linked list)
- Tree and graph traversals (e.g., finding the lowest common ancestor, implementing BFS/DFS)
- Dynamic programming problems (e.g., longest common subsequence, knapsack problem)
- Sorting and searching algorithms (e.g., implementing quicksort, binary search in a rotated array)
- Hash table implementations and applications
- Stack and queue problems
- Bit manipulation questions
- System design problems (e.g., designing a URL shortener, designing a social media feed)
Example Whiteboard Interview Question
Let’s walk through an example of how to approach a whiteboard interview question using the best practices we’ve discussed:
Problem: Given an array of integers, find two numbers such that they add up to a specific target number.
Approach:
- Understand the problem: We need to find two numbers in an array that sum up to a given target.
- Analyze input/output: Input is an array of integers and a target sum. Output is the indices of the two numbers.
- Brainstorm solutions: We could use a brute-force approach (nested loops) or use a hash table for optimization.
- Choose an approach: Let’s use the hash table approach for better time complexity.
- Plan the solution: We’ll iterate through the array once, using a hash table to store complements.
- Implement the solution:
def two_sum(nums, target):
complement_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in complement_dict:
return [complement_dict[complement], i]
complement_dict[num] = i
return [] # No solution found
- Test and debug: Let’s walk through an example: nums = [2, 7, 11, 15], target = 9
- Analyze complexity: Time complexity is O(n), space complexity is O(n) due to the hash table.
- Optimize: This solution is already optimized for time complexity. We could potentially optimize for space in specific scenarios.
Conclusion
Mastering whiteboard interviews takes time and practice, but by following these best practices, you’ll be well-prepared to showcase your problem-solving skills and technical knowledge. Remember that the interview is not just about finding the correct solution, but also about demonstrating your thought process, communication skills, and ability to work through challenging problems.
As you prepare for your interviews, take advantage of resources like AlgoCademy, which offers interactive coding tutorials and AI-powered assistance to help you progress from beginner-level coding to interview-ready skills. With consistent practice and the right mindset, you’ll be well on your way to acing your whiteboard interviews and landing your dream job at top tech companies.
Good luck with your interview preparation, and remember that each interview, regardless of the outcome, is an opportunity to learn and grow as a developer!