Mastering the N-Queens Problem: A Comprehensive Guide for Coding Interviews
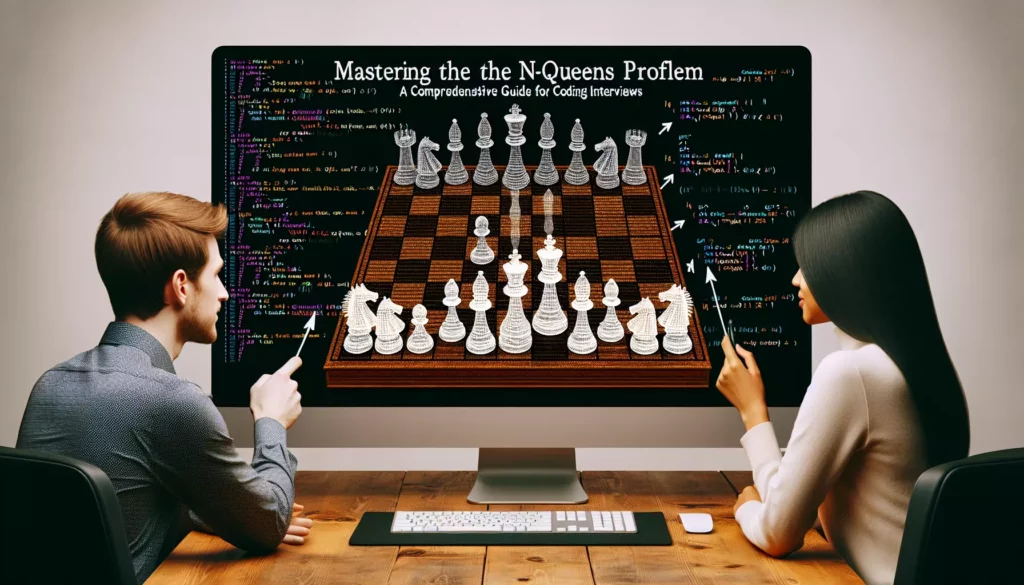
Welcome to AlgoCademy’s in-depth exploration of the N-Queens problem, a classic challenge that frequently appears in coding interviews, especially for prestigious tech companies like FAANG (Facebook, Amazon, Apple, Netflix, and Google). This problem is not just a test of your coding skills; it’s a gateway to understanding complex algorithmic concepts and honing your problem-solving abilities.
What is the N-Queens Problem?
The N-Queens problem is a puzzle where you need to place N chess queens on an N×N chessboard so that no two queens threaten each other. In chess, a queen can attack horizontally, vertically, and diagonally. The challenge is to find all possible arrangements where no queen is under attack from any other queen.
This problem is significant for several reasons:
- It tests your ability to think recursively and use backtracking algorithms.
- It challenges your spatial reasoning and pattern recognition skills.
- It’s an excellent example of how to approach and solve complex problems systematically.
- It has real-world applications in areas like constraint satisfaction problems and optimization.
Understanding the Problem
Before diving into the solution, let’s break down the problem:
- You have an N×N chessboard.
- You need to place N queens on this board.
- No two queens should be able to attack each other.
- You need to find all possible solutions.
For example, for a 4×4 board (N = 4), there are two possible solutions:
Solution 1:
. Q . .
. . . Q
Q . . .
. . Q .
Solution 2:
. . Q .
Q . . .
. . . Q
. Q . .
Where ‘Q’ represents a queen and ‘.’ represents an empty square.
Approaching the Solution
The most efficient way to solve the N-Queens problem is using a backtracking algorithm. Backtracking is an algorithmic technique that considers searching every possible combination in order to solve a computational problem. Here’s a step-by-step approach:
- Start in the leftmost column
- If all queens are placed, return true
- Try all rows in the current column:
- If the queen can be placed safely, mark this [row, column] as part of the solution
- Recursively check if placing the queen here leads to a solution
- If placing the queen in [row, column] leads to a solution, return true
- If placing the queen doesn’t lead to a solution, unmark this [row, column] (backtrack) and try other rows
- If all rows have been tried and nothing worked, return false to trigger backtracking
Implementing the Solution
Let’s implement this solution in Python. We’ll start with a function to check if a queen can be placed on the board safely:
def is_safe(board, row, col, n):
# Check this row on left side
for i in range(col):
if board[row][i] == 1:
return False
# Check upper diagonal on left side
for i, j in zip(range(row, -1, -1), range(col, -1, -1)):
if board[i][j] == 1:
return False
# Check lower diagonal on left side
for i, j in zip(range(row, n, 1), range(col, -1, -1)):
if board[i][j] == 1:
return False
return True
Now, let’s implement the main solving function using backtracking:
def solve_n_queens_util(board, col, n):
if col >= n:
return True
for i in range(n):
if is_safe(board, i, col, n):
board[i][col] = 1
if solve_n_queens_util(board, col + 1, n):
return True
board[i][col] = 0 # Backtrack
return False
def solve_n_queens(n):
board = [[0 for _ in range(n)] for _ in range(n)]
if not solve_n_queens_util(board, 0, n):
print("Solution does not exist")
return False
print_solution(board)
return True
def print_solution(board):
for row in board:
print(" ".join("Q" if x else "." for x in row))
This implementation finds one solution. To find all solutions, we need to modify our approach slightly:
def solve_n_queens_all(n):
board = [[0 for _ in range(n)] for _ in range(n)]
solutions = []
def backtrack(col):
if col == n:
solutions.append(["".join("Q" if x else "." for x in row) for row in board])
return
for i in range(n):
if is_safe(board, i, col, n):
board[i][col] = 1
backtrack(col + 1)
board[i][col] = 0 # Backtrack
backtrack(0)
return solutions
# Example usage:
n = 4
all_solutions = solve_n_queens_all(n)
for i, solution in enumerate(all_solutions, 1):
print(f"Solution {i}:")
for row in solution:
print(row)
print()
Time and Space Complexity
The time complexity of the N-Queens problem is O(N!), where N is the number of queens (which is also the size of the board). This is because, in the worst case, we have to try all possible combinations of queen placements.
The space complexity is O(N^2) for the board representation, plus the recursion stack which can go up to O(N) deep.
Optimizations and Variations
While the backtracking solution is efficient for most purposes, there are several optimizations and variations worth considering:
1. Bit Manipulation
For large values of N, we can use bit manipulation to represent the board state, which can significantly speed up the process of checking for conflicts.
2. Symmetry Reduction
We can reduce the number of solutions we need to find by considering symmetries. For example, if we find a solution, we know that its mirror image is also a solution.
3. Iterative Approach
While the recursive backtracking approach is intuitive, an iterative approach can be more efficient in terms of memory usage, especially for large N.
4. Parallel Processing
For very large N, we can parallelize the search process, exploring different branches of the solution space concurrently.
Real-World Applications
While the N-Queens problem might seem like a purely academic exercise, it has several real-world applications:
- VLSI Design: In Very Large Scale Integration (VLSI) chip design, the N-Queens problem can be used to model the placement of components to minimize interference.
- Resource Allocation: The problem can be generalized to model resource allocation problems where resources cannot interfere with each other.
- Load Balancing: In distributed computing, the N-Queens problem can be used to model load balancing across servers.
- Constraint Satisfaction Problems: The N-Queens problem is a classic example of a constraint satisfaction problem, which has applications in scheduling, planning, and configuration.
Common Interview Questions and Tips
When tackling the N-Queens problem in a coding interview, keep these points in mind:
- Understand the Problem: Make sure you fully understand the problem before starting to code. Ask clarifying questions if needed.
- Explain Your Approach: Before diving into coding, explain your approach to the interviewer. This shows your problem-solving process.
- Start Simple: Begin with a simple implementation and then optimize. It’s better to have a working solution that you can improve than to get stuck trying to create a perfect solution from the start.
- Consider Edge Cases: Discuss how your solution handles edge cases, like N = 1 or N = 2.
- Analyze Complexity: Be prepared to discuss the time and space complexity of your solution.
- Optimization Ideas: Even if you don’t implement them, discussing potential optimizations shows depth of understanding.
Practice and Further Learning
To truly master the N-Queens problem and similar backtracking challenges, consider the following steps:
- Implement Variations: Try implementing the optimizations mentioned earlier, like bit manipulation or an iterative approach.
- Solve Related Problems: Tackle similar backtracking problems like Sudoku Solver, Knight’s Tour, or Graph Coloring.
- Visualize the Process: Create a visualization of your algorithm to better understand how backtracking works.
- Time Your Solutions: Compare the runtime of different implementations to understand the impact of optimizations.
- Explore Parallel Implementations: If you’re up for a challenge, try implementing a parallel version of the algorithm.
Conclusion
The N-Queens problem is a fascinating challenge that combines elements of mathematics, computer science, and problem-solving. By mastering this problem, you’re not just preparing for coding interviews; you’re developing skills that are fundamental to tackling a wide range of complex algorithmic challenges.
Remember, the key to success with problems like N-Queens is practice and perseverance. Don’t be discouraged if you don’t grasp it immediately – each attempt brings you closer to mastery. Keep exploring, keep coding, and keep pushing your boundaries.
At AlgoCademy, we believe in the power of continuous learning and improvement. We encourage you to use our interactive coding platform to practice the N-Queens problem and other algorithmic challenges. Our AI-powered assistance and step-by-step guidance are here to support you on your journey from beginner to coding expert.
Happy coding, and may your queens never threaten each other!