Mastering the Longest Palindromic Substring Problem: A Comprehensive Guide
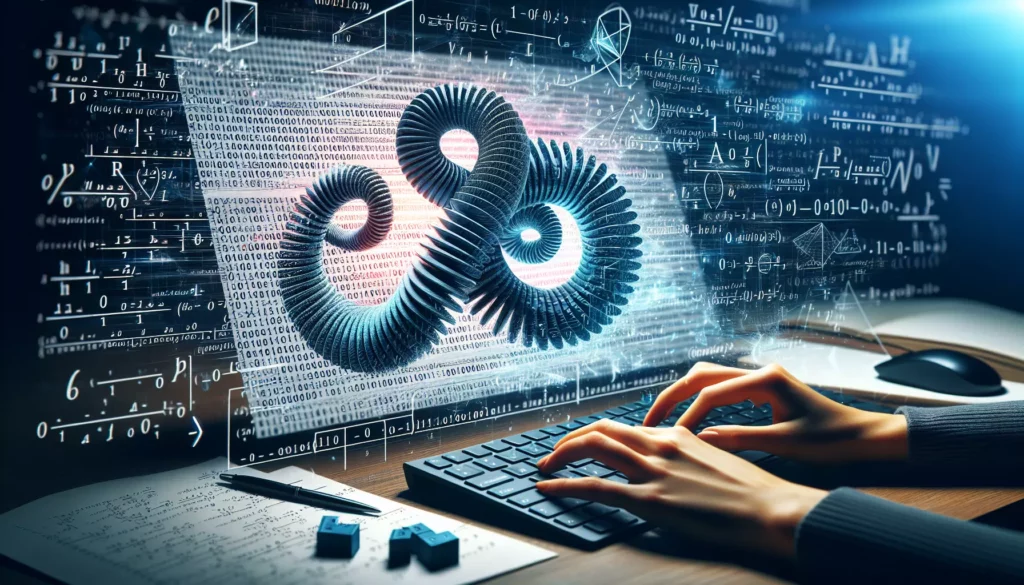
Welcome to AlgoCademy’s in-depth exploration of the Longest Palindromic Substring problem! This classic algorithmic challenge is a favorite among interviewers at top tech companies and is an excellent exercise for honing your problem-solving skills. In this comprehensive guide, we’ll dive deep into the problem, explore multiple solutions, and provide you with the tools you need to tackle this challenge confidently in your next coding interview.
Table of Contents
- Introduction to Palindromes and the Problem Statement
- The Naive Approach: Brute Force Solution
- Dynamic Programming: Optimizing with Tabulation
- Manacher’s Algorithm: The Optimal Solution
- Implementing the Solutions in Python
- Time and Space Complexity Analysis
- Interview Tips and Common Pitfalls
- Related Practice Problems
- Conclusion and Next Steps
1. Introduction to Palindromes and the Problem Statement
Before we dive into the solutions, let’s ensure we have a solid understanding of palindromes and the problem at hand.
What is a Palindrome?
A palindrome is a sequence of characters that reads the same forward and backward. For example:
- “racecar” is a palindrome
- “level” is a palindrome
- “A man, a plan, a canal: Panama” is a palindrome (ignoring spaces and punctuation)
The Longest Palindromic Substring Problem
Given a string s
, find the longest substring that is a palindrome. If there are multiple such substrings with the same length, return any one of them.
For example:
- Input:
s = "babad"
- Output:
"bab"
or"aba"
(both are valid answers)
This problem tests your ability to work with strings, recognize patterns, and optimize your solution for efficiency. It’s a common question in technical interviews because it can be solved using various approaches, each with different time and space complexities.
2. The Naive Approach: Brute Force Solution
Let’s start with the most straightforward approach to solving this problem: the brute force method. While this may not be the most efficient solution, it’s important to understand as it forms the basis for more optimized approaches.
The Algorithm
- Generate all possible substrings of the given string.
- Check if each substring is a palindrome.
- Keep track of the longest palindromic substring found.
- Return the longest palindromic substring.
Python Implementation
def longestPalindrome(s: str) -> str:
def isPalindrome(sub: str) -> bool:
return sub == sub[::-1]
n = len(s)
longest = ""
for i in range(n):
for j in range(i, n):
substring = s[i:j+1]
if isPalindrome(substring) and len(substring) > len(longest):
longest = substring
return longest
This solution works, but it’s not efficient for large inputs. Let’s analyze its time complexity:
- Generating all substrings takes O(n^2) time, where n is the length of the string.
- Checking if each substring is a palindrome takes O(n) time.
- The total time complexity is O(n^3).
While this approach is intuitive and easy to implement, it’s not suitable for large-scale problems or competitive programming scenarios. However, understanding this solution is crucial as it helps in developing more optimized approaches.
3. Dynamic Programming: Optimizing with Tabulation
Now that we understand the brute force approach, let’s explore a more efficient solution using dynamic programming. This method significantly reduces the time complexity by avoiding redundant computations.
The Dynamic Programming Approach
The key idea behind the dynamic programming solution is to build a table (2D array) where dp[i][j]
represents whether the substring s[i:j+1]
is a palindrome or not. We can fill this table using the following logic:
- All substrings of length 1 are palindromes.
- For substrings of length 2, check if both characters are the same.
- For substrings of length 3 or more, check if the first and last characters are the same, and if the substring between them is a palindrome.
Python Implementation
def longestPalindrome(s: str) -> str:
n = len(s)
dp = [[False] * n for _ in range(n)]
longest = s[0]
# All substrings of length 1 are palindromes
for i in range(n):
dp[i][i] = True
# Check for substrings of length 2
for i in range(n - 1):
if s[i] == s[i + 1]:
dp[i][i + 1] = True
longest = s[i:i + 2]
# Check for substrings of length 3 or more
for length in range(3, n + 1):
for i in range(n - length + 1):
j = i + length - 1
if s[i] == s[j] and dp[i + 1][j - 1]:
dp[i][j] = True
if length > len(longest):
longest = s[i:j + 1]
return longest
Time and Space Complexity
- Time Complexity: O(n^2), where n is the length of the string.
- Space Complexity: O(n^2) to store the dp table.
This dynamic programming solution is a significant improvement over the brute force approach, especially for larger inputs. It achieves this by storing and reusing intermediate results, avoiding redundant calculations.
4. Manacher’s Algorithm: The Optimal Solution
While the dynamic programming solution is efficient, there’s an even more optimized algorithm for solving the Longest Palindromic Substring problem: Manacher’s Algorithm. This algorithm achieves linear time complexity, making it the most efficient known solution to this problem.
Understanding Manacher’s Algorithm
Manacher’s Algorithm works by transforming the original string and using previously computed results to avoid unnecessary computations. Here’s a high-level overview of how it works:
- Transform the string by inserting special characters (e.g., ‘#’) between each character and at the start and end. This handles both odd and even length palindromes uniformly.
- Maintain an array P where P[i] represents the radius of the palindrome centered at index i in the transformed string.
- Use two variables: C (center of the current palindrome) and R (right boundary of the current palindrome).
- Iterate through the string, updating C and R, and computing P[i] for each position.
- Use previously computed values to avoid redundant checks when possible.
Python Implementation of Manacher’s Algorithm
def longestPalindrome(s: str) -> str:
# Transform the string
T = '#' + '#'.join(s) + '#'
n = len(T)
P = [0] * n
C = R = 0
for i in range(n):
mirror = 2 * C - i
if i < R:
P[i] = min(R - i, P[mirror])
# Attempt to expand palindrome centered at i
while i + (1 + P[i]) < n and i - (1 + P[i]) >= 0 and T[i + (1 + P[i])] == T[i - (1 + P[i])]:
P[i] += 1
# If palindrome centered at i expands past R,
# adjust center based on expanded palindrome.
if i + P[i] > R:
C, R = i, i + P[i]
# Find the maximum element in P
max_len, center_index = max((n, i) for i, n in enumerate(P))
start = (center_index - max_len) // 2
return s[start: start + max_len]
Time and Space Complexity
- Time Complexity: O(n), where n is the length of the string.
- Space Complexity: O(n) to store the transformed string and the P array.
Manacher’s Algorithm achieves linear time complexity, making it the most efficient solution for the Longest Palindromic Substring problem. However, it’s important to note that while this algorithm is theoretically optimal, the implementation can be complex and may not always be the expected solution in an interview setting.
5. Implementing the Solutions in Python
Now that we’ve explored different approaches to solving the Longest Palindromic Substring problem, let’s dive deeper into the implementation details and best practices for coding these solutions in Python.
Best Practices for Implementation
- Use Type Hints: Python 3.5+ supports type hinting, which can make your code more readable and catch potential type-related errors early.
- Write Clear Comments: Explain complex parts of your algorithm, especially for approaches like Manacher’s Algorithm.
- Use Descriptive Variable Names: Choose names that clearly convey the purpose of each variable.
- Handle Edge Cases: Consider empty strings or single-character inputs in your implementation.
- Follow PEP 8: Adhere to Python’s style guide for consistent and readable code.
Implementing the Dynamic Programming Solution
Let’s revisit the dynamic programming solution with these best practices in mind:
from typing import List
def longest_palindrome(s: str) -> str:
if not s:
return ""
n: int = len(s)
dp: List[List[bool]] = [[False] * n for _ in range(n)]
start: int = 0
max_length: int = 1
# All substrings of length 1 are palindromes
for i in range(n):
dp[i][i] = True
# Check for substrings of length 2
for i in range(n - 1):
if s[i] == s[i + 1]:
dp[i][i + 1] = True
start = i
max_length = 2
# Check for substrings of length 3 or more
for length in range(3, n + 1):
for i in range(n - length + 1):
j: int = i + length - 1
if s[i] == s[j] and dp[i + 1][j - 1]:
dp[i][j] = True
if length > max_length:
start = i
max_length = length
return s[start:start + max_length]
This implementation includes type hints, clear variable names, and handles the edge case of an empty string. The logic remains the same as before, but the code is now more readable and maintainable.
Testing Your Implementation
It’s crucial to test your implementation with various input cases. Here’s a simple test suite you can use:
def test_longest_palindrome():
assert longest_palindrome("babad") in ["bab", "aba"]
assert longest_palindrome("cbbd") == "bb"
assert longest_palindrome("a") == "a"
assert longest_palindrome("") == ""
assert longest_palindrome("abcdefghijklmnopqrstuvwxyz") in list("abcdefghijklmnopqrstuvwxyz")
print("All tests passed!")
test_longest_palindrome()
This test suite covers various scenarios, including edge cases and strings with no palindromes longer than one character.
6. Time and Space Complexity Analysis
Understanding the time and space complexity of different solutions is crucial for choosing the right approach in various scenarios. Let’s analyze the complexity of each method we’ve discussed:
Brute Force Approach
- Time Complexity: O(n^3)
- Generating all substrings: O(n^2)
- Checking each substring for palindrome: O(n)
- Space Complexity: O(1) or O(n) if we consider the space needed to store the result
Dynamic Programming Approach
- Time Complexity: O(n^2)
- We fill a 2D table of size n x n
- Space Complexity: O(n^2)
- We use a 2D table of size n x n to store intermediate results
Manacher’s Algorithm
- Time Complexity: O(n)
- The algorithm processes each character only a constant number of times
- Space Complexity: O(n)
- We use additional arrays of length proportional to n
Choosing the Right Approach
The choice of algorithm depends on various factors:
- Input Size: For very small inputs, the brute force method might be sufficient.
- Time Constraints: If efficiency is crucial, Manacher’s Algorithm is the best choice.
- Space Constraints: If memory is limited, the brute force method uses the least space.
- Code Complexity: The dynamic programming solution offers a good balance between efficiency and implementation simplicity.
In most interview scenarios, implementing the dynamic programming solution would be the expected approach, as it demonstrates a good understanding of optimization techniques without the complexity of Manacher’s Algorithm.
7. Interview Tips and Common Pitfalls
When tackling the Longest Palindromic Substring problem in an interview setting, keep these tips in mind to maximize your chances of success:
Interview Tips
- Start with the Brute Force: Begin by explaining the naive approach. This shows you can think through the problem systematically.
- Analyze and Optimize: Discuss the time and space complexity of the brute force method, then propose optimizations.
- Explain Your Thought Process: Verbalize your thinking as you work through the problem. Interviewers are often more interested in your problem-solving approach than the final solution.
- Consider Edge Cases: Discuss how your solution handles empty strings, single-character strings, and strings with no palindromes longer than one character.
- Code Clearly: Write clean, well-commented code. Use meaningful variable names and follow standard coding conventions.
- Test Your Solution: After implementing, walk through a few test cases to verify your solution works correctly.
Common Pitfalls to Avoid
- Overlooking Even-Length Palindromes: Ensure your solution handles both odd and even-length palindromes correctly.
- Inefficient Palindrome Checking: In the brute force approach, checking each substring for palindrome property can be optimized.
- Incorrect Dynamic Programming State: When using DP, make sure your state transition is correct and covers all cases.
- Off-by-One Errors: Be careful with string indices, especially when extracting substrings.
- Assuming ASCII Characters: Unless specified, don’t assume the input string only contains ASCII characters. Your solution should work for Unicode strings as well.
- Ignoring Space Complexity: While focusing on time complexity, don’t forget to consider and optimize space usage where possible.
Follow-up Questions to Prepare For
Interviewers might ask these follow-up questions to test your understanding:
- How would you modify your solution to return all longest palindromic substrings if there are multiple?
- Can you optimize your solution to use O(1) space?
- How would you handle very large strings that don’t fit into memory?
- Can you extend your solution to find the longest palindromic subsequence instead of substring?
Being prepared for these questions demonstrates a deeper understanding of the problem and related concepts.
8. Related Practice Problems
To further strengthen your skills in handling string manipulation and dynamic programming problems, consider practicing these related problems:
- Palindromic Substrings: Count the number of palindromic substrings in a given string.
- Longest Palindromic Subsequence: Find the length of the longest palindromic subsequence in a string.
- Shortest Palindrome: Find the shortest palindrome you can form by adding characters in front of a given string.
- Palindrome Partitioning: Partition a string such that every substring of the partition is a palindrome.
- Longest Common Substring: Find the longest string which is a substring of two or more strings.
These problems will help you apply the concepts and techniques you’ve learned in different contexts, enhancing your problem-solving skills and preparing you for a wider range of interview questions.
9. Conclusion and Next Steps
Congratulations on making it through this comprehensive guide on the Longest Palindromic Substring problem! You’ve learned about various approaches to solve this classic algorithmic challenge, from the intuitive brute force method to the highly optimized Manacher’s Algorithm.
Key Takeaways
- Understanding the problem and its variations is crucial for developing effective solutions.
- The brute force approach, while intuitive, is often too slow for large inputs.
- Dynamic programming offers a good balance between efficiency and implementation complexity.
- Manacher’s Algorithm provides the optimal time complexity but may be overly complex for many scenarios.
- Analyzing time and space complexity is essential for choosing the right approach.
- Practice and preparation are key to successfully tackling this problem in interview settings.
Next Steps
To continue improving your algorithmic skills and prepare for technical interviews, consider the following steps:
- Practice Regularly: Solve related problems on platforms like LeetCode, HackerRank, or CodeSignal.
- Study Other String Algorithms: Explore KMP, Rabin-Karp, and other string matching algorithms.
- Dive Deeper into Dynamic Programming: Master other DP problems to strengthen your problem-solving skills.
- Mock Interviews: Participate in mock interviews to practice explaining your thought process and coding under pressure.
- Review Data Structures: Ensure you have a solid understanding of fundamental data structures, as they often play a crucial role in optimizing solutions.
- Explore Advanced Topics: Look into more advanced string algorithms and their applications in real-world scenarios.
Remember, becoming proficient in algorithmic problem-solving is a journey. Consistent practice, a willingness to learn from mistakes, and exposure to a variety of problems will help you build the skills and confidence needed to excel in technical interviews.
Keep coding, stay curious, and happy problem-solving!