Mastering the Take-Home Assignment: Your Key to Landing That Dream Developer Job
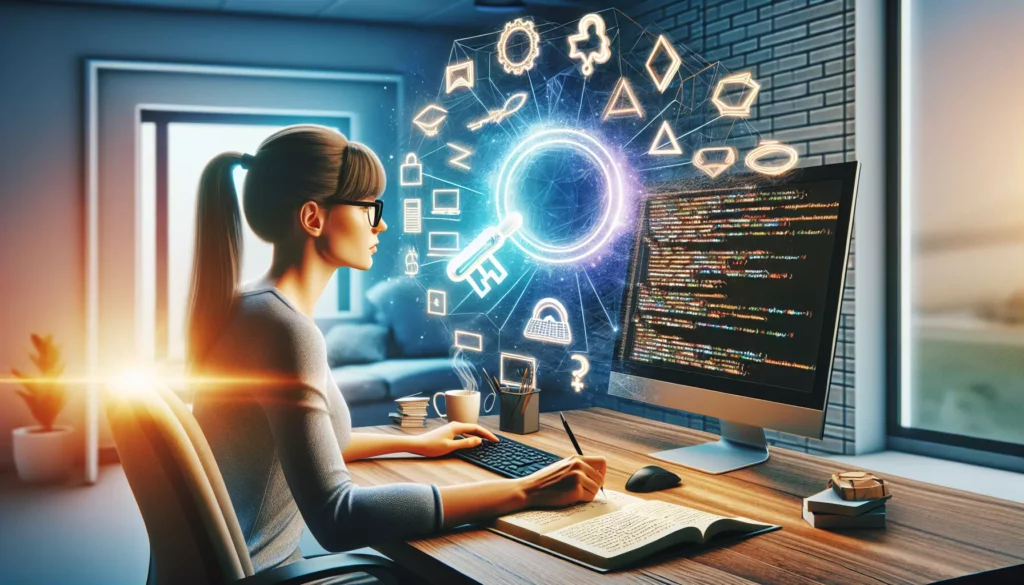
In the ever-evolving landscape of tech recruitment, the take-home assignment has emerged as a popular and effective method for assessing candidates’ skills. This approach allows companies to evaluate a candidate’s abilities in a real-world context, free from the pressures of on-the-spot coding interviews. For aspiring developers, particularly those aiming for roles at top tech companies, mastering the take-home assignment is crucial. In this comprehensive guide, we’ll explore the ins and outs of take-home assignments, provide strategies for success, and even walk through a sample assignment to help you prepare for your next job application.
Understanding Take-Home Assignments
Take-home assignments are a form of technical assessment where candidates are given a problem or project to complete on their own time, typically over a period of a few days. These assignments are designed to test a range of skills, including:
- Coding proficiency
- Problem-solving abilities
- Creativity in approach
- Attention to detail
- Real-world development skills
Unlike whiteboard interviews or timed coding challenges, take-home assignments offer a more relaxed environment that closely mimics actual work scenarios. This format is particularly common for roles such as full-stack developers, mobile developers, and web developers.
The Advantages of Take-Home Assignments
Take-home assignments offer several benefits for both candidates and employers:
- Realistic Assessment: They provide a more accurate representation of a candidate’s abilities in a real-world setting.
- Reduced Stress: Candidates can work at their own pace without the pressure of an interviewer watching.
- Deeper Evaluation: Employers can assess a wider range of skills, including code organization, documentation, and attention to detail.
- Fair Playing Field: This format can be particularly beneficial for candidates who may not perform well in high-pressure, on-the-spot coding interviews.
Common Types of Take-Home Assignments
Take-home assignments can vary widely depending on the company and the role. Some common types include:
- Building a Small Web Application: This could involve creating a simple CRUD (Create, Read, Update, Delete) application or a basic frontend interface.
- Implementing a Specific Feature: You might be asked to add functionality to an existing codebase.
- Solving an Algorithmic Problem: This could involve optimizing a function or implementing a complex algorithm.
- Data Analysis Task: For roles involving data science or analysis, you might be given a dataset to clean, analyze, and derive insights from.
- Bug Fixing: You could be presented with a piece of code containing bugs and asked to identify and fix them.
Strategies for Success
To excel in your take-home assignment, consider the following strategies:
1. Read the Instructions Carefully
Before diving into the code, make sure you understand the requirements thoroughly. Pay attention to:
- The specific problem or feature you’re asked to implement
- Any constraints or limitations mentioned
- The expected deliverables (e.g., code, documentation, tests)
- The deadline for submission
2. Plan Your Approach
Take some time to plan your solution before you start coding. This might include:
- Sketching out the overall architecture
- Breaking down the problem into smaller, manageable tasks
- Deciding on the technologies or libraries you’ll use
3. Focus on Code Quality
Remember that your code will be reviewed by experienced developers. Ensure your code is:
- Clean and well-organized
- Properly commented and documented
- Following best practices and design patterns
- Efficient and optimized where possible
4. Write Tests
Even if not explicitly required, including unit tests demonstrates your commitment to code quality and your understanding of test-driven development principles.
5. Document Your Work
Provide clear documentation on how to run your code, any assumptions you made, and explanations for key decisions in your implementation.
6. Manage Your Time Wisely
While you may have several days to complete the assignment, it’s important to manage your time effectively:
- Set aside dedicated time for the assignment
- Break the work into manageable chunks
- Leave time for testing and refactoring
- Ensure you submit before the deadline
A Sample Take-Home Assignment
To help you prepare, let’s walk through a sample take-home assignment that you might encounter in a job application process. This example is designed to test full-stack development skills.
Assignment: Build a Simple Task Management API
Requirements:
- Create a RESTful API for a task management system
- Implement CRUD operations for tasks
- Include user authentication
- Use a database of your choice for data persistence
- Provide documentation on how to run and use the API
- Include unit tests for key functionality
- Bonus: Implement a simple frontend to interact with the API
Time Limit: 3 days
Approach to Solving the Assignment
Let’s break down how you might approach this assignment:
1. Planning
Start by outlining the structure of your API:
- Decide on the technology stack (e.g., Node.js with Express for the backend, MongoDB for the database)
- Plan the API endpoints (e.g., /tasks, /users)
- Sketch out the data models for tasks and users
2. Setting Up the Project
Initialize your project and set up the basic structure:
mkdir task-management-api
cd task-management-api
npm init -y
npm install express mongoose bcrypt jsonwebtoken
npm install --save-dev jest supertest
3. Implementing the Core Functionality
Start with the basic Express server setup:
// server.js
const express = require('express');
const mongoose = require('mongoose');
const app = express();
app.use(express.json());
mongoose.connect('mongodb://localhost/task-manager', { useNewUrlParser: true, useUnifiedTopology: true });
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => console.log(`Server running on port ${PORT}`));
Next, create your models:
// models/User.js
const mongoose = require('mongoose');
const bcrypt = require('bcrypt');
const userSchema = new mongoose.Schema({
username: { type: String, required: true, unique: true },
password: { type: String, required: true }
});
userSchema.pre('save', async function(next) {
if (this.isModified('password')) {
this.password = await bcrypt.hash(this.password, 8);
}
next();
});
module.exports = mongoose.model('User', userSchema);
// models/Task.js
const mongoose = require('mongoose');
const taskSchema = new mongoose.Schema({
title: { type: String, required: true },
description: String,
completed: { type: Boolean, default: false },
owner: { type: mongoose.Schema.Types.ObjectId, required: true, ref: 'User' }
});
module.exports = mongoose.model('Task', taskSchema);
Implement authentication middleware:
// middleware/auth.js
const jwt = require('jsonwebtoken');
const User = require('../models/User');
const auth = async (req, res, next) => {
try {
const token = req.header('Authorization').replace('Bearer ', '');
const decoded = jwt.verify(token, 'your_jwt_secret');
const user = await User.findOne({ _id: decoded._id });
if (!user) {
throw new Error();
}
req.token = token;
req.user = user;
next();
} catch (e) {
res.status(401).send({ error: 'Please authenticate.' });
}
};
module.exports = auth;
Create routes for users and tasks:
// routes/users.js
const express = require('express');
const User = require('../models/User');
const bcrypt = require('bcrypt');
const jwt = require('jsonwebtoken');
const router = new express.Router();
router.post('/users', async (req, res) => {
const user = new User(req.body);
try {
await user.save();
const token = jwt.sign({ _id: user._id.toString() }, 'your_jwt_secret');
res.status(201).send({ user, token });
} catch (e) {
res.status(400).send(e);
}
});
router.post('/users/login', async (req, res) => {
try {
const user = await User.findOne({ username: req.body.username });
if (!user) {
throw new Error('Unable to login');
}
const isMatch = await bcrypt.compare(req.body.password, user.password);
if (!isMatch) {
throw new Error('Unable to login');
}
const token = jwt.sign({ _id: user._id.toString() }, 'your_jwt_secret');
res.send({ user, token });
} catch (e) {
res.status(400).send();
}
});
module.exports = router;
// routes/tasks.js
const express = require('express');
const Task = require('../models/Task');
const auth = require('../middleware/auth');
const router = new express.Router();
router.post('/tasks', auth, async (req, res) => {
const task = new Task({
...req.body,
owner: req.user._id
});
try {
await task.save();
res.status(201).send(task);
} catch (e) {
res.status(400).send(e);
}
});
router.get('/tasks', auth, async (req, res) => {
try {
const tasks = await Task.find({ owner: req.user._id });
res.send(tasks);
} catch (e) {
res.status(500).send();
}
});
router.get('/tasks/:id', auth, async (req, res) => {
const _id = req.params.id;
try {
const task = await Task.findOne({ _id, owner: req.user._id });
if (!task) {
return res.status(404).send();
}
res.send(task);
} catch (e) {
res.status(500).send();
}
});
router.patch('/tasks/:id', auth, async (req, res) => {
const updates = Object.keys(req.body);
const allowedUpdates = ['title', 'description', 'completed'];
const isValidOperation = updates.every((update) => allowedUpdates.includes(update));
if (!isValidOperation) {
return res.status(400).send({ error: 'Invalid updates!' });
}
try {
const task = await Task.findOne({ _id: req.params.id, owner: req.user._id });
if (!task) {
return res.status(404).send();
}
updates.forEach((update) => task[update] = req.body[update]);
await task.save();
res.send(task);
} catch (e) {
res.status(400).send(e);
}
});
router.delete('/tasks/:id', auth, async (req, res) => {
try {
const task = await Task.findOneAndDelete({ _id: req.params.id, owner: req.user._id });
if (!task) {
return res.status(404).send();
}
res.send(task);
} catch (e) {
res.status(500).send();
}
});
module.exports = router;
4. Writing Tests
Create test files for your routes:
// tests/task.test.js
const request = require('supertest');
const app = require('../server');
const Task = require('../models/Task');
const { userOneId, userOne, setupDatabase } = require('./fixtures/db');
beforeEach(setupDatabase);
test('Should create task for user', async () => {
const response = await request(app)
.post('/tasks')
.set('Authorization', `Bearer ${userOne.tokens[0].token}`)
.send({
title: 'Test task'
})
.expect(201);
const task = await Task.findById(response.body._id);
expect(task).not.toBeNull();
expect(task.completed).toEqual(false);
});
// Add more tests for other routes...
5. Documentation
Create a README.md file with instructions on how to set up and run your project:
# Task Management API
This is a simple task management API built with Node.js, Express, and MongoDB.
## Setup
1. Clone the repository
2. Install dependencies: `npm install`
3. Start MongoDB
4. Create a `.env` file with your MongoDB connection string and JWT secret
5. Run the server: `npm start`
## API Endpoints
### Users
- POST /users - Create a new user
- POST /users/login - Login user
### Tasks
- POST /tasks - Create a new task
- GET /tasks - Get all tasks for authenticated user
- GET /tasks/:id - Get a specific task
- PATCH /tasks/:id - Update a task
- DELETE /tasks/:id - Delete a task
## Running Tests
Run `npm test` to execute the test suite.
6. Bonus: Simple Frontend
If time allows, you could create a simple React frontend to interact with your API. This would demonstrate your full-stack capabilities.
After Completing the Assignment
Once you’ve finished your take-home assignment, take these final steps:
- Review Your Work: Go through your code one last time to catch any errors or areas for improvement.
- Test Thoroughly: Ensure all features work as expected and all tests pass.
- Polish Your Documentation: Make sure your README and any other documentation are clear and comprehensive.
- Prepare an Explanation: Be ready to discuss your implementation decisions in a follow-up interview.
Conclusion
Take-home assignments are an excellent opportunity to showcase your skills in a real-world context. By approaching them strategically and delivering high-quality work, you can significantly boost your chances of landing that dream developer job. Remember, the goal is not just to complete the assignment, but to demonstrate your problem-solving abilities, coding skills, and attention to detail.
As you prepare for your next job application, consider using platforms like AlgoCademy to sharpen your coding skills and practice tackling complex problems. With its focus on algorithmic thinking and practical coding skills, AlgoCademy can be an invaluable resource in your journey to becoming a top-tier developer.
Good luck with your next take-home assignment, and may it be the key that unlocks the door to your next great opportunity in the world of software development!