Mastering Visual Studio Code: A Comprehensive Guide for Developers
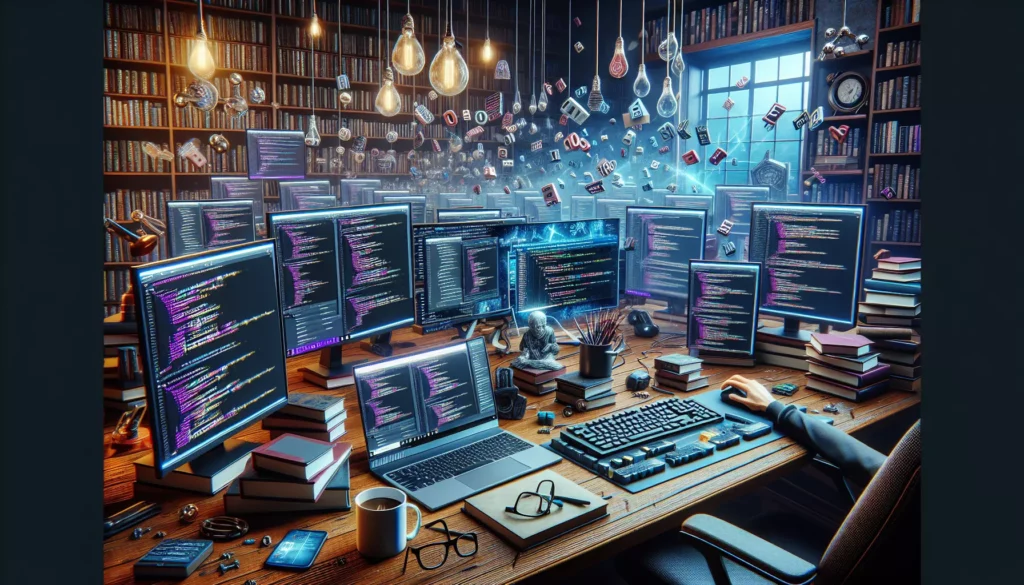
Visual Studio Code, often referred to as VS Code, has become one of the most popular and versatile code editors in recent years. Developed by Microsoft, this free, open-source editor offers a perfect balance of simplicity and power, making it an ideal choice for both beginners and experienced developers. In this comprehensive guide, we’ll explore the features, tips, and tricks that will help you become a VS Code power user and boost your coding productivity.
Table of Contents
- Introduction to Visual Studio Code
- Installation and Setup
- Understanding the Interface
- Essential Features
- Extending Functionality with Extensions
- Customization and Personalization
- Mastering Keyboard Shortcuts
- Debugging in VS Code
- Git Integration
- Integrated Terminal
- Remote Development
- Performance Tips and Tricks
- Conclusion
1. Introduction to Visual Studio Code
Visual Studio Code is a lightweight yet powerful source code editor that runs on your desktop. It comes with built-in support for JavaScript, TypeScript, and Node.js, and has a rich ecosystem of extensions for other languages and runtimes (such as C++, C#, Java, Python, PHP, Go, and .NET).
Some key features that make VS Code stand out include:
- IntelliSense: Provides smart completions based on variable types, function definitions, and imported modules.
- Debugging: Built-in debugging support for Node.js and can be extended to other runtimes.
- Git Commands: Built-in Git commands for easy version control.
- Extensibility: A wide range of extensions available to customize and enhance functionality.
- Cross-platform: Available for Windows, macOS, and Linux.
2. Installation and Setup
Installing Visual Studio Code is straightforward:
- Visit the official Visual Studio Code website.
- Download the appropriate version for your operating system (Windows, macOS, or Linux).
- Run the installer and follow the prompts.
- Once installed, launch VS Code.
After installation, you might want to configure some initial settings. You can access the settings by pressing Ctrl+,
(or Cmd+,
on macOS) or by clicking on the gear icon in the lower-left corner and selecting “Settings”.
Some initial settings you might want to consider:
- Auto Save: Enable auto-save to automatically save your files.
- Font Size: Adjust the font size for better readability.
- Color Theme: Choose a color theme that suits your preference.
- Word Wrap: Enable word wrap for long lines of code.
3. Understanding the Interface
Visual Studio Code’s interface is clean and intuitive. Let’s break down the main components:
- Activity Bar: The leftmost panel with icons for different views (File Explorer, Search, Source Control, Run and Debug, and Extensions).
- Side Bar: Opens when you click an Activity Bar icon, showing relevant information.
- Editor Groups: The main area where you edit your files. You can have multiple editor groups side by side.
- Panel: A resizable panel at the bottom for output, debug console, terminals, and problems.
- Status Bar: At the bottom, showing information about the opened project and files.
You can resize these components by dragging their edges, and you can toggle the visibility of the Side Bar (Ctrl+B
) and Panel (Ctrl+J
) for a cleaner interface when needed.
4. Essential Features
Visual Studio Code comes packed with features that make coding more efficient and enjoyable. Here are some essential features you should know:
4.1 IntelliSense
IntelliSense provides intelligent code completions based on language semantics and analysis of your source code. It supports a wide range of programming languages out of the box and can be extended for others.
To use IntelliSense:
- Start typing in a file, and suggestions will appear automatically.
- Use
Ctrl+Space
to manually trigger suggestions. - Navigate through suggestions using arrow keys and press
Tab
orEnter
to accept a suggestion.
4.2 Code Navigation
VS Code offers powerful code navigation features:
- Go to Definition:
F12
orCtrl+Click
on a symbol. - Peek Definition:
Alt+F12
to view a definition inline. - Find All References:
Shift+F12
to see all references of a symbol. - Go to Symbol:
Ctrl+Shift+O
to navigate to symbols within a file. - Go to Line:
Ctrl+G
to jump to a specific line number.
4.3 Code Refactoring
VS Code supports various refactoring operations:
- Rename Symbol:
F2
to rename a variable, function, or class across your project. - Extract Method: Select code and use the context menu to extract it into a new method.
- Move to a new file: Right-click on a class or function and select “Move to a new file”.
4.4 Multi-cursor Editing
Multi-cursor editing allows you to edit multiple parts of your file simultaneously:
Alt+Click
to add additional cursors.Ctrl+Alt+Up/Down
to add cursors above or below.Ctrl+D
to select the next occurrence of the current selection.
5. Extending Functionality with Extensions
One of VS Code’s strongest features is its extensibility. The VS Code Marketplace offers thousands of extensions that can add new languages, themes, debuggers, and other tools to your installation.
To install an extension:
- Click on the Extensions view in the Activity Bar (or press
Ctrl+Shift+X
). - Search for the extension you want.
- Click the “Install” button next to the extension.
Here are some popular extensions to consider:
- Python: Adds Python language support.
- ESLint: Integrates ESLint JavaScript linting into VS Code.
- Prettier: Code formatter for JavaScript, TypeScript, and more.
- GitLens: Supercharges Git capabilities in VS Code.
- Live Server: Launch a local development server with live reload feature.
6. Customization and Personalization
Visual Studio Code is highly customizable. You can personalize various aspects of the editor to suit your preferences and workflow.
6.1 Color Themes
To change the color theme:
- Press
Ctrl+K Ctrl+T
or go to File > Preferences > Color Theme. - Use the arrow keys to preview different themes.
- Press Enter to select a theme.
6.2 File Icon Themes
To change the file icon theme:
- Go to File > Preferences > File Icon Theme.
- Choose from the available icon themes or install new ones from the Marketplace.
6.3 User Settings
You can customize VS Code’s behavior through user settings:
- Open the Command Palette (
Ctrl+Shift+P
) and type “settings”. - Choose “Preferences: Open Settings (UI)” or “Preferences: Open Settings (JSON)”.
- Modify settings as needed.
Some useful settings to consider:
"editor.fontSize": 14,
"editor.wordWrap": "on",
"editor.tabSize": 2,
"editor.renderWhitespace": "all",
"files.autoSave": "afterDelay",
"workbench.startupEditor": "newUntitledFile"
7. Mastering Keyboard Shortcuts
Keyboard shortcuts can significantly speed up your workflow in VS Code. Here are some essential shortcuts to remember:
Ctrl+P
: Quick Open, Go to FileCtrl+Shift+P
: Show Command PaletteCtrl+Shift+N
: New WindowCtrl+Shift+W
: Close WindowCtrl+X
: Cut line (empty selection)Ctrl+C
: Copy line (empty selection)Alt+↑ / ↓
: Move line up/downShift+Alt+↓ / ↑
: Copy line up/downCtrl+Shift+K
: Delete lineCtrl+Enter
: Insert line belowCtrl+Shift+Enter
: Insert line aboveCtrl+Shift+\
: Jump to matching bracketCtrl+] / [
: Indent/outdent lineCtrl+/
: Toggle line commentShift+Alt+A
: Toggle block commentAlt+Z
: Toggle word wrap
You can view and customize keyboard shortcuts by going to File > Preferences > Keyboard Shortcuts or by pressing Ctrl+K Ctrl+S
.
8. Debugging in VS Code
Visual Studio Code has built-in debugging support for Node.js runtime and can be extended to other languages through extensions. Here’s how to use the debugger:
- Set breakpoints in your code by clicking on the gutter to the left of the line numbers.
- Open the Debug view in the Activity Bar (
Ctrl+Shift+D
). - Click on the gear icon to configure a launch.json file if it doesn’t exist.
- Select the environment that matches your project (e.g., Node.js).
- Press F5 to start debugging.
While debugging, you can:
- Step over (
F10
): Execute the current line and move to the next one. - Step into (
F11
): Step into a function call. - Step out (
Shift+F11
): Step out of the current function. - Continue (
F5
): Run until the next breakpoint. - Stop (
Shift+F5
): Stop the debugging session.
9. Git Integration
VS Code has excellent built-in support for Git. Here’s how to use it:
- Open the Source Control view in the Activity Bar (
Ctrl+Shift+G
). - If your project isn’t a Git repository, click on “Initialize Repository” to create one.
- Stage changes by clicking the + icon next to modified files.
- Enter a commit message in the text box at the top of the Source Control view.
- Click the checkmark icon or press
Ctrl+Enter
to commit the changes.
Other useful Git commands in VS Code:
- Pull: Click on the ellipsis (…) in the Source Control view and select “Pull”.
- Push: After committing, click on the sync icon in the Status Bar.
- Create Branch: Click on the branch name in the Status Bar and select “Create new branch”.
- Switch Branch: Click on the branch name in the Status Bar and select the branch to switch to.
10. Integrated Terminal
VS Code includes an integrated terminal, which allows you to execute command-line tasks without leaving the editor. To use the integrated terminal:
- Open the terminal with
Ctrl+`
(backtick) or go to View > Terminal. - You can create multiple terminal instances and switch between them.
- Use the dropdown in the terminal panel to change the shell type (e.g., PowerShell, Command Prompt, bash).
Useful terminal shortcuts:
Ctrl+Shift+`
: Create a new terminalCtrl+Shift+C
: Copy selected textCtrl+Shift+V
: Paste into terminalAlt+↑ / ↓
: Scroll up/downCtrl+Home / End
: Scroll to top/bottom
11. Remote Development
VS Code supports remote development, allowing you to use a container, remote machine, or Windows Subsystem for Linux (WSL) as a full-featured development environment. To get started with remote development:
- Install the Remote Development extension pack from the VS Code Marketplace.
- Use the Command Palette (
Ctrl+Shift+P
) and search for “Remote-SSH: Connect to Host” to connect to a remote machine. - For container development, use “Remote-Containers: Open Folder in Container”.
- For WSL, use “Remote-WSL: New Window” to open a new window connected to WSL.
Remote development allows you to:
- Work with different operating systems from your local VS Code installation.
- Develop in containers with a consistent, reproducible toolchain.
- Access more powerful hardware for tasks like compilation or data processing.
12. Performance Tips and Tricks
To keep VS Code running smoothly, especially when working with large projects, consider these tips:
- Exclude folders: Add large or unnecessary folders to the
files.exclude
setting to prevent VS Code from watching them. - Disable extensions: Disable extensions you’re not actively using to reduce memory usage.
- Use workspace trust: Enable workspace trust to restrict automatic code execution in unfamiliar projects.
- Adjust search settings: Modify
search.exclude
to ignore certain files or folders during searches. - Update regularly: Keep VS Code and its extensions up to date for the latest performance improvements.
Example settings for performance optimization:
"files.exclude": {
"**/node_modules": true,
"**/bower_components": true
},
"search.exclude": {
"**/node_modules": true,
"**/bower_components": true
},
"files.watcherExclude": {
"**/.git/objects/**": true,
"**/node_modules/**": true
}
13. Conclusion
Visual Studio Code is a powerful, flexible, and user-friendly code editor that can significantly enhance your coding experience. By mastering its features, customizing it to your needs, and leveraging its extensive ecosystem of extensions, you can create a highly efficient development environment tailored to your workflow.
Remember that becoming proficient with VS Code is an ongoing process. Regularly explore new features, extensions, and shortcuts to continually improve your productivity. The official VS Code documentation is an excellent resource for deeper dives into specific features and updates.
As you continue your journey with Visual Studio Code, don’t hesitate to experiment with different configurations and workflows. Share your discoveries with fellow developers and learn from their experiences. With practice and exploration, you’ll find that VS Code becomes an indispensable tool in your development arsenal, helping you write better code more efficiently.
Happy coding!