Mastering the Painting Fence Algorithm: A Comprehensive Guide
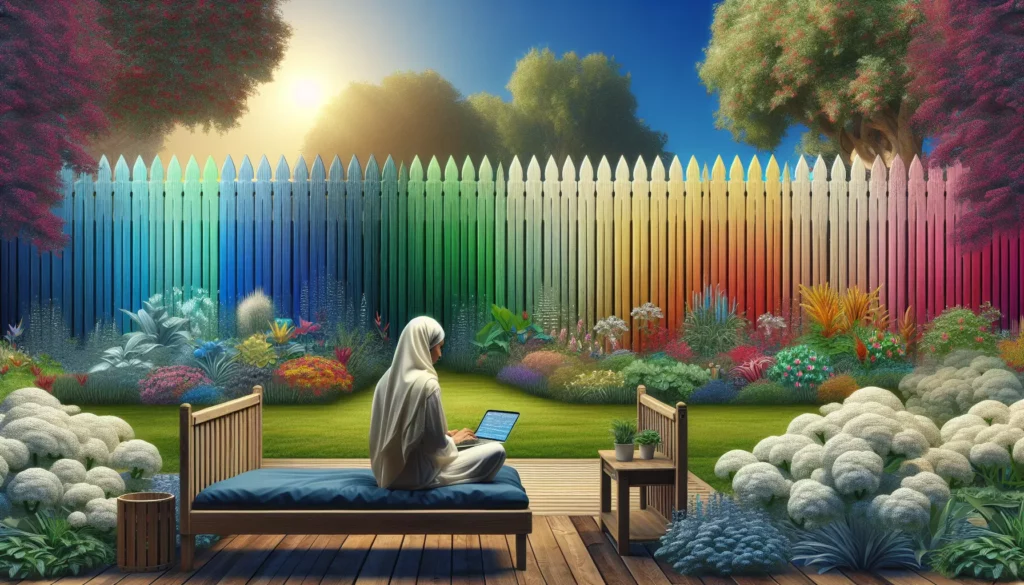
In the world of coding interviews and algorithmic problem-solving, the Painting Fence Algorithm stands out as an intriguing challenge that tests a programmer’s ability to think creatively and optimize solutions. This problem, often encountered in technical interviews at top tech companies, is not just a test of coding skills but also a gateway to understanding dynamic programming concepts. In this comprehensive guide, we’ll dive deep into the Painting Fence Algorithm, exploring its nuances, implementation strategies, and real-world applications.
Understanding the Painting Fence Problem
Before we delve into the solution, let’s clearly define the problem:
You have a fence with n posts and k colors. You need to paint all the posts such that no more than two adjacent fence posts have the same color. How many ways can you paint the fence?
This problem might seem simple at first glance, but it quickly becomes complex as you increase the number of posts and colors. The challenge lies in finding an efficient solution that can handle large inputs without excessive time or memory usage.
Breaking Down the Problem
To approach this problem, we need to break it down into smaller, manageable parts. Let’s consider the possible scenarios:
- When n = 1 (one post), we have k ways to paint it.
- When n = 2 (two posts), we have k * k ways to paint them (we can use any color for both posts).
- For n ≥ 3, we need to consider two cases:
- The last two posts have different colors
- The last two posts have the same color
The key to solving this problem efficiently lies in recognizing the pattern that emerges as we increase the number of posts.
Developing the Algorithm
Let’s develop our algorithm step by step:
1. Base Cases
We start by handling our base cases:
- If n = 0, return 0 (no ways to paint zero posts)
- If n = 1, return k (k ways to paint one post)
- If n = 2, return k * k (k * k ways to paint two posts)
2. Recursive Relation
For n ≥ 3, we can express the number of ways to paint n posts in terms of the number of ways to paint (n-1) and (n-2) posts:
ways(n) = (k-1) * (ways(n-1) + ways(n-2))
This relation comes from the fact that:
- To paint the nth post differently from the (n-1)th post, we have (k-1) choices, and this can be done in (k-1) * ways(n-1) ways.
- To paint the nth post the same as the (n-1)th post, we must ensure that the (n-1)th post is different from the (n-2)th post. This can be done in (k-1) * ways(n-2) ways.
3. Dynamic Programming Approach
While we could implement this recursively, a dynamic programming approach will be more efficient. We’ll use a bottom-up approach to build our solution:
function numWays(n, k):
if n == 0:
return 0
if n == 1:
return k
if n == 2:
return k * k
dp = [0] * (n + 1)
dp[1] = k
dp[2] = k * k
for i in range(3, n + 1):
dp[i] = (k - 1) * (dp[i-1] + dp[i-2])
return dp[n]
Implementing the Solution
Now that we have our algorithm, let’s implement it in Python:
def num_ways_to_paint_fence(n: int, k: int) -> int:
if n == 0:
return 0
if n == 1:
return k
if n == 2:
return k * k
dp = [0] * (n + 1)
dp[1] = k
dp[2] = k * k
for i in range(3, n + 1):
dp[i] = (k - 1) * (dp[i-1] + dp[i-2])
return dp[n]
# Test the function
print(num_ways_to_paint_fence(3, 2)) # Output: 6
print(num_ways_to_paint_fence(4, 3)) # Output: 66
This implementation has a time complexity of O(n) and a space complexity of O(n), which is efficient for most practical purposes.
Optimizing the Solution
While our current solution is good, we can optimize it further. Notice that at each step, we only need the values from the previous two steps. This means we can reduce our space complexity to O(1) by using just two variables instead of an array:
def num_ways_to_paint_fence_optimized(n: int, k: int) -> int:
if n == 0:
return 0
if n == 1:
return k
if n == 2:
return k * k
prev_prev = k
prev = k * k
for i in range(3, n + 1):
current = (k - 1) * (prev + prev_prev)
prev_prev = prev
prev = current
return prev
# Test the optimized function
print(num_ways_to_paint_fence_optimized(3, 2)) # Output: 6
print(num_ways_to_paint_fence_optimized(4, 3)) # Output: 66
This optimized version maintains the O(n) time complexity while reducing the space complexity to O(1).
Understanding the Time and Space Complexity
Let’s break down the complexity of our solution:
Time Complexity
The time complexity of both implementations is O(n). We iterate through the posts once, performing constant-time operations at each step.
Space Complexity
- Original implementation: O(n) – We use an array of size n+1 to store intermediate results.
- Optimized implementation: O(1) – We only use three variables regardless of the input size.
The optimization from O(n) to O(1) space can be significant for large inputs, especially in memory-constrained environments.
Common Pitfalls and Edge Cases
When implementing the Painting Fence Algorithm, be aware of these common pitfalls:
- Forgetting base cases: Always handle n = 0, n = 1, and n = 2 separately.
- Integer overflow: For large values of n and k, the result might exceed the range of a 32-bit integer. Consider using long integers or implementing modulo arithmetic if required.
- Misunderstanding the problem: Remember, the constraint is that no more than two adjacent posts can have the same color, not that adjacent posts must have different colors.
Variations of the Problem
The Painting Fence Algorithm can be modified to create interesting variations:
- Different color constraints: What if no three consecutive posts can have the same color?
- Circular fence: How would the solution change if the fence was circular (the first and last post are adjacent)?
- Limited paint supply: What if you had a limited amount of each color?
These variations can help deepen your understanding of dynamic programming and combinatorics.
Real-world Applications
While painting fences might seem like a purely academic exercise, the underlying principles have real-world applications:
- Resource Allocation: In computer networks, assigning channels to devices while minimizing interference.
- Scheduling: Creating schedules that avoid conflicts, such as exam timetables or shift rosters.
- Game Development: Generating diverse, rule-based content for games.
- Cryptography: Designing secure encoding schemes with specific constraints.
Interview Strategies
If you encounter this problem in an interview, consider the following strategies:
- Clarify the problem: Ensure you understand all constraints and requirements.
- Start with examples: Work through small examples to identify patterns.
- Think aloud: Share your thought process with the interviewer.
- Optimize incrementally: Start with a basic solution, then improve it step by step.
- Test your solution: Use edge cases to verify your implementation.
Practice Problems
To reinforce your understanding of the Painting Fence Algorithm and similar dynamic programming problems, try these practice problems:
- Climbing Stairs (LeetCode 70): Similar recurrence relation, great for beginners.
- House Robber (LeetCode 198): Another problem solvable with a similar approach.
- Decode Ways (LeetCode 91): A more complex problem that builds on similar principles.
Conclusion
The Painting Fence Algorithm is a classic example of how seemingly simple problems can lead to elegant and efficient solutions through dynamic programming. By mastering this algorithm, you’re not just solving a specific problem — you’re developing a problem-solving mindset that will serve you well in tackling a wide range of algorithmic challenges.
Remember, the key to mastering algorithms like this is practice and persistence. Don’t be discouraged if you don’t grasp it immediately. Keep working through examples, implement the solution in different programming languages, and try to apply the principles to similar problems.
As you continue your journey in algorithmic problem-solving, you’ll find that the skills you develop here — breaking down complex problems, recognizing patterns, and optimizing solutions — will be invaluable in your career as a software developer or engineer.
Happy coding, and may your fences always be colorful and algorithmically painted!