Mastering Water Container Problems: Techniques and Strategies for Efficient Solutions
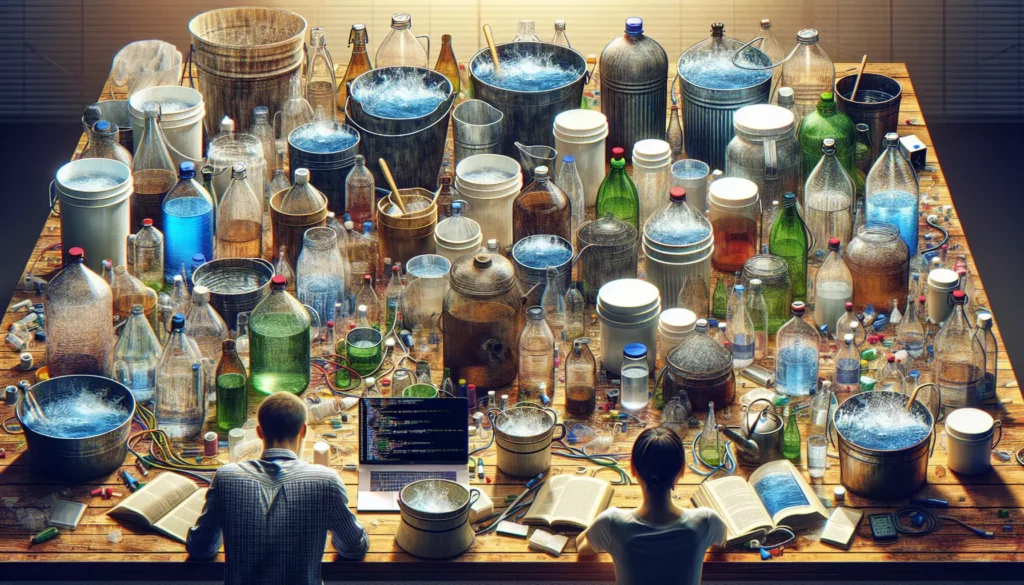
Water container problems are a classic category of algorithmic challenges that frequently appear in coding interviews, particularly for positions at top tech companies. These problems test a programmer’s ability to think critically, optimize algorithms, and implement efficient solutions. In this comprehensive guide, we’ll explore various techniques for solving water container problems, providing you with the tools and knowledge to tackle these challenges confidently.
Understanding Water Container Problems
Before diving into specific techniques, it’s essential to understand what water container problems are and why they’re important in the context of coding interviews and algorithmic thinking.
What are Water Container Problems?
Water container problems typically involve scenarios where you need to calculate the amount of water that can be trapped or contained within a structure. These structures are often represented as arrays of integers, where each integer represents the height of a “wall” or “elevation” at that position.
Common variations of water container problems include:
- Trapping Rain Water: Calculate the amount of water that can be trapped between walls of varying heights.
- Container With Most Water: Find the maximum amount of water that can be contained between two walls.
- Pour Water: Simulate the flow of water over a terrain and determine its final resting place.
Why are Water Container Problems Important?
Water container problems are valuable in coding interviews and algorithmic practice for several reasons:
- Problem-solving skills: They require creative thinking and the ability to visualize and manipulate data structures.
- Optimization: Many water container problems have both brute force and optimized solutions, allowing interviewers to assess a candidate’s ability to improve algorithm efficiency.
- Real-world applications: The concepts behind these problems can be applied to various real-world scenarios, such as analyzing terrain for flood risk or optimizing container storage in logistics.
- Multiple approach possibilities: These problems often have several valid solution approaches, showcasing a programmer’s versatility and understanding of different algorithmic techniques.
Technique 1: Two-Pointer Approach
The two-pointer approach is a powerful technique for solving many water container problems efficiently. This method involves using two pointers that move towards each other from opposite ends of the array.
How it Works
- Initialize two pointers, one at the start of the array (left) and one at the end (right).
- Compare the heights at the left and right pointers.
- Move the pointer with the smaller height towards the center.
- Update the maximum water amount if applicable.
- Repeat steps 2-4 until the pointers meet.
Example: Container With Most Water
Let’s apply the two-pointer technique to the “Container With Most Water” problem:
class Solution {
public:
int maxArea(vector<int>& height) {
int left = 0;
int right = height.size() - 1;
int maxWater = 0;
while (left < right) {
int width = right - left;
int containerHeight = min(height[left], height[right]);
int water = width * containerHeight;
maxWater = max(maxWater, water);
if (height[left] < height[right]) {
left++;
} else {
right--;
}
}
return maxWater;
}
};
This solution has a time complexity of O(n) and a space complexity of O(1), making it highly efficient for large inputs.
When to Use the Two-Pointer Approach
The two-pointer technique is particularly useful when:
- You’re dealing with a sorted or partially sorted array.
- You need to find a pair of elements that satisfy certain conditions.
- You want to optimize space complexity by avoiding additional data structures.
Technique 2: Dynamic Programming
Dynamic programming is a powerful method for solving complex problems by breaking them down into simpler subproblems. It’s particularly useful for water container problems that involve calculating cumulative effects or optimizing over a range of possibilities.
How it Works
- Identify the subproblems and define the recurrence relation.
- Create arrays to store the solutions to subproblems.
- Fill the arrays in a bottom-up manner, solving smaller subproblems first.
- Use the stored results to solve larger subproblems efficiently.
Example: Trapping Rain Water
Let’s apply dynamic programming to the “Trapping Rain Water” problem:
class Solution {
public:
int trap(vector<int>& height) {
int n = height.size();
if (n <= 2) return 0;
vector<int> leftMax(n), rightMax(n);
leftMax[0] = height[0];
rightMax[n-1] = height[n-1];
for (int i = 1; i < n; i++) {
leftMax[i] = max(leftMax[i-1], height[i]);
}
for (int i = n-2; i >= 0; i--) {
rightMax[i] = max(rightMax[i+1], height[i]);
}
int water = 0;
for (int i = 0; i < n; i++) {
water += min(leftMax[i], rightMax[i]) - height[i];
}
return water;
}
};
This solution has a time complexity of O(n) and a space complexity of O(n), where n is the number of elements in the height array.
When to Use Dynamic Programming
Dynamic programming is particularly effective when:
- The problem exhibits overlapping subproblems and optimal substructure.
- You need to find an optimal solution among many possible solutions.
- The problem involves making a series of interconnected decisions.
Technique 3: Stack-based Approach
A stack-based approach can be incredibly useful for certain water container problems, especially those that involve tracking heights and calculating areas or volumes.
How it Works
- Initialize an empty stack to store indices or heights.
- Iterate through the array, pushing elements onto the stack.
- When a condition is met (e.g., a higher wall is found), pop elements from the stack and perform calculations.
- Continue until all elements have been processed.
Example: Trapping Rain Water (Stack Solution)
Here’s how we can solve the “Trapping Rain Water” problem using a stack:
class Solution {
public:
int trap(vector<int>& height) {
stack<int> s;
int water = 0;
int current = 0;
while (current < height.size()) {
while (!s.empty() && height[current] > height[s.top()]) {
int top = s.top();
s.pop();
if (s.empty()) {
break;
}
int distance = current - s.top() - 1;
int boundedHeight = min(height[current], height[s.top()]) - height[top];
water += distance * boundedHeight;
}
s.push(current++);
}
return water;
}
};
This solution has a time complexity of O(n) and a space complexity of O(n) in the worst case, where n is the number of elements in the height array.
When to Use the Stack-based Approach
The stack-based approach is particularly useful when:
- You need to keep track of a monotonic sequence of elements.
- The problem involves finding the next greater or smaller element.
- You need to calculate areas or volumes between elements.
Technique 4: Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of finding a global optimum. While not always guaranteed to find the best solution, greedy approaches can be very efficient for certain water container problems.
How it Works
- Identify the greedy choice at each step.
- Prove that a greedy choice is always safe.
- Demonstrate that by making greedy choices, you can arrive at the optimal solution.
Example: Container With Most Water (Greedy Approach)
Let’s revisit the “Container With Most Water” problem with a greedy approach:
class Solution {
public:
int maxArea(vector<int>& height) {
int left = 0, right = height.size() - 1;
int maxWater = 0;
while (left < right) {
int water = min(height[left], height[right]) * (right - left);
maxWater = max(maxWater, water);
if (height[left] < height[right]) {
left++;
} else {
right--;
}
}
return maxWater;
}
};
This greedy solution has a time complexity of O(n) and a space complexity of O(1), making it highly efficient.
When to Use Greedy Algorithms
Greedy algorithms are particularly effective when:
- The problem has optimal substructure (an optimal solution can be constructed from optimal solutions of its subproblems).
- The problem has the greedy choice property (a locally optimal choice leads to a globally optimal solution).
- You need a simple and efficient algorithm, even if it might not always produce the absolute best result.
Technique 5: Sliding Window
The sliding window technique is a powerful approach for solving problems that involve subarrays or subsequences. While not as commonly used for traditional water container problems, it can be applied to certain variations or related challenges.
How it Works
- Define a window with a start and end pointer.
- Expand or contract the window based on certain conditions.
- Process the elements within the window.
- Update the result as needed.
Example: Maximum Water Container in a Fixed-Size Window
Let’s consider a variation where we need to find the maximum water container within a fixed-size window:
class Solution {
public:
int maxWaterInWindow(vector<int>& height, int k) {
int n = height.size();
if (n < 2 || k < 2 || k > n) return 0;
int maxWater = 0;
deque<int> maxHeightIndices;
for (int i = 0; i < n; i++) {
while (!maxHeightIndices.empty() && maxHeightIndices.front() <= i - k) {
maxHeightIndices.pop_front();
}
while (!maxHeightIndices.empty() && height[maxHeightIndices.back()] < height[i]) {
maxHeightIndices.pop_back();
}
maxHeightIndices.push_back(i);
if (i >= k - 1) {
int leftHeight = height[maxHeightIndices.front()];
int rightHeight = height[i];
int water = min(leftHeight, rightHeight) * (k - 1);
maxWater = max(maxWater, water);
}
}
return maxWater;
}
};
This solution has a time complexity of O(n) and a space complexity of O(k), where n is the number of elements in the height array and k is the window size.
When to Use the Sliding Window Technique
The sliding window technique is particularly useful when:
- You need to process subarrays or subsequences of a fixed size.
- The problem involves finding a contiguous sequence that satisfies certain conditions.
- You want to optimize the time complexity by avoiding redundant computations.
Advanced Techniques and Optimizations
As you become more proficient in solving water container problems, you can explore advanced techniques and optimizations to further improve your solutions:
1. Segment Trees
Segment trees can be used to efficiently query and update range information, which can be helpful in certain water container problems that involve dynamic changes or range-based calculations.
2. Monotonic Stack/Queue
A monotonic stack or queue maintains elements in a strictly increasing or decreasing order, which can be useful for problems that require finding the next greater or smaller element efficiently.
3. Bit Manipulation
In some cases, bit manipulation techniques can be used to optimize space usage or perform certain operations more efficiently, especially when dealing with constraints or flags in water container problems.
4. Parallel Processing
For extremely large datasets, consider parallel processing techniques to distribute the workload across multiple cores or machines, potentially improving performance significantly.
Common Pitfalls and How to Avoid Them
When solving water container problems, be aware of these common pitfalls:
- Overlooking edge cases: Always consider empty arrays, single-element arrays, and other boundary conditions.
- Inefficient implementations: Be mindful of unnecessary nested loops or redundant calculations that can lead to poor time complexity.
- Incorrect assumptions: Don’t assume that the maximum height will always contribute to the solution. Consider all possible scenarios.
- Overcomplicating the solution: Sometimes, a simpler approach (like two pointers) can be more efficient than a complex dynamic programming solution.
- Ignoring space complexity: While optimizing for time, don’t forget to consider the space usage of your solution, especially for large inputs.
Practice and Further Learning
To master water container problems and related algorithmic challenges, consider the following steps:
- Solve varied problems: Practice with different variations of water container problems on platforms like LeetCode, HackerRank, or AlgoCademy.
- Analyze multiple solutions: For each problem, try to come up with different approaches and compare their time and space complexities.
- Study related topics: Deepen your understanding of data structures like stacks, queues, and trees, as well as algorithms like dynamic programming and greedy algorithms.
- Participate in coding contests: Join competitive programming contests to challenge yourself and learn from others’ solutions.
- Review and reflect: After solving a problem, take time to reflect on your approach and consider how you might improve it.
Conclusion
Water container problems are a fascinating category of algorithmic challenges that test a programmer’s ability to think critically, optimize solutions, and implement efficient algorithms. By mastering techniques like two-pointer approaches, dynamic programming, stack-based solutions, greedy algorithms, and sliding windows, you’ll be well-equipped to tackle a wide range of water container problems and related challenges in coding interviews and competitive programming scenarios.
Remember that the key to success is not just knowing these techniques, but also understanding when and how to apply them effectively. As you practice and gain experience, you’ll develop the intuition to quickly identify the most suitable approach for each problem you encounter.
Keep challenging yourself, exploring new problems, and refining your skills. With dedication and practice, you’ll be well on your way to becoming an expert in solving water container problems and a strong candidate for positions at top tech companies.