Mastering Version Control Systems: A Comprehensive Guide to Git
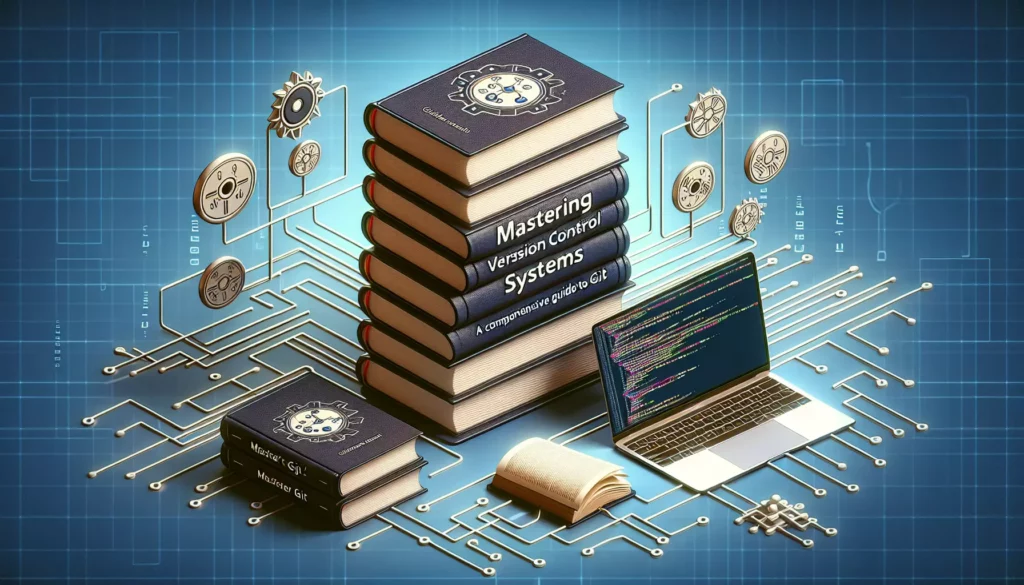
In the ever-evolving world of software development, version control systems (VCS) have become an indispensable tool for developers of all levels. Among these systems, Git stands out as the most widely used and powerful option. Whether you’re a beginner coder or an experienced programmer preparing for technical interviews at major tech companies, mastering Git is crucial for your success in the field.
In this comprehensive guide, we’ll explore the ins and outs of Git, from basic concepts to advanced techniques. By the end of this article, you’ll have a solid understanding of how to use Git effectively in your coding projects and collaborate seamlessly with other developers.
Table of Contents
- Understanding Version Control Systems
- Git Basics: Getting Started
- Essential Git Commands
- Branching and Merging
- Working with Remote Repositories
- Collaboration Workflows
- Advanced Git Techniques
- Git Best Practices
- Git Tools and Integrations
- Troubleshooting Common Git Issues
- Git in Technical Interviews
- Conclusion
1. Understanding Version Control Systems
Before diving into Git specifically, it’s essential to understand what version control systems are and why they’re crucial in software development.
What is a Version Control System?
A version control system is a tool that helps developers track and manage changes to their code over time. It allows multiple people to work on the same project simultaneously, keeping a record of who made what changes and when. This enables developers to:
- Collaborate effectively on large projects
- Revert to previous versions of code if needed
- Experiment with new features without affecting the main codebase
- Track the history of a project and understand how it has evolved
Types of Version Control Systems
There are three main types of version control systems:
- Local Version Control Systems: These systems keep track of files on your local computer. While simple, they don’t support collaboration with other developers.
- Centralized Version Control Systems (CVCS): These systems use a central server to store all versions of a project. Examples include Subversion (SVN) and Perforce.
- Distributed Version Control Systems (DVCS): In these systems, every developer has a full copy of the repository, including its history. Git is the most popular DVCS.
Git falls into the third category, offering numerous advantages over other types of VCS, particularly in terms of speed, flexibility, and collaboration capabilities.
2. Git Basics: Getting Started
Now that we understand the importance of version control, let’s dive into Git specifically.
Installing Git
To get started with Git, you’ll need to install it on your computer. Visit the official Git website and download the appropriate version for your operating system.
Configuring Git
After installation, you’ll need to configure Git with your name and email address. Open a terminal or command prompt and run the following commands:
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
Creating a Git Repository
To start using Git in a project, you need to initialize a repository. Navigate to your project directory in the terminal and run:
git init
This creates a new Git repository in your current directory.
Understanding the Git Workflow
The basic Git workflow consists of three main areas:
- Working Directory: Where you make changes to your files
- Staging Area: Where you prepare changes for a commit
- Repository: Where Git permanently stores changes as commits
Understanding this workflow is crucial for effective use of Git.
3. Essential Git Commands
To effectively use Git, you need to be familiar with its core commands. Here are some of the most essential Git commands you’ll use regularly:
git status
This command shows the current state of your working directory and staging area:
git status
git add
Use this command to add changes to the staging area:
git add filename.txt # Add a specific file
git add . # Add all changes
git commit
This command creates a new commit with the changes in the staging area:
git commit -m "Your commit message here"
git log
Use this to view the commit history:
git log
git diff
This command shows the differences between your working directory and the last commit:
git diff
git checkout
Use this to switch between branches or restore files:
git checkout branch-name # Switch to a branch
git checkout -- filename.txt # Discard changes in a file
4. Branching and Merging
Branching is one of Git’s most powerful features, allowing developers to work on different versions of a project simultaneously.
Creating and Switching Branches
To create a new branch:
git branch new-branch-name
To switch to the new branch:
git checkout new-branch-name
Or, you can create and switch to a new branch in one command:
git checkout -b new-branch-name
Merging Branches
To merge changes from one branch into another:
git checkout main # Switch to the main branch
git merge feature-branch # Merge the feature branch into main
Resolving Merge Conflicts
Sometimes, Git can’t automatically merge changes, resulting in a merge conflict. To resolve conflicts:
- Open the conflicting files and look for the conflict markers (<<<<<<<, =======, >>>>>>>)
- Manually edit the files to resolve the conflicts
- Use
git add
to mark the conflicts as resolved - Complete the merge with
git commit
5. Working with Remote Repositories
Remote repositories allow you to collaborate with other developers and back up your work.
Adding a Remote Repository
To add a remote repository:
git remote add origin https://github.com/username/repository.git
Pushing Changes to a Remote
To push your local changes to a remote repository:
git push origin branch-name
Pulling Changes from a Remote
To fetch and merge changes from a remote repository:
git pull origin branch-name
Cloning a Repository
To create a local copy of a remote repository:
git clone https://github.com/username/repository.git
6. Collaboration Workflows
Effective collaboration is key to successful software development. Git supports various collaboration workflows:
Centralized Workflow
In this simple workflow, all developers work on the main branch and push directly to the central repository. While straightforward, this can lead to conflicts in busy projects.
Feature Branch Workflow
This workflow involves creating a new branch for each feature or bug fix. This isolates changes and makes it easier to review and merge code.
Gitflow Workflow
Gitflow is a more structured workflow that defines specific branch types for different purposes (e.g., feature branches, release branches, hotfix branches).
Forking Workflow
Common in open-source projects, this workflow involves creating a personal copy (fork) of a repository, making changes, and then submitting a pull request to the original repository.
7. Advanced Git Techniques
As you become more comfortable with Git, you can explore more advanced techniques to enhance your workflow:
Interactive Rebasing
Interactive rebasing allows you to modify a series of commits before merging them into another branch:
git rebase -i HEAD~3 # Interactively rebase the last 3 commits
Cherry-picking
Cherry-picking allows you to apply specific commits from one branch to another:
git cherry-pick commit-hash
Stashing
Stashing allows you to temporarily save changes without committing them:
git stash # Stash changes
git stash pop # Apply and remove the latest stash
Git Hooks
Git hooks are scripts that run automatically before or after certain Git commands. They can be used to enforce coding standards, run tests, or trigger deployments.
8. Git Best Practices
To make the most of Git, follow these best practices:
- Write clear, descriptive commit messages
- Commit early and often
- Use branches for new features and bug fixes
- Keep your commits atomic (i.e., each commit should represent a single logical change)
- Regularly pull changes from the remote repository
- Use .gitignore to exclude unnecessary files
- Review your changes before committing
- Use meaningful branch names
9. Git Tools and Integrations
While Git is primarily used through the command line, there are many tools and integrations that can enhance your Git workflow:
GUI Clients
- GitKraken
- SourceTree
- GitHub Desktop
IDE Integrations
- Visual Studio Code Git integration
- IntelliJ IDEA Git integration
- Eclipse EGit
Continuous Integration/Continuous Deployment (CI/CD) Tools
- Jenkins
- Travis CI
- CircleCI
10. Troubleshooting Common Git Issues
Even experienced developers encounter Git issues from time to time. Here are some common problems and their solutions:
Accidentally Committing to the Wrong Branch
If you’ve committed changes to the wrong branch, you can use git reset
to undo the commit, then stash your changes, switch to the correct branch, and apply the stash.
Undoing a Pushed Commit
To undo a pushed commit, you can use git revert
:
git revert commit-hash
Resolving “Detached HEAD” State
If you find yourself in a detached HEAD state, you can create a new branch to save your work:
git checkout -b new-branch-name
Recovering Lost Commits
If you’ve lost commits due to a hard reset or branch deletion, you can often recover them using git reflog
.
11. Git in Technical Interviews
For those preparing for technical interviews, especially at major tech companies, understanding Git is crucial. Here are some Git-related topics that might come up in interviews:
- Explaining the Git workflow and basic commands
- Describing different branching strategies
- Discussing how to resolve merge conflicts
- Explaining the difference between merge and rebase
- Describing how to undo changes in Git
- Discussing best practices for collaboration using Git
Be prepared to not only explain these concepts but also to demonstrate your practical knowledge of Git commands and workflows.
12. Conclusion
Mastering Git is an essential skill for any developer, from beginners to those preparing for technical interviews at top tech companies. By understanding the fundamentals of version control, mastering essential Git commands, and exploring advanced techniques, you’ll be well-equipped to manage your code effectively and collaborate seamlessly with other developers.
Remember that becoming proficient with Git takes practice. Don’t be afraid to experiment with different commands and workflows in your personal projects. As you gain experience, you’ll develop a deeper understanding of Git’s capabilities and how to leverage them in your development process.
Whether you’re using Git for personal projects, contributing to open-source software, or preparing for a career at a major tech company, the skills you’ve learned in this guide will serve you well throughout your journey as a developer. Keep exploring, keep practicing, and keep pushing yourself to master the art of version control with Git.