Mastering the Whiteboard Interview: A Comprehensive Guide
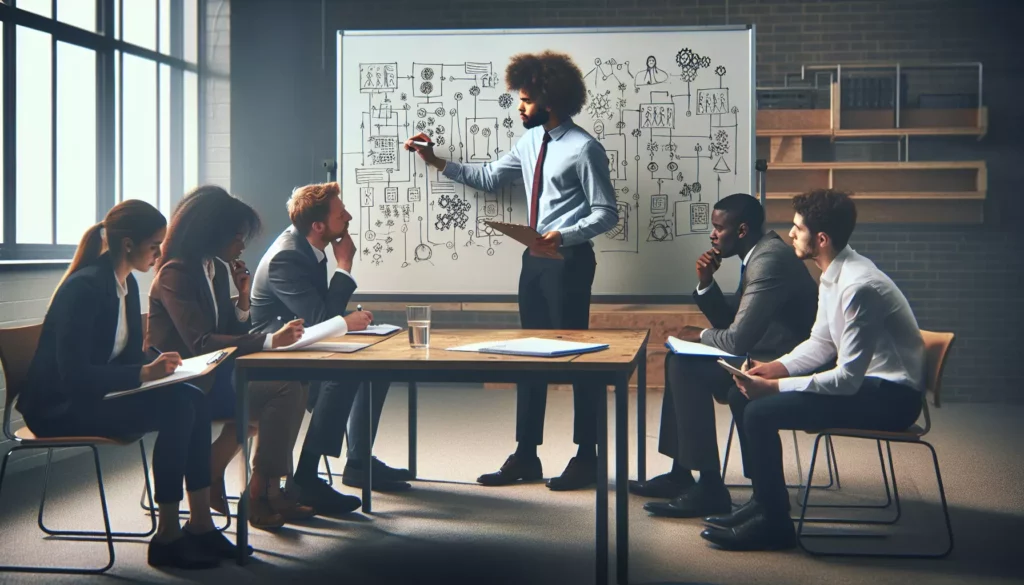
In the competitive world of tech recruitment, the whiteboard interview has become a staple for assessing candidates’ problem-solving skills, algorithmic thinking, and ability to communicate complex ideas. This article will dive deep into the nuances of whiteboard interviews, providing you with strategies to excel and land your dream job in software engineering or system design roles.
Understanding the Whiteboard Interview
A whiteboard interview is a technical assessment where candidates are asked to solve coding or system design problems on a whiteboard or piece of paper, without the aid of a computer. This format tests not only your coding abilities but also your problem-solving skills, communication, and ability to think on your feet.
Key Aspects of Whiteboard Interviews:
- Focus: Solving coding or system design problems without a computer
- Skills Tested: Algorithm design, problem-solving, communication, and coding without an IDE
- Format: Whiteboard or paper-based coding while explaining your thought process out loud
- Typical Roles: Software engineers, system designers
Preparing for a Whiteboard Interview
Success in a whiteboard interview requires thorough preparation. Here are some key areas to focus on:
1. Brush Up on Data Structures and Algorithms
A solid understanding of fundamental data structures and algorithms is crucial. Review the following:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Sorting and Searching algorithms
- Dynamic Programming
- Recursion
2. Practice Problem-Solving
Regularly solve coding problems on platforms like LeetCode, HackerRank, or CodeSignal. Focus on understanding the problem, developing an approach, and implementing a solution without relying on an IDE.
3. Improve Your Communication Skills
Practice explaining your thought process out loud while solving problems. This will help you articulate your ideas clearly during the interview.
4. Learn to Code Without an IDE
Practice writing code on paper or a whiteboard to get comfortable with coding without auto-complete or syntax highlighting.
The Whiteboard Interview Process
Understanding the typical flow of a whiteboard interview can help you navigate it more confidently. Here’s what you can expect:
1. Problem Presentation
The interviewer will present you with a problem. It could be a coding challenge, algorithm design, or system design question.
2. Clarification
Ask questions to ensure you fully understand the problem and requirements. This shows your attention to detail and communication skills.
3. Brainstorming
Think out loud as you consider different approaches to solve the problem. Discuss trade-offs between different solutions.
4. Solution Design
Outline your chosen approach on the whiteboard. This could include pseudocode, diagrams, or high-level descriptions of your algorithm.
5. Implementation
Write the actual code on the whiteboard. Remember to keep it clean, readable, and well-organized.
6. Testing and Optimization
Walk through your solution with test cases. Discuss the time and space complexity of your solution and potential optimizations.
Strategies for Success
Here are some key strategies to help you excel in your whiteboard interview:
1. Think Out Loud
Verbalize your thought process throughout the interview. This gives the interviewer insight into your problem-solving approach and allows them to provide hints if needed.
2. Start with a Simple Solution
Begin with a brute-force approach if you can’t immediately see an optimal solution. You can then work on optimizing it.
3. Use Pseudocode
If you’re unsure about syntax, use pseudocode to outline your algorithm before diving into the actual implementation.
4. Draw Diagrams
Visual representations can help clarify your thoughts and communicate complex ideas more effectively.
5. Test Your Code
After implementing your solution, walk through it with test cases to catch any errors or edge cases.
6. Analyze Time and Space Complexity
Be prepared to discuss the efficiency of your solution in terms of time and space complexity.
Common Whiteboard Interview Questions
While the specific questions can vary widely, here are some common types of problems you might encounter in a whiteboard interview:
1. Linked List Operations
Questions involving linked lists are common in whiteboard interviews. Let’s look at an example:
Example: Detect a Cycle in a Linked List
Here’s a Python implementation of the Floyd’s Cycle-Finding Algorithm (also known as the “tortoise and hare” algorithm) to detect a cycle in a linked list:
class ListNode:
def __init__(self, x):
self.val = x
self.next = None
def has_cycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
This algorithm uses two pointers, ‘slow’ and ‘fast’, moving at different speeds through the linked list. If there’s a cycle, the fast pointer will eventually catch up to the slow pointer.
2. Array Manipulation
Array-based problems are also frequently asked. Here’s an example:
Example: Two Sum Problem
Given an array of integers and a target sum, find two numbers in the array that add up to the target.
def two_sum(nums, target):
num_dict = {}
for i, num in enumerate(nums):
complement = target - num
if complement in num_dict:
return [num_dict[complement], i]
num_dict[num] = i
return []
This solution uses a hash table to achieve O(n) time complexity.
3. String Manipulation
String problems are common and can range from simple to complex. Here’s an example:
Example: Reverse Words in a String
def reverse_words(s):
# Split the string into words
words = s.split()
# Reverse the list of words
words.reverse()
# Join the words back into a string
return ' '.join(words)
This solution splits the string into words, reverses the list of words, and then joins them back together.
4. Tree Traversal
Tree-related problems, especially binary trees, are frequently asked. Here’s an example of in-order traversal:
Example: In-order Traversal of a Binary Tree
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def inorder_traversal(root):
result = []
def inorder(node):
if node:
inorder(node.left)
result.append(node.val)
inorder(node.right)
inorder(root)
return result
This recursive solution performs an in-order traversal of a binary tree, visiting the left subtree, then the root, then the right subtree.
5. Graph Algorithms
Graph problems can be more complex but are important for many roles. Here’s a simple example of Depth-First Search (DFS):
Example: Depth-First Search on a Graph
def dfs(graph, start, visited=None):
if visited is None:
visited = set()
visited.add(start)
print(start)
for next in graph[start] - visited:
dfs(graph, next, visited)
return visited
# Example usage:
graph = {'A': set(['B', 'C']),
'B': set(['A', 'D', 'E']),
'C': set(['A', 'F']),
'D': set(['B']),
'E': set(['B', 'F']),
'F': set(['C', 'E'])}
dfs(graph, 'A')
This implementation uses recursion to perform a depth-first search on a graph represented as an adjacency list.
System Design Questions
For more senior roles, you might encounter system design questions. These are typically more open-ended and test your ability to design large-scale systems. Here are some tips for approaching system design questions:
1. Clarify Requirements
Start by asking questions to understand the scope and constraints of the system you’re designing.
2. Estimate Scale
Consider the scale of the system – how many users, how much data, what kind of traffic patterns, etc.
3. Define APIs
Outline the main APIs that the system will need to support.
4. Define Data Model
Sketch out the main entities and their relationships.
5. High-level Design
Draw a block diagram of the main components of the system.
6. Detailed Design
Dive deeper into one or two components based on the interviewer’s interest.
7. Identify and Address Bottlenecks
Discuss potential bottlenecks and how you might address them.
Common Mistakes to Avoid
Being aware of common pitfalls can help you navigate your whiteboard interview more successfully. Here are some mistakes to avoid:
1. Jumping into Coding Too Quickly
Take time to understand the problem and plan your approach before starting to write code.
2. Not Asking Clarifying Questions
Don’t hesitate to ask for clarification if anything about the problem is unclear.
3. Staying Silent
Remember to think out loud and explain your thought process throughout the interview.
4. Ignoring Edge Cases
Consider and discuss edge cases in your solution.
5. Not Managing Time Effectively
Keep an eye on the time and pace yourself accordingly.
6. Getting Stuck and Not Moving On
If you’re stuck on a particular part of the problem, consider moving on and coming back to it later if time allows.
Handling Nervousness and Pressure
Whiteboard interviews can be stressful, but there are strategies to manage nervousness and perform at your best:
1. Practice, Practice, Practice
The more you practice solving problems on a whiteboard, the more comfortable you’ll feel during the actual interview.
2. Take Deep Breaths
If you feel yourself getting nervous, take a moment to take a few deep breaths.
3. Remember It’s a Conversation
Try to view the interview as a collaborative problem-solving session rather than a test.
4. It’s Okay to Make Mistakes
Remember that interviewers are often more interested in your problem-solving process than in whether you get the perfect solution immediately.
5. Use the Whiteboard to Your Advantage
Use the whiteboard to organize your thoughts, draw diagrams, or write down key information from the problem statement.
After the Interview
Once your whiteboard interview is complete, take some time to reflect on the experience:
1. Self-Evaluation
Think about what went well and areas where you could improve.
2. Follow Up
Send a thank-you note to your interviewer, reiterating your interest in the position.
3. Continue Learning
Regardless of the outcome, use the interview as a learning experience to further improve your skills.
Conclusion
Whiteboard interviews can be challenging, but with proper preparation and the right mindset, they can also be an opportunity to showcase your problem-solving skills and technical knowledge. Remember that the goal is not just to solve the problem, but to demonstrate your thought process, communication skills, and ability to work through complex problems methodically.
By understanding the format, preparing thoroughly, and applying the strategies outlined in this guide, you’ll be well-equipped to tackle your next whiteboard interview with confidence. Whether you’re a seasoned professional or just starting your career in software engineering or system design, mastering the art of the whiteboard interview is a valuable skill that can open doors to exciting opportunities in the tech industry.
Keep practicing, stay curious, and approach each interview as a chance to learn and grow. With persistence and the right preparation, you’ll be well on your way to acing your whiteboard interviews and landing your dream job in tech.