Mastering the Unknown: Strategies for Tackling Unfamiliar Coding Problems
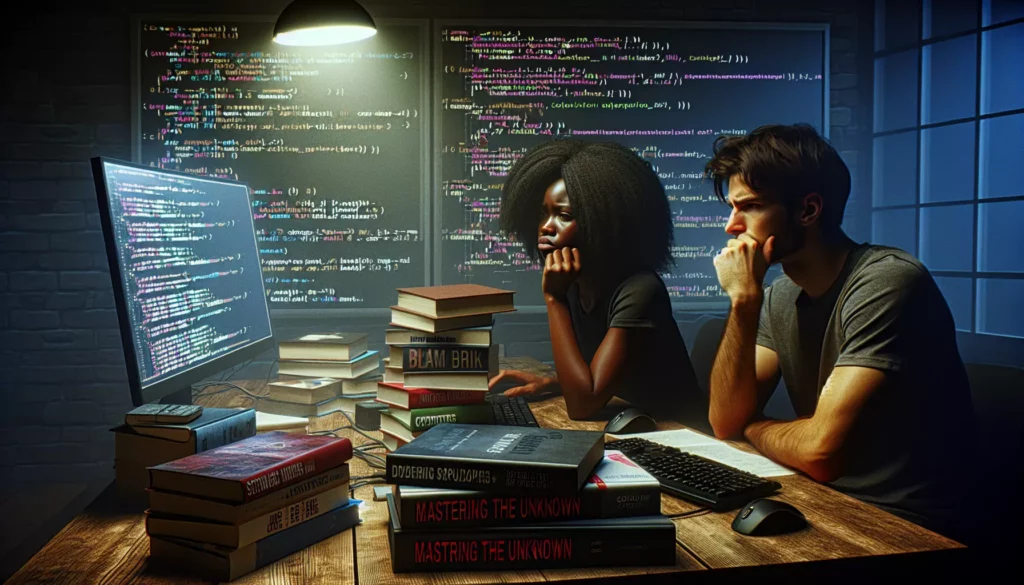
In the ever-evolving world of software development, encountering unfamiliar coding problems is not just common—it’s inevitable. Whether you’re a seasoned programmer or just starting your journey, the ability to approach and solve new challenges is a critical skill that can set you apart in the competitive tech industry. This article will explore effective strategies for tackling unfamiliar coding problems, helping you build confidence and expertise in your problem-solving abilities.
1. Understanding the Problem: The First Step to Success
Before diving into code, it’s crucial to fully understand the problem at hand. This initial step is often overlooked, but it’s the foundation for a successful solution.
1.1 Read and Reread the Problem Statement
Take the time to carefully read the problem statement multiple times. Look for key information such as:
- Input format and constraints
- Expected output
- Any special conditions or edge cases
- Time and space complexity requirements
1.2 Break Down the Problem
Divide the problem into smaller, manageable sub-problems. This approach, known as “divide and conquer,” can make complex issues more approachable.
1.3 Identify the Core Challenge
Try to pinpoint the main difficulty or the key concept that the problem is testing. Is it about data structures, algorithms, or a specific programming paradigm?
2. Plan Your Approach: Strategize Before Coding
With a clear understanding of the problem, it’s time to plan your attack. This phase is where you’ll outline your solution without getting bogged down in implementation details.
2.1 Brainstorm Potential Solutions
Consider multiple approaches to solving the problem. Even if some ideas seem far-fetched, write them down. Sometimes, a combination of different strategies can lead to an optimal solution.
2.2 Use Pseudocode
Write out your algorithm in plain English or pseudocode. This step helps you focus on the logic without worrying about syntax. For example:
1. Initialize an empty result array
2. For each element in the input array:
2.1 If element meets condition X:
2.1.1 Add element to result array
3. Sort the result array
4. Return the result array
2.3 Consider Time and Space Complexity
Evaluate the efficiency of your proposed solution. Will it meet the required time and space constraints? If not, can you optimize it?
3. Start Simple: Build a Foundation
When faced with a complex problem, it’s often beneficial to start with a simplified version and gradually build up to the full solution.
3.1 Solve a Simpler Version
Begin by solving a less complex version of the problem. This can help you understand the core logic and identify potential pitfalls.
3.2 Use Brute Force First
Implement a brute force solution if possible. While it may not be efficient, it can serve as a baseline and help you verify your understanding of the problem.
3.3 Incrementally Add Complexity
Gradually introduce additional requirements and optimize your solution step by step. This incremental approach can lead to insights that might be missed when tackling the full problem all at once.
4. Leverage Your Knowledge: Connect to Familiar Concepts
Even when facing an unfamiliar problem, you can often draw upon your existing knowledge and experience.
4.1 Identify Similar Problems
Try to recall similar problems you’ve encountered in the past. How did you approach them? Can those strategies be adapted to the current challenge?
4.2 Apply Known Algorithms and Data Structures
Consider whether any standard algorithms or data structures could be applicable. For example:
- Sorting algorithms for ordered data
- Hash tables for quick lookups
- Graph algorithms for network-related problems
- Dynamic programming for optimization problems
4.3 Use Analogies
Try to draw analogies between the unfamiliar problem and concepts you understand well. This can provide new perspectives and solution ideas.
5. Visualize the Problem: A Picture is Worth a Thousand Lines of Code
Visual representations can often provide clarity and insight when tackling complex problems.
5.1 Draw Diagrams
Use flowcharts, tree structures, or other diagrams to represent the problem and your solution visually. This can help you identify patterns and relationships that might not be apparent from the text alone.
5.2 Use Examples
Work through specific examples of the problem, tracing the steps of your proposed solution. This can help you catch logical errors and edge cases.
5.3 Create a Mental Model
Develop a mental model of how your solution works. This can make it easier to reason about the problem and explain your approach to others.
6. Implement and Test: Bringing Your Solution to Life
With a solid plan in place, it’s time to implement your solution and verify its correctness.
6.1 Write Clean, Modular Code
Even when working on a new problem, strive to write clean, well-organized code. This makes it easier to debug and refine your solution. For example:
def solve_problem(input_data):
processed_data = preprocess_data(input_data)
result = apply_algorithm(processed_data)
return postprocess_result(result)
def preprocess_data(data):
# Implementation details
def apply_algorithm(data):
# Core logic of the solution
def postprocess_result(result):
# Final touches on the output
6.2 Test with Multiple Cases
Create a variety of test cases, including:
- Simple, straightforward cases
- Edge cases and corner cases
- Large input sizes to test performance
6.3 Use Debugging Techniques
When your solution doesn’t work as expected, employ effective debugging strategies:
- Use print statements or logging to track the flow of your program
- Utilize a debugger to step through the code line by line
- Check intermediate results to pinpoint where the logic breaks down
7. Optimize and Refine: Polishing Your Solution
Once you have a working solution, look for ways to improve its efficiency and readability.
7.1 Analyze Time and Space Complexity
Carefully consider the time and space complexity of your solution. Can you reduce the number of nested loops or minimize memory usage?
7.2 Look for Redundancies
Identify any repeated calculations or redundant steps in your code. Can these be eliminated or optimized?
7.3 Consider Alternative Data Structures
Evaluate whether different data structures could improve the efficiency of your solution. For example, using a heap instead of a sorted array for maintaining a dynamic set of elements.
8. Learn and Reflect: Grow from the Experience
Each unfamiliar problem you tackle is an opportunity for growth and learning.
8.1 Review Other Solutions
After solving the problem, look at other people’s solutions. What approaches did they take? How do they compare to yours in terms of efficiency and readability?
8.2 Identify Key Takeaways
Reflect on what you learned from the problem. Did you encounter a new algorithm or data structure? Did you discover a clever way to optimize a particular operation?
8.3 Document Your Process
Keep notes on the problems you solve, including:
- The problem statement
- Your approach and reasoning
- Any challenges you faced and how you overcame them
- Key insights or lessons learned
This documentation can serve as a valuable resource for future problem-solving endeavors.
9. Cultivate a Problem-Solving Mindset
Developing the right mindset is crucial for consistently tackling unfamiliar coding problems.
9.1 Embrace the Challenge
View unfamiliar problems as opportunities to learn and grow, rather than obstacles to overcome. Adopt a growth mindset that sees challenges as a chance to expand your skills.
9.2 Practice Regularly
Make problem-solving a habit. Regularly engage with coding challenges on platforms like LeetCode, HackerRank, or AlgoCademy to expose yourself to a wide range of problems and keep your skills sharp.
9.3 Collaborate and Discuss
Engage with other programmers through coding forums, study groups, or pair programming sessions. Discussing problems and solutions with others can provide new perspectives and deepen your understanding.
10. Leverage Tools and Resources
Take advantage of the wealth of tools and resources available to support your problem-solving efforts.
10.1 Use Online Coding Environments
Platforms like AlgoCademy offer interactive coding environments where you can experiment with your solutions and receive immediate feedback. These tools can significantly speed up your development and testing process.
10.2 Consult Documentation and References
Keep official language documentation and algorithm references handy. Sometimes, the solution to an unfamiliar problem lies in a built-in function or a well-known algorithm that you may not have used before.
10.3 Utilize AI-Powered Assistance
AI-powered coding assistants, like those provided by AlgoCademy, can offer hints, explain concepts, and even suggest optimizations for your code. While it’s important not to rely too heavily on these tools, they can be valuable aids in your learning process.
Conclusion: Embracing the Journey of Continuous Learning
Tackling unfamiliar coding problems is an essential skill for any programmer, regardless of experience level. By following the strategies outlined in this article—from thorough problem analysis and strategic planning to implementation, testing, and optimization—you can approach new challenges with confidence and creativity.
Remember that becoming proficient at solving unfamiliar problems is a journey, not a destination. Each problem you encounter is an opportunity to expand your knowledge, refine your skills, and grow as a developer. Embrace the challenge, stay curious, and never stop learning.
As you continue to develop your problem-solving abilities, consider leveraging platforms like AlgoCademy, which offer structured learning paths, interactive coding environments, and AI-powered assistance. These resources can provide the guidance and practice you need to master the art of tackling unfamiliar coding problems and prepare for technical interviews at top tech companies.
With persistence, practice, and the right approach, you’ll find that even the most daunting coding challenges become opportunities for growth and innovation. Happy coding!