Mastering Whiteboard Interviews: A Comprehensive Guide to Preparation and Success
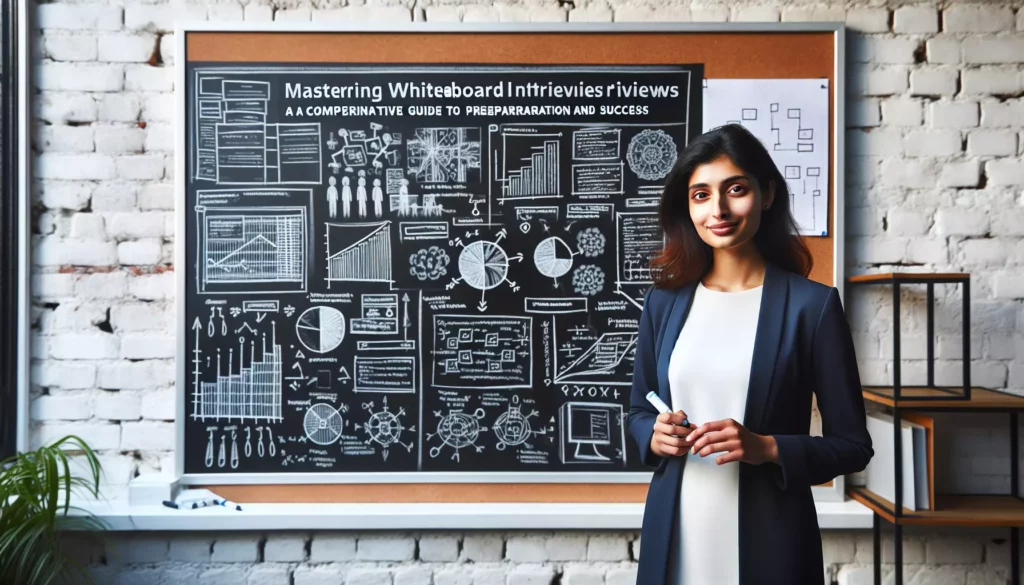
In the competitive world of tech hiring, whiteboard interviews have become a staple of the recruitment process, especially for software engineering positions. These interviews can be intimidating, but with the right preparation and mindset, you can excel and showcase your problem-solving skills effectively. In this comprehensive guide, we’ll explore what whiteboard interviews are, why companies use them, and most importantly, how you can prepare to ace them.
What Are Whiteboard Interviews?
Whiteboard interviews, also known as coding interviews or technical interviews, are a type of job interview where candidates are asked to solve programming problems or design systems on a whiteboard. These interviews typically involve the following elements:
- A problem or scenario presented by the interviewer
- A whiteboard or similar writing surface
- An expectation for the candidate to think aloud and explain their thought process
- Interaction with the interviewer, including answering questions and receiving hints
- Writing code or drawing diagrams to illustrate solutions
The primary goal of whiteboard interviews is to assess a candidate’s problem-solving skills, coding ability, and communication in a high-pressure situation. While they have been a subject of debate in the tech industry, many companies, especially larger tech firms often referred to as FAANG (Facebook, Amazon, Apple, Netflix, Google), continue to use them as part of their hiring process.
Why Do Companies Use Whiteboard Interviews?
Companies employ whiteboard interviews for several reasons:
- Problem-solving assessment: They allow interviewers to observe how candidates approach and solve complex problems in real-time.
- Communication skills: Candidates must explain their thought process clearly, demonstrating their ability to collaborate and convey technical concepts.
- Coding proficiency: While not always focused on syntactically perfect code, these interviews reveal a candidate’s understanding of programming concepts and algorithms.
- Stress management: The high-pressure environment tests how candidates perform under stress, a common situation in many tech roles.
- Adaptability: Interviewers can adjust the difficulty or add constraints, assessing how candidates adapt to changing requirements.
How to Prepare for Whiteboard Interviews
Preparing for whiteboard interviews requires a multifaceted approach. Here are key strategies to help you succeed:
1. Master the Fundamentals
Before diving into complex algorithms, ensure you have a solid grasp of fundamental computer science concepts:
- Data structures (arrays, linked lists, trees, graphs, hash tables)
- Basic algorithms (sorting, searching, traversal)
- Time and space complexity analysis
- Object-oriented programming principles
- System design basics
Resources like AlgoCademy offer interactive coding tutorials and problem sets that can help you reinforce these fundamentals in a practical, hands-on way.
2. Practice Problem-Solving
Regular practice is crucial for improving your problem-solving skills:
- Solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal
- Participate in coding competitions or hackathons
- Work through problems in interview preparation books
- Use AlgoCademy’s AI-powered assistance to guide you through complex problems
Aim to solve a variety of problems, focusing on different data structures and algorithms. Start with easier problems and gradually increase the difficulty as you improve.
3. Learn to Communicate Your Thought Process
A crucial aspect of whiteboard interviews is explaining your approach:
- Practice thinking aloud while solving problems
- Explain your reasoning for choosing specific approaches
- Ask clarifying questions about the problem
- Discuss trade-offs between different solutions
Consider recording yourself or practicing with a friend to get comfortable verbalizing your thoughts.
4. Develop a Problem-Solving Framework
Having a structured approach to problem-solving can help you stay organized during the interview. Here’s a sample framework:
- Understand the problem: Ask questions, clarify requirements
- Identify inputs and outputs
- Consider edge cases and constraints
- Brainstorm potential approaches
- Choose an approach and explain your reasoning
- Write pseudocode or outline the solution
- Implement the solution
- Test and optimize
Practice applying this framework to various problems to make it second nature.
5. Improve Your Coding Speed and Accuracy
While whiteboard interviews don’t require perfect syntax, being able to write code quickly and accurately is beneficial:
- Practice coding without an IDE to simulate whiteboard conditions
- Focus on writing clean, readable code
- Learn common coding patterns and techniques
- Use platforms like AlgoCademy that offer timed coding challenges
6. Study System Design
For more senior positions, system design questions are common in whiteboard interviews:
- Learn about distributed systems, scalability, and reliability
- Practice designing high-level architectures for popular services (e.g., Twitter, Netflix)
- Understand trade-offs in system design decisions
7. Mock Interviews
Simulating the interview experience is invaluable:
- Ask friends or colleagues to conduct mock interviews
- Use online platforms that offer mock interview services
- Record your mock interviews to review and improve
Pay attention to your body language, tone, and how you handle pressure during these practice sessions.
Common Whiteboard Interview Mistakes to Avoid
Being aware of common pitfalls can help you navigate whiteboard interviews more successfully:
- Diving into coding too quickly: Take time to understand the problem and plan your approach.
- Staying silent: Remember to communicate your thoughts throughout the process.
- Ignoring edge cases: Always consider and discuss potential edge cases in your solution.
- Not asking for clarification: Don’t hesitate to ask questions if something is unclear.
- Getting stuck without making progress: If you’re stuck, explain your thought process and ask for hints.
- Writing messy or illegible code: Practice writing clearly on a whiteboard or similar surface.
- Failing to test your solution: Always walk through your solution with test cases.
Whiteboard Interview Tips and Tricks
Here are some additional tips to help you excel in your whiteboard interview:
- Arrive early and calm your nerves: Give yourself time to relax and focus before the interview.
- Bring your own markers: Having reliable tools can boost your confidence.
- Use the entire whiteboard: Organize your work spatially to keep your thoughts clear.
- Write legibly: Clear handwriting helps both you and the interviewer follow your work.
- Use pseudocode when appropriate: Sometimes, high-level logic is more important than perfect syntax.
- Draw diagrams: Visual representations can clarify your thoughts and impress interviewers.
- Think aloud, but thoughtfully: Balance explanation with focused problem-solving.
- Be open to hints: View interviewer suggestions as collaborative problem-solving, not as failures.
- Show enthusiasm: Demonstrate your passion for problem-solving and technology.
- Practice good time management: Allocate your time wisely between understanding, planning, coding, and testing.
Sample Whiteboard Interview Problem
To give you a taste of what to expect, here’s a sample whiteboard interview problem:
Problem: Given a binary tree, write a function to check if it is a valid binary search tree (BST).
Here’s how you might approach this problem in a whiteboard interview:
- Clarify the problem:
- Confirm the definition of a BST (all left subtree values are less than the node, all right subtree values are greater)
- Ask about the data structure for the tree nodes
- Inquire about handling duplicate values
- Discuss approach:
- Explain that we can use an in-order traversal, checking if each node’s value is greater than the previous
- Alternatively, describe a recursive approach checking each node against valid ranges
- Write pseudocode:
function isValidBST(root): return checkBST(root, -infinity, +infinity) function checkBST(node, min, max): if node is null: return true if node.val <= min or node.val >= max: return false return checkBST(node.left, min, node.val) and checkBST(node.right, node.val, max)
- Implement the solution:
class TreeNode: def __init__(self, val=0, left=None, right=None): self.val = val self.left = left self.right = right def isValidBST(root: TreeNode) -> bool: def validate(node, low=-float('inf'), high=float('inf')): if not node: return True if node.val <= low or node.val >= high: return False return (validate(node.left, low, node.val) and validate(node.right, node.val, high)) return validate(root)
- Test the solution:
- Walk through the code with a simple valid BST
- Test with an invalid BST
- Consider edge cases like an empty tree or a tree with one node
- Analyze time and space complexity:
- Time complexity: O(n) where n is the number of nodes (we visit each node once)
- Space complexity: O(h) where h is the height of the tree (due to recursion stack)
Remember, the key is not just to solve the problem, but to communicate your thought process clearly throughout the interview.
Conclusion
Whiteboard interviews can be challenging, but with proper preparation and practice, you can approach them with confidence. Focus on strengthening your problem-solving skills, practicing clear communication, and developing a structured approach to tackling complex problems. Utilize resources like AlgoCademy to hone your skills through interactive tutorials and AI-assisted problem-solving.
Remember that the goal of these interviews is not just to arrive at the correct solution, but to demonstrate your thought process, problem-solving abilities, and communication skills. Embrace the challenge, stay calm under pressure, and view each interview as an opportunity to learn and improve.
By following the strategies outlined in this guide and consistently practicing, you’ll be well-prepared to tackle whiteboard interviews and showcase your skills effectively. Good luck with your interview preparation!