Mastering Tree Data Structures: A Comprehensive Guide to Approaching Tree Problems
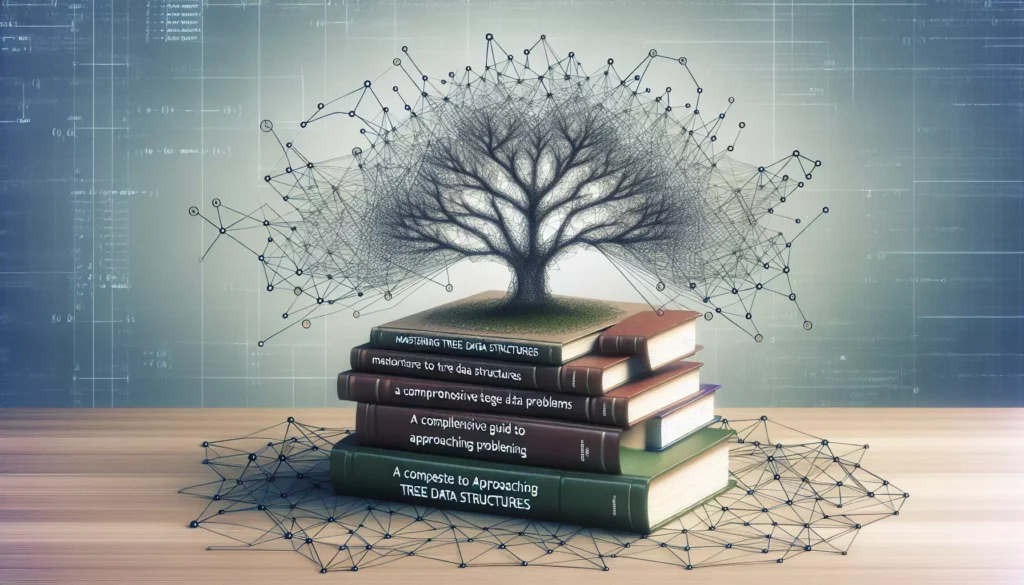
In the world of computer science and programming, tree data structures play a crucial role in organizing and manipulating hierarchical data. Whether you’re a beginner coder or preparing for technical interviews at major tech companies, understanding how to approach tree problems is essential. This comprehensive guide will walk you through the fundamentals of tree data structures, common types of trees, and strategies for solving tree-related problems efficiently.
Understanding Tree Data Structures
Before diving into problem-solving strategies, it’s important to have a solid grasp of what tree data structures are and why they’re important.
What is a Tree?
A tree is a hierarchical data structure consisting of nodes connected by edges. It has the following characteristics:
- One node is designated as the root of the tree.
- Every node (except the root) is connected to exactly one parent node.
- Each node can have zero or more child nodes.
- Nodes with no children are called leaf nodes.
Why are Trees Important?
Trees are used in various applications and algorithms due to their efficient organization of data:
- File systems in operating systems
- DOM (Document Object Model) in web browsers
- Expression parsing in compilers
- Implementing efficient search algorithms (e.g., Binary Search Trees)
- Representing hierarchical data (e.g., organizational structures)
Common Types of Trees
Before we delve into problem-solving strategies, let’s review some common types of trees you’ll encounter in coding interviews and real-world applications.
1. Binary Trees
A binary tree is a tree data structure in which each node has at most two children, referred to as the left child and the right child.
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode(int val) {
this.val = val;
this.left = null;
this.right = null;
}
}
2. Binary Search Trees (BST)
A binary search tree is a special type of binary tree with the following properties:
- The left subtree of a node contains only nodes with keys less than the node’s key.
- The right subtree of a node contains only nodes with keys greater than the node’s key.
- Both the left and right subtrees must also be binary search trees.
3. AVL Trees
An AVL tree is a self-balancing binary search tree where the heights of the left and right subtrees of any node differ by at most one.
4. Red-Black Trees
A red-black tree is another type of self-balancing binary search tree with additional properties to ensure balance.
5. N-ary Trees
An N-ary tree is a tree in which each node can have any number of children.
class TreeNode {
int val;
List<TreeNode> children;
TreeNode(int val) {
this.val = val;
this.children = new ArrayList<>();
}
}
Strategies for Approaching Tree Problems
Now that we’ve covered the basics, let’s explore strategies for tackling tree-related problems effectively.
1. Understand the Problem
Before diving into code, make sure you fully understand the problem statement and requirements:
- What type of tree are you working with?
- What operations need to be performed on the tree?
- Are there any specific constraints or edge cases to consider?
2. Visualize the Tree
Drawing out the tree structure can help you better understand the problem and devise a solution:
- Sketch a sample tree on paper or a whiteboard.
- Walk through the problem manually using your sample tree.
- Identify patterns or relationships between nodes that could be useful in solving the problem.
3. Choose the Right Traversal Method
Tree traversal is a fundamental operation in many tree-related problems. Choose the appropriate traversal method based on the problem requirements:
Depth-First Search (DFS)
- Pre-order traversal: Root, Left, Right
- In-order traversal: Left, Root, Right
- Post-order traversal: Left, Right, Root
// Pre-order traversal
void preOrder(TreeNode root) {
if (root == null) return;
System.out.print(root.val + " ");
preOrder(root.left);
preOrder(root.right);
}
// In-order traversal
void inOrder(TreeNode root) {
if (root == null) return;
inOrder(root.left);
System.out.print(root.val + " ");
inOrder(root.right);
}
// Post-order traversal
void postOrder(TreeNode root) {
if (root == null) return;
postOrder(root.left);
postOrder(root.right);
System.out.print(root.val + " ");
}
Breadth-First Search (BFS)
- Level-order traversal: Visit nodes level by level, from left to right
void levelOrder(TreeNode root) {
if (root == null) return;
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(root);
while (!queue.isEmpty()) {
int levelSize = queue.size();
for (int i = 0; i < levelSize; i++) {
TreeNode node = queue.poll();
System.out.print(node.val + " ");
if (node.left != null) queue.offer(node.left);
if (node.right != null) queue.offer(node.right);
}
System.out.println(); // Move to the next level
}
}
4. Identify Recursive Patterns
Many tree problems can be solved recursively. Look for patterns that can be applied to subtrees:
- What is the base case (e.g., leaf node or null node)?
- How can you break down the problem into smaller subproblems?
- How do you combine solutions from subtrees to solve the overall problem?
5. Consider Iterative Solutions
While recursive solutions are often intuitive for tree problems, iterative approaches can be more efficient in terms of space complexity:
- Use a stack to simulate recursion for DFS traversals.
- Use a queue for BFS traversals.
// Iterative in-order traversal
void inOrderIterative(TreeNode root) {
Stack<TreeNode> stack = new Stack<>();
TreeNode current = root;
while (current != null || !stack.isEmpty()) {
while (current != null) {
stack.push(current);
current = current.left;
}
current = stack.pop();
System.out.print(current.val + " ");
current = current.right;
}
}
6. Utilize Tree Properties
Leverage specific properties of the tree type you’re working with:
- For BSTs, use the ordering property to optimize search, insertion, and deletion operations.
- For balanced trees (e.g., AVL, Red-Black), consider how balance is maintained during operations.
7. Handle Edge Cases
Always consider and handle edge cases in your solutions:
- Empty tree (null root)
- Tree with only one node
- Unbalanced or skewed trees
8. Optimize Your Solution
After implementing a working solution, consider ways to optimize it:
- Can you reduce the time complexity?
- Can you improve the space complexity?
- Are there any redundant operations that can be eliminated?
Common Tree Problem Patterns
As you practice more tree problems, you’ll start to recognize common patterns. Here are some frequently encountered problem types and strategies to approach them:
1. Tree Traversal Problems
These problems involve visiting all nodes in a specific order or finding a particular node.
Strategy: Choose the appropriate traversal method (DFS or BFS) based on the problem requirements.
Example Problem: Print all nodes at a given level in a binary tree.
void printNodesAtLevel(TreeNode root, int targetLevel) {
if (root == null) return;
Queue<TreeNode> queue = new LinkedList<>();
queue.offer(root);
int level = 0;
while (!queue.isEmpty()) {
int size = queue.size();
level++;
for (int i = 0; i < size; i++) {
TreeNode node = queue.poll();
if (level == targetLevel) {
System.out.print(node.val + " ");
}
if (node.left != null) queue.offer(node.left);
if (node.right != null) queue.offer(node.right);
}
if (level == targetLevel) break;
}
}
2. Tree Construction Problems
These problems involve building a tree from given data or reconstructing a tree from traversal results.
Strategy: Understand the properties of the tree you’re constructing and use recursion to build subtrees.
Example Problem: Construct a binary tree from inorder and preorder traversal arrays.
class Solution {
int preIndex = 0;
Map<Integer, Integer> inorderMap = new HashMap<>();
public TreeNode buildTree(int[] preorder, int[] inorder) {
for (int i = 0; i < inorder.length; i++) {
inorderMap.put(inorder[i], i);
}
return buildTreeHelper(preorder, 0, inorder.length - 1);
}
private TreeNode buildTreeHelper(int[] preorder, int inStart, int inEnd) {
if (inStart > inEnd) return null;
TreeNode root = new TreeNode(preorder[preIndex++]);
int inIndex = inorderMap.get(root.val);
root.left = buildTreeHelper(preorder, inStart, inIndex - 1);
root.right = buildTreeHelper(preorder, inIndex + 1, inEnd);
return root;
}
}
3. Tree Modification Problems
These problems involve modifying the structure or values of a tree.
Strategy: Use recursion to modify subtrees and handle the base cases carefully.
Example Problem: Invert a binary tree.
TreeNode invertTree(TreeNode root) {
if (root == null) return null;
TreeNode left = invertTree(root.left);
TreeNode right = invertTree(root.right);
root.left = right;
root.right = left;
return root;
}
4. Path Problems
These problems involve finding or verifying paths in a tree that satisfy certain conditions.
Strategy: Use DFS with backtracking to explore all possible paths.
Example Problem: Find all root-to-leaf paths that sum to a given target.
class Solution {
List<List<Integer>> result = new ArrayList<>();
public List<List<Integer>> pathSum(TreeNode root, int targetSum) {
if (root == null) return result;
dfs(root, targetSum, new ArrayList<>());
return result;
}
private void dfs(TreeNode node, int remainingSum, List<Integer> path) {
if (node == null) return;
path.add(node.val);
if (node.left == null && node.right == null && remainingSum == node.val) {
result.add(new ArrayList<>(path));
} else {
dfs(node.left, remainingSum - node.val, path);
dfs(node.right, remainingSum - node.val, path);
}
path.remove(path.size() - 1);
}
}
5. Lowest Common Ancestor (LCA) Problems
These problems involve finding the lowest common ancestor of two nodes in a tree.
Strategy: Use recursion to search for the nodes and identify the LCA based on the search results.
Example Problem: Find the lowest common ancestor of two nodes in a binary tree.
TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
if (root == null || root == p || root == q) return root;
TreeNode left = lowestCommonAncestor(root.left, p, q);
TreeNode right = lowestCommonAncestor(root.right, p, q);
if (left != null && right != null) return root;
return (left != null) ? left : right;
}
Practice and Improvement
To master tree problems, consistent practice is key. Here are some tips to improve your skills:
- Solve diverse problems: Practice a variety of tree problems to expose yourself to different patterns and techniques.
- Implement from scratch: Try implementing basic tree operations (insertion, deletion, search) for different types of trees to strengthen your understanding.
- Analyze time and space complexity: For each solution, calculate and optimize the time and space complexity.
- Review and learn from others: After solving a problem, look at other solutions to learn alternative approaches and optimizations.
- Mock interviews: Practice solving tree problems under time pressure to simulate interview conditions.
Conclusion
Tree data structures are fundamental in computer science and are frequently featured in coding interviews, especially for roles at major tech companies. By understanding the different types of trees, mastering traversal techniques, and practicing various problem-solving strategies, you’ll be well-equipped to tackle tree-related challenges in your coding journey and technical interviews.
Remember that the key to success is not just memorizing solutions but understanding the underlying principles and patterns. As you continue to practice and refine your skills, you’ll develop a intuitive sense for approaching tree problems efficiently and effectively.
Keep coding, stay curious, and happy tree traversing!