Mastering the Two Pointers Technique: A Comprehensive Guide for Coding Interviews
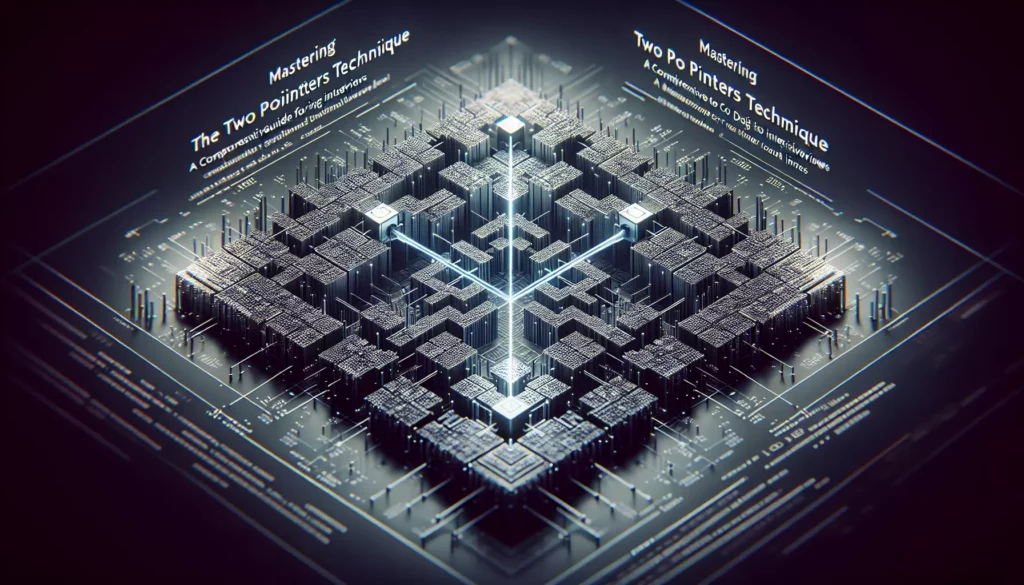
Welcome to our in-depth exploration of the Two Pointers technique, a powerful algorithmic approach that’s essential for coding interviews, especially when targeting top tech companies like FAANG (Facebook, Amazon, Apple, Netflix, Google). Whether you’re a beginner looking to enhance your problem-solving skills or an experienced developer preparing for technical interviews, understanding and mastering the Two Pointers technique can significantly boost your coding prowess.
Table of Contents
- Introduction to Two Pointers
- When to Use Two Pointers
- Types of Two Pointers Approaches
- Common Two Pointers Problems and Solutions
- Tips and Tricks for Implementing Two Pointers
- Practice Problems
- Advanced Two Pointers Techniques
- Conclusion
1. Introduction to Two Pointers
The Two Pointers technique is a simple yet effective approach to solving various algorithmic problems, particularly those involving arrays or linked lists. As the name suggests, this method involves using two pointers to traverse a data structure, often moving them towards each other or in the same direction based on certain conditions.
The primary advantage of the Two Pointers technique is its ability to reduce the time complexity of algorithms from O(n^2) to O(n) in many cases, making it an invaluable tool for optimizing solutions.
Basic Concept
At its core, the Two Pointers technique involves initializing two pointers, typically at different positions within an array or linked list, and then manipulating these pointers based on the problem’s requirements. The pointers can move towards each other, in the same direction, or even at different speeds.
2. When to Use Two Pointers
The Two Pointers technique is particularly useful in the following scenarios:
- Searching for pairs in a sorted array
- Reversing an array or string
- Removing duplicates from a sorted array
- Palindrome checking
- Sliding window problems
- Linked list cycle detection
- Merging two sorted arrays
Recognizing when to apply the Two Pointers technique is crucial for solving problems efficiently during coding interviews. Let’s delve deeper into some of these scenarios.
Searching for Pairs in a Sorted Array
When you need to find a pair of elements in a sorted array that sum up to a target value, the Two Pointers technique is highly effective. Instead of using nested loops (which would result in O(n^2) time complexity), you can use two pointers starting from both ends of the array and move them based on the sum of the elements they point to.
Reversing an Array or String
Two Pointers is an excellent choice for reversing an array or string in-place. By placing one pointer at the start and another at the end, you can swap elements as you move the pointers towards the center.
Removing Duplicates from a Sorted Array
When dealing with a sorted array, you can use two pointers to efficiently remove duplicates. One pointer keeps track of the position for the next unique element, while the other pointer scans through the array.
3. Types of Two Pointers Approaches
There are several variations of the Two Pointers technique, each suited for different types of problems:
3.1 Opposite Directional Pointers
In this approach, one pointer starts at the beginning of the array or string, while the other starts at the end. The pointers then move towards each other. This is commonly used in problems like reversing an array or finding a pair with a specific sum in a sorted array.
3.2 Same Directional Pointers
Here, both pointers start from the same end (usually the beginning) of the data structure but move at different speeds or with different conditions. This approach is often used in problems like removing duplicates from a sorted array or finding a cycle in a linked list.
3.3 Sliding Window
While not always categorized under Two Pointers, the Sliding Window technique can be considered a variation where two pointers define the boundaries of a window that slides over the data. This is useful for problems involving subarrays or substring with certain properties.
4. Common Two Pointers Problems and Solutions
Let’s explore some common problems that can be efficiently solved using the Two Pointers technique, along with their solutions.
4.1 Two Sum II – Input Array is Sorted
Problem: Given a sorted array of integers and a target sum, find two numbers such that they add up to the target.
Solution:
def twoSum(numbers, target):
left, right = 0, len(numbers) - 1
while left < right:
current_sum = numbers[left] + numbers[right]
if current_sum == target:
return [left + 1, right + 1] # Adding 1 for 1-based indexing
elif current_sum < target:
left += 1
else:
right -= 1
return [] # No solution found
This solution has a time complexity of O(n) and space complexity of O(1).
4.2 Remove Duplicates from Sorted Array
Problem: Given a sorted array, remove the duplicates in-place such that each element appears only once and return the new length.
Solution:
def removeDuplicates(nums):
if not nums:
return 0
# Initialize the pointer for the position to place the next unique element
next_unique = 1
# Iterate through the array starting from the second element
for i in range(1, len(nums)):
# If the current element is different from the previous one
if nums[i] != nums[i-1]:
# Place it at the next_unique position
nums[next_unique] = nums[i]
next_unique += 1
return next_unique
This solution modifies the array in-place and has a time complexity of O(n) and space complexity of O(1).
4.3 Valid Palindrome
Problem: Given a string, determine if it is a palindrome, considering only alphanumeric characters and ignoring cases.
Solution:
def isPalindrome(s):
# Convert to lowercase and remove non-alphanumeric characters
s = ''.join(c.lower() for c in s if c.isalnum())
left, right = 0, len(s) - 1
while left < right:
if s[left] != s[right]:
return False
left += 1
right -= 1
return True
This solution has a time complexity of O(n) and space complexity of O(1) if we consider the space used for the filtered string as part of the input.
5. Tips and Tricks for Implementing Two Pointers
To effectively implement the Two Pointers technique in your coding interviews, consider the following tips:
- Identify the problem type: Recognize patterns in the problem statement that hint at using Two Pointers, such as searching in a sorted array or dealing with palindromes.
- Choose the right variation: Decide whether to use opposite directional pointers, same directional pointers, or a sliding window based on the problem requirements.
- Initialize pointers correctly: Make sure to set the initial positions of your pointers appropriately. For example, in a sorted array problem, you might start with one pointer at each end.
- Move pointers strategically: Develop a clear strategy for when and how to move each pointer. This often involves comparing values at the pointer positions and making decisions based on the problem’s goals.
- Handle edge cases: Always consider edge cases, such as empty arrays or single-element arrays, in your implementation.
- Optimize space usage: Two Pointers often allows for in-place modifications, which can be a significant advantage in terms of space complexity.
- Practice, practice, practice: The more problems you solve using Two Pointers, the better you’ll become at recognizing when and how to apply this technique.
6. Practice Problems
To reinforce your understanding of the Two Pointers technique, try solving these practice problems:
- Container With Most Water (LeetCode 11): Given n non-negative integers representing the height of walls, find two walls that together with the x-axis forms a container that holds the most water.
- 3Sum (LeetCode 15): Given an array of integers, find all unique triplets in the array which gives the sum of zero.
- Sort Colors (LeetCode 75): Given an array with n objects colored red, white or blue, sort them in-place so that objects of the same color are adjacent.
- Longest Substring Without Repeating Characters (LeetCode 3): Given a string, find the length of the longest substring without repeating characters.
- Trapping Rain Water (LeetCode 42): Given n non-negative integers representing an elevation map where the width of each bar is 1, compute how much water it is able to trap after raining.
These problems cover a range of difficulties and will help you apply the Two Pointers technique in various scenarios.
7. Advanced Two Pointers Techniques
As you become more comfortable with the basic Two Pointers approach, you can explore some advanced variations and applications:
7.1 Three Pointers
Some problems may require the use of three pointers instead of two. This is often seen in problems like the Dutch National Flag problem (Sort Colors) or when dealing with three-way partitioning.
7.2 Fast and Slow Pointers
This technique, also known as Floyd’s Cycle-Finding Algorithm or the “tortoise and hare” algorithm, is particularly useful for detecting cycles in linked lists or arrays. One pointer (the “slow” pointer) moves one step at a time, while the other (the “fast” pointer) moves two steps at a time.
7.3 Multiple Arrays
Two Pointers can be applied to problems involving multiple arrays, such as merging two or more sorted arrays or finding common elements across arrays.
7.4 Sliding Window with Two Pointers
Combining the Sliding Window technique with Two Pointers can be powerful for solving substring or subarray problems with specific constraints.
Example: Linked List Cycle Detection
Here’s an example of using the Fast and Slow Pointers technique to detect a cycle in a linked list:
class ListNode:
def __init__(self, x):
self.val = x
self.next = None
def hasCycle(head):
if not head or not head.next:
return False
slow = head
fast = head.next
while slow != fast:
if not fast or not fast.next:
return False
slow = slow.next
fast = fast.next.next
return True
This algorithm has a time complexity of O(n) and space complexity of O(1), making it highly efficient for cycle detection.
8. Conclusion
The Two Pointers technique is a versatile and powerful tool in the arsenal of any programmer preparing for coding interviews, especially those targeting top tech companies. Its ability to optimize solutions, often reducing time complexity from O(n^2) to O(n), makes it invaluable for tackling a wide range of problems involving arrays, strings, and linked lists.
By mastering the various types of Two Pointers approaches – from opposite directional to same directional, and even advanced variations like Fast and Slow Pointers – you’ll be well-equipped to handle many common interview questions efficiently.
Remember, the key to success with the Two Pointers technique lies in practice and pattern recognition. As you solve more problems, you’ll develop an intuition for when and how to apply this technique effectively. Keep challenging yourself with diverse problems, and don’t hesitate to revisit and refine your solutions.
Happy coding, and best of luck in your interviews!