Mastering Version Control Systems: A Comprehensive Guide to Git
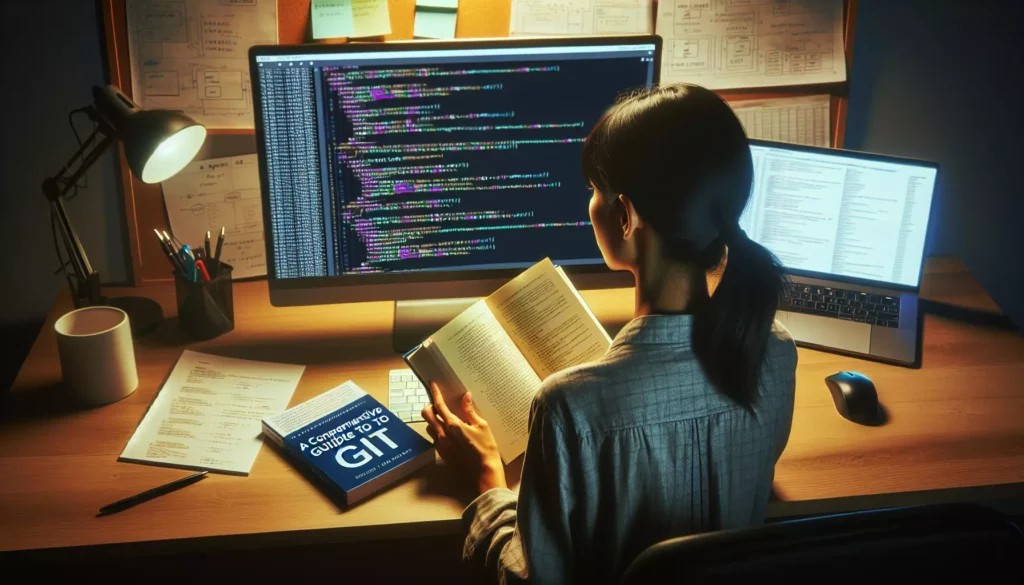
In the ever-evolving world of software development, version control systems (VCS) have become an indispensable tool for developers of all levels. Among these systems, Git stands out as the most widely used and powerful option. Whether you’re a beginner just starting your coding journey or an experienced developer looking to refine your skills, mastering Git can significantly enhance your productivity and collaboration abilities. In this comprehensive guide, we’ll explore the ins and outs of Git, providing you with the knowledge and techniques needed to become proficient in this essential tool.
Table of Contents
- Introduction to Version Control and Git
- Git Basics: Getting Started
- Understanding Git Workflow
- Branching and Merging Strategies
- Collaborating with Git
- Advanced Git Techniques
- Best Practices and Tips
- Git Tools and Integrations
- Troubleshooting Common Git Issues
- Conclusion
1. Introduction to Version Control and Git
Version control is a system that helps track changes to files over time, allowing multiple people to work on a project simultaneously. It provides a way to manage different versions of your code, revert changes if needed, and collaborate effectively with team members.
Git, created by Linus Torvalds in 2005, is a distributed version control system that has become the de facto standard in the software development industry. Its distributed nature means that each developer has a complete copy of the project’s history on their local machine, enabling offline work and providing a backup of the entire repository.
Key benefits of using Git include:
- Track changes and maintain a history of your project
- Collaborate with others seamlessly
- Create and manage multiple versions of your code (branches)
- Easily revert to previous versions if needed
- Work offline and sync changes later
2. Git Basics: Getting Started
To begin your journey with Git, you’ll need to install it on your machine. Visit the official Git website and download the appropriate version for your operating system.
Once installed, you’ll want to configure Git with your name and email address. Open a terminal or command prompt and enter the following commands:
git config --global user.name "Your Name"
git config --global user.email "your.email@example.com"
Now that Git is set up, let’s cover some basic commands:
- git init: Initialize a new Git repository in your current directory
- git clone [url]: Create a copy of a remote repository on your local machine
- git add [file]: Stage changes for commit
- git commit -m “Commit message”: Commit staged changes with a descriptive message
- git status: Check the status of your working directory and staging area
- git log: View commit history
Here’s an example of how to create a new repository and make your first commit:
mkdir my-project
cd my-project
git init
echo "# My Project" > README.md
git add README.md
git commit -m "Initial commit: Add README"
3. Understanding Git Workflow
The Git workflow typically involves three main areas:
- Working Directory: Where you make changes to your files
- Staging Area: Where you prepare changes for commit
- Repository: Where committed changes are stored
The basic workflow follows these steps:
- Make changes in your working directory
- Stage changes using
git add
- Commit changes using
git commit
- Push changes to a remote repository (if applicable) using
git push
Understanding this workflow is crucial for effective version control. It allows you to selectively choose which changes to include in each commit, creating a clean and organized history of your project.
4. Branching and Merging Strategies
Branching is one of Git’s most powerful features. It allows you to diverge from the main line of development and work on different features or experiments without affecting the main codebase.
Key branching commands:
- git branch: List, create, or delete branches
- git checkout -b [branch-name]: Create and switch to a new branch
- git merge [branch]: Merge changes from one branch into another
A common branching strategy is the “Feature Branch Workflow”:
- Create a new branch for each feature or bug fix
- Work on the feature in isolation
- Open a pull request to merge the changes back into the main branch
- Review and discuss the changes
- Merge the feature branch and delete it once complete
Here’s an example of creating and merging a feature branch:
git checkout -b feature/new-login-page
# Make changes and commit them
git push origin feature/new-login-page
# Open a pull request on GitHub or your preferred platform
# After review and approval
git checkout main
git merge feature/new-login-page
git push origin main
git branch -d feature/new-login-page
5. Collaborating with Git
Git shines when it comes to collaboration. Here are some key concepts and commands for working with others:
- Remote repositories: Versions of your project hosted on the internet or network
- git remote: Manage remote repositories
- git fetch: Download changes from a remote repository without merging
- git pull: Fetch and merge changes from a remote repository
- git push: Upload local changes to a remote repository
- Pull requests: A way to propose changes and discuss them before merging
When collaborating, it’s important to keep your local repository up to date:
git fetch origin
git merge origin/main
# Or, combine fetch and merge with:
git pull origin main
Remember to push your changes regularly to keep the remote repository updated:
git push origin your-branch-name
6. Advanced Git Techniques
As you become more comfortable with Git, you can explore advanced techniques to enhance your workflow:
- Interactive rebasing: Rewrite, reorder, or squash commits
- Cherry-picking: Apply specific commits from one branch to another
- Stashing: Temporarily store uncommitted changes
- Submodules: Include other Git repositories within your project
- Bisect: Binary search through your project’s history to find bugs
Here’s an example of interactive rebasing to squash multiple commits:
git rebase -i HEAD~3
# In the interactive editor, change 'pick' to 'squash' for the commits you want to combine
# Save and close the editor, then edit the commit message
7. Best Practices and Tips
To make the most of Git, consider these best practices:
- Write clear, concise commit messages
- Commit early and often
- Use branches for new features and bug fixes
- Keep your commits atomic (one logical change per commit)
- Pull changes from the remote repository before pushing
- Use .gitignore to exclude unnecessary files
- Regularly review and clean up your branches
A good commit message structure:
Short (50 chars or less) summary of changes
More detailed explanatory text, if necessary. Wrap it to about 72
characters or so. The blank line separating the summary from the body
is critical (unless you omit the body entirely).
Further paragraphs come after blank lines.
- Bullet points are okay, too
- Use a hyphen or asterisk followed by a single space
8. Git Tools and Integrations
While the command line is powerful, there are many tools and integrations that can enhance your Git experience:
- GUI Clients: GitKraken, SourceTree, GitHub Desktop
- IDE Integrations: Most modern IDEs have built-in Git support
- Hosting Platforms: GitHub, GitLab, Bitbucket
- CI/CD Tools: Jenkins, Travis CI, GitLab CI/CD
- Git Extensions: git-flow, git-lfs (Large File Storage)
These tools can simplify complex Git operations, provide visual representations of your repository, and integrate version control into your development workflow seamlessly.
9. Troubleshooting Common Git Issues
Even experienced developers encounter Git issues from time to time. Here are some common problems and their solutions:
- Merge conflicts: Occur when Git can’t automatically merge changes
- Solution: Manually edit the conflicting files, stage the changes, and commit
- Accidentally committed to the wrong branch
- Solution: Use
git reset
to undo the commit, stash or cherry-pick the changes, then apply them to the correct branch
- Solution: Use
- Undoing commits
- Solution: Use
git revert
for public branches orgit reset
for local branches
- Solution: Use
- Large files causing slow performance
- Solution: Use Git Large File Storage (LFS) for large binary files
When in doubt, don’t hesitate to consult Git’s documentation or seek help from the community. Remember, many developers have faced similar issues, and solutions are often readily available.
10. Conclusion
Mastering Git is an invaluable skill for any developer. It not only helps you manage your code more effectively but also enhances your ability to collaborate with others and contribute to open-source projects. As you continue to work with Git, you’ll discover new techniques and workflows that suit your specific needs.
Remember that becoming proficient with Git is a journey. Start with the basics, practice regularly, and gradually incorporate more advanced techniques into your workflow. Don’t be afraid to experiment in a test repository to understand how different commands work.
By investing time in learning Git, you’re not just mastering a tool; you’re adopting a mindset that values code organization, collaboration, and continuous improvement. These skills will serve you well throughout your career as a developer, whether you’re working on personal projects, contributing to open-source initiatives, or collaborating in a professional setting.
As you continue your journey with Git, consider exploring more advanced topics such as Git hooks, custom Git commands, and integrating Git into your continuous integration and deployment pipelines. The more you use Git, the more you’ll appreciate its power and flexibility in managing your codebase.
Happy coding, and may your commits always be clear and your merges conflict-free!