Mastering the Minimum Window Substring Problem: A Comprehensive Guide
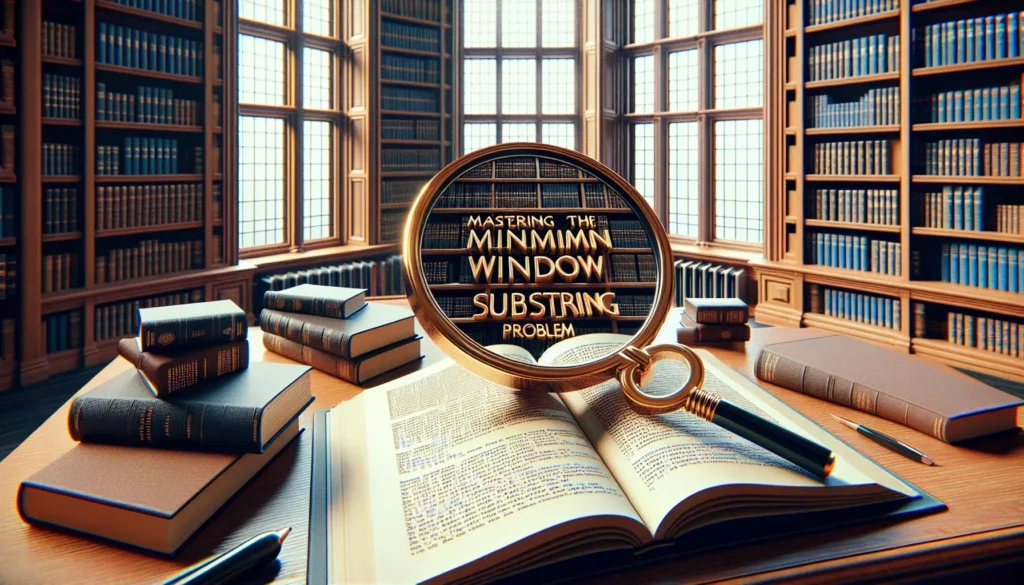
Welcome to another in-depth tutorial from AlgoCademy! Today, we’re diving into a classic algorithmic problem that frequently appears in technical interviews, especially those conducted by major tech companies. Our focus is on the “Minimum Window Substring” problem, a challenging yet fascinating puzzle that tests your string manipulation and sliding window technique skills.
Understanding the Problem
Before we delve into the solution, let’s clearly define the Minimum Window Substring problem:
Given two strings
s
andt
, return the minimum window substring ofs
such that every character int
(including duplicates) is included in the window. If there is no such substring, return the empty string""
.
In other words, we need to find the smallest substring in s
that contains all the characters of t
, maintaining their frequency.
Example:
Input: s = "ADOBECODEBANC", t = "ABC"
Output: "BANC"
Explanation: The minimum window substring "BANC" includes 'A', 'B', and 'C' from string t.
Breaking Down the Problem
To solve this problem efficiently, we’ll use the sliding window technique. Here’s a step-by-step breakdown of our approach:
- Create two hash maps (or frequency arrays) to keep track of character frequencies in
t
and the current window ins
. - Use two pointers,
left
andright
, to define the current window ins
. - Move the
right
pointer to expand the window until we have a valid window (contains all characters fromt
). - Once we have a valid window, move the
left
pointer to contract the window, updating the minimum window as we go. - Repeat steps 3 and 4 until we’ve processed the entire string
s
.
Implementing the Solution
Let’s implement this solution in Python. We’ll break it down into smaller functions for better readability and understanding.
Step 1: Creating the Character Frequency Maps
from collections import Counter
def create_t_frequency(t):
return Counter(t)
def create_window_frequency():
return Counter()
We use Python’s Counter
class from the collections
module to efficiently keep track of character frequencies.
Step 2: Checking if the Current Window is Valid
def is_window_valid(t_freq, window_freq):
for char, count in t_freq.items():
if window_freq[char] < count:
return False
return True
This function checks if the current window contains all characters from t
with their required frequencies.
Step 3: The Main Function
def min_window(s, t):
if not s or not t:
return ""
t_freq = create_t_frequency(t)
window_freq = create_window_frequency()
left = 0
min_length = float('inf')
min_window = ""
for right in range(len(s)):
# Expand the window
window_freq[s[right]] += 1
# Contract the window if it's valid
while is_window_valid(t_freq, window_freq):
if right - left + 1 < min_length:
min_length = right - left + 1
min_window = s[left:right+1]
window_freq[s[left]] -= 1
left += 1
return min_window
This is where we implement the sliding window technique. We expand the window by moving the right
pointer and contract it by moving the left
pointer when we have a valid window.
Time and Space Complexity Analysis
Let’s analyze the time and space complexity of our solution:
Time Complexity
The time complexity of this solution is O(|S| + |T|), where |S| and |T| are the lengths of strings s and t respectively.
- We iterate through the string
s
once with theright
pointer, which takes O(|S|) time. - For each iteration, we might move the
left
pointer, but theleft
pointer can move at most |S| times throughout the entire process. - Creating the frequency map for
t
takes O(|T|) time.
Space Complexity
The space complexity is O(K), where K is the number of unique characters in the string t
.
- We use two hash maps (or frequency arrays) to store character frequencies.
- In the worst case, if all characters in
t
are unique, we’ll need O(|T|) space. - However, since we’re dealing with strings, the number of possible characters is usually limited (e.g., 26 for lowercase English letters), so we can consider the space complexity as O(1) for most practical purposes.
Common Pitfalls and Edge Cases
When solving the Minimum Window Substring problem, be aware of these common pitfalls and edge cases:
- Empty Strings: Always check if either
s
ort
is empty at the beginning of your function. - Case Sensitivity: Clarify whether the problem is case-sensitive. ‘A’ and ‘a’ are treated as different characters unless specified otherwise.
- Duplicate Characters: Remember that
t
may contain duplicate characters, and your solution must account for their frequency. - No Valid Window: There might be cases where no valid window exists. Your function should return an empty string in such cases.
- Single Character Strings: Test your solution with single-character strings for both
s
andt
.
Optimizations and Variations
While our solution is already quite efficient, there are a few optimizations and variations we can consider:
1. Using an Array Instead of a Hash Map
If we know that the input strings only contain certain characters (e.g., lowercase English letters), we can use an array instead of a hash map for better performance:
def min_window_optimized(s, t):
def array_to_index(char):
return ord(char) - ord('a')
t_freq = [0] * 26
window_freq = [0] * 26
for char in t:
t_freq[array_to_index(char)] += 1
# Rest of the code remains similar, but use array indexing instead of dict access
# ...
2. Counting Required Characters
Instead of checking all characters in t_freq
every time, we can keep a count of how many unique characters we still need:
def min_window_with_count(s, t):
t_freq = Counter(t)
window_freq = Counter()
required = len(t_freq)
formed = 0
left = 0
min_length = float('inf')
min_window = ""
for right in range(len(s)):
window_freq[s[right]] += 1
if s[right] in t_freq and window_freq[s[right]] == t_freq[s[right]]:
formed += 1
while formed == required:
if right - left + 1 < min_length:
min_length = right - left + 1
min_window = s[left:right+1]
window_freq[s[left]] -= 1
if s[left] in t_freq and window_freq[s[left]] < t_freq[s[left]]:
formed -= 1
left += 1
return min_window
This optimization reduces the number of checks we need to perform when determining if a window is valid.
Related Problems and Techniques
The Minimum Window Substring problem is part of a family of problems that can be solved using the sliding window technique. Here are some related problems you might want to explore:
- Longest Substring Without Repeating Characters: Find the length of the longest substring without repeating characters.
- Longest Substring with At Most K Distinct Characters: Find the longest substring that contains at most K distinct characters.
- Substring with Concatenation of All Words: Find all starting indices of substring(s) in a given string that is a concatenation of each word in a given list exactly once.
- Smallest Range Covering Elements from K Lists: Find the smallest range that includes at least one number from each of the k lists.
These problems all involve manipulating strings or arrays and often require similar techniques such as sliding windows, two-pointer approaches, or hash maps for efficient solutions.
Practical Applications
Understanding and being able to solve the Minimum Window Substring problem isn’t just about acing technical interviews. This problem and the techniques used to solve it have practical applications in various areas of software development:
- Text Processing and Analysis: In natural language processing tasks, you might need to find the smallest segment of text that contains a specific set of words or phrases.
- Genetic Sequence Analysis: In bioinformatics, similar techniques can be used to find the shortest DNA sequence that contains all of a set of target sequences.
- Network Packet Analysis: When analyzing network traffic, you might need to find the smallest window of packets that contains all packets of interest.
- Data Streaming: In real-time data processing, you might need to find the smallest window of time that contains all events of interest.
- Image Processing: Similar concepts can be applied to find the smallest region in an image that contains all pixels of interest.
Conclusion
The Minimum Window Substring problem is a classic example of how seemingly complex problems can be solved efficiently with the right approach. By mastering this problem, you’re not only preparing yourself for technical interviews but also honing your problem-solving skills and algorithmic thinking.
Remember, the key to solving such problems is to break them down into smaller, manageable steps and to consider optimization techniques like the sliding window approach. Practice is crucial – try implementing the solution yourself, test it with various inputs, and experiment with the optimizations we discussed.
At AlgoCademy, we believe that understanding these fundamental algorithms and data structures is crucial for becoming a proficient programmer. Keep practicing, keep learning, and don’t hesitate to tackle more challenging problems. The skills you develop here will serve you well throughout your programming career.
Happy coding, and we’ll see you in the next tutorial!