Mastering Time and Space Complexity Analysis
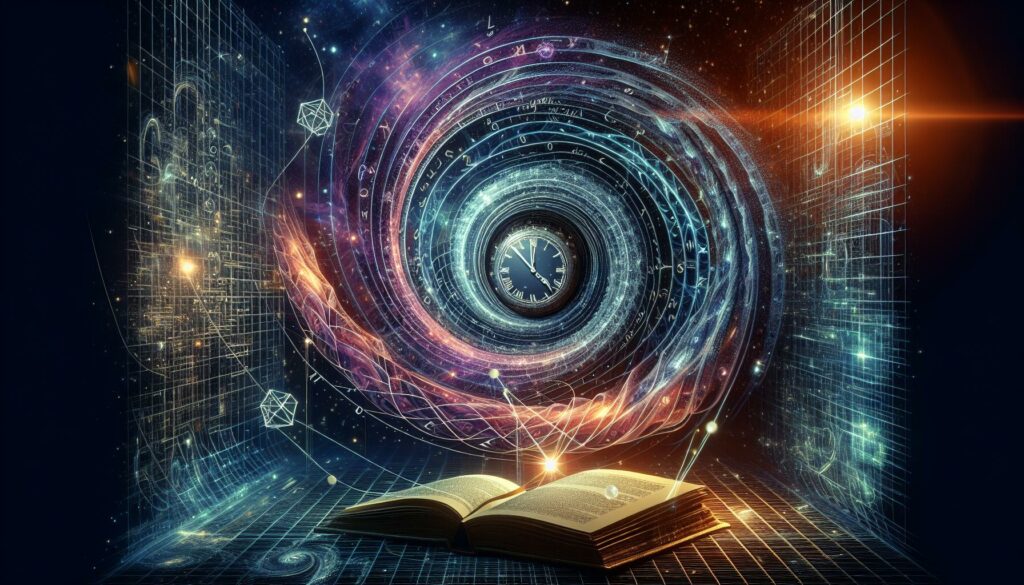
In the world of coding and algorithm design, efficiency is key. As software systems grow larger and more complex, the need for optimized algorithms becomes increasingly critical. This is where time and space complexity analysis comes into play. Understanding these concepts is essential for any programmer looking to write efficient code and excel in technical interviews, especially for positions at major tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google).
In this comprehensive guide, we’ll dive deep into the intricacies of time and space complexity analysis, providing you with the knowledge and tools to evaluate and optimize your algorithms effectively. Whether you’re a beginner looking to strengthen your foundational skills or an experienced developer preparing for technical interviews, this article will help you master these crucial concepts.
What is Time Complexity?
Time complexity is a measure of how the runtime of an algorithm increases as the size of the input grows. It’s typically expressed using Big O notation, which provides an upper bound on the growth rate of an algorithm’s runtime. Understanding time complexity allows developers to predict how their algorithms will perform with different input sizes and compare the efficiency of various solutions to a problem.
Common Time Complexities
- O(1) – Constant Time: The algorithm takes the same amount of time regardless of the input size.
- O(log n) – Logarithmic Time: The runtime grows logarithmically with the input size.
- O(n) – Linear Time: The runtime grows linearly with the input size.
- O(n log n) – Linearithmic Time: Common in efficient sorting algorithms like mergesort and quicksort.
- O(n^2) – Quadratic Time: Often seen in nested loops or less efficient sorting algorithms.
- O(2^n) – Exponential Time: The runtime doubles with each additional input element.
Analyzing Time Complexity
To analyze the time complexity of an algorithm, follow these steps:
- Identify the basic operations in the algorithm (e.g., comparisons, assignments).
- Count how many times each operation is executed.
- Express the count in terms of the input size (n).
- Keep only the highest-order term and drop any constants.
- Express the result using Big O notation.
Let’s look at an example to illustrate this process:
def find_max(arr):
max_val = arr[0]
for i in range(1, len(arr)):
if arr[i] > max_val:
max_val = arr[i]
return max_val
In this function:
- The basic operations are comparisons and assignments.
- The comparison
arr[i] > max_val
is executed n-1 times (where n is the length of the array). - The assignment
max_val = arr[i]
is executed at most n-1 times. - The highest-order term is n.
- Therefore, the time complexity is O(n).
What is Space Complexity?
Space complexity refers to the amount of memory an algorithm uses relative to the input size. Like time complexity, it’s typically expressed using Big O notation. Understanding space complexity is crucial for developing memory-efficient algorithms, especially when working with large datasets or on systems with limited resources.
Common Space Complexities
- O(1) – Constant Space: The algorithm uses a fixed amount of memory regardless of input size.
- O(n) – Linear Space: The memory usage grows linearly with the input size.
- O(n^2) – Quadratic Space: Often seen when creating 2D arrays or matrices based on input size.
- O(log n) – Logarithmic Space: Common in recursive algorithms that use the call stack efficiently.
Analyzing Space Complexity
To analyze the space complexity of an algorithm, consider:
- The amount of memory used by the input.
- Any additional data structures created by the algorithm.
- The maximum depth of the call stack for recursive algorithms.
Let’s examine an example:
def create_matrix(n):
matrix = []
for i in range(n):
row = [0] * n
matrix.append(row)
return matrix
In this function:
- We create a 2D array (matrix) of size n x n.
- Each element of the matrix takes constant space.
- The total space used grows quadratically with n.
- Therefore, the space complexity is O(n^2).
The Relationship Between Time and Space Complexity
Time and space complexity often have a trade-off relationship. In many cases, you can improve the time complexity of an algorithm by using more memory (increasing space complexity), or reduce space usage at the cost of increased runtime. This trade-off is a fundamental concept in algorithm design and optimization.
Example: Time-Space Trade-off
Consider the problem of finding if a number exists in an unsorted array. We have two approaches:
- Linear Search: O(n) time, O(1) space
- Hash Set: O(1) average time for lookups, O(n) space
Let’s implement both approaches:
def linear_search(arr, target):
for num in arr:
if num == target:
return True
return False
def hash_set_search(arr, target):
num_set = set(arr)
return target in num_set
The linear search has a time complexity of O(n) but uses constant space. The hash set approach has an average time complexity of O(1) for lookups but requires O(n) space to store all elements in the set. This illustrates how we can trade space for time in algorithm design.
Techniques for Improving Time and Space Complexity
Understanding time and space complexity is just the first step. The real challenge lies in optimizing your algorithms to achieve better performance. Here are some techniques to improve both time and space complexity:
1. Use Appropriate Data Structures
Choosing the right data structure can significantly impact both time and space complexity. For example:
- Use hash tables for fast lookups (O(1) average time).
- Use balanced binary search trees for ordered data with O(log n) operations.
- Use arrays for constant-time access to elements by index.
2. Optimize Loops
Nested loops often lead to higher time complexities. Try to reduce the number of nested loops or optimize their contents. For example:
// Before optimization: O(n^2)
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
// Do something
}
}
// After optimization: O(n)
for (int i = 0; i < n; i++) {
// Do something
}
for (int j = 0; j < n; j++) {
// Do something else
}
3. Use Memoization and Dynamic Programming
For problems with overlapping subproblems, use memoization or dynamic programming to avoid redundant calculations. This can significantly reduce time complexity at the cost of some additional space.
def fibonacci(n, memo={}):
if n in memo:
return memo[n]
if n <= 1:
return n
memo[n] = fibonacci(n-1, memo) + fibonacci(n-2, memo)
return memo[n]
4. Implement Efficient Sorting and Searching Algorithms
Use efficient sorting algorithms like Quicksort or Mergesort (O(n log n)) instead of less efficient ones like Bubble Sort (O(n^2)). For searching, consider binary search (O(log n)) for sorted data instead of linear search (O(n)).
5. Avoid Unnecessary Computations
Look for opportunities to reduce redundant calculations or operations. Sometimes, precomputing values or using mathematical properties can lead to significant optimizations.
6. Use In-place Algorithms
When possible, modify data structures in-place to save space. This is particularly useful for array manipulations.
def reverse_array(arr):
left, right = 0, len(arr) - 1
while left < right:
arr[left], arr[right] = arr[right], arr[left]
left += 1
right -= 1
return arr
Common Pitfalls in Complexity Analysis
When analyzing time and space complexity, be aware of these common pitfalls:
1. Ignoring Constants
While Big O notation ignores constants, they can be significant for small inputs. An O(n) algorithm with a large constant factor might be slower than an O(n log n) algorithm for small n.
2. Focusing Only on Worst-case Scenarios
While worst-case analysis is important, also consider average-case and best-case scenarios. Some algorithms, like Quicksort, have a worst-case of O(n^2) but an average-case of O(n log n).
3. Overlooking Hidden Loops
Be cautious of hidden loops in built-in functions or methods. For example, the in
operator for lists in Python is O(n), not O(1).
4. Misunderstanding Recursive Complexity
Analyzing the complexity of recursive algorithms can be tricky. Use recurrence relations or the Master Theorem for accurate analysis.
5. Neglecting Space Complexity
Don’t focus solely on time complexity. Space complexity is equally important, especially when dealing with large datasets or memory-constrained environments.
Advanced Topics in Complexity Analysis
As you become more proficient in complexity analysis, consider exploring these advanced topics:
1. Amortized Analysis
Amortized analysis considers the average performance of a sequence of operations, rather than the worst-case scenario for each operation. This is particularly useful for data structures like dynamic arrays, where occasional expensive operations are balanced by many cheap ones.
2. Randomized Algorithms
Some algorithms use randomization to achieve better average-case performance. Analyzing these algorithms often involves probabilistic methods and expected running times.
3. NP-Completeness
Understanding NP-completeness is crucial for recognizing problems that are inherently difficult to solve efficiently. This knowledge can guide you in choosing appropriate algorithms or heuristics for hard problems.
4. Lower Bounds
While Big O notation provides an upper bound, understanding lower bounds helps in determining whether an algorithm is optimal or if there’s room for improvement.
5. Complexity Classes
Familiarize yourself with different complexity classes (P, NP, PSPACE, etc.) to better understand the inherent difficulty of various computational problems.
Practical Applications in Technical Interviews
Mastering time and space complexity analysis is crucial for success in technical interviews, especially at FAANG companies. Here’s how to apply your knowledge effectively:
1. Analyze Given Solutions
When presented with a problem, always analyze the time and space complexity of your initial solution. This demonstrates your understanding and sets the stage for optimization discussions.
2. Discuss Trade-offs
Be prepared to discuss the trade-offs between different solutions. Explain why you might choose a solution with higher space complexity but better time complexity, or vice versa.
3. Optimize Incrementally
Start with a working solution, then incrementally optimize it. This approach showcases your problem-solving process and ability to improve algorithms.
4. Consider Edge Cases
Discuss how your solution performs for different input sizes and edge cases. This demonstrates a thorough understanding of complexity analysis.
5. Use Complexity as a Hint
If an interviewer asks for a solution with a specific time or space complexity, use this as a hint for the type of algorithm or data structure to use.
Conclusion
Mastering time and space complexity analysis is a fundamental skill for any serious programmer. It enables you to write efficient, scalable code and excel in technical interviews. By understanding these concepts and practicing their application, you’ll be well-equipped to tackle complex algorithmic challenges and optimize your solutions effectively.
Remember, the key to mastering complexity analysis is practice. Work through diverse programming problems, analyze your solutions, and constantly seek ways to optimize. With dedication and consistent effort, you’ll develop the intuition and skills necessary to excel in algorithm design and implementation.
As you continue your journey in programming and prepare for technical interviews, keep honing your skills in complexity analysis. It’s not just about passing interviews; it’s about becoming a better, more efficient programmer capable of solving real-world problems at scale. Happy coding!