Mastering the Middle Engineer Technical Interview: A Comprehensive Preparation Guide
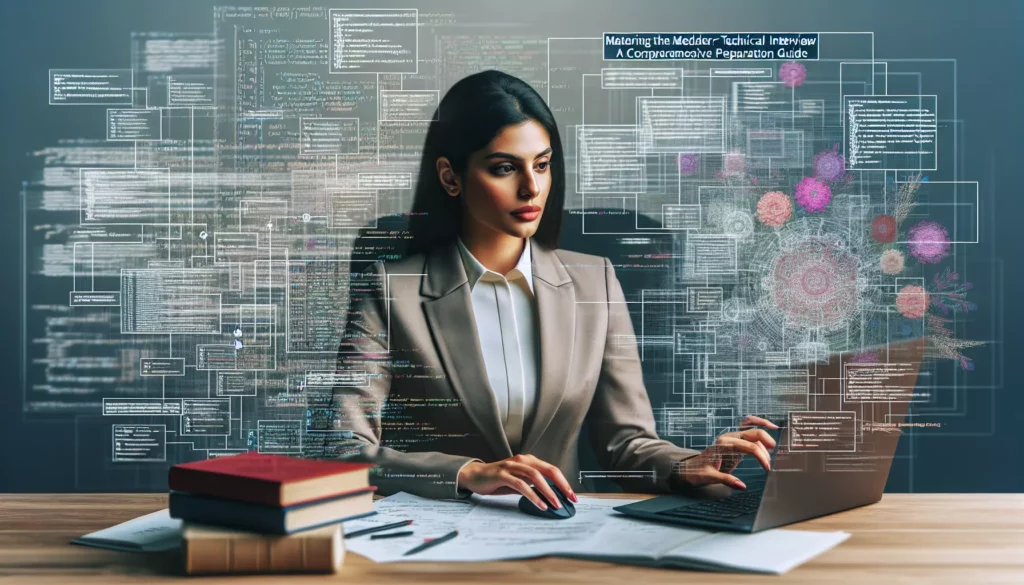
As the tech industry continues to evolve and grow, the demand for skilled software engineers remains high. For those looking to advance their careers and take on more challenging roles, the middle engineer position is an attractive next step. However, securing such a position often requires passing a rigorous technical interview process. In this comprehensive guide, we’ll explore the key areas to focus on and strategies to employ when preparing for a middle engineer technical interview.
Understanding the Middle Engineer Role
Before diving into interview preparation, it’s crucial to understand what companies are looking for in a middle engineer. Typically, a middle engineer is expected to:
- Have 3-5 years of professional software development experience
- Possess strong coding skills and a deep understanding of data structures and algorithms
- Be proficient in at least one programming language and familiar with others
- Understand software design principles and architectural patterns
- Have experience with version control systems, particularly Git
- Be able to write clean, maintainable, and efficient code
- Have good problem-solving and debugging skills
- Be familiar with Agile methodologies and software development lifecycle
With these expectations in mind, let’s break down the key areas to focus on during your interview preparation.
Core Technical Skills
1. Data Structures and Algorithms
A solid grasp of data structures and algorithms is fundamental for any software engineer. For a middle engineer position, you should be comfortable with:
- Arrays and Strings
- Linked Lists
- Stacks and Queues
- Trees and Graphs
- Hash Tables
- Heaps
- Sorting and Searching algorithms
- Dynamic Programming
- Greedy Algorithms
Practice implementing these data structures from scratch and solving problems that utilize them. Platforms like LeetCode, HackerRank, and AlgoExpert offer a wealth of practice problems to hone your skills.
2. Time and Space Complexity Analysis
Understanding the efficiency of your algorithms is crucial. Be prepared to analyze and discuss the time and space complexity of your solutions using Big O notation. This skill demonstrates your ability to write optimized code and make informed decisions about algorithm selection.
3. System Design
While system design questions are more common in senior engineer interviews, middle engineers should have a basic understanding of system architecture. Familiarize yourself with concepts such as:
- Client-server architecture
- Database design (SQL vs. NoSQL)
- Caching mechanisms
- Load balancing
- Microservices architecture
- RESTful API design
Practice explaining these concepts and how you would apply them in real-world scenarios.
Programming Languages and Frameworks
As a middle engineer, you should have deep knowledge of at least one programming language and be familiar with its ecosystem. Common languages for technical interviews include:
- Java
- Python
- JavaScript
- C++
- Go
Be prepared to discuss the strengths and weaknesses of your chosen language, as well as demonstrate proficiency in writing idiomatic code. Additionally, familiarize yourself with popular frameworks and libraries in your language’s ecosystem.
Example: Java Proficiency
If you’re interviewing for a Java position, you should be comfortable with:
- Core Java concepts (OOP, Collections, Generics, etc.)
- Java 8+ features (Lambdas, Streams, Optional, etc.)
- Multithreading and concurrency
- Spring Framework
- JUnit for testing
- Build tools like Maven or Gradle
Coding Best Practices
Writing clean, maintainable code is a key expectation for middle engineers. During your interview, demonstrate your commitment to best practices by:
- Writing modular and reusable code
- Using meaningful variable and function names
- Adding appropriate comments and documentation
- Handling edge cases and errors gracefully
- Following the SOLID principles of object-oriented design
- Writing unit tests for your code
Problem-Solving Approach
Your problem-solving approach is just as important as the final solution. Interviewers want to see how you think and tackle challenges. Follow these steps when solving problems during your interview:
- Clarify the problem: Ask questions to ensure you understand the requirements and constraints.
- Discuss potential approaches: Before diving into coding, talk through different strategies to solve the problem.
- Choose an approach: Select the most appropriate solution based on time/space complexity and other factors.
- Write pseudocode: Outline your solution before writing actual code.
- Implement the solution: Write clean, efficient code to solve the problem.
- Test your solution: Walk through your code with sample inputs, including edge cases.
- Optimize: Discuss potential optimizations or alternative approaches.
Remember to think out loud during this process, as it gives the interviewer insight into your thought process and problem-solving skills.
Behavioral Questions
While technical skills are crucial, companies also value soft skills and cultural fit. Be prepared to answer behavioral questions that assess your:
- Teamwork and collaboration abilities
- Communication skills
- Conflict resolution strategies
- Leadership potential
- Ability to give and receive feedback
- Adaptability and learning agility
Use the STAR method (Situation, Task, Action, Result) to structure your responses to behavioral questions, providing concrete examples from your past experiences.
Mock Interviews and Practice
The best way to prepare for a technical interview is through consistent practice. Consider the following strategies:
- Conduct mock interviews with friends, colleagues, or online platforms
- Time yourself when solving coding problems to simulate interview conditions
- Practice explaining your thought process out loud as you solve problems
- Record yourself during mock interviews to identify areas for improvement
- Join coding communities or study groups for peer support and practice
Company-Specific Preparation
Each company has its own culture, tech stack, and interview process. Research the company you’re interviewing with and tailor your preparation accordingly:
- Review the company’s products, services, and recent news
- Understand their tech stack and any specific technologies they use
- Read reviews on sites like Glassdoor to get insights into their interview process
- Prepare questions to ask your interviewers about the company and role
Common Interview Questions for Middle Engineers
While every interview is unique, here are some common types of questions you might encounter:
1. Algorithm and Data Structure Questions
Example: Implement a function to find the longest palindromic substring in a given string.
public String longestPalindrome(String s) {
if (s == null || s.length() < 2) {
return s;
}
int start = 0, maxLength = 1;
for (int i = 0; i < s.length(); i++) {
int len1 = expandAroundCenter(s, i, i);
int len2 = expandAroundCenter(s, i, i + 1);
int len = Math.max(len1, len2);
if (len > maxLength) {
start = i - (len - 1) / 2;
maxLength = len;
}
}
return s.substring(start, start + maxLength);
}
private int expandAroundCenter(String s, int left, int right) {
while (left >= 0 && right < s.length() && s.charAt(left) == s.charAt(right)) {
left--;
right++;
}
return right - left - 1;
}
2. System Design Questions
Example: Design a URL shortening service like bit.ly.
Key points to discuss:
- Hash function to generate short URLs
- Database schema for storing mappings
- Caching strategy for frequently accessed URLs
- Load balancing for high traffic
- Analytics and tracking features
3. Coding Best Practices
Example: Refactor the following code to improve its readability and maintainability:
public static void p(int[] a) {
for(int i=0;i<a.length;i++) {
for(int j=i+1;j<a.length;j++) {
if(a[i]>a[j]) {
int t=a[i];
a[i]=a[j];
a[j]=t;
}
}
}
for(int i=0;i<a.length;i++)System.out.print(a[i]+" ");
}
Refactored version:
public static void sortAndPrintArray(int[] array) {
bubbleSort(array);
printArray(array);
}
private static void bubbleSort(int[] array) {
for (int i = 0; i < array.length - 1; i++) {
for (int j = 0; j < array.length - i - 1; j++) {
if (array[j] > array[j + 1]) {
swap(array, j, j + 1);
}
}
}
}
private static void swap(int[] array, int i, int j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
}
private static void printArray(int[] array) {
for (int element : array) {
System.out.print(element + " ");
}
System.out.println();
}
4. Language-Specific Questions
Example (Java): Explain the differences between ArrayList and LinkedList. When would you choose one over the other?
Key points to discuss:
- Underlying data structures (dynamic array vs. doubly-linked list)
- Time complexity for common operations (get, add, remove)
- Memory usage
- Use cases for each (frequent access vs. frequent insertions/deletions)
5. Behavioral Questions
Example: Describe a time when you had to work on a project with a tight deadline. How did you manage your time and ensure the project was completed successfully?
Use the STAR method to structure your response:
- Situation: Describe the project and the tight deadline
- Task: Explain your role and responsibilities
- Action: Detail the steps you took to manage time and ensure completion
- Result: Share the outcome and any lessons learned
Interview Day Tips
On the day of your interview, keep these tips in mind:
- Get a good night’s sleep and eat a healthy meal before the interview
- Arrive early or ensure your virtual setup is ready well in advance
- Bring a notebook and pen for in-person interviews
- Stay calm and take deep breaths if you feel nervous
- Listen carefully to the questions and ask for clarification if needed
- Be honest about what you know and don’t know
- Show enthusiasm for the role and company
- Thank the interviewer for their time at the end of the interview
Post-Interview Follow-Up
After the interview:
- Send a thank-you email within 24 hours, reiterating your interest in the position
- Reflect on the interview experience and note areas for improvement
- Follow up with the recruiter if you haven’t heard back within the specified timeframe
Conclusion
Preparing for a middle engineer technical interview requires dedication, practice, and a well-rounded approach. By focusing on core technical skills, problem-solving abilities, and soft skills, you’ll be well-equipped to tackle the challenges of the interview process. Remember that interviewing is a skill in itself, and like any skill, it improves with practice. Stay persistent, learn from each interview experience, and continue to grow as a developer. With thorough preparation and the right mindset, you’ll be well on your way to securing that middle engineer position and advancing your career in the tech industry.
Good luck with your interview preparation, and may your hard work lead you to success in your next role as a middle engineer!